PxMassProperties Class Reference
[Extensions]
Utility class to compute and manipulate mass and inertia tensor properties.
More...
#include <PxMassProperties.h>
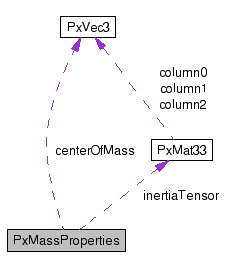
Public Member Functions | |
PX_FORCE_INLINE | PxMassProperties () |
Default constructor. | |
PX_FORCE_INLINE | PxMassProperties (const PxReal m, const PxMat33 &inertiaT, const PxVec3 &com) |
Construct from individual elements. | |
PxMassProperties (const PxGeometry &geometry) | |
Compute mass properties based on a provided geometry structure. | |
PX_FORCE_INLINE PxMassProperties | operator* (const PxReal scale) const |
Scale mass properties. | |
PX_FORCE_INLINE void | translate (const PxVec3 &t) |
Translate the center of mass by a given vector and adjust the inertia tensor accordingly. | |
Static Public Member Functions | |
static PX_FORCE_INLINE PxVec3 | getMassSpaceInertia (const PxMat33 &inertia, PxQuat &massFrame) |
Get the entries of the diagonalized inertia tensor and the corresponding reference rotation. | |
static PX_FORCE_INLINE PxMat33 | translateInertia (const PxMat33 &inertia, const PxReal mass, const PxVec3 &t) |
Translate an inertia tensor using the parallel axis theorem. | |
static PX_FORCE_INLINE PxMat33 | rotateInertia (const PxMat33 &inertia, const PxQuat &q) |
Rotate an inertia tensor around the center of mass. | |
static PxMat33 | scaleInertia (const PxMat33 &inertia, const PxQuat &scaleRotation, const PxVec3 &scale) |
Non-uniform scaling of the inertia tensor. | |
static PxMassProperties | sum (const PxMassProperties *props, const PxTransform *transforms, const PxU32 count) |
Sum up individual mass properties. | |
Public Attributes | |
PxMat33 | inertiaTensor |
The inertia tensor of the object. | |
PxVec3 | centerOfMass |
The center of mass of the object. | |
PxReal | mass |
The mass of the object. |
Detailed Description
Utility class to compute and manipulate mass and inertia tensor properties.In most cases PxRigidBodyExt::updateMassAndInertia(), PxRigidBodyExt::setMassAndUpdateInertia() should be enough to setup the mass properties of a rigid body. This utility class targets users that need to customize the mass properties computation.
Constructor & Destructor Documentation
PX_FORCE_INLINE PxMassProperties::PxMassProperties | ( | ) | [inline] |
Default constructor.
PX_FORCE_INLINE PxMassProperties::PxMassProperties | ( | const PxReal | m, | |
const PxMat33 & | inertiaT, | |||
const PxVec3 & | com | |||
) | [inline] |
Construct from individual elements.
PxMassProperties::PxMassProperties | ( | const PxGeometry & | geometry | ) | [inline] |
Compute mass properties based on a provided geometry structure.
This constructor assumes the geometry has a density of 1. Mass and inertia tensor scale linearly with density.
- Parameters:
-
[in] geometry The geometry to compute the mass properties for. Supported geometry types are: sphere, box, capsule and convex mesh.
References PxConvexMeshGeometry::convexMesh, PxMat33::createDiagonal(), PxGeometryType::eBOX, PxGeometryType::eCAPSULE, PxGeometryType::eCONVEXMESH, PxGeometryType::eGEOMETRY_COUNT, PxGeometryType::eHEIGHTFIELD, PxGeometryType::eINVALID, PxGeometryType::ePLANE, PxGeometryType::eSPHERE, PxGeometryType::eTRIANGLEMESH, PxConvexMesh::getMassInformation(), PxGeometry::getType(), PxBoxGeometry::halfExtents, PxCapsuleGeometry::halfHeight, PxVec3::multiply(), PX_ASSERT, PxIsFinite(), PxPi, PxCapsuleGeometry::radius, PxSphereGeometry::radius, PxQuat::rotate(), PxQuat::rotateInv(), PxMeshScale::rotation, PxMeshScale::scale, PxConvexMeshGeometry::scale, PxVec3::x, PxVec3::y, and PxVec3::z.
Member Function Documentation
static PX_FORCE_INLINE PxVec3 PxMassProperties::getMassSpaceInertia | ( | const PxMat33 & | inertia, | |
PxQuat & | massFrame | |||
) | [inline, static] |
Get the entries of the diagonalized inertia tensor and the corresponding reference rotation.
- Parameters:
-
[in] inertia The inertia tensor to diagonalize. [out] massFrame The frame the diagonalized tensor refers to.
- Returns:
- The entries of the diagonalized inertia tensor.
References PxMat33::column0, PxMat33::column1, PxMat33::column2, PxQuat::isFinite(), PxVec3::isFinite(), PX_ASSERT, and PxDiagonalize().
PX_FORCE_INLINE PxMassProperties PxMassProperties::operator* | ( | const PxReal | scale | ) | const [inline] |
Scale mass properties.
- Parameters:
-
[in] scale The linear scaling factor to apply to the mass properties.
- Returns:
- The scaled mass properties.
References PX_ASSERT, and PxIsFinite().
static PX_FORCE_INLINE PxMat33 PxMassProperties::rotateInertia | ( | const PxMat33 & | inertia, | |
const PxQuat & | q | |||
) | [inline, static] |
Rotate an inertia tensor around the center of mass.
- Parameters:
-
[in] inertia The inertia tensor to rotate. [in] q The rotation to apply to the inertia tensor.
- Returns:
- The rotated inertia tensor.
References PxMat33::column0, PxMat33::column1, PxMat33::column2, PxMat33::getTranspose(), PxVec3::isFinite(), PxQuat::isUnit(), and PX_ASSERT.
static PxMat33 PxMassProperties::scaleInertia | ( | const PxMat33 & | inertia, | |
const PxQuat & | scaleRotation, | |||
const PxVec3 & | scale | |||
) | [inline, static] |
Non-uniform scaling of the inertia tensor.
- Parameters:
-
[in] inertia The inertia tensor to scale. [in] scaleRotation The frame of the provided scaling factors. [in] scale The scaling factor for each axis (relative to the frame specified in scaleRotation).
- Returns:
- The scaled inertia tensor.
References PxMat33::column0, PxMat33::column1, PxMat33::column2, PxVec3::dot(), PxQuat::getConjugate(), PxVec3::isFinite(), PxQuat::isUnit(), PxVec3::multiply(), PX_ASSERT, PxVec3::x, PxVec3::y, and PxVec3::z.
static PxMassProperties PxMassProperties::sum | ( | const PxMassProperties * | props, | |
const PxTransform * | transforms, | |||
const PxU32 | count | |||
) | [inline, static] |
Sum up individual mass properties.
- Parameters:
-
[in] props Array of mass properties to sum up. [in] transforms Reference transforms for each mass properties entry. [in] count The number of mass properties to sum up.
- Returns:
- The summed up mass properties.
References PxMat33::column0, PxMat33::column1, PxMat33::column2, inertiaTensor, PxVec3::isFinite(), mass, PX_ASSERT, PxIsFinite(), PxZero, and PxTransform::transform().
PX_FORCE_INLINE void PxMassProperties::translate | ( | const PxVec3 & | t | ) | [inline] |
Translate the center of mass by a given vector and adjust the inertia tensor accordingly.
- Parameters:
-
[in] t The translation vector for the center of mass.
References PxVec3::isFinite(), and PX_ASSERT.
static PX_FORCE_INLINE PxMat33 PxMassProperties::translateInertia | ( | const PxMat33 & | inertia, | |
const PxReal | mass, | |||
const PxVec3 & | t | |||
) | [inline, static] |
Translate an inertia tensor using the parallel axis theorem.
- Parameters:
-
[in] inertia The inertia tensor to translate. [in] mass The mass of the object. [in] t The relative frame to translate the inertia tensor to.
- Returns:
- The translated inertia tensor.
References PxMat33::column0, PxMat33::column1, PxMat33::column2, PxVec3::isFinite(), PX_ASSERT, PxIsFinite(), PxVec3::x, PxVec3::y, and PxVec3::z.
Member Data Documentation
The center of mass of the object.
PxReal PxMassProperties::mass |
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com