PxCloth Class Reference
[Cloth]
Set of connected particles tailored towards simulating character cloth.
More...
#include <PxCloth.h>
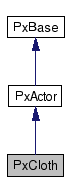
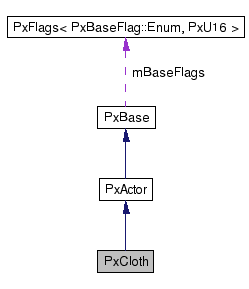
Public Member Functions | |
virtual void | release ()=0 |
Deletes the cloth. Do not keep a reference to the deleted instance. | |
virtual PxClothFabric * | getFabric () const =0 |
Returns a pointer to the corresponding cloth fabric. | |
virtual PxBounds3 | getWorldBounds (float inflation=1.01f) const =0 |
Returns world space bounding box. | |
virtual PxU32 | getNbParticles () const =0 |
Returns the number of particles. | |
virtual PxClothParticleData * | lockParticleData (PxDataAccessFlags flags)=0 |
Acquires access to the cloth particle data. | |
virtual PxClothParticleData * | lockParticleData () const =0 |
Acquires read access to the cloth particle data. | |
virtual void | setParticles (const PxClothParticle *currentParticles, const PxClothParticle *previousParticles)=0 |
Updates cloth particle location or inverse weight for current and previous particle state. | |
virtual void | setClothFlag (PxClothFlag::Enum flag, bool value)=0 |
Sets cloth flags (e.g. use GPU for simulation, enable CCD, collide against scene). | |
virtual void | setClothFlags (PxClothFlags inFlags)=0 |
Set all cloth flags. | |
virtual PxClothFlags | getClothFlags () const =0 |
Returns cloth flags. | |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
Integration | |
Functions related to particle integration. | |
virtual void | setTargetPose (const PxTransform &pose)=0 |
Sets pose that the cloth should move to by the end of the next simulate() call. | |
virtual void | setGlobalPose (const PxTransform &pose)=0 |
Sets current pose of the cloth without affecting inertia. | |
virtual PxTransform | getGlobalPose () const =0 |
Returns global pose. | |
virtual void | setSolverFrequency (PxReal frequency)=0 |
Sets the solver frequency parameter. | |
virtual PxReal | getSolverFrequency () const =0 |
Returns solver frequency. | |
virtual PxReal | getPreviousTimeStep () const =0 |
Returns previous time step size. | |
virtual void | setStiffnessFrequency (PxReal frequency)=0 |
Sets the stiffness frequency parameter. | |
virtual PxReal | getStiffnessFrequency () const =0 |
Returns stiffness frequency. | |
virtual void | setLinearInertiaScale (PxVec3 scale)=0 |
Sets the acceleration scale factor to adjust inertia effect from global pose changes. | |
virtual PxVec3 | getLinearInertiaScale () const =0 |
Returns linear acceleration scale parameter. | |
virtual void | setAngularInertiaScale (PxVec3 scale)=0 |
Sets the acceleration scale factor to adjust inertia effect from global pose changes. | |
virtual PxVec3 | getAngularInertiaScale () const =0 |
Returns angular acceleration scale parameter. | |
virtual void | setCentrifugalInertiaScale (PxVec3 scale)=0 |
Sets the acceleration scale factor to adjust inertia effect from global pose changes. | |
virtual PxVec3 | getCentrifugalInertiaScale () const =0 |
Returns centrifugal acceleration scale parameter. | |
virtual void | setInertiaScale (PxReal scale)=0 |
Same as setLinearInertiaScale(PxVec3(scale)); setAngularInertiaScale(PxVec3(scale)); getCentrifugalInertiaScale(PxVec3(scale)); . | |
virtual void | setDampingCoefficient (PxVec3 dampingCoefficient)=0 |
Sets the damping coefficient. | |
virtual PxVec3 | getDampingCoefficient () const =0 |
Returns the damping coefficient. | |
virtual void | setLinearDragCoefficient (PxVec3 dragCoefficient)=0 |
Sets the linear drag coefficient. | |
virtual PxVec3 | getLinearDragCoefficient () const =0 |
Returns the linear drag coefficient. | |
virtual void | setAngularDragCoefficient (PxVec3 dragCoefficient)=0 |
Sets the angular drag coefficient. | |
virtual PxVec3 | getAngularDragCoefficient () const =0 |
Returns the angular drag coefficient. | |
virtual void | setDragCoefficient (PxReal scale)=0 |
Same as setLinearDragCoefficient(PxVec3(coefficient)); setAngularDragCoefficient(PxVec3(coefficient)); . | |
virtual void | setExternalAcceleration (PxVec3 acceleration)=0 |
Sets external particle accelerations. | |
virtual PxVec3 | getExternalAcceleration () const =0 |
Returns external acceleration. | |
virtual void | setParticleAccelerations (const PxVec4 *particleAccelerations)=0 |
Updates particle accelerations, w component is ignored. | |
virtual bool | getParticleAccelerations (PxVec4 *particleAccelerationsBuffer) const =0 |
Copies particle accelerations to the user provided buffer. | |
virtual PxU32 | getNbParticleAccelerations () const =0 |
Returns the number of particle accelerations. | |
virtual PxVec3 | getWindVelocity () const =0 |
Returns the velocity of the wind affecting the fabric's triangles. | |
virtual void | setWindVelocity (PxVec3)=0 |
Sets the velocity of the wind affecting the fabric's triangles. | |
virtual PxReal | getWindDrag () const =0 |
Returns the coefficient of the air drag on the fabric's triangles. | |
virtual void | setWindDrag (PxReal)=0 |
Sets the coefficient of the air drag on the fabric's triangles. | |
virtual PxReal | getWindLift () const =0 |
Returns the coefficient of the air lift on the fabric's triangles. | |
virtual void | setWindLift (PxReal)=0 |
Sets the coefficient of the air lift on the fabric's triangles. | |
Constraints | |
Functions related to particle and distance constaints. | |
virtual void | setMotionConstraints (const PxClothParticleMotionConstraint *motionConstraints)=0 |
Updates motion constraints (position and radius of the constraint sphere). | |
virtual bool | getMotionConstraints (PxClothParticleMotionConstraint *motionConstraintsBuffer) const =0 |
Copies motion constraints to the user provided buffer. | |
virtual PxU32 | getNbMotionConstraints () const =0 |
Returns the number of motion constraints. | |
virtual void | setMotionConstraintConfig (const PxClothMotionConstraintConfig &config)=0 |
Specifies motion constraint scale, bias, and stiffness. | |
virtual PxClothMotionConstraintConfig | getMotionConstraintConfig () const =0 |
Reads back scale and bias factor for motion constraints. | |
virtual void | setSeparationConstraints (const PxClothParticleSeparationConstraint *separationConstraints)=0 |
Updates separation constraints (position and radius of the constraint sphere). | |
virtual bool | getSeparationConstraints (PxClothParticleSeparationConstraint *separationConstraintsBuffer) const =0 |
Copies separation constraints to the user provided buffer. | |
virtual PxU32 | getNbSeparationConstraints () const =0 |
Returns the number of separation constraints. | |
virtual void | clearInterpolation ()=0 |
Assign current to previous positions for collision shapes, motion constraints, and separation constraints. | |
virtual void | setStretchConfig (PxClothFabricPhaseType::Enum type, const PxClothStretchConfig &config)=0 |
Sets the solver parameters for the vertical solver phases. | |
virtual PxClothStretchConfig | getStretchConfig (PxClothFabricPhaseType::Enum type) const =0 |
Returns the solver parameters for one of the phase types. | |
virtual void | setTetherConfig (const PxClothTetherConfig &config)=0 |
Sets the stiffness parameters for the tether constraints. | |
virtual PxClothTetherConfig | getTetherConfig () const =0 |
Returns the stiffness parameters for the tether constraints. | |
Collision | |
Functions related to particle collision. | |
virtual void | addCollisionSphere (const PxClothCollisionSphere &sphere)=0 |
Adds a new collision sphere. | |
virtual void | removeCollisionSphere (PxU32 index)=0 |
Removes collision sphere. | |
virtual void | setCollisionSpheres (const PxClothCollisionSphere *spheresBuffer, PxU32 count)=0 |
Updates location and radii of collision spheres. | |
virtual PxU32 | getNbCollisionSpheres () const =0 |
Returns the number of collision spheres. | |
virtual void | addCollisionCapsule (PxU32 first, PxU32 second)=0 |
Adds a new collision capsule. | |
virtual void | removeCollisionCapsule (PxU32 index)=0 |
Removes a collision capsule. | |
virtual PxU32 | getNbCollisionCapsules () const =0 |
Returns the number of collision capsules. | |
virtual void | addCollisionPlane (const PxClothCollisionPlane &plane)=0 |
Adds a collision plane. | |
virtual void | removeCollisionPlane (PxU32 index)=0 |
Removes a collision plane. | |
virtual void | setCollisionPlanes (const PxClothCollisionPlane *planesBuffer, PxU32 count)=0 |
Updates positions of collision planes. | |
virtual PxU32 | getNbCollisionPlanes () const =0 |
Returns the number of collision planes. | |
virtual void | addCollisionConvex (PxU32 mask)=0 |
Adds a new collision convex. | |
virtual void | removeCollisionConvex (PxU32 index)=0 |
Removes a collision convex. | |
virtual PxU32 | getNbCollisionConvexes () const =0 |
Returns the number of collision convexes. | |
virtual void | addCollisionTriangle (const PxClothCollisionTriangle &triangle)=0 |
Adds a new collision triangle. | |
virtual void | removeCollisionTriangle (PxU32 index)=0 |
Removes a collision triangle. | |
virtual void | setCollisionTriangles (const PxClothCollisionTriangle *trianglesBuffer, PxU32 count)=0 |
Updates positions of collision triangles. | |
virtual PxU32 | getNbCollisionTriangles () const =0 |
Returns the number of collision triangles. | |
virtual void | getCollisionData (PxClothCollisionSphere *spheresBuffer, PxU32 *capsulesBuffer, PxClothCollisionPlane *planesBuffer, PxU32 *convexesBuffer, PxClothCollisionTriangle *trianglesBuffer) const =0 |
Retrieves the collision shapes. | |
virtual void | setVirtualParticles (PxU32 numVirtualParticles, const PxU32 *indices, PxU32 numWeights, const PxVec3 *weights)=0 |
Assigns virtual particles. | |
virtual PxU32 | getNbVirtualParticles () const =0 |
Returns the number of virtual particles. | |
virtual void | getVirtualParticles (PxU32 *indicesBuffer) const =0 |
Copies index array of virtual particles to the user provided buffer. | |
virtual PxU32 | getNbVirtualParticleWeights () const =0 |
Returns the number of the virtual particle weights. | |
virtual void | getVirtualParticleWeights (PxVec3 *weightsBuffer) const =0 |
Copies weight table of virtual particles to the user provided buffer. | |
virtual void | setFrictionCoefficient (PxReal frictionCoefficient)=0 |
Sets the collision friction coefficient. | |
virtual PxReal | getFrictionCoefficient () const =0 |
Returns the friction coefficient. | |
virtual void | setCollisionMassScale (PxReal scalingCoefficient)=0 |
Sets the collision mass scaling coefficient. | |
virtual PxReal | getCollisionMassScale () const =0 |
Returns the mass-scaling coefficient. | |
Self-Collision | |
Functions related to particle against particle collision. | |
virtual void | setSelfCollisionDistance (PxReal distance)=0 |
Sets the self collision distance. | |
virtual PxReal | getSelfCollisionDistance () const =0 |
Returns the self-collision distance. | |
virtual void | setSelfCollisionStiffness (PxReal stiffness)=0 |
Sets the self collision stiffness. | |
virtual PxReal | getSelfCollisionStiffness () const =0 |
Returns the self-collision stiffness. | |
virtual void | setSelfCollisionIndices (const PxU32 *indices, PxU32 nbIndices)=0 |
Sets a subset of cloth particles which participate in self-collision. | |
virtual bool | getSelfCollisionIndices (PxU32 *indices) const =0 |
Copies array of particles participating in self-collision to the user provided buffer. | |
virtual PxU32 | getNbSelfCollisionIndices () const =0 |
Returns the number of particles participating in self-collision. | |
virtual void | setRestPositions (const PxVec4 *restPositions)=0 |
Sets the cloth particles rest positions. | |
virtual bool | getRestPositions (PxVec4 *restPositions) const =0 |
Copies array of rest positions to the user provided buffer. | |
virtual PxU32 | getNbRestPositions () const =0 |
Returns the number of rest positions. | |
Inter-Collision | |
Functions related to collision between cloth instances. | |
virtual void | setSimulationFilterData (const PxFilterData &data)=0 |
Sets the user definable collision filter data. | |
virtual PxFilterData | getSimulationFilterData () const =0 |
Retrieves the object's collision filter data. | |
Scene-Collision | |
Functions related to collision against scene objects. | |
virtual void | setContactOffset (PxReal offset)=0 |
Sets the width by which the cloth bounding box is increased to find nearby scene collision shapes. | |
virtual PxReal | getContactOffset () const =0 |
Returns cloth contact offset. | |
virtual void | setRestOffset (PxReal offset)=0 |
Sets the minimum distance between colliding cloth particles and scene shapes. | |
virtual PxReal | getRestOffset () const =0 |
Returns cloth rest offset. | |
Sleeping | |
Functions related to sleeping. | |
virtual void | setSleepLinearVelocity (PxReal threshold)=0 |
Sets the velocity threshold for putting cloth in sleep state. | |
virtual PxReal | getSleepLinearVelocity () const =0 |
Returns the velocity threshold for putting cloth in sleep state. | |
virtual void | setWakeCounter (PxReal wakeCounterValue)=0 |
Sets the wake counter for the cloth. | |
virtual PxReal | getWakeCounter () const =0 |
Returns the wake counter of the cloth. | |
virtual void | wakeUp ()=0 |
Forces cloth to wake up from sleep state. | |
virtual void | putToSleep ()=0 |
Forces cloth to be put in sleep state. | |
virtual bool | isSleeping () const =0 |
Returns true if cloth is in sleep state. | |
Protected Member Functions | |
PX_INLINE | PxCloth (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxCloth (PxBaseFlags baseFlags) |
virtual | ~PxCloth () |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
Set of connected particles tailored towards simulating character cloth.A cloth object consists of the following components:
- A set of particles that sample the cloth. The sampling does not need to be regular. Particles are simulated in local space, which allows tuning the effect of changes to the global pose on the particles.
- Distance, bending, shearing, and tether constraints between particles. These are stored in a PxClothFabric instance which can be shared across cloth instances.
- Spheres, capsules, convexes, and triangle collision shapes. These shapes are all treated separately to the main PhysX rigid body scene.
- Virtual particles can be used to improve collision at a finer scale than the cloth sampling.
- Motion and separation constraints are used to limit the particle movement within or outside of a sphere.
- Deprecated:
- The PhysX cloth feature has been deprecated in PhysX version 3.4.1
- See also:
- PxPhysics.createCloth
Constructor & Destructor Documentation
PX_INLINE PxCloth::PxCloth | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxCloth::PxCloth | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PxCloth::~PxCloth | ( | ) | [inline, protected, virtual] |
Member Function Documentation
Adds a new collision capsule.
A collision capsule is defined as the bounding volume of two spheres.
- Parameters:
-
[in] first Index of first sphere. [in] second Index of second sphere.
- Note:
- A maximum of 32 capsules are supported.
Spheres referenced by a capsule need to be defined before simulating the scene, see addCollisionSphere/setCollisionSpheres.
virtual void PxCloth::addCollisionConvex | ( | PxU32 | mask | ) | [pure virtual] |
Adds a new collision convex.
A collision convex is defined as the intersection of planes.
- Parameters:
-
[in] mask The bitmask of the planes that make up the convex.
- Note:
- Planes referenced by a collision convex need to be defined before simulating the scene, see addCollisionPlane/setCollisionPlanes.
virtual void PxCloth::addCollisionPlane | ( | const PxClothCollisionPlane & | plane | ) | [pure virtual] |
Adds a collision plane.
- Parameters:
-
[in] plane New collision plane.
- Note:
- Planes are not used for collision until they are added to a convex object, see addCollisionConvex().
A maximum of 32 planes are supported.
virtual void PxCloth::addCollisionSphere | ( | const PxClothCollisionSphere & | sphere | ) | [pure virtual] |
Adds a new collision sphere.
- Parameters:
-
[in] sphere New collision sphere.
- Note:
- A maximum of 32 spheres are supported.
virtual void PxCloth::addCollisionTriangle | ( | const PxClothCollisionTriangle & | triangle | ) | [pure virtual] |
Adds a new collision triangle.
- Parameters:
-
[in] triangle New collision triangle.
- Note:
- GPU cloth is limited to 500 triangles per instance.
virtual void PxCloth::clearInterpolation | ( | ) | [pure virtual] |
Assign current to previous positions for collision shapes, motion constraints, and separation constraints.
This allows to prevent false interpolation after leaping to an animation frame, for example. After calling clearInterpolation(), the current positions will be used without interpolation. New positions can be set afterwards to interpolate to by the end of the next frame.
virtual PxVec3 PxCloth::getAngularDragCoefficient | ( | ) | const [pure virtual] |
Returns the angular drag coefficient.
- Returns:
- Angular drag coefficient.
virtual PxVec3 PxCloth::getAngularInertiaScale | ( | ) | const [pure virtual] |
Returns angular acceleration scale parameter.
- Returns:
- Angular acceleration scale parameter.
virtual PxVec3 PxCloth::getCentrifugalInertiaScale | ( | ) | const [pure virtual] |
Returns centrifugal acceleration scale parameter.
- Returns:
- Centrifugal acceleration scale parameter.
virtual PxClothFlags PxCloth::getClothFlags | ( | ) | const [pure virtual] |
Returns cloth flags.
- Returns:
- Cloth flags.
virtual void PxCloth::getCollisionData | ( | PxClothCollisionSphere * | spheresBuffer, | |
PxU32 * | capsulesBuffer, | |||
PxClothCollisionPlane * | planesBuffer, | |||
PxU32 * | convexesBuffer, | |||
PxClothCollisionTriangle * | trianglesBuffer | |||
) | const [pure virtual] |
Retrieves the collision shapes.
Returns collision spheres, capsules, convexes, and triangles that were added through the addCollision*() methods and modified through the setCollision*() methods.
- Parameters:
-
[out] spheresBuffer Spheres destination buffer, must be NULL or the same length as getNbCollisionSpheres(). [out] capsulesBuffer Capsules destination buffer, must be NULL or the same length as 2*getNbCollisionCapsules(). [out] planesBuffer Planes destination buffer, must be NULL or the same length as getNbCollisionPlanes(). [out] convexesBuffer Convexes destination buffer, must be NULL or the same length as getNbCollisionConvexes(). [out] trianglesBuffer Triangles destination buffer, must be NULL or the same length as getNbCollisionTriangles().
- Note:
- Returns the positions at the end of the next simulate() call as specified by the setCollision*() methods.
virtual PxReal PxCloth::getCollisionMassScale | ( | ) | const [pure virtual] |
Returns the mass-scaling coefficient.
- Returns:
- Mass-scaling coefficient.
virtual const char* PxCloth::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Implements PxBase.
virtual PxReal PxCloth::getContactOffset | ( | ) | const [pure virtual] |
virtual PxVec3 PxCloth::getDampingCoefficient | ( | ) | const [pure virtual] |
Returns the damping coefficient.
- Returns:
- Damping coefficient.
virtual PxVec3 PxCloth::getExternalAcceleration | ( | ) | const [pure virtual] |
Returns external acceleration.
- Returns:
- External acceleration in global coordinates.
virtual PxClothFabric* PxCloth::getFabric | ( | ) | const [pure virtual] |
Returns a pointer to the corresponding cloth fabric.
- Returns:
- The associated cloth fabric.
virtual PxReal PxCloth::getFrictionCoefficient | ( | ) | const [pure virtual] |
Returns the friction coefficient.
- Returns:
- Friction coefficient.
virtual PxTransform PxCloth::getGlobalPose | ( | ) | const [pure virtual] |
Returns global pose.
- Returns:
- Global pose as specified by the last setGlobalPose() or setTargetPose() call.
virtual PxVec3 PxCloth::getLinearDragCoefficient | ( | ) | const [pure virtual] |
Returns the linear drag coefficient.
- Returns:
- Linear drag coefficient.
virtual PxVec3 PxCloth::getLinearInertiaScale | ( | ) | const [pure virtual] |
Returns linear acceleration scale parameter.
- Returns:
- Linear acceleration scale parameter.
virtual PxClothMotionConstraintConfig PxCloth::getMotionConstraintConfig | ( | ) | const [pure virtual] |
virtual bool PxCloth::getMotionConstraints | ( | PxClothParticleMotionConstraint * | motionConstraintsBuffer | ) | const [pure virtual] |
Copies motion constraints to the user provided buffer.
- Parameters:
-
[out] motionConstraintsBuffer Destination buffer, must be at least getNbMotionConstraints().
- Returns:
- True if the copy was successful.
virtual PxU32 PxCloth::getNbCollisionCapsules | ( | ) | const [pure virtual] |
Returns the number of collision capsules.
- Returns:
- Number of collision capsules.
virtual PxU32 PxCloth::getNbCollisionConvexes | ( | ) | const [pure virtual] |
Returns the number of collision convexes.
- Returns:
- Number of collision convexes.
virtual PxU32 PxCloth::getNbCollisionPlanes | ( | ) | const [pure virtual] |
Returns the number of collision planes.
- Returns:
- Number of collision planes.
virtual PxU32 PxCloth::getNbCollisionSpheres | ( | ) | const [pure virtual] |
Returns the number of collision spheres.
- Returns:
- Number of collision spheres.
virtual PxU32 PxCloth::getNbCollisionTriangles | ( | ) | const [pure virtual] |
Returns the number of collision triangles.
- Returns:
- Number of collision triangles.
virtual PxU32 PxCloth::getNbMotionConstraints | ( | ) | const [pure virtual] |
Returns the number of motion constraints.
- Returns:
- Number of motion constraints (same as getNbParticles() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbParticleAccelerations | ( | ) | const [pure virtual] |
Returns the number of particle accelerations.
- Returns:
- Number of particle accelerations (same as getNbParticles() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbParticles | ( | ) | const [pure virtual] |
Returns the number of particles.
- Returns:
- Number of particles.
virtual PxU32 PxCloth::getNbRestPositions | ( | ) | const [pure virtual] |
Returns the number of rest positions.
- Returns:
- Number of rest positions (same as getNbParticles() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbSelfCollisionIndices | ( | ) | const [pure virtual] |
Returns the number of particles participating in self-collision.
- Returns:
- Size of particle subset participating in self-collision, or 0 if all particles are used.
virtual PxU32 PxCloth::getNbSeparationConstraints | ( | ) | const [pure virtual] |
Returns the number of separation constraints.
- Returns:
- Number of separation constraints (same as getNbParticles() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbVirtualParticles | ( | ) | const [pure virtual] |
Returns the number of virtual particles.
- Returns:
- Number of virtual particles.
virtual PxU32 PxCloth::getNbVirtualParticleWeights | ( | ) | const [pure virtual] |
Returns the number of the virtual particle weights.
- Returns:
- Number of virtual particle weights.
virtual bool PxCloth::getParticleAccelerations | ( | PxVec4 * | particleAccelerationsBuffer | ) | const [pure virtual] |
Copies particle accelerations to the user provided buffer.
- Parameters:
-
[out] particleAccelerationsBuffer Destination buffer, must be at least getNbParticleAccelerations().
- Returns:
- true if the copy was successful.
virtual PxReal PxCloth::getPreviousTimeStep | ( | ) | const [pure virtual] |
Returns previous time step size.
Time between sampling of previous and current particle positions for computing particle velocity.
- Returns:
- Previous time step size.
virtual PxReal PxCloth::getRestOffset | ( | ) | const [pure virtual] |
virtual bool PxCloth::getRestPositions | ( | PxVec4 * | restPositions | ) | const [pure virtual] |
Copies array of rest positions to the user provided buffer.
- Parameters:
-
[out] restPositions Destination buffer, must be at least getNbParticles() in length.
- Returns:
- true if the copy was successful.
virtual PxReal PxCloth::getSelfCollisionDistance | ( | ) | const [pure virtual] |
Returns the self-collision distance.
- Returns:
- Self-collision distance.
virtual bool PxCloth::getSelfCollisionIndices | ( | PxU32 * | indices | ) | const [pure virtual] |
Copies array of particles participating in self-collision to the user provided buffer.
- Parameters:
-
[out] indices Destination buffer, must be at least getNbSelfCollisionIndices() in length.
- Returns:
- true if the copy was successful.
virtual PxReal PxCloth::getSelfCollisionStiffness | ( | ) | const [pure virtual] |
Returns the self-collision stiffness.
- Returns:
- Self-collision stiffness.
virtual bool PxCloth::getSeparationConstraints | ( | PxClothParticleSeparationConstraint * | separationConstraintsBuffer | ) | const [pure virtual] |
Copies separation constraints to the user provided buffer.
- Parameters:
-
[out] separationConstraintsBuffer Destination buffer, must be at least getNbSeparationConstraints().
- Returns:
- True if the copy was successful.
virtual PxFilterData PxCloth::getSimulationFilterData | ( | ) | const [pure virtual] |
Retrieves the object's collision filter data.
- Returns:
- Associated filter data
- See also:
- setSimulationFilterData() PxFilterData
virtual PxReal PxCloth::getSleepLinearVelocity | ( | ) | const [pure virtual] |
Returns the velocity threshold for putting cloth in sleep state.
- Returns:
- Velocity threshold for putting cloth in sleep state.
virtual PxReal PxCloth::getSolverFrequency | ( | ) | const [pure virtual] |
Returns solver frequency.
- Returns:
- Solver frequency.
virtual PxReal PxCloth::getStiffnessFrequency | ( | ) | const [pure virtual] |
Returns stiffness frequency.
- Returns:
- Stiffness frequency.
- See also:
- setStiffnessFrequency() for details.
virtual PxClothStretchConfig PxCloth::getStretchConfig | ( | PxClothFabricPhaseType::Enum | type | ) | const [pure virtual] |
Returns the solver parameters for one of the phase types.
- Parameters:
-
[in] type Type of phases to return the config for.
- Returns:
- Vertical solver parameters.
virtual PxClothTetherConfig PxCloth::getTetherConfig | ( | ) | const [pure virtual] |
Returns the stiffness parameters for the tether constraints.
- Returns:
- Tether solver parameters.
virtual void PxCloth::getVirtualParticles | ( | PxU32 * | indicesBuffer | ) | const [pure virtual] |
Copies index array of virtual particles to the user provided buffer.
- Parameters:
-
[out] indicesBuffer Destination buffer, must be at least 4*getNbVirtualParticles().
- See also:
- setVirtualParticles()
virtual void PxCloth::getVirtualParticleWeights | ( | PxVec3 * | weightsBuffer | ) | const [pure virtual] |
Copies weight table of virtual particles to the user provided buffer.
- Parameters:
-
[out] weightsBuffer Destination buffer, must be at least getNbVirtualParticleWeights().
virtual PxReal PxCloth::getWakeCounter | ( | ) | const [pure virtual] |
Returns the wake counter of the cloth.
- Returns:
- The wake counter of the cloth.
- See also:
- isSleeping() setWakeCounter()
virtual PxReal PxCloth::getWindDrag | ( | ) | const [pure virtual] |
Returns the coefficient of the air drag on the fabric's triangles.
virtual PxReal PxCloth::getWindLift | ( | ) | const [pure virtual] |
Returns the coefficient of the air lift on the fabric's triangles.
virtual PxVec3 PxCloth::getWindVelocity | ( | ) | const [pure virtual] |
Returns the velocity of the wind affecting the fabric's triangles.
virtual PxBounds3 PxCloth::getWorldBounds | ( | float | inflation = 1.01f |
) | const [pure virtual] |
Returns world space bounding box.
- Parameters:
-
[in] inflation Scale factor for computed world bounds. Box extents are multiplied by this value.
- Returns:
- Particle bounds in global coordinates.
Implements PxActor.
virtual bool PxCloth::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxActor.
References PxActor::isKindOf().
virtual bool PxCloth::isSleeping | ( | ) | const [pure virtual] |
Returns true if cloth is in sleep state.
- Note:
- It is invalid to use this method if the cloth has not been added to a scene already.
- Returns:
- True if cloth is in sleep state.
virtual PxClothParticleData* PxCloth::lockParticleData | ( | ) | const [pure virtual] |
Acquires read access to the cloth particle data.
- Returns:
- PxClothParticleData pointer which provides access to positions and weight.
- Note:
- This function is equivalent to lockParticleData(PxDataAccessFlag::eREADABLE).
virtual PxClothParticleData* PxCloth::lockParticleData | ( | PxDataAccessFlags | flags | ) | [pure virtual] |
Acquires access to the cloth particle data.
This function returns a pointer to a PxClothParticleData instance providing access to the PxClothParticle array of the current and previous iteration. The user is responsible for calling PxClothParticleData::unlock() after reading or updating the data. In case the lock has been requested using PxDataAccessFlag::eWRITABLE, the unlock() call copies the arrays pointed to by PxClothParticleData::particles/previousParticles back to the internal particle buffer. Updating the data when a read-only lock has been requested results in undefined behavior. Requesting multiple concurrent read-only locks is supported, but no other lock may be active when requesting a write lock.
If PxDataAccessFlag::eDEVICE is set in flags then the returned pointers will be to CUDA device memory, this can be used for direct interop with graphics APIs. Note that these pointers should only be considered valid until PxClothParticleData::unlock() is called and should not be stored. PxDataAccessFlag::eDEVICE implies read and write access, and changing the particles/previousParticles members results in undefined behavior.
- Parameters:
-
flags Specifies if particle data is read or written.
- Returns:
- PxClothParticleData pointer which provides access to positions and weight.
virtual void PxCloth::putToSleep | ( | ) | [pure virtual] |
Forces cloth to be put in sleep state.
- Note:
- It is invalid to use this method if the cloth has not been added to a scene already.
virtual void PxCloth::release | ( | ) | [pure virtual] |
virtual void PxCloth::removeCollisionCapsule | ( | PxU32 | index | ) | [pure virtual] |
Removes a collision capsule.
- Parameters:
-
[in] index Index of capsule to remove.
- Note:
- The indices of capsules added after
index
are decremented by 1.
virtual void PxCloth::removeCollisionConvex | ( | PxU32 | index | ) | [pure virtual] |
Removes a collision convex.
- Parameters:
-
[in] index Index of convex to remove.
- Note:
- The indices of convexes added after
index
are decremented by 1.Planes referenced by this convex will not be removed.
virtual void PxCloth::removeCollisionPlane | ( | PxU32 | index | ) | [pure virtual] |
Removes a collision plane.
- Parameters:
-
[in] index Index of plane to remove.
- Note:
- The indices of planes added after
index
are decremented by 1.Convexes that reference the plane will have the plane removed from their mask. If after removal a convex consists of zero planes, it will also be removed.
virtual void PxCloth::removeCollisionSphere | ( | PxU32 | index | ) | [pure virtual] |
Removes collision sphere.
- Parameters:
-
[in] index Index of sphere to remove.
- Note:
- The indices of spheres added after
index
are decremented by 1.Capsules made from the sphere to be removed are removed as well.
virtual void PxCloth::removeCollisionTriangle | ( | PxU32 | index | ) | [pure virtual] |
Removes a collision triangle.
- Parameters:
-
[in] index Index of triangle to remove.
- Note:
- The indices of triangles added after
index
are decremented by 1.
virtual void PxCloth::setAngularDragCoefficient | ( | PxVec3 | dragCoefficient | ) | [pure virtual] |
Sets the angular drag coefficient.
The angular drag coefficient is the portion of the pose rotation that is applied to each particle per stiffness period (see PxCloth::setStiffnessFrequency).
- Note:
- The scale is specified independently for each local rotation axis.
- Parameters:
-
[in] dragCoefficient New angular drag coefficient between 0.0f and 1.0 (default: 0.0).
- Note:
- The drag coefficient shouldn't be set higher than the damping coefficient.
virtual void PxCloth::setAngularInertiaScale | ( | PxVec3 | scale | ) | [pure virtual] |
Sets the acceleration scale factor to adjust inertia effect from global pose changes.
- Parameters:
-
[in] scale New scale factor between 0.0 (no inertia) and 1.0 (full inertia) (default: 1.0).
- Note:
- The scale is specified independently for each local rotation axis.
A value of 0.0 disables all inertia effects of rotations applied through setTargetPos().
- See also:
- setTargetPose()
virtual void PxCloth::setCentrifugalInertiaScale | ( | PxVec3 | scale | ) | [pure virtual] |
Sets the acceleration scale factor to adjust inertia effect from global pose changes.
- Parameters:
-
[in] scale New scale factor between 0.0 (no centrifugal force) and 1.0 (full centrifugal force) (default: 1.0).
- Note:
- The scale is specified independently for each local rotation axis.
A value of 0.0 disables all centrifugal forces of rotations applied through setTargetPos().
- See also:
- setTargetPose()
virtual void PxCloth::setClothFlag | ( | PxClothFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
Sets cloth flags (e.g. use GPU for simulation, enable CCD, collide against scene).
- Parameters:
-
[in] flag Mask of which flags to set. [in] value Value to set flags to.
virtual void PxCloth::setClothFlags | ( | PxClothFlags | inFlags | ) | [pure virtual] |
Set all cloth flags.
- Parameters:
-
[in] inFlags Bit mask of flag values
virtual void PxCloth::setCollisionMassScale | ( | PxReal | scalingCoefficient | ) | [pure virtual] |
Sets the collision mass scaling coefficient.
During collision it is possible to artificially increase the mass of a colliding particle, this has an effect comparable to making constraints attached to the particle stiffer and can help reduce stretching and interpenetration around collision shapes.
- Parameters:
-
[in] scalingCoefficient Unitless multiplier that can take on values > 1 (default: 0.0).
virtual void PxCloth::setCollisionPlanes | ( | const PxClothCollisionPlane * | planesBuffer, | |
PxU32 | count | |||
) | [pure virtual] |
Updates positions of collision planes.
- Parameters:
-
[in] planesBuffer New plane positions by the end of the next simulate() call. [in] count New number of collision planes.
- Note:
- You can also use this function to change the number of collision planes.
A maximum of 32 planes are supported.
- See also:
- clearInterpolation()
virtual void PxCloth::setCollisionSpheres | ( | const PxClothCollisionSphere * | spheresBuffer, | |
PxU32 | count | |||
) | [pure virtual] |
Updates location and radii of collision spheres.
- Parameters:
-
[in] spheresBuffer New sphere positions and radii by the end of the next simulate() call. [in] count New number of collision spheres.
- Note:
- You can also use this function to change the number of collision spheres.
A maximum of 32 spheres are supported.
- See also:
- clearInterpolation()
virtual void PxCloth::setCollisionTriangles | ( | const PxClothCollisionTriangle * | trianglesBuffer, | |
PxU32 | count | |||
) | [pure virtual] |
Updates positions of collision triangles.
- Parameters:
-
[in] trianglesBuffer New triangle positions by the end of the next simulate() call. [in] count New number of collision triangles.
- Note:
- You can also use this function to change the number of collision triangles.
GPU cloth is limited to 500 triangles per instance.
- See also:
- clearInterpolation()
virtual void PxCloth::setContactOffset | ( | PxReal | offset | ) | [pure virtual] |
Sets the width by which the cloth bounding box is increased to find nearby scene collision shapes.
The cloth particles collide against shapes in the scene that intersect the cloth bounding box enlarged by the contact offset (if the eSCENE_COLLISION flag is set). Default: 0.0f
- Parameters:
-
[in] offset Range: [0, PX_MAX_F32)
- See also:
- getContactOffset setRestOffset
virtual void PxCloth::setDampingCoefficient | ( | PxVec3 | dampingCoefficient | ) | [pure virtual] |
Sets the damping coefficient.
The damping coefficient is the portion of local particle velocity that is canceled per stiffness period (see PxCloth::setStiffnessFrequency).
- Note:
- The scale is specified independently for each local space axis.
- Parameters:
-
[in] dampingCoefficient New damping coefficient between 0.0 and 1.0 (default: 0.0).
virtual void PxCloth::setDragCoefficient | ( | PxReal | scale | ) | [pure virtual] |
Same as setLinearDragCoefficient(PxVec3(coefficient)); setAngularDragCoefficient(PxVec3(coefficient));
.
virtual void PxCloth::setExternalAcceleration | ( | PxVec3 | acceleration | ) | [pure virtual] |
Sets external particle accelerations.
- Parameters:
-
[in] acceleration New acceleration in global coordinates (default: 0.0).
- Note:
- Use this to implement simple wind etc.
virtual void PxCloth::setFrictionCoefficient | ( | PxReal | frictionCoefficient | ) | [pure virtual] |
Sets the collision friction coefficient.
- Parameters:
-
[in] frictionCoefficient New friction coefficient between 0.0 and 1.0 (default: 0.0).
- Note:
- Currently only spheres and capsules impose friction on the colliding particles.
virtual void PxCloth::setGlobalPose | ( | const PxTransform & | pose | ) | [pure virtual] |
Sets current pose of the cloth without affecting inertia.
Use this to reset the pose (e.g. teleporting).
- Parameters:
-
[in] pose New global pose.
- Note:
- No pose interpolation is performed.
Inertia is not preserved.
- See also:
- setTargetPose() for inertia preserving method.
virtual void PxCloth::setInertiaScale | ( | PxReal | scale | ) | [pure virtual] |
Same as setLinearInertiaScale(PxVec3(scale)); setAngularInertiaScale(PxVec3(scale)); getCentrifugalInertiaScale(PxVec3(scale));
.
virtual void PxCloth::setLinearDragCoefficient | ( | PxVec3 | dragCoefficient | ) | [pure virtual] |
Sets the linear drag coefficient.
The linear drag coefficient is the portion of the pose translation that is applied to each particle per stiffness period (see PxCloth::setStiffnessFrequency).
- Note:
- The scale is specified independently for each local space axis.
- Parameters:
-
[in] dragCoefficient New linear drag coefficient between 0.0f and 1.0 (default: 0.0).
- Note:
- The drag coefficient shouldn't be set higher than the damping coefficient.
virtual void PxCloth::setLinearInertiaScale | ( | PxVec3 | scale | ) | [pure virtual] |
Sets the acceleration scale factor to adjust inertia effect from global pose changes.
- Parameters:
-
[in] scale New scale factor between 0.0 (no inertia) and 1.0 (full inertia) (default: 1.0).
- Note:
- The scale is specified independently for each local coordinate axis.
A value of 0.0 disables all inertia effects of translations applied through setTargetPos().
- See also:
- setTargetPose()
virtual void PxCloth::setMotionConstraintConfig | ( | const PxClothMotionConstraintConfig & | config | ) | [pure virtual] |
Specifies motion constraint scale, bias, and stiffness.
- Parameters:
-
[in] config Motion constraints solver parameters.
virtual void PxCloth::setMotionConstraints | ( | const PxClothParticleMotionConstraint * | motionConstraints | ) | [pure virtual] |
Updates motion constraints (position and radius of the constraint sphere).
- Parameters:
-
[in] motionConstraints motion constraints at the end of the next simulate() call.
- Note:
- The motionConstraints must either be null to disable motion constraints, or be the same length as the number of particles, see getNbParticles().
- See also:
- clearInterpolation()
virtual void PxCloth::setParticleAccelerations | ( | const PxVec4 * | particleAccelerations | ) | [pure virtual] |
Updates particle accelerations, w component is ignored.
- Parameters:
-
[in] particleAccelerations New particle accelerations.
- Note:
- The particleAccelerations must either be null to disable accelerations, or be the same length as the number of particles, see getNbParticles().
virtual void PxCloth::setParticles | ( | const PxClothParticle * | currentParticles, | |
const PxClothParticle * | previousParticles | |||
) | [pure virtual] |
Updates cloth particle location or inverse weight for current and previous particle state.
- Parameters:
-
[in] currentParticles The particle data for the current particle state or NULL if the state should not be changed. [in] previousParticles The particle data for the previous particle state or NULL if the state should not be changed.
- Note:
- The invWeight stored in previousParticles is the new particle inverse mass, or zero for a static particle. However, if invWeight stored in currentParticles is non-zero, it is still used once for the next particle integration and fabric solve.
If currentParticles or previousParticles are non-NULL then they must be the length specified by getNbParticles().
This can be used to teleport particles (use same positions for current and previous).
- See also:
- PxClothParticle
virtual void PxCloth::setRestOffset | ( | PxReal | offset | ) | [pure virtual] |
Sets the minimum distance between colliding cloth particles and scene shapes.
Cloth particles colliding against shapes in the scene get no closer to the shape's surface than specified by the rest offset (if the eSCENE_COLLISION flag is set). Default: 0.0f
- Parameters:
-
[in] offset Range: [0, PX_MAX_F32)
- See also:
- getRestOffset setContactOffset
virtual void PxCloth::setRestPositions | ( | const PxVec4 * | restPositions | ) | [pure virtual] |
Sets the cloth particles rest positions.
If non-null the cloth self-collision will consider the rest positions by discarding particle->particle collision where the distance between the associated rest particles is < the self collision distance. This allows self-collision distances that are larger than the minimum edge length in the mesh. Typically this function should be called with the same positions used to construct the cloth instance.
- Parameters:
-
[in] restPositions Undeformed particle positions, the w component will be ignored
- Note:
- restPositions must either be null to disable rest position consideration, or be the same length as the number of particles, see getNbParticles().
virtual void PxCloth::setSelfCollisionDistance | ( | PxReal | distance | ) | [pure virtual] |
Sets the self collision distance.
A value larger than 0.0 enables particle versus particle collision.
- Parameters:
-
[in] distance Minimum distance at which two particles repel each other (default: 0.0).
virtual void PxCloth::setSelfCollisionIndices | ( | const PxU32 * | indices, | |
PxU32 | nbIndices | |||
) | [pure virtual] |
Sets a subset of cloth particles which participate in self-collision.
If non-null the cloth self-collision will consider a subset of particles instead of all the particles. This can be used to improve self-collision performance or to increase the minimum distance between two self-colliding particles (and therefore the maximum sensible self-collision distance).
- Parameters:
-
[in] indices array of particle indices which participate in self-collision. [in] nbIndices number of particle indices, or 0 to use all particles for self-collision.
- Note:
- These indices will also be used if cloth inter-collision is enabled.
virtual void PxCloth::setSelfCollisionStiffness | ( | PxReal | stiffness | ) | [pure virtual] |
Sets the self collision stiffness.
Self-collision stiffness controls how much two particles repel each other when they are closer than the self-collision distance.
- Parameters:
-
[in] stiffness Fraction of distance residual to resolve per iteration (default: 1.0).
virtual void PxCloth::setSeparationConstraints | ( | const PxClothParticleSeparationConstraint * | separationConstraints | ) | [pure virtual] |
Updates separation constraints (position and radius of the constraint sphere).
- Parameters:
-
[in] separationConstraints separation constraints at the end of the next simulate() call.
- Note:
- The separationConstraints must either be null to disable separation constraints, or be the same length as the number of particles, see getNbParticles().
- See also:
- clearInterpolation()
virtual void PxCloth::setSimulationFilterData | ( | const PxFilterData & | data | ) | [pure virtual] |
Sets the user definable collision filter data.
- Parameters:
-
data The data that will be returned in the PxScene filter shader callback.
- Note:
- To disable collision on a cloth actor it is sufficient to set the filter data to some non-zero value (if using the SDK's default filter shader).
- See also:
- PxSimulationFilterShader PxFilterData
virtual void PxCloth::setSleepLinearVelocity | ( | PxReal | threshold | ) | [pure virtual] |
Sets the velocity threshold for putting cloth in sleep state.
If none of the particles moves faster (in local space) than the threshold for a while, the cloth will be put in sleep state and simulation will be skipped.
- Parameters:
-
[in] threshold Velocity threshold (default: 0.0f)
virtual void PxCloth::setSolverFrequency | ( | PxReal | frequency | ) | [pure virtual] |
Sets the solver frequency parameter.
Solver frequency specifies how often the simulation step is computed per second. For example, a value of 60 represents one simulation step per frame in a 60fps scene. A value of 120 will represent two simulation steps per frame, etc.
- Parameters:
-
[in] frequency Solver frequency per second (default: 60.0).
virtual void PxCloth::setStiffnessFrequency | ( | PxReal | frequency | ) | [pure virtual] |
Sets the stiffness frequency parameter.
The stiffness frequency controls the power-law nonlinearity of all rate of change parameters (stretch stiffness, shear stiffness, bending stiffness, tether stiffness, self-collision stiffness, motion constraint stiffness, damp coefficient, linear and angular drag coefficients). Increasing the frequency avoids numerical cancellation for values near zero or one, but increases the non-linearity of the parameter. It is not recommended to change this parameter after cloth initialization. For example, the portion of edge overstretch removed per second is equal to the stretch stiffness raised to the power of the stiffness frequency.
- Parameters:
-
[in] frequency Stiffness frequency per second (default: 10.0).
virtual void PxCloth::setStretchConfig | ( | PxClothFabricPhaseType::Enum | type, | |
const PxClothStretchConfig & | config | |||
) | [pure virtual] |
Sets the solver parameters for the vertical solver phases.
- Parameters:
-
[in] config Stretch solver parameters. [in] type Type of phases to set config for.
virtual void PxCloth::setTargetPose | ( | const PxTransform & | pose | ) | [pure virtual] |
Sets pose that the cloth should move to by the end of the next simulate() call.
This function will move the cloth in world space. The resulting simulation may reflect inertia effect as a result of pose acceleration.
- Parameters:
-
[in] pose Target pose at the end of the next simulate() call.
- See also:
- setGlobalPose() to move Cloth without inertia effect.
virtual void PxCloth::setTetherConfig | ( | const PxClothTetherConfig & | config | ) | [pure virtual] |
Sets the stiffness parameters for the tether constraints.
- Parameters:
-
[in] config Tether constraints solver parameters.
virtual void PxCloth::setVirtualParticles | ( | PxU32 | numVirtualParticles, | |
const PxU32 * | indices, | |||
PxU32 | numWeights, | |||
const PxVec3 * | weights | |||
) | [pure virtual] |
Assigns virtual particles.
Virtual particles provide more robust and accurate collision handling against collision spheres and capsules. More virtual particles will generally increase the accuracy of collision handling, and thus a sufficient number of virtual particles can mimic triangle-based collision handling.
Virtual particles are specified as barycentric interpolation of real particles: The position of a virtual particle is w0 * P0 + w1 * P1 + w2 * P2, where P0, P1, P2 real particle positions. The barycentric weights w0, w1, w2 are stored in a separate table so they can be shared across multiple virtual particles.
- Parameters:
-
[in] numVirtualParticles total number of virtual particles. [in] indices Each virtual particle has four indices, the first three for real particle indices, and the last for the weight table index. Thus, the length of indices needs to be 4*numVirtualParticles. [in] numWeights total number of unique weights triples. [in] weights array for barycentric weights.
- Note:
- Virtual particles only incur a runtime cost during the collision stage. Still, it is advisable to only use virtual particles for areas where high collision accuracy is desired. (e.g. sleeve around elbow).
virtual void PxCloth::setWakeCounter | ( | PxReal | wakeCounterValue | ) | [pure virtual] |
Sets the wake counter for the cloth.
The wake counter value determines how long all particles need to move less than the velocity threshold until the cloth is put to sleep (see setSleepLinearVelocity()).
- Note:
- Passing in a positive value will wake the cloth up automatically. Default: 0.395 (which corresponds to 20 frames for a time step of 0.02)
- Parameters:
-
[in] wakeCounterValue Wake counter value. Range: [0, PX_MAX_F32)
- See also:
- isSleeping() getWakeCounter()
virtual void PxCloth::setWindDrag | ( | PxReal | ) | [pure virtual] |
Sets the coefficient of the air drag on the fabric's triangles.
virtual void PxCloth::setWindLift | ( | PxReal | ) | [pure virtual] |
Sets the coefficient of the air lift on the fabric's triangles.
virtual void PxCloth::setWindVelocity | ( | PxVec3 | ) | [pure virtual] |
Sets the velocity of the wind affecting the fabric's triangles.
virtual void PxCloth::wakeUp | ( | ) | [pure virtual] |
Forces cloth to wake up from sleep state.
- Note:
- This will set the wake counter of the cloth to the value specified in PxSceneDesc::wakeCounterResetValue.
It is invalid to use this method if the cloth has not been added to a scene already.
- See also:
- isSleeping() putToSleep()
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com