PxClothFabric Class Reference
[Cloth]
A cloth fabric is a structure that contains all the internal solver constraints of a cloth mesh.
More...
#include <PxClothFabric.h>
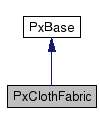
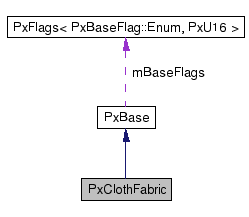
Public Member Functions | |
virtual void | release ()=0 |
Decrements the cloth fabric's reference count, and releases it if the new reference count is zero. | |
virtual PxU32 | getNbParticles () const =0 |
Returns number of particles. | |
virtual PxU32 | getNbPhases () const =0 |
Returns number of phases. | |
virtual PxU32 | getNbRestvalues () const =0 |
Returns number of rest values. | |
virtual PxU32 | getNbSets () const =0 |
Returns number of sets. | |
virtual PxU32 | getNbParticleIndices () const =0 |
Get number of particle indices. | |
virtual PxU32 | getNbTethers () const =0 |
Get number of tether constraints. | |
virtual PxU32 | getPhases (PxClothFabricPhase *userPhaseBuffer, PxU32 bufferSize) const =0 |
Copies the phase array to a user specified buffer. | |
virtual PxU32 | getSets (PxU32 *userSetBuffer, PxU32 bufferSize) const =0 |
Copies the set array to a user specified buffer. | |
virtual PxU32 | getParticleIndices (PxU32 *userParticleIndexBuffer, PxU32 bufferSize) const =0 |
Copies the particle indices array to a user specified buffer. | |
virtual PxU32 | getRestvalues (PxReal *userRestvalueBuffer, PxU32 bufferSize) const =0 |
Copies the rest values array to a user specified buffer. | |
virtual PxU32 | getTetherAnchors (PxU32 *userAnchorBuffer, PxU32 bufferSize) const =0 |
Copies the tether anchors array to a user specified buffer. | |
virtual PxU32 | getTetherLengths (PxReal *userLengthBuffer, PxU32 bufferSize) const =0 |
Copies the tether lengths array to a user specified buffer. | |
virtual void | scaleRestlengths (PxReal scale)=0 |
Scale all rest values of length dependent constraints. | |
virtual PxU32 | getReferenceCount () const =0 |
Reference count of the cloth instance. | |
virtual void | acquireReference ()=0 |
Acquires a counted reference to a cloth fabric. | |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
Protected Member Functions | |
PX_INLINE | PxClothFabric (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxClothFabric (PxBaseFlags baseFlags) |
virtual | ~PxClothFabric () |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A cloth fabric is a structure that contains all the internal solver constraints of a cloth mesh.
A fabric consists of phases
that represent a group of internal constraints of the same type. Each phase references an array of rest-values
and a set
of particle indices. The data representation for the fabric has layers of indirect indices:
- All particle indices for the constraints of the fabric are stored in one linear array and referenced by the sets.
- Each constraint (particle index pair) has one entry in the restvalues array.
- The set array holds the prefix sum of the number of constraints per set and is referenced by the phases.
- A phase consists of the type of constraints, the index of the set referencing the indices.
- Deprecated:
- The PhysX cloth feature has been deprecated in PhysX version 3.4.1
- See also:
- The fabric structure can be created from a mesh using PxClothFabricCreate. Alternatively, the fabric data can be saved into a stream (see PxClothFabricCooker.save()) and later created from the stream using PxPhysics.createClothFabric(PxInputStream&).
Constructor & Destructor Documentation
PX_INLINE PxClothFabric::PxClothFabric | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxClothFabric::PxClothFabric | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PxClothFabric::~PxClothFabric | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual void PxClothFabric::acquireReference | ( | ) | [pure virtual] |
Acquires a counted reference to a cloth fabric.
This method increases the reference count of the cloth fabric by 1. Decrement the reference count by calling release()
virtual const char* PxClothFabric::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Implements PxBase.
virtual PxU32 PxClothFabric::getNbParticleIndices | ( | ) | const [pure virtual] |
Get number of particle indices.
- Returns:
- The size of the particle indices array.
virtual PxU32 PxClothFabric::getNbParticles | ( | ) | const [pure virtual] |
Returns number of particles.
- Returns:
- The number of particles needed when creating a PxCloth instance from the fabric.
virtual PxU32 PxClothFabric::getNbPhases | ( | ) | const [pure virtual] |
Returns number of phases.
- Returns:
- The number of solver phases.
virtual PxU32 PxClothFabric::getNbRestvalues | ( | ) | const [pure virtual] |
Returns number of rest values.
- Returns:
- The size of the rest values array.
virtual PxU32 PxClothFabric::getNbSets | ( | ) | const [pure virtual] |
Returns number of sets.
- Returns:
- The size of the set array.
virtual PxU32 PxClothFabric::getNbTethers | ( | ) | const [pure virtual] |
Get number of tether constraints.
- Returns:
- The size of the tether anchors and lengths arrays.
virtual PxU32 PxClothFabric::getParticleIndices | ( | PxU32 * | userParticleIndexBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the particle indices array to a user specified buffer.
The particle indices array determines which particles are affected by each constraint. It has the same length as getNbParticleIndices() and twice the number of constraints.
- Parameters:
-
[in] userParticleIndexBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userParticleIndexBuffer, should be at least getNbParticleIndices().
- Returns:
- getNbParticleIndices() if the copy was successful, 0 otherwise.
- Note:
- This function is potentially slow. Consider caching the (static) result instead of retrieving it multiple times.
virtual PxU32 PxClothFabric::getPhases | ( | PxClothFabricPhase * | userPhaseBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the phase array to a user specified buffer.
The phase array is a mapping of the phase index to the corresponding phase. The array has the same length as getNbPhases().
- Parameters:
-
[in] userPhaseBuffer Destination buffer to copy the phase data to. [in] bufferSize Size of userPhaseBuffer, should be at least getNbPhases().
- Returns:
- getNbPhases() if the copy was successful, 0 otherwise.
- Note:
- This function is potentially slow. Consider caching the (static) result instead of retrieving it multiple times.
virtual PxU32 PxClothFabric::getReferenceCount | ( | ) | const [pure virtual] |
Reference count of the cloth instance.
At creation, the reference count of the fabric is 1. Every cloth instance referencing this fabric increments the count by 1. When the reference count reaches 0, and only then, the fabric gets released automatically.
- See also:
- PxCloth
virtual PxU32 PxClothFabric::getRestvalues | ( | PxReal * | userRestvalueBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the rest values array to a user specified buffer.
The rest values array holds the target value of the constraint in rest state, for example edge length for stretch constraints. It stores one value per constraint, so its length is half of getNbParticleIndices(), and it has the same length as getNbRestvalues(). The rest-values are stored in the order they are (indirectly) referenced by the phases.
- Parameters:
-
[in] userRestvalueBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userRestvalueBuffer, should be at least getNbRestvalues().
- Returns:
- getNbRestvalues() if the copy was successful, 0 otherwise.
- Note:
- This function is potentially slow. Between calling scaleRestlengths(), consider caching the result instead of retrieving it multiple times.
virtual PxU32 PxClothFabric::getSets | ( | PxU32 * | userSetBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the set array to a user specified buffer.
The set array is the inclusive prefix sum of the number of constraints per set. It has the same length as getNbSets().
- Parameters:
-
[in] userSetBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userSetBuffer, should be at least getNbSets().
- Returns:
- getNbSets() if the copy was successful, 0 otherwise.
- Note:
- Indices of the i-th set are stored at indices [i?2*set[i-1]:0, 2*set[i]) in the indices array.
This function is potentially slow. Consider caching the (static) result instead of retrieving it multiple times.
virtual PxU32 PxClothFabric::getTetherAnchors | ( | PxU32 * | userAnchorBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the tether anchors array to a user specified buffer.
The tether anchors array stores for each particle the index of another particle from which it cannot move further away than specified by the tether lengths array.
- Parameters:
-
[in] userAnchorBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userAnchorBuffer, should be at least getNbTethers().
- Returns:
- getNbTethers() if the copy was successful, 0 otherwise.
- Note:
- This function is potentially slow, consider caching the result instead of retrieving the data multiple times.
- See also:
- getTetherLengths, getNbTethers
virtual PxU32 PxClothFabric::getTetherLengths | ( | PxReal * | userLengthBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the tether lengths array to a user specified buffer.
The tether lengths array stores for each particle how far it is allowed to move away from the particle specified by the tether anchor array.
- Parameters:
-
[in] userLengthBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userLengthBuffer, should be at least getNbTethers().
- Returns:
- getNbTethers() if the copy was successful, 0 otherwise.
- Note:
- This function is potentially slow. Between calling scaleRestlengths(), consider caching the result instead of retrieving it multiple times.
- See also:
- getTetherAnchors, getNbTethers
virtual bool PxClothFabric::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
References PxBase::isKindOf().
virtual void PxClothFabric::release | ( | ) | [pure virtual] |
Decrements the cloth fabric's reference count, and releases it if the new reference count is zero.
- See also:
- PxPhysics.createClothFabric()
Implements PxBase.
virtual void PxClothFabric::scaleRestlengths | ( | PxReal | scale | ) | [pure virtual] |
Scale all rest values of length dependent constraints.
- Parameters:
-
[in] scale The scale factor to multiply each rest value with.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com