![]() |
dotmailer SDK
Tools to access and manage an account in the dotMailer system
|
Campaign Factory - Provides functions to manage campaigns, including sending them to address books and contacts, and accessing email stats. More...
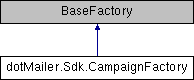
Public Member Functions | |
DmAddressBookCollection | ListAddressBooksForCampaign (int campaignId) |
Returns an AddressBook collection interface to a list of the address books that the specified campaign has been sent to | |
DmCampaignContactStatsCollection | ListCampaignClickers (int campaignId, DateTime modifiedSince) |
Returns a list of DmCampaignContactStat for each contact that opened the campaign email | |
DmCampaignContactStatsCollection | ListCampaignClickers (int campaignId) |
Returns a list of DmCampaignContactStat for each contact that opened the campaign email | |
DmCampaignRoiDetailCollection | ListCampaignRoiDetailSinceDate (int campaignId, DateTime sinceDate) |
Returns DmCampaignRoiDetailCollection of ROI details since the specified date for the specified campaign | |
DmCampaignContactActivityCollection | ListCampaignActivitiesSinceDate (int campaignId, DateTime sinceDate) |
Returns a list of contacts that were sent a specified campaign after a certain dateTime. | |
DmCampaignContactActivityCollection | ListCampaignActivities (int campaignId) |
Returns a list of contacts that were sent a specified campaign. | |
DmCampaignCollection | ListCampaigns () |
Returns a list of the campaigns in the dotMailer account | |
DmCampaignOpenerCollection | GetCampaignOpeners (int campaignId) |
Returns a collection of campaign openers for the specified Id | |
SendResult | SendCampaignToAddressBooks (SplitTestSendOptions options, int campaignId, IEnumerable< IDmAddressBook > addressBooks, DateTime sendDate) |
Send the specified campaign to the collection of address books If the campaign is a split-test campaign, then the options are used to determine how the campaign is sent | |
SendResultCollection | SendCampaignToContacts (int campaignId, IEnumerable< IDmContact > contacts) |
Sends the specified campaign to the specified collection of contacts. | |
SendResultCollection | SendCampaignToContacts (int campaignId, IEnumerable< IDmContact > contacts, DateTime sendDate) |
Sends the specified campaign to the specified collection of contacts. | |
void | UpdateSendResult (SendResult result) |
Takes the specified send result, and polls the webservice for an update of the status. | |
SendResult | UpdateSendResult (ImportGuid apiSendResultGuid) |
takes the specified send result guid, and polls the webservice for an update of the status | |
void | UpdateSendResult (SendResultCollection results) |
takes the specified send result collection, and polls the webservice for an update of the status | |
bool | SetCampaignCustomFromAddress (int campaignId, int fromAddressId) |
Sets the custom from address for the specified campaign | |
DmCampaign | GetCampaign (int campaignId) |
Returns that campaign that matches the specified Id | |
DmCampaignCollection | GetCampaign (string campaignName) |
Returns a list of campaigns that match the specified name | |
bool | UpdateCampaign (IDmCampaign iCampaign) |
Updates the campaign | |
DmCampaign | CreateCampaign (IDmCampaign iCampaign) |
Creates a new campaign | |
DmContactCollection | ListContactsHardBounced (int campaignId) |
Returns a list of the contacts that bounce for the specified campaign | |
DmCampaignCollection | ListSentCampaignsWithActivitySinceDate (DateTime sinceDate) |
Returns a collection of campaigns that have shown activity since the specified date | |
DmCampaign | CreateNewDmCampaign () |
Creates a new DmCampaign Object | |
DmCampaign | CreateNewDmCampaign (IDmCampaign campaignInterface) |
Creates a new DmCampaign Object, from an existing IDmCampaign Instance | |
DmCampaignPageViewCollection | GetCampaignPageViewsSinceDate (int campaignId, DateTime sinceDate) |
Gets a collection of page views for the specified campaign, since the specified date | |
DmCampaignSocialBookmarkCollection | GetCampaignSocialBookmarks (int campaignId) |
Gets the social bookmarks for the specified campaign | |
DmCampaignSummary | GetCampaignSummary (int campaignId) |
Get the campaign summary for the specified campaign | |
DmCampaign | CopyCampaign (IDmCampaign campaignToCopy) |
Used to duplicate an existing campaign in your dotMailer account including the campaign's dynamic content. | |
void | DetachDocumentFromCampaign (int campaignId, int documentId) |
Removes an attachment from a campaign. | |
void | DetachDocumentFromCampaign (IDmCampaign campaign, IDmDocument document) |
Removes an attachment from a campaign. | |
void | AttachDocumentToCampaign (int campaignId, int documentId) |
Attaches a previously uploaded document to a campaign. | |
void | AttachDocumentToCampaign (IDmCampaign campaign, IDmDocument document) |
Attaches a previously uploaded document to a campaign. | |
DmDocumentCollection | ListCampaignAttachments (int campaignId) |
Returns a list of documents attached to the specified campaign. | |
Detailed Description
Campaign Factory - Provides functions to manage campaigns, including sending them to address books and contacts, and accessing email stats.
Member Function Documentation
void dotMailer.Sdk.CampaignFactory.AttachDocumentToCampaign | ( | int | campaignId, |
int | documentId | ||
) |
Attaches a previously uploaded document to a campaign.
- Parameters
-
campaignId The ID of the campaign that you wish to attach the document to. documentId The ID of the document that you wish to attach.
void dotMailer.Sdk.CampaignFactory.AttachDocumentToCampaign | ( | IDmCampaign | campaign, |
IDmDocument | document | ||
) |
Attaches a previously uploaded document to a campaign.
- Parameters
-
campaign The campaign that you wish to attach the document to. document The document that you wish to attach.
DmCampaign dotMailer.Sdk.CampaignFactory.CopyCampaign | ( | IDmCampaign | campaignToCopy | ) |
Used to duplicate an existing campaign in your dotMailer account including the campaign's dynamic content.
- Parameters
-
campaignToCopy The campaign to copy.
- Returns
- A copy of the campaign passed.
DmCampaign dotMailer.Sdk.CampaignFactory.CreateCampaign | ( | IDmCampaign | iCampaign | ) |
Creates a new campaign
- Parameters
-
iCampaign Interface to the campaign information
- Returns
- DmCampaign
- Exceptions
-
DmException
DmCampaign dotMailer.Sdk.CampaignFactory.CreateNewDmCampaign | ( | ) |
Creates a new DmCampaign Object
- Returns
- DmAddressBook
DmCampaign dotMailer.Sdk.CampaignFactory.CreateNewDmCampaign | ( | IDmCampaign | campaignInterface | ) |
Creates a new DmCampaign Object, from an existing IDmCampaign Instance
- Returns
- DmAddressBook
void dotMailer.Sdk.CampaignFactory.DetachDocumentFromCampaign | ( | int | campaignId, |
int | documentId | ||
) |
Removes an attachment from a campaign.
- Parameters
-
campaignId The ID of the campaign that you wish to remove the attachment from. documentId The ID of the document that you wish to remove.
void dotMailer.Sdk.CampaignFactory.DetachDocumentFromCampaign | ( | IDmCampaign | campaign, |
IDmDocument | document | ||
) |
Removes an attachment from a campaign.
- Parameters
-
campaign The campaign that you wish to remove the attachment from. document The document that you wish to remove.
DmCampaign dotMailer.Sdk.CampaignFactory.GetCampaign | ( | int | campaignId | ) |
Returns that campaign that matches the specified Id
- Parameters
-
campaignId the id of the campaign of interest
- Returns
- Exceptions
-
DmException
DmCampaignCollection dotMailer.Sdk.CampaignFactory.GetCampaign | ( | string | campaignName | ) |
Returns a list of campaigns that match the specified name
- Parameters
-
campaignName the name of the campaign to retrieve
- Returns
- DmCampaignCollection
- Exceptions
-
DmException
DmCampaignOpenerCollection dotMailer.Sdk.CampaignFactory.GetCampaignOpeners | ( | int | campaignId | ) |
Returns a collection of campaign openers for the specified Id
- Parameters
-
campaignId the id of the campaign on interest
- Returns
- DmCampaignOpenerCollection
- Exceptions
-
DmException
DmCampaignPageViewCollection dotMailer.Sdk.CampaignFactory.GetCampaignPageViewsSinceDate | ( | int | campaignId, |
DateTime | sinceDate | ||
) |
Gets a collection of page views for the specified campaign, since the specified date
- Parameters
-
campaignId the id of the campaign sinceDate the start date
- Returns
- DmCampaignPageViewCollection
DmCampaignSocialBookmarkCollection dotMailer.Sdk.CampaignFactory.GetCampaignSocialBookmarks | ( | int | campaignId | ) |
Gets the social bookmarks for the specified campaign
- Parameters
-
campaignId campaign to retrieve bookmarks for
- Returns
- DmCampaignSocialBookmarkCollection
DmCampaignSummary dotMailer.Sdk.CampaignFactory.GetCampaignSummary | ( | int | campaignId | ) |
Get the campaign summary for the specified campaign
- Parameters
-
campaignId the id of the campaign
- Returns
- DmCampaignSummary
DmAddressBookCollection dotMailer.Sdk.CampaignFactory.ListAddressBooksForCampaign | ( | int | campaignId | ) |
Returns an AddressBook collection interface to a list of the address books that the specified campaign has been sent to
- Parameters
-
campaignId Id of the campaign
- Returns
DmCampaignContactActivityCollection dotMailer.Sdk.CampaignFactory.ListCampaignActivities | ( | int | campaignId | ) |
Returns a list of contacts that were sent a specified campaign.
- Returns
DmCampaignContactActivityCollection dotMailer.Sdk.CampaignFactory.ListCampaignActivitiesSinceDate | ( | int | campaignId, |
DateTime | sinceDate | ||
) |
Returns a list of contacts that were sent a specified campaign after a certain dateTime.
- Returns
DmDocumentCollection dotMailer.Sdk.CampaignFactory.ListCampaignAttachments | ( | int | campaignId | ) |
Returns a list of documents attached to the specified campaign.
- Parameters
-
campaignId The campaign for which a list of attachments is required.
- Returns
DmCampaignContactStatsCollection dotMailer.Sdk.CampaignFactory.ListCampaignClickers | ( | int | campaignId, |
DateTime | modifiedSince | ||
) |
Returns a list of DmCampaignContactStat for each contact that opened the campaign email
- Parameters
-
campaignId id of campaign modifiedSince filter results since specified date
- Returns
DmCampaignContactStatsCollection dotMailer.Sdk.CampaignFactory.ListCampaignClickers | ( | int | campaignId | ) |
Returns a list of DmCampaignContactStat for each contact that opened the campaign email
- Returns
- Exceptions
-
DmException
DmCampaignRoiDetailCollection dotMailer.Sdk.CampaignFactory.ListCampaignRoiDetailSinceDate | ( | int | campaignId, |
DateTime | sinceDate | ||
) |
Returns DmCampaignRoiDetailCollection of ROI details since the specified date for the specified campaign
- Parameters
-
campaignId the id of the campaign of interest sinceDate the date to start looking from
- Returns
- DmCampaignRoiDetailCollection
- Exceptions
-
DmException
DmCampaignCollection dotMailer.Sdk.CampaignFactory.ListCampaigns | ( | ) |
Returns a list of the campaigns in the dotMailer account
- Returns
- DmCampaignCollection
- Exceptions
-
DmException
DmContactCollection dotMailer.Sdk.CampaignFactory.ListContactsHardBounced | ( | int | campaignId | ) |
Returns a list of the contacts that bounce for the specified campaign
- Parameters
-
campaignId The id of the campaign
- Returns
- DmContactCollection
- Exceptions
-
DmException
DmCampaignCollection dotMailer.Sdk.CampaignFactory.ListSentCampaignsWithActivitySinceDate | ( | DateTime | sinceDate | ) |
Returns a collection of campaigns that have shown activity since the specified date
- Parameters
-
sinceDate the start date
- Returns
- DmCampaignCollection
- Exceptions
-
DmException
SendResult dotMailer.Sdk.CampaignFactory.SendCampaignToAddressBooks | ( | SplitTestSendOptions | options, |
int | campaignId, | ||
IEnumerable< IDmAddressBook > | addressBooks, | ||
DateTime | sendDate | ||
) |
Send the specified campaign to the collection of address books If the campaign is a split-test campaign, then the options are used to determine how the campaign is sent
- Parameters
-
options Send Options for split test sending campaignId The id of the campaign to send addressBooks collection of address books sendDate The date/time to start sending
- Returns
- Collection of send results
SendResultCollection dotMailer.Sdk.CampaignFactory.SendCampaignToContacts | ( | int | campaignId, |
IEnumerable< IDmContact > | contacts | ||
) |
Sends the specified campaign to the specified collection of contacts.
- Parameters
-
campaignId the id of the campaign to send contacts collection of contacts
- Returns
- Send Result Collection
SendResultCollection dotMailer.Sdk.CampaignFactory.SendCampaignToContacts | ( | int | campaignId, |
IEnumerable< IDmContact > | contacts, | ||
DateTime | sendDate | ||
) |
Sends the specified campaign to the specified collection of contacts.
- Parameters
-
campaignId the id of the campaign to send contacts collection of contacts sendDate the date/time to send the campaign
- Returns
- Send Result Collection
bool dotMailer.Sdk.CampaignFactory.SetCampaignCustomFromAddress | ( | int | campaignId, |
int | fromAddressId | ||
) |
Sets the custom from address for the specified campaign
- Parameters
-
campaignId The id of the campaign fromAddressId the id of the custom from address
- Exceptions
-
DmException
bool dotMailer.Sdk.CampaignFactory.UpdateCampaign | ( | IDmCampaign | iCampaign | ) |
Updates the campaign
- Parameters
-
iCampaign Interface to the campaign information
- Returns
- true if the campaign was updated
- Exceptions
-
DmException
void dotMailer.Sdk.CampaignFactory.UpdateSendResult | ( | SendResult | result | ) |
Takes the specified send result, and polls the webservice for an update of the status.
- Parameters
-
result The SendResult
SendResult dotMailer.Sdk.CampaignFactory.UpdateSendResult | ( | ImportGuid | apiSendResultGuid | ) |
takes the specified send result guid, and polls the webservice for an update of the status
- Parameters
-
apiSendResultGuid The SendResult api Guid
void dotMailer.Sdk.CampaignFactory.UpdateSendResult | ( | SendResultCollection | results | ) |
takes the specified send result collection, and polls the webservice for an update of the status
- Parameters
-
results The SendResult Collection
Generated by
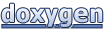