STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_sram.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to drive the 00006 * IS61WV102416BLL-10MLI SRAM memory mounted on STM32L4R9I-EVAL board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive the IS61WV102416BLL-10MLI SRAM external memory mounted 00013 on STM32L4R9I-EVAL evaluation board. 00014 00015 (#) This driver does not need a specific component driver for the SRAM device 00016 to be included with. 00017 00018 (#) Initialization steps: 00019 (++) Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00020 function includes the MSP layer hardware resources initialization and the 00021 FMC controller configuration to interface with the external SRAM memory. 00022 00023 (#) SRAM read/write operations 00024 (++) SRAM external memory can be accessed with read/write operations once it is 00025 initialized. 00026 Read/write operation can be performed with AHB access using the functions 00027 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00028 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00029 (++) The AHB access is performed with 16-bit width transaction, the DMA transfer 00030 configuration is fixed at single (no burst) halfword transfer 00031 (see the SRAM_MspInit() static function). 00032 (++) User can implement his own functions for read/write access with his desired 00033 configurations. 00034 (++) If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00035 is called in IRQ handler file, to serve the generated interrupt once the DMA 00036 transfer is complete. 00037 @endverbatim 00038 ****************************************************************************** 00039 * @attention 00040 * 00041 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00042 * 00043 * Redistribution and use in source and binary forms, with or without modification, 00044 * are permitted provided that the following conditions are met: 00045 * 1. Redistributions of source code must retain the above copyright notice, 00046 * this list of conditions and the following disclaimer. 00047 * 2. Redistributions in binary form must reproduce the above copyright notice, 00048 * this list of conditions and the following disclaimer in the documentation 00049 * and/or other materials provided with the distribution. 00050 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00051 * may be used to endorse or promote products derived from this software 00052 * without specific prior written permission. 00053 * 00054 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00055 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00056 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00057 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00058 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00059 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00060 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00061 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00062 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00063 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00064 * 00065 ****************************************************************************** 00066 */ 00067 00068 /* Includes ------------------------------------------------------------------*/ 00069 #include "stm32l4r9i_eval_sram.h" 00070 00071 /** @addtogroup BSP 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32L4R9I_EVAL 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM32L4R9I_EVAL_SRAM STM32L4R9I_EVAL SRAM 00080 * @{ 00081 */ 00082 00083 00084 /* Private variables ---------------------------------------------------------*/ 00085 00086 /** @defgroup STM32L4R9I_EVAL_SRAM_Private_Variables Private Variables 00087 * @{ 00088 */ 00089 static SRAM_HandleTypeDef sramHandle; 00090 static FMC_NORSRAM_TimingTypeDef Timing; 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /* Private macros ------------------------------------------------------------*/ 00097 /* Private constants ---------------------------------------------------------*/ 00098 00099 /** @defgroup STM32L4R9I_EVAL_SRAM_Private_Constants Private Constants 00100 * @{ 00101 */ 00102 /* Timings for SRAM IS61WV102416BLL-10MLI */ 00103 #define SRAM_ADDR_SETUP_TIME 2 /* 10ns with a clock at 120 MHz (period of 8.33 ns) */ 00104 #define SRAM_DATA_SETUP_TIME 1 /* 6ns with a clock at 120 MHz (period of 8.33 ns) */ 00105 #define SRAM_DATA_HOLD_TIME 0 00106 #define SRAM_TURN_AROUND_TIME 1 /* 4ns with a clock at 120 MHz (period of 8.33 ns) */ 00107 00108 /** 00109 * @} 00110 */ 00111 00112 /* Private function prototypes -----------------------------------------------*/ 00113 00114 /** @defgroup STM32L4R9I_EVAL_SRAM_Private_Functions Private Functions 00115 * @{ 00116 */ 00117 static void SRAM_MspInit(void); 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /* Private functions ---------------------------------------------------------*/ 00124 00125 /** @defgroup STM32L4R9I_EVAL_SRAM_Exported_Functions Exported Functions 00126 * @{ 00127 */ 00128 00129 /** 00130 * @brief Initializes the SRAM device. 00131 * @retval SRAM status 00132 */ 00133 uint8_t BSP_SRAM_Init(void) 00134 { 00135 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00136 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00137 00138 /* SRAM device configuration */ 00139 Timing.AddressSetupTime = SRAM_ADDR_SETUP_TIME; 00140 Timing.AddressHoldTime = 1; /* Min value, Don't care on SRAM Access mode A */ 00141 Timing.DataSetupTime = SRAM_DATA_SETUP_TIME; 00142 Timing.DataHoldTime = SRAM_DATA_HOLD_TIME; 00143 Timing.CLKDivision = 2; /* Min value, Don't care on SRAM Access mode A */ 00144 Timing.DataLatency = 2; /* Min value, Don't care on SRAM Access mode A */ 00145 Timing.BusTurnAroundDuration = SRAM_TURN_AROUND_TIME; 00146 Timing.AccessMode = FMC_ACCESS_MODE_A; 00147 00148 sramHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00149 sramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00150 sramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00151 sramHandle.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00152 sramHandle.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00153 sramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00154 sramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00155 sramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00156 sramHandle.Init.WriteBurst = FMC_WRITE_BURST_DISABLE; 00157 sramHandle.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00158 sramHandle.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00159 sramHandle.Init.NBLSetupTime = 0; 00160 sramHandle.Init.PageSize = FMC_PAGE_SIZE_NONE; 00161 00162 /* SRAM controller initialization */ 00163 SRAM_MspInit(); 00164 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00165 { 00166 return SRAM_ERROR; 00167 } 00168 else 00169 { 00170 return SRAM_OK; 00171 } 00172 } 00173 00174 /** 00175 * @brief Reads an amount of data from the SRAM device in polling mode. 00176 * @param uwStartAddress: Read start address 00177 * @param pData: Pointer to data to be read 00178 * @param uwDataSize: Size of read data from the memory 00179 * @retval SRAM status 00180 */ 00181 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00182 { 00183 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00184 { 00185 return SRAM_ERROR; 00186 } 00187 else 00188 { 00189 return SRAM_OK; 00190 } 00191 } 00192 00193 /** 00194 * @brief Reads an amount of data from the SRAM device in DMA mode. 00195 * @param uwStartAddress: Read start address 00196 * @param pData: Pointer to data to be read 00197 * @param uwDataSize: Size of read data from the memory 00198 * @retval SRAM status 00199 */ 00200 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00201 { 00202 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00203 { 00204 return SRAM_ERROR; 00205 } 00206 else 00207 { 00208 return SRAM_OK; 00209 } 00210 } 00211 00212 /** 00213 * @brief Writes an amount of data from the SRAM device in polling mode. 00214 * @param uwStartAddress: Write start address 00215 * @param pData: Pointer to data to be written 00216 * @param uwDataSize: Size of written data from the memory 00217 * @retval SRAM status 00218 */ 00219 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00220 { 00221 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00222 { 00223 return SRAM_ERROR; 00224 } 00225 else 00226 { 00227 return SRAM_OK; 00228 } 00229 } 00230 00231 /** 00232 * @brief Writes an amount of data from the SRAM device in DMA mode. 00233 * @param uwStartAddress: Write start address 00234 * @param pData: Pointer to data to be written 00235 * @param uwDataSize: Size of written data from the memory 00236 * @retval SRAM status 00237 */ 00238 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00239 { 00240 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00241 { 00242 return SRAM_ERROR; 00243 } 00244 else 00245 { 00246 return SRAM_OK; 00247 } 00248 } 00249 00250 /** 00251 * @brief Handles SRAM DMA transfer interrupt request. 00252 * @retval None 00253 */ 00254 void BSP_SRAM_DMA_IRQHandler(void) 00255 { 00256 HAL_DMA_IRQHandler(sramHandle.hdma); 00257 } 00258 00259 /** @addtogroup STM32L476G_EVAL_SRAM_Private_Functions 00260 * @{ 00261 */ 00262 00263 /** 00264 * @brief Initializes SRAM MSP. 00265 * @retval None 00266 */ 00267 static void SRAM_MspInit(void) 00268 { 00269 static DMA_HandleTypeDef dmaHandle; 00270 GPIO_InitTypeDef gpioinitstruct; 00271 SRAM_HandleTypeDef *hsram = &sramHandle; 00272 00273 /* Enable FMC clock */ 00274 __HAL_RCC_FMC_CLK_ENABLE(); 00275 00276 /* Enable chosen DMAx clock */ 00277 SRAM_DMAx_CLK_ENABLE(); 00278 00279 /* Enable GPIOs clock */ 00280 __HAL_RCC_GPIOD_CLK_ENABLE(); 00281 __HAL_RCC_GPIOE_CLK_ENABLE(); 00282 __HAL_RCC_GPIOF_CLK_ENABLE(); 00283 __HAL_RCC_GPIOG_CLK_ENABLE(); 00284 __HAL_RCC_PWR_CLK_ENABLE(); 00285 HAL_PWREx_EnableVddIO2(); 00286 00287 /* Common GPIO configuration */ 00288 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00289 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00290 gpioinitstruct.Alternate = GPIO_AF12_FMC; 00291 00292 /*## NE configuration #######*/ 00293 /* NE1 : SRAM */ 00294 gpioinitstruct.Pull = GPIO_PULLUP; 00295 gpioinitstruct.Pin = GPIO_PIN_7; 00296 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00297 /* NE3 : NOR */ 00298 /* NE4 : TFT LCD */ 00299 gpioinitstruct.Pin = GPIO_PIN_10 | GPIO_PIN_12; 00300 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00301 00302 /*## NOE and NWE configuration #######*/ 00303 gpioinitstruct.Pin = GPIO_PIN_4 | GPIO_PIN_5; 00304 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00305 00306 /*## NBL0, NBL1 configuration #######*/ 00307 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1; 00308 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00309 00310 /*## Address Bus #######*/ 00311 /* GPIOD configuration */ 00312 gpioinitstruct.Pull = GPIO_NOPULL; 00313 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00314 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00315 00316 /* GPIOE configuration */ 00317 gpioinitstruct.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00318 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00319 00320 /* GPIOF configuration */ 00321 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00322 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00323 GPIO_PIN_14 | GPIO_PIN_15; 00324 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00325 00326 /* GPIOG configuration */ 00327 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00328 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00329 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00330 00331 /*## Data Bus #######*/ 00332 /* GPIOD configuration */ 00333 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00334 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00335 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00336 00337 /* GPIOE configuration */ 00338 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00339 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00340 GPIO_PIN_14 | GPIO_PIN_15; 00341 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00342 00343 /* Configure common DMA parameters */ 00344 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00345 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00346 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00347 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00348 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00349 dmaHandle.Init.Mode = DMA_NORMAL; 00350 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00351 00352 dmaHandle.Instance = SRAM_DMAx_CHANNEL; 00353 00354 /* Associate the DMA handle */ 00355 __HAL_LINKDMA(hsram, hdma, dmaHandle); 00356 00357 /* Deinitialize the DMA channel for new transfer */ 00358 HAL_DMA_DeInit(&dmaHandle); 00359 00360 /* Configure the DMA channel */ 00361 HAL_DMA_Init(&dmaHandle); 00362 00363 /* NVIC configuration for DMA transfer complete interrupt */ 00364 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 5, 0); 00365 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00366 } 00367 00368 /** 00369 * @} 00370 */ 00371 00372 /** 00373 * @} 00374 */ 00375 00376 /** 00377 * @} 00378 */ 00379 00380 /** 00381 * @} 00382 */ 00383 00384 /** 00385 * @} 00386 */ 00387 00388 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
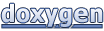