STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_rgb_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_rgb_ts.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the Touch 00006 * Screen on STM32746G-Discovery board. 00007 @verbatim 00008 1. How To use this driver: 00009 -------------------------- 00010 - This driver is used to drive the touch screen module of the STM32L4R9I_EVAL 00011 board on the RK043FN48H-CT672B 480x272 LCD screen with capacitive touch screen. 00012 - The FT5336 component driver must be included in project files according to 00013 the touch screen driver present on this board. 00014 00015 2. Driver description: 00016 --------------------- 00017 + Initialization steps: 00018 o Initialize the TS module using the BSP_RGB_TS_Init() function. This 00019 function includes the MSP layer hardware resources initialization and the 00020 communication layer configuration to start the TS use. The LCD size properties 00021 (x and y) are passed as parameters. 00022 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00023 by calling the function BSP_RGB_TS_ITConfig(). The TS interrupt mode is generated 00024 as an external interrupt whenever a touch is detected. 00025 The interrupt mode internally uses the IO functionalities driver driven by 00026 the IO expander, to configure the IT line. 00027 00028 + Touch screen use 00029 o The touch screen state is captured whenever the function BSP_RGB_TS_GetState() is 00030 used. This function returns information about the last LCD touch occurred 00031 in the TS_StateTypeDef structure. 00032 o If TS interrupt mode is used, the function BSP_RGB_TS_ITGetStatus() is needed to get 00033 the interrupt status. To clear the IT pending bits, you should call the 00034 function BSP_RGB_TS_ITClear(). 00035 o The IT is handled using the corresponding external interrupt IRQ handler, 00036 the user IT callback treatment is implemented on the same external interrupt 00037 callback. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Includes ------------------------------------------------------------------*/ 00070 #include "stm32l4r9i_eval_ts.h" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32L4R9I_EVAL 00077 * @{ 00078 */ 00079 00080 #if defined(USE_ROCKTECH_480x272) 00081 00082 /** @defgroup STM32L4R9I_EVAL_RGB_TS STM32L4R9I_EVAL RGB TS 00083 * @{ 00084 */ 00085 00086 /** @defgroup STM32L4R9I_EVAL_RGB_TS_Private_Variables Private Variables 00087 * @{ 00088 */ 00089 static TS_DrvTypeDef *tsDriver; 00090 static uint16_t tsXBoundary, tsYBoundary; 00091 static uint8_t tsOrientation; 00092 static uint8_t I2cAddress; 00093 /** 00094 * @} 00095 */ 00096 00097 /** @defgroup STM32L4R9I_EVAL_RGB_TS_Exported_Functions Exported Functions 00098 * @{ 00099 */ 00100 00101 /** 00102 * @brief Initializes and configures the touch screen functionalities and 00103 * configures all necessary hardware resources (GPIOs, I2C, clocks..). 00104 * @param ts_SizeX: Maximum X size of the TS area on LCD 00105 * @param ts_SizeY: Maximum Y size of the TS area on LCD 00106 * @retval TS_OK if all initializations are OK. Other value if error. 00107 */ 00108 uint8_t BSP_RGB_TS_Init(uint16_t ts_SizeX, uint16_t ts_SizeY) 00109 { 00110 uint8_t status = TS_OK; 00111 tsXBoundary = ts_SizeX; 00112 tsYBoundary = ts_SizeY; 00113 00114 /* Read ID and verify if the touch screen driver is ready */ 00115 ft5336_ts_drv.Init(TS_RGB_I2C_ADDRESS); 00116 if(ft5336_ts_drv.ReadID(TS_RGB_I2C_ADDRESS) == FT5336_ID_VALUE) 00117 { 00118 /* Initialize the TS driver structure */ 00119 tsDriver = &ft5336_ts_drv; 00120 I2cAddress = TS_RGB_I2C_ADDRESS; 00121 tsOrientation = TS_SWAP_XY; 00122 00123 /* Initialize the TS driver */ 00124 tsDriver->Start(I2cAddress); 00125 } 00126 else 00127 { 00128 status = TS_DEVICE_NOT_FOUND; 00129 } 00130 00131 return status; 00132 } 00133 00134 /** 00135 * @brief DeInitializes the TouchScreen. 00136 * @retval TS state 00137 */ 00138 uint8_t BSP_RGB_TS_DeInit(void) 00139 { 00140 /* Actually ts_driver does not provide a DeInit function */ 00141 return TS_OK; 00142 } 00143 00144 /** 00145 * @brief Configures and enables the touch screen interrupts. 00146 * @retval TS_OK if all initializations are OK. Other value if error. 00147 */ 00148 uint8_t BSP_RGB_TS_ITConfig(void) 00149 { 00150 GPIO_InitTypeDef gpio_init_structure; 00151 00152 /* Configure Interrupt mode for TS detection pin */ 00153 gpio_init_structure.Pin = TS_RGB_INT_PIN; 00154 gpio_init_structure.Pull = GPIO_NOPULL; 00155 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00156 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00157 HAL_GPIO_Init(TS_RGB_INT_GPIO_PORT, &gpio_init_structure); 00158 00159 /* Enable and set Touch screen EXTI Interrupt to the lowest priority */ 00160 HAL_NVIC_SetPriority((IRQn_Type)(TS_RGB_INT_EXTI_IRQn), 0x0F, 0x00); 00161 HAL_NVIC_EnableIRQ((IRQn_Type)(TS_RGB_INT_EXTI_IRQn)); 00162 00163 /* Enable the TS ITs */ 00164 tsDriver->EnableIT(I2cAddress); 00165 00166 return TS_OK; 00167 } 00168 00169 /** 00170 * @brief Gets the touch screen interrupt status. 00171 * @retval TS_OK if all initializations are OK. Other value if error. 00172 */ 00173 uint8_t BSP_RGB_TS_ITGetStatus(void) 00174 { 00175 /* Return the TS IT status */ 00176 return (tsDriver->GetITStatus(I2cAddress)); 00177 } 00178 00179 /** 00180 * @brief Returns status and positions of the touch screen. 00181 * @param TS_State: Pointer to touch screen current state structure 00182 * @retval TS_OK if all initializations are OK. Other value if error. 00183 */ 00184 uint8_t BSP_RGB_TS_GetState(TS_StateTypeDef *TS_State) 00185 { 00186 static uint32_t _x[TS_MAX_NB_TOUCH] = {0, 0}; 00187 static uint32_t _y[TS_MAX_NB_TOUCH] = {0, 0}; 00188 uint8_t ts_status = TS_OK; 00189 uint16_t x[TS_MAX_NB_TOUCH]; 00190 uint16_t y[TS_MAX_NB_TOUCH]; 00191 uint16_t brute_x[TS_MAX_NB_TOUCH]; 00192 uint16_t brute_y[TS_MAX_NB_TOUCH]; 00193 uint16_t x_diff; 00194 uint16_t y_diff; 00195 uint32_t index; 00196 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00197 uint32_t weight = 0; 00198 uint32_t area = 0; 00199 uint32_t event = 0; 00200 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00201 00202 /* Check and update the number of touches active detected */ 00203 TS_State->touchDetected = tsDriver->DetectTouch(I2cAddress); 00204 00205 if(TS_State->touchDetected) 00206 { 00207 for(index=0; index < TS_State->touchDetected; index++) 00208 { 00209 /* Get each touch coordinates */ 00210 tsDriver->GetXY(I2cAddress, &(brute_x[index]), &(brute_y[index])); 00211 00212 if(tsOrientation == TS_SWAP_NONE) 00213 { 00214 x[index] = brute_x[index]; 00215 y[index] = brute_y[index]; 00216 } 00217 00218 if(tsOrientation & TS_SWAP_X) 00219 { 00220 x[index] = 4096 - brute_x[index]; 00221 } 00222 00223 if(tsOrientation & TS_SWAP_Y) 00224 { 00225 y[index] = 4096 - brute_y[index]; 00226 } 00227 00228 if(tsOrientation & TS_SWAP_XY) 00229 { 00230 y[index] = brute_x[index]; 00231 x[index] = brute_y[index]; 00232 } 00233 00234 x_diff = x[index] > _x[index]? (x[index] - _x[index]): (_x[index] - x[index]); 00235 y_diff = y[index] > _y[index]? (y[index] - _y[index]): (_y[index] - y[index]); 00236 00237 if ((x_diff + y_diff) > 5) 00238 { 00239 _x[index] = x[index]; 00240 _y[index] = y[index]; 00241 } 00242 00243 if(I2cAddress == FT5336_I2C_SLAVE_ADDRESS) 00244 { 00245 TS_State->touchX[index] = x[index]; 00246 TS_State->touchY[index] = y[index]; 00247 } 00248 else 00249 { 00250 /* 2^12 = 4096 : indexes are expressed on a dynamic of 4096 */ 00251 TS_State->touchX[index] = (tsXBoundary * _x[index]) >> 12; 00252 TS_State->touchY[index] = (tsYBoundary * _y[index]) >> 12; 00253 } 00254 00255 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00256 00257 /* Get touch info related to the current touch */ 00258 ft5336_TS_GetTouchInfo(I2cAddress, index, &weight, &area, &event); 00259 00260 /* Update TS_State structure */ 00261 TS_State->touchWeight[index] = weight; 00262 TS_State->touchArea[index] = area; 00263 00264 /* Remap touch event */ 00265 switch(event) 00266 { 00267 case FT5336_TOUCH_EVT_FLAG_PRESS_DOWN : 00268 TS_State->touchEventId[index] = TOUCH_EVENT_PRESS_DOWN; 00269 break; 00270 case FT5336_TOUCH_EVT_FLAG_LIFT_UP : 00271 TS_State->touchEventId[index] = TOUCH_EVENT_LIFT_UP; 00272 break; 00273 case FT5336_TOUCH_EVT_FLAG_CONTACT : 00274 TS_State->touchEventId[index] = TOUCH_EVENT_CONTACT; 00275 break; 00276 case FT5336_TOUCH_EVT_FLAG_NO_EVENT : 00277 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00278 break; 00279 default : 00280 ts_status = TS_ERROR; 00281 break; 00282 } /* of switch(event) */ 00283 00284 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00285 00286 } /* of for(index=0; index < TS_State->touchDetected; index++) */ 00287 00288 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00289 /* Get gesture Id */ 00290 ts_status = BSP_RGB_TS_Get_GestureId(TS_State); 00291 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00292 00293 } /* end of if(TS_State->touchDetected != 0) */ 00294 00295 return (ts_status); 00296 } 00297 00298 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00299 /** 00300 * @brief Update gesture Id following a touch detected. 00301 * @param TS_State: Pointer to touch screen current state structure 00302 * @retval TS_OK if all initializations are OK. Other value if error. 00303 */ 00304 uint8_t BSP_RGB_TS_Get_GestureId(TS_StateTypeDef *TS_State) 00305 { 00306 uint32_t gestureId = 0; 00307 uint8_t ts_status = TS_OK; 00308 00309 /* Get gesture Id */ 00310 ft5336_TS_GetGestureID(I2cAddress, &gestureId); 00311 00312 /* Remap gesture Id to a TS_GestureIdTypeDef value */ 00313 switch(gestureId) 00314 { 00315 case FT5336_GEST_ID_NO_GESTURE : 00316 TS_State->gestureId = GEST_ID_NO_GESTURE; 00317 break; 00318 case FT5336_GEST_ID_MOVE_UP : 00319 TS_State->gestureId = GEST_ID_MOVE_UP; 00320 break; 00321 case FT5336_GEST_ID_MOVE_RIGHT : 00322 TS_State->gestureId = GEST_ID_MOVE_RIGHT; 00323 break; 00324 case FT5336_GEST_ID_MOVE_DOWN : 00325 TS_State->gestureId = GEST_ID_MOVE_DOWN; 00326 break; 00327 case FT5336_GEST_ID_MOVE_LEFT : 00328 TS_State->gestureId = GEST_ID_MOVE_LEFT; 00329 break; 00330 case FT5336_GEST_ID_ZOOM_IN : 00331 TS_State->gestureId = GEST_ID_ZOOM_IN; 00332 break; 00333 case FT5336_GEST_ID_ZOOM_OUT : 00334 TS_State->gestureId = GEST_ID_ZOOM_OUT; 00335 break; 00336 default : 00337 ts_status = TS_ERROR; 00338 break; 00339 } /* of switch(gestureId) */ 00340 00341 return(ts_status); 00342 } 00343 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00344 00345 /** 00346 * @brief Clears all touch screen interrupts. 00347 */ 00348 void BSP_RGB_TS_ITClear(void) 00349 { 00350 /* Clear TS IT pending bits */ 00351 tsDriver->ClearIT(I2cAddress); 00352 } 00353 00354 /** 00355 * @brief Function used to reset all touch data before a new acquisition 00356 * of touch information. 00357 * @param TS_State: Pointer to touch screen current state structure 00358 * @retval TS_OK if OK, TE_ERROR if problem found. 00359 */ 00360 uint8_t BSP_RGB_TS_ResetTouchData(TS_StateTypeDef *TS_State) 00361 { 00362 uint8_t ts_status = TS_ERROR; 00363 uint32_t index; 00364 00365 if (TS_State != (TS_StateTypeDef *)NULL) 00366 { 00367 TS_State->gestureId = GEST_ID_NO_GESTURE; 00368 TS_State->touchDetected = 0; 00369 00370 for(index = 0; index < TS_MAX_NB_TOUCH; index++) 00371 { 00372 TS_State->touchX[index] = 0; 00373 TS_State->touchY[index] = 0; 00374 TS_State->touchArea[index] = 0; 00375 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00376 TS_State->touchWeight[index] = 0; 00377 } 00378 00379 ts_status = TS_OK; 00380 00381 } /* of if (TS_State != (TS_StateTypeDef *)NULL) */ 00382 00383 return (ts_status); 00384 } 00385 00386 /** 00387 * @} 00388 */ 00389 00390 /** 00391 * @} 00392 */ 00393 00394 #endif /* USE_ROCKTECH_480x272 */ 00395 00396 /** 00397 * @} 00398 */ 00399 00400 /** 00401 * @} 00402 */ 00403 00404 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
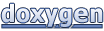