STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_rgb_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_rgb_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00006 * mounted on STM32L4R9I_EVAL board. 00007 @verbatim 00008 1. How To use this driver: 00009 -------------------------- 00010 - This driver is used to drive directly an LCD TFT using the LTDC controller. 00011 - This driver uses timing and setting for RK043FN48H LCD. 00012 00013 2. Driver description: 00014 --------------------- 00015 + Initialization steps: 00016 o Initialize the LCD using the BSP_RGB_LCD_Init() function. 00017 o Apply the Layer configuration using the BSP_RGB_LCD_LayerDefaultInit() function. 00018 o Select the LCD layer to be used using the BSP_RGB_LCD_SelectLayer() function. 00019 o Enable the LCD display using the BSP_RGB_LCD_DisplayOn() function. 00020 00021 + Options 00022 o Configure and enable the color keying functionality using the 00023 BSP_RGB_LCD_SetColorKeying() function. 00024 o Modify in the fly the transparency and/or the frame buffer address 00025 using the following functions: 00026 - BSP_RGB_LCD_SetTransparency() 00027 - BSP_RGB_LCD_SetLayerAddress() 00028 00029 + Display on LCD 00030 o Clear the hole LCD using BSP_RGB_LCD_Clear() function or only one specified string 00031 line using the BSP_RGB_LCD_ClearStringLine() function. 00032 o Display a character on the specified line and column using the BSP_RGB_LCD_DisplayChar() 00033 function or a complete string line using the BSP_RGB_LCD_DisplayStringAtLine() function. 00034 o Display a string line on the specified position (x,y in pixel) and align mode 00035 using the BSP_RGB_LCD_DisplayStringAtLine() function. 00036 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00037 on LCD using the available set of functions. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Includes ------------------------------------------------------------------*/ 00070 #include "stm32l4r9i_eval_lcd.h" 00071 #include "../../../Utilities/Fonts/fonts.h" 00072 #include "../../../Utilities/Fonts/font24.c" 00073 #include "../../../Utilities/Fonts/font20.c" 00074 #include "../../../Utilities/Fonts/font16.c" 00075 #include "../../../Utilities/Fonts/font12.c" 00076 #include "../../../Utilities/Fonts/font8.c" 00077 00078 /** @addtogroup BSP 00079 * @{ 00080 */ 00081 00082 /** @addtogroup STM32L4R9I_EVAL 00083 * @{ 00084 */ 00085 00086 /** @defgroup STM32L4R9I_EVAL_LCD STM32L4R9I_EVAL LCD 00087 * @{ 00088 */ 00089 00090 /** @defgroup STM32L4R9I_EVAL_RGB_LCD STM32L4R9I_EVAL RGB LCD 00091 * @{ 00092 */ 00093 00094 #if defined(USE_ROCKTECH_480x272) 00095 00096 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Private_Defines Private Defines 00097 * @{ 00098 */ 00099 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00100 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00101 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Private_Macros Private Macros 00107 * @{ 00108 */ 00109 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Exported_Variables Exported Variables 00115 * @{ 00116 */ 00117 /* DMA2D handle */ 00118 DMA2D_HandleTypeDef hdma2d_eval; 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Private_Variables Private Variables 00124 * @{ 00125 */ 00126 /* Physical frame buffer for active layer */ 00127 uint32_t PhysFrameBuffer[RK043FN48H_HEIGHT * RK043FN48H_WIDTH]; /* 480*272 pixels with 32bpp */ 00128 00129 /* LTDC handle */ 00130 LTDC_HandleTypeDef hltdc_eval; 00131 00132 /* Default LCD configuration with LCD Layer 1 */ 00133 static uint32_t ActiveLayer = LTDC_DEFAULT_ACTIVE_LAYER; 00134 static LCD_DrawPropTypeDef DrawProp[LTDC_MAX_LAYER_NUMBER]; 00135 /** 00136 * @} 00137 */ 00138 00139 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Private_FunctionPrototypes Private Function Prototypes 00140 * @{ 00141 */ 00142 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00143 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00144 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00145 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00146 /** 00147 * @} 00148 */ 00149 00150 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Exported_Functions Exported Functions 00151 * @{ 00152 */ 00153 00154 /** 00155 * @brief Initializes the LCD. 00156 * @retval LCD state 00157 */ 00158 uint8_t BSP_RGB_LCD_Init(void) 00159 { 00160 /* Select the used LCD */ 00161 00162 /* The RK043FN48H LCD 480x272 is selected */ 00163 /* Timing Configuration */ 00164 hltdc_eval.Init.HorizontalSync = (RK043FN48H_HSYNC - 1); 00165 hltdc_eval.Init.VerticalSync = (RK043FN48H_VSYNC - 1); 00166 hltdc_eval.Init.AccumulatedHBP = (RK043FN48H_HSYNC + RK043FN48H_HBP - 1); 00167 hltdc_eval.Init.AccumulatedVBP = (RK043FN48H_VSYNC + RK043FN48H_VBP - 1); 00168 hltdc_eval.Init.AccumulatedActiveH = (RK043FN48H_HEIGHT + RK043FN48H_VSYNC + RK043FN48H_VBP - 1); 00169 hltdc_eval.Init.AccumulatedActiveW = (RK043FN48H_WIDTH + RK043FN48H_HSYNC + RK043FN48H_HBP - 1); 00170 hltdc_eval.Init.TotalHeigh = (RK043FN48H_HEIGHT + RK043FN48H_VSYNC + RK043FN48H_VBP + RK043FN48H_VFP - 1); 00171 hltdc_eval.Init.TotalWidth = (RK043FN48H_WIDTH + RK043FN48H_HSYNC + RK043FN48H_HBP + RK043FN48H_HFP - 1); 00172 00173 /* LCD clock configuration */ 00174 BSP_RGB_LCD_ClockConfig(&hltdc_eval, NULL); 00175 00176 /* Initialize the LCD pixel width and pixel height */ 00177 hltdc_eval.LayerCfg->ImageWidth = RK043FN48H_WIDTH; 00178 hltdc_eval.LayerCfg->ImageHeight = RK043FN48H_HEIGHT; 00179 00180 /* Background value */ 00181 hltdc_eval.Init.Backcolor.Blue = 0; 00182 hltdc_eval.Init.Backcolor.Green = 0; 00183 hltdc_eval.Init.Backcolor.Red = 0; 00184 00185 /* Polarity */ 00186 hltdc_eval.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00187 hltdc_eval.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00188 hltdc_eval.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00189 hltdc_eval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00190 hltdc_eval.Instance = LTDC; 00191 00192 if(HAL_LTDC_GetState(&hltdc_eval) == HAL_LTDC_STATE_RESET) 00193 { 00194 /* Initialize the LCD Msp: this __weak function can be rewritten by the application */ 00195 BSP_RGB_LCD_MspInit(&hltdc_eval, NULL); 00196 } 00197 HAL_LTDC_Init(&hltdc_eval); 00198 00199 /* Screen initialization */ 00200 BSP_IO_WritePin(LCD_RGB_RST_PIN, IO_PIN_RESET ); 00201 HAL_Delay(2); 00202 BSP_IO_WritePin(LCD_RGB_RST_PIN, IO_PIN_SET ); 00203 00204 /* Assert display enable LCD_DISP pin via MFX expander */ 00205 BSP_IO_WritePin(LCD_RGB_DISP_PIN, IO_PIN_SET); 00206 00207 /* Assert backlight LCD_BL_CTRL pin */ 00208 HAL_GPIO_WritePin(LCD_RGB_BL_CTRL_GPIO_PORT, LCD_RGB_BL_CTRL_PIN, GPIO_PIN_SET); 00209 00210 /* Initialize the font */ 00211 BSP_RGB_LCD_SetFont(&LCD_DEFAULT_FONT); 00212 00213 return LCD_OK; 00214 } 00215 00216 /** 00217 * @brief DeInitializes the LCD. 00218 * @retval LCD state 00219 */ 00220 uint8_t BSP_RGB_LCD_DeInit(void) 00221 { 00222 /* Initialize the hltdc_eval Instance parameter */ 00223 hltdc_eval.Instance = LTDC; 00224 00225 /* Disable LTDC block */ 00226 __HAL_LTDC_DISABLE(&hltdc_eval); 00227 00228 /* DeInit the LTDC */ 00229 HAL_LTDC_DeInit(&hltdc_eval); 00230 00231 /* DeInit the LTDC MSP : this __weak function can be rewritten by the application */ 00232 BSP_RGB_LCD_MspDeInit(&hltdc_eval, NULL); 00233 00234 return LCD_OK; 00235 } 00236 00237 /** 00238 * @brief Gets the LCD X size. 00239 * @retval Used LCD X size 00240 */ 00241 uint32_t BSP_RGB_LCD_GetXSize(void) 00242 { 00243 return hltdc_eval.LayerCfg[ActiveLayer].ImageWidth; 00244 } 00245 00246 /** 00247 * @brief Gets the LCD Y size. 00248 * @retval Used LCD Y size 00249 */ 00250 uint32_t BSP_RGB_LCD_GetYSize(void) 00251 { 00252 return hltdc_eval.LayerCfg[ActiveLayer].ImageHeight; 00253 } 00254 00255 /** 00256 * @brief Set the LCD X size. 00257 * @param imageWidthPixels : image width in pixels unit 00258 * @retval None 00259 */ 00260 void BSP_RGB_LCD_SetXSize(uint32_t imageWidthPixels) 00261 { 00262 hltdc_eval.LayerCfg[ActiveLayer].ImageWidth = imageWidthPixels; 00263 } 00264 00265 /** 00266 * @brief Set the LCD Y size. 00267 * @param imageHeightPixels : image height in lines unit 00268 * @retval None 00269 */ 00270 void BSP_RGB_LCD_SetYSize(uint32_t imageHeightPixels) 00271 { 00272 hltdc_eval.LayerCfg[ActiveLayer].ImageHeight = imageHeightPixels; 00273 } 00274 00275 /** 00276 * @brief Initializes the LCD layer in ARGB8888 format (32 bits per pixel). 00277 * @param LayerIndex: Layer foreground or background 00278 * @param FB_Address: Layer frame buffer 00279 * @retval None 00280 */ 00281 void BSP_RGB_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00282 { 00283 LCD_LayerCfgTypeDef layer_cfg; 00284 00285 /* Layer Init */ 00286 layer_cfg.WindowX0 = 0; 00287 layer_cfg.WindowX1 = BSP_RGB_LCD_GetXSize(); 00288 layer_cfg.WindowY0 = 0; 00289 layer_cfg.WindowY1 = BSP_RGB_LCD_GetYSize(); 00290 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00291 layer_cfg.FBStartAdress = FB_Address; 00292 layer_cfg.Alpha = 255; 00293 layer_cfg.Alpha0 = 0; 00294 layer_cfg.Backcolor.Blue = 0; 00295 layer_cfg.Backcolor.Green = 0; 00296 layer_cfg.Backcolor.Red = 0; 00297 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00298 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00299 layer_cfg.ImageWidth = BSP_RGB_LCD_GetXSize(); 00300 layer_cfg.ImageHeight = BSP_RGB_LCD_GetYSize(); 00301 00302 HAL_LTDC_ConfigLayer(&hltdc_eval, &layer_cfg, LayerIndex); 00303 00304 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00305 DrawProp[LayerIndex].pFont = &Font24; 00306 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00307 } 00308 00309 /** 00310 * @brief Initializes the LCD layer in RGB565 format (16 bits per pixel). 00311 * @param LayerIndex: Layer foreground or background 00312 * @param FB_Address: Layer frame buffer 00313 * @retval None 00314 */ 00315 void BSP_RGB_LCD_LayerRgb565Init(uint16_t LayerIndex, uint32_t FB_Address) 00316 { 00317 LCD_LayerCfgTypeDef layer_cfg; 00318 00319 /* Layer Init */ 00320 layer_cfg.WindowX0 = 0; 00321 layer_cfg.WindowX1 = BSP_RGB_LCD_GetXSize(); 00322 layer_cfg.WindowY0 = 0; 00323 layer_cfg.WindowY1 = BSP_RGB_LCD_GetYSize(); 00324 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_RGB565; 00325 layer_cfg.FBStartAdress = FB_Address; 00326 layer_cfg.Alpha = 255; 00327 layer_cfg.Alpha0 = 0; 00328 layer_cfg.Backcolor.Blue = 0; 00329 layer_cfg.Backcolor.Green = 0; 00330 layer_cfg.Backcolor.Red = 0; 00331 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00332 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00333 layer_cfg.ImageWidth = BSP_RGB_LCD_GetXSize(); 00334 layer_cfg.ImageHeight = BSP_RGB_LCD_GetYSize(); 00335 00336 HAL_LTDC_ConfigLayer(&hltdc_eval, &layer_cfg, LayerIndex); 00337 00338 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00339 DrawProp[LayerIndex].pFont = &Font24; 00340 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00341 } 00342 00343 /** 00344 * @brief Selects the LCD Layer. 00345 * @param LayerIndex: Layer foreground or background 00346 * @retval None 00347 */ 00348 void BSP_RGB_LCD_SelectLayer(uint32_t LayerIndex) 00349 { 00350 ActiveLayer = LayerIndex; 00351 } 00352 00353 /** 00354 * @brief Sets an LCD Layer visible 00355 * @param LayerIndex: Visible Layer 00356 * @param State: New state of the specified layer 00357 * This parameter can be one of the following values: 00358 * @arg ENABLE 00359 * @arg DISABLE 00360 * @retval None 00361 */ 00362 void BSP_RGB_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00363 { 00364 if(State == ENABLE) 00365 { 00366 __HAL_LTDC_LAYER_ENABLE(&hltdc_eval, LayerIndex); 00367 } 00368 else 00369 { 00370 __HAL_LTDC_LAYER_DISABLE(&hltdc_eval, LayerIndex); 00371 } 00372 __HAL_LTDC_RELOAD_IMMEDIATE_CONFIG(&hltdc_eval); 00373 } 00374 00375 /** 00376 * @brief Sets an LCD Layer visible without reloading. 00377 * @param LayerIndex: Visible Layer 00378 * @param State: New state of the specified layer 00379 * This parameter can be one of the following values: 00380 * @arg ENABLE 00381 * @arg DISABLE 00382 * @retval None 00383 */ 00384 void BSP_RGB_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State) 00385 { 00386 if(State == ENABLE) 00387 { 00388 __HAL_LTDC_LAYER_ENABLE(&hltdc_eval, LayerIndex); 00389 } 00390 else 00391 { 00392 __HAL_LTDC_LAYER_DISABLE(&hltdc_eval, LayerIndex); 00393 } 00394 /* Do not set the Reload */ 00395 } 00396 00397 /** 00398 * @brief Configures the transparency. 00399 * @param LayerIndex: Layer foreground or background. 00400 * @param Transparency: Transparency 00401 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00402 * @retval None 00403 */ 00404 void BSP_RGB_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00405 { 00406 HAL_LTDC_SetAlpha(&hltdc_eval, Transparency, LayerIndex); 00407 } 00408 00409 /** 00410 * @brief Configures the transparency without reloading. 00411 * @param LayerIndex: Layer foreground or background. 00412 * @param Transparency: Transparency 00413 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00414 * @retval None 00415 */ 00416 void BSP_RGB_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency) 00417 { 00418 HAL_LTDC_SetAlpha_NoReload(&hltdc_eval, Transparency, LayerIndex); 00419 } 00420 00421 /** 00422 * @brief Sets an LCD layer frame buffer address. 00423 * @param LayerIndex: Layer foreground or background 00424 * @param Address: New LCD frame buffer value 00425 * @retval None 00426 */ 00427 void BSP_RGB_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00428 { 00429 HAL_LTDC_SetAddress(&hltdc_eval, Address, LayerIndex); 00430 } 00431 00432 /** 00433 * @brief Sets an LCD layer frame buffer address without reloading. 00434 * @param LayerIndex: Layer foreground or background 00435 * @param Address: New LCD frame buffer value 00436 * @retval None 00437 */ 00438 void BSP_RGB_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address) 00439 { 00440 HAL_LTDC_SetAddress_NoReload(&hltdc_eval, Address, LayerIndex); 00441 } 00442 00443 /** 00444 * @brief Sets display window. 00445 * @param LayerIndex: Layer index 00446 * @param Xpos: LCD X position 00447 * @param Ypos: LCD Y position 00448 * @param Width: LCD window width 00449 * @param Height: LCD window height 00450 * @retval None 00451 */ 00452 void BSP_RGB_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00453 { 00454 /* Reconfigure the layer size */ 00455 HAL_LTDC_SetWindowSize(&hltdc_eval, Width, Height, LayerIndex); 00456 00457 /* Reconfigure the layer position */ 00458 HAL_LTDC_SetWindowPosition(&hltdc_eval, Xpos, Ypos, LayerIndex); 00459 } 00460 00461 /** 00462 * @brief Sets display window without reloading. 00463 * @param LayerIndex: Layer index 00464 * @param Xpos: LCD X position 00465 * @param Ypos: LCD Y position 00466 * @param Width: LCD window width 00467 * @param Height: LCD window height 00468 * @retval None 00469 */ 00470 void BSP_RGB_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00471 { 00472 /* Reconfigure the layer size */ 00473 HAL_LTDC_SetWindowSize_NoReload(&hltdc_eval, Width, Height, LayerIndex); 00474 00475 /* Reconfigure the layer position */ 00476 HAL_LTDC_SetWindowPosition_NoReload(&hltdc_eval, Xpos, Ypos, LayerIndex); 00477 } 00478 00479 /** 00480 * @brief Configures and sets the color keying. 00481 * @param LayerIndex: Layer foreground or background 00482 * @param RGBValue: Color reference 00483 * @retval None 00484 */ 00485 void BSP_RGB_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00486 { 00487 /* Configure and Enable the color Keying for LCD Layer */ 00488 HAL_LTDC_ConfigColorKeying(&hltdc_eval, RGBValue, LayerIndex); 00489 HAL_LTDC_EnableColorKeying(&hltdc_eval, LayerIndex); 00490 } 00491 00492 /** 00493 * @brief Configures and sets the color keying without reloading. 00494 * @param LayerIndex: Layer foreground or background 00495 * @param RGBValue: Color reference 00496 * @retval None 00497 */ 00498 void BSP_RGB_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue) 00499 { 00500 /* Configure and Enable the color Keying for LCD Layer */ 00501 HAL_LTDC_ConfigColorKeying_NoReload(&hltdc_eval, RGBValue, LayerIndex); 00502 HAL_LTDC_EnableColorKeying_NoReload(&hltdc_eval, LayerIndex); 00503 } 00504 00505 /** 00506 * @brief Disables the color keying. 00507 * @param LayerIndex: Layer foreground or background 00508 * @retval None 00509 */ 00510 void BSP_RGB_LCD_ResetColorKeying(uint32_t LayerIndex) 00511 { 00512 /* Disable the color Keying for LCD Layer */ 00513 HAL_LTDC_DisableColorKeying(&hltdc_eval, LayerIndex); 00514 } 00515 00516 /** 00517 * @brief Disables the color keying without reloading. 00518 * @param LayerIndex: Layer foreground or background 00519 * @retval None 00520 */ 00521 void BSP_RGB_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex) 00522 { 00523 /* Disable the color Keying for LCD Layer */ 00524 HAL_LTDC_DisableColorKeying_NoReload(&hltdc_eval, LayerIndex); 00525 } 00526 00527 /** 00528 * @brief Disables the color keying without reloading. 00529 * @param ReloadType: can be one of the following values 00530 * - LCD_RELOAD_IMMEDIATE 00531 * - LCD_RELOAD_VERTICAL_BLANKING 00532 * @retval None 00533 */ 00534 void BSP_RGB_LCD_Reload(uint32_t ReloadType) 00535 { 00536 HAL_LTDC_Reload (&hltdc_eval, ReloadType); 00537 } 00538 00539 /** 00540 * @brief Sets the LCD text color. 00541 * @param Color: Text color code ARGB(8-8-8-8) 00542 * @retval None 00543 */ 00544 void BSP_RGB_LCD_SetTextColor(uint32_t Color) 00545 { 00546 DrawProp[ActiveLayer].TextColor = Color; 00547 } 00548 00549 /** 00550 * @brief Gets the LCD text color. 00551 * @retval Used text color. 00552 */ 00553 uint32_t BSP_RGB_LCD_GetTextColor(void) 00554 { 00555 return DrawProp[ActiveLayer].TextColor; 00556 } 00557 00558 /** 00559 * @brief Sets the LCD background color. 00560 * @param Color: Layer background color code ARGB(8-8-8-8) 00561 * @retval None 00562 */ 00563 void BSP_RGB_LCD_SetBackColor(uint32_t Color) 00564 { 00565 DrawProp[ActiveLayer].BackColor = Color; 00566 } 00567 00568 /** 00569 * @brief Gets the LCD background color. 00570 * @retval Used background colour 00571 */ 00572 uint32_t BSP_RGB_LCD_GetBackColor(void) 00573 { 00574 return DrawProp[ActiveLayer].BackColor; 00575 } 00576 00577 /** 00578 * @brief Sets the LCD text font. 00579 * @param fonts: Layer font to be used 00580 * @retval None 00581 */ 00582 void BSP_RGB_LCD_SetFont(sFONT *fonts) 00583 { 00584 DrawProp[ActiveLayer].pFont = fonts; 00585 } 00586 00587 /** 00588 * @brief Gets the LCD text font. 00589 * @retval Used layer font 00590 */ 00591 sFONT *BSP_RGB_LCD_GetFont(void) 00592 { 00593 return DrawProp[ActiveLayer].pFont; 00594 } 00595 00596 /** 00597 * @brief Reads an LCD pixel. 00598 * @param Xpos: X position 00599 * @param Ypos: Y position 00600 * @retval RGB pixel color 00601 */ 00602 uint32_t BSP_RGB_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00603 { 00604 uint32_t ret = 0; 00605 00606 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00607 { 00608 /* Read data value from SDRAM memory */ 00609 ret = *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_RGB_LCD_GetXSize() + Xpos))); 00610 } 00611 else if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00612 { 00613 /* Read data value from SDRAM memory */ 00614 ret = (*(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_RGB_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00615 } 00616 else if((hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00617 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00618 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00619 { 00620 /* Read data value from SDRAM memory */ 00621 ret = *(__IO uint16_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_RGB_LCD_GetXSize() + Xpos))); 00622 } 00623 else 00624 { 00625 /* Read data value from SDRAM memory */ 00626 ret = *(__IO uint8_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_RGB_LCD_GetXSize() + Xpos))); 00627 } 00628 00629 return ret; 00630 } 00631 00632 /** 00633 * @brief Clears the hole LCD. 00634 * @param Color: Color of the background 00635 * @retval None 00636 */ 00637 void BSP_RGB_LCD_Clear(uint32_t Color) 00638 { 00639 /* Clear the LCD */ 00640 LL_FillBuffer(ActiveLayer, (uint32_t *)(hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress), BSP_RGB_LCD_GetXSize(), BSP_RGB_LCD_GetYSize(), 0, Color); 00641 } 00642 00643 /** 00644 * @brief Clears the selected line. 00645 * @param Line: Line to be cleared 00646 * @retval None 00647 */ 00648 void BSP_RGB_LCD_ClearStringLine(uint32_t Line) 00649 { 00650 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00651 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00652 00653 /* Draw rectangle with background color */ 00654 BSP_RGB_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_RGB_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00655 00656 DrawProp[ActiveLayer].TextColor = color_backup; 00657 BSP_RGB_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00658 } 00659 00660 /** 00661 * @brief Displays one character. 00662 * @param Xpos: Start column address 00663 * @param Ypos: Line where to display the character shape. 00664 * @param Ascii: Character ascii code 00665 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00666 * @retval None 00667 */ 00668 void BSP_RGB_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00669 { 00670 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00671 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00672 } 00673 00674 /** 00675 * @brief Displays characters on the LCD. 00676 * @param Xpos: X position (in pixel) 00677 * @param Ypos: Y position (in pixel) 00678 * @param Text: Pointer to string to display on LCD 00679 * @param Mode: Display mode 00680 * This parameter can be one of the following values: 00681 * @arg CENTER_MODE 00682 * @arg RIGHT_MODE 00683 * @arg LEFT_MODE 00684 * @retval None 00685 */ 00686 void BSP_RGB_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00687 { 00688 uint16_t ref_column = 1, i = 0; 00689 uint32_t size = 0, xsize = 0; 00690 uint8_t *ptr = Text; 00691 00692 /* Get the text size */ 00693 while (*ptr++) size ++ ; 00694 00695 /* Characters number per line */ 00696 xsize = (BSP_RGB_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00697 00698 switch (Mode) 00699 { 00700 case CENTER_MODE: 00701 { 00702 ref_column = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00703 break; 00704 } 00705 case LEFT_MODE: 00706 { 00707 ref_column = Xpos; 00708 break; 00709 } 00710 case RIGHT_MODE: 00711 { 00712 ref_column = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00713 break; 00714 } 00715 default: 00716 { 00717 ref_column = Xpos; 00718 break; 00719 } 00720 } 00721 00722 /* Check that the Start column is located in the screen */ 00723 if ((ref_column < 1) || (ref_column >= 0x8000)) 00724 { 00725 ref_column = 1; 00726 } 00727 00728 /* Send the string character by character on LCD */ 00729 while ((*Text != 0) & (((BSP_RGB_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00730 { 00731 /* Display one character on LCD */ 00732 BSP_RGB_LCD_DisplayChar(ref_column, Ypos, *Text); 00733 /* Decrement the column position by 16 */ 00734 ref_column += DrawProp[ActiveLayer].pFont->Width; 00735 /* Point on the next character */ 00736 Text++; 00737 i++; 00738 } 00739 } 00740 00741 /** 00742 * @brief Displays a maximum of 60 characters on the LCD. 00743 * @param Line: Line where to display the character shape 00744 * @param ptr: Pointer to string to display on LCD 00745 * @retval None 00746 */ 00747 void BSP_RGB_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00748 { 00749 BSP_RGB_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00750 } 00751 00752 /** 00753 * @brief Draws an horizontal line. 00754 * @param Xpos: X position 00755 * @param Ypos: Y position 00756 * @param Length: Line length 00757 * @retval None 00758 */ 00759 void BSP_RGB_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00760 { 00761 uint32_t Xaddress = 0; 00762 00763 /* Get the line address */ 00764 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 00765 { /* RGB565 format */ 00766 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 2*(BSP_RGB_LCD_GetXSize()*Ypos + Xpos); 00767 } 00768 else 00769 { /* ARGB8888 format */ 00770 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_RGB_LCD_GetXSize()*Ypos + Xpos); 00771 } 00772 00773 /* Write line */ 00774 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00775 } 00776 00777 /** 00778 * @brief Draws a vertical line. 00779 * @param Xpos: X position 00780 * @param Ypos: Y position 00781 * @param Length: Line length 00782 * @retval None 00783 */ 00784 void BSP_RGB_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00785 { 00786 uint32_t Xaddress = 0; 00787 00788 /* Get the line address */ 00789 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 00790 { /* RGB565 format */ 00791 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 2*(BSP_RGB_LCD_GetXSize()*Ypos + Xpos); 00792 } 00793 else 00794 { /* ARGB8888 format */ 00795 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_RGB_LCD_GetXSize()*Ypos + Xpos); 00796 } 00797 00798 /* Write line */ 00799 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_RGB_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00800 } 00801 00802 /** 00803 * @brief Draws an uni-line (between two points). 00804 * @param x1: Point 1 X position 00805 * @param y1: Point 1 Y position 00806 * @param x2: Point 2 X position 00807 * @param y2: Point 2 Y position 00808 * @retval None 00809 */ 00810 void BSP_RGB_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00811 { 00812 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00813 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 00814 curpixel = 0; 00815 00816 deltax = ABS(x2 - x1); /* The difference between the x's */ 00817 deltay = ABS(y2 - y1); /* The difference between the y's */ 00818 x = x1; /* Start x off at the first pixel */ 00819 y = y1; /* Start y off at the first pixel */ 00820 00821 if (x2 >= x1) /* The x-values are increasing */ 00822 { 00823 xinc1 = 1; 00824 xinc2 = 1; 00825 } 00826 else /* The x-values are decreasing */ 00827 { 00828 xinc1 = -1; 00829 xinc2 = -1; 00830 } 00831 00832 if (y2 >= y1) /* The y-values are increasing */ 00833 { 00834 yinc1 = 1; 00835 yinc2 = 1; 00836 } 00837 else /* The y-values are decreasing */ 00838 { 00839 yinc1 = -1; 00840 yinc2 = -1; 00841 } 00842 00843 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00844 { 00845 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00846 yinc2 = 0; /* Don't change the y for every iteration */ 00847 den = deltax; 00848 num = deltax / 2; 00849 num_add = deltay; 00850 num_pixels = deltax; /* There are more x-values than y-values */ 00851 } 00852 else /* There is at least one y-value for every x-value */ 00853 { 00854 xinc2 = 0; /* Don't change the x for every iteration */ 00855 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00856 den = deltay; 00857 num = deltay / 2; 00858 num_add = deltax; 00859 num_pixels = deltay; /* There are more y-values than x-values */ 00860 } 00861 00862 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 00863 { 00864 BSP_RGB_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00865 num += num_add; /* Increase the numerator by the top of the fraction */ 00866 if (num >= den) /* Check if numerator >= denominator */ 00867 { 00868 num -= den; /* Calculate the new numerator value */ 00869 x += xinc1; /* Change the x as appropriate */ 00870 y += yinc1; /* Change the y as appropriate */ 00871 } 00872 x += xinc2; /* Change the x as appropriate */ 00873 y += yinc2; /* Change the y as appropriate */ 00874 } 00875 } 00876 00877 /** 00878 * @brief Draws a rectangle. 00879 * @param Xpos: X position 00880 * @param Ypos: Y position 00881 * @param Width: Rectangle width 00882 * @param Height: Rectangle height 00883 * @retval None 00884 */ 00885 void BSP_RGB_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00886 { 00887 /* Draw horizontal lines */ 00888 BSP_RGB_LCD_DrawHLine(Xpos, Ypos, Width); 00889 BSP_RGB_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00890 00891 /* Draw vertical lines */ 00892 BSP_RGB_LCD_DrawVLine(Xpos, Ypos, Height); 00893 BSP_RGB_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00894 } 00895 00896 /** 00897 * @brief Draws a circle. 00898 * @param Xpos: X position 00899 * @param Ypos: Y position 00900 * @param Radius: Circle radius 00901 * @retval None 00902 */ 00903 void BSP_RGB_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00904 { 00905 int32_t decision; /* Decision Variable */ 00906 uint32_t current_x; /* Current X Value */ 00907 uint32_t current_y; /* Current Y Value */ 00908 00909 decision = 3 - (Radius << 1); 00910 current_x = 0; 00911 current_y = Radius; 00912 00913 while (current_x <= current_y) 00914 { 00915 BSP_RGB_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00916 00917 BSP_RGB_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00918 00919 BSP_RGB_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00920 00921 BSP_RGB_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00922 00923 BSP_RGB_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00924 00925 BSP_RGB_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00926 00927 BSP_RGB_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00928 00929 BSP_RGB_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00930 00931 if (decision < 0) 00932 { 00933 decision += (current_x << 2) + 6; 00934 } 00935 else 00936 { 00937 decision += ((current_x - current_y) << 2) + 10; 00938 current_y--; 00939 } 00940 current_x++; 00941 } 00942 } 00943 00944 /** 00945 * @brief Draws an poly-line (between many points). 00946 * @param Points: Pointer to the points array 00947 * @param PointCount: Number of points 00948 * @retval None 00949 */ 00950 void BSP_RGB_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00951 { 00952 int16_t x = 0, y = 0; 00953 00954 if(PointCount < 2) 00955 { 00956 return; 00957 } 00958 00959 BSP_RGB_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00960 00961 while(--PointCount) 00962 { 00963 x = Points->X; 00964 y = Points->Y; 00965 Points++; 00966 BSP_RGB_LCD_DrawLine(x, y, Points->X, Points->Y); 00967 } 00968 } 00969 00970 /** 00971 * @brief Draws an ellipse on LCD. 00972 * @param Xpos: X position 00973 * @param Ypos: Y position 00974 * @param XRadius: Ellipse X radius 00975 * @param YRadius: Ellipse Y radius 00976 * @retval None 00977 */ 00978 void BSP_RGB_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00979 { 00980 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00981 float k = 0, rad1 = 0, rad2 = 0; 00982 00983 rad1 = XRadius; 00984 rad2 = YRadius; 00985 00986 k = (float)(rad2/rad1); 00987 00988 do { 00989 BSP_RGB_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00990 BSP_RGB_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00991 BSP_RGB_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00992 BSP_RGB_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00993 00994 e2 = err; 00995 if (e2 <= x) { 00996 err += ++x*2+1; 00997 if (-y == x && e2 <= y) e2 = 0; 00998 } 00999 if (e2 > y) err += ++y*2+1; 01000 } 01001 while (y <= 0); 01002 } 01003 01004 /** 01005 * @brief Draws a pixel on LCD. 01006 * @param Xpos: X position 01007 * @param Ypos: Y position 01008 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 01009 * @retval None 01010 */ 01011 void BSP_RGB_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01012 { 01013 /* Write data value to all SDRAM memory */ 01014 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 01015 { /* RGB565 format */ 01016 *(__IO uint16_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_RGB_LCD_GetXSize() + Xpos))) = (uint16_t)RGB_Code; 01017 } 01018 else 01019 { /* ARGB8888 format */ 01020 *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_RGB_LCD_GetXSize() + Xpos))) = RGB_Code; 01021 } 01022 } 01023 01024 /** 01025 * @brief Draws a bitmap picture loaded in the internal Flash in ARGB888 format (32 bits per pixel). 01026 * @param Xpos: Bmp X position in the LCD 01027 * @param Ypos: Bmp Y position in the LCD 01028 * @param pbmp: Pointer to Bmp picture address in the internal Flash 01029 * @retval None 01030 */ 01031 void BSP_RGB_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 01032 { 01033 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 01034 uint32_t address; 01035 uint32_t input_color_mode = 0; 01036 01037 /* Get bitmap data address offset */ 01038 index = *(__IO uint16_t *) (pbmp + 10); 01039 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 01040 01041 /* Read bitmap width */ 01042 width = *(uint16_t *) (pbmp + 18); 01043 width |= (*(uint16_t *) (pbmp + 20)) << 16; 01044 01045 /* Read bitmap height */ 01046 height = *(uint16_t *) (pbmp + 22); 01047 height |= (*(uint16_t *) (pbmp + 24)) << 16; 01048 01049 /* Read bit/pixel */ 01050 bit_pixel = *(uint16_t *) (pbmp + 28); 01051 01052 /* Set the address */ 01053 address = hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_RGB_LCD_GetXSize()*Ypos) + Xpos)*(4)); 01054 01055 /* Get the layer pixel format */ 01056 if ((bit_pixel/8) == 4) 01057 { 01058 input_color_mode = DMA2D_INPUT_ARGB8888; 01059 } 01060 else if ((bit_pixel/8) == 2) 01061 { 01062 input_color_mode = DMA2D_INPUT_RGB565; 01063 } 01064 else 01065 { 01066 input_color_mode = DMA2D_INPUT_RGB888; 01067 } 01068 01069 /* Bypass the bitmap header */ 01070 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 01071 01072 /* Convert picture to ARGB8888 pixel format */ 01073 for(index=0; index < height; index++) 01074 { 01075 /* Pixel format conversion */ 01076 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)address, width, input_color_mode); 01077 01078 /* Increment the source and destination buffers */ 01079 address+= (BSP_RGB_LCD_GetXSize()*4); 01080 pbmp -= width*(bit_pixel/8); 01081 } 01082 } 01083 01084 /** 01085 * @brief Draws a full rectangle. 01086 * @param Xpos: X position 01087 * @param Ypos: Y position 01088 * @param Width: Rectangle width 01089 * @param Height: Rectangle height 01090 * @retval None 01091 */ 01092 void BSP_RGB_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01093 { 01094 uint32_t x_address = 0; 01095 01096 /* Set the text color */ 01097 BSP_RGB_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01098 01099 /* Get the rectangle start address */ 01100 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 01101 { /* RGB565 format */ 01102 x_address = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 2*(BSP_RGB_LCD_GetXSize()*Ypos + Xpos); 01103 } 01104 else 01105 { /* ARGB8888 format */ 01106 x_address = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_RGB_LCD_GetXSize()*Ypos + Xpos); 01107 } 01108 /* Fill the rectangle */ 01109 LL_FillBuffer(ActiveLayer, (uint32_t *)x_address, Width, Height, (BSP_RGB_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 01110 } 01111 01112 /** 01113 * @brief Draws a full circle. 01114 * @param Xpos: X position 01115 * @param Ypos: Y position 01116 * @param Radius: Circle radius 01117 * @retval None 01118 */ 01119 void BSP_RGB_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01120 { 01121 int32_t decision; /* Decision Variable */ 01122 uint32_t current_x; /* Current X Value */ 01123 uint32_t current_y; /* Current Y Value */ 01124 01125 decision = 3 - (Radius << 1); 01126 01127 current_x = 0; 01128 current_y = Radius; 01129 01130 BSP_RGB_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01131 01132 while (current_x <= current_y) 01133 { 01134 if(current_y > 0) 01135 { 01136 BSP_RGB_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 01137 BSP_RGB_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 01138 } 01139 01140 if(current_x > 0) 01141 { 01142 BSP_RGB_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 01143 BSP_RGB_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 01144 } 01145 if (decision < 0) 01146 { 01147 decision += (current_x << 2) + 6; 01148 } 01149 else 01150 { 01151 decision += ((current_x - current_y) << 2) + 10; 01152 current_y--; 01153 } 01154 current_x++; 01155 } 01156 01157 BSP_RGB_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01158 BSP_RGB_LCD_DrawCircle(Xpos, Ypos, Radius); 01159 } 01160 01161 /** 01162 * @brief Draws a full poly-line (between many points). 01163 * @param Points: Pointer to the points array 01164 * @param PointCount: Number of points 01165 * @retval None 01166 */ 01167 void BSP_RGB_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01168 { 01169 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01170 uint16_t image_left = 0, image_right = 0, image_top = 0, image_bottom = 0; 01171 01172 image_left = image_right = Points->X; 01173 image_top= image_bottom = Points->Y; 01174 01175 for(counter = 1; counter < PointCount; counter++) 01176 { 01177 pixelX = POLY_X(counter); 01178 if(pixelX < image_left) 01179 { 01180 image_left = pixelX; 01181 } 01182 if(pixelX > image_right) 01183 { 01184 image_right = pixelX; 01185 } 01186 01187 pixelY = POLY_Y(counter); 01188 if(pixelY < image_top) 01189 { 01190 image_top = pixelY; 01191 } 01192 if(pixelY > image_bottom) 01193 { 01194 image_bottom = pixelY; 01195 } 01196 } 01197 01198 if(PointCount < 2) 01199 { 01200 return; 01201 } 01202 01203 X_center = (image_left + image_right)/2; 01204 Y_center = (image_bottom + image_top)/2; 01205 01206 X_first = Points->X; 01207 Y_first = Points->Y; 01208 01209 while(--PointCount) 01210 { 01211 X = Points->X; 01212 Y = Points->Y; 01213 Points++; 01214 X2 = Points->X; 01215 Y2 = Points->Y; 01216 01217 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01218 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01219 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01220 } 01221 01222 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01223 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01224 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01225 } 01226 01227 /** 01228 * @brief Draws a full ellipse. 01229 * @param Xpos: X position 01230 * @param Ypos: Y position 01231 * @param XRadius: Ellipse X radius 01232 * @param YRadius: Ellipse Y radius 01233 * @retval None 01234 */ 01235 void BSP_RGB_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01236 { 01237 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01238 float k = 0, rad1 = 0, rad2 = 0; 01239 01240 rad1 = XRadius; 01241 rad2 = YRadius; 01242 01243 k = (float)(rad2/rad1); 01244 01245 do 01246 { 01247 BSP_RGB_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 01248 BSP_RGB_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 01249 01250 e2 = err; 01251 if (e2 <= x) 01252 { 01253 err += ++x*2+1; 01254 if (-y == x && e2 <= y) e2 = 0; 01255 } 01256 if (e2 > y) err += ++y*2+1; 01257 } 01258 while (y <= 0); 01259 } 01260 01261 /** 01262 * @brief Enables the display. 01263 * @retval None 01264 */ 01265 void BSP_RGB_LCD_DisplayOn(void) 01266 { 01267 /* Display On */ 01268 __HAL_LTDC_ENABLE(&hltdc_eval); 01269 BSP_IO_WritePin(LCD_RGB_DISP_PIN, GPIO_PIN_SET); /* Assert LCD_RGB_DISP pin */ 01270 HAL_GPIO_WritePin(LCD_RGB_BL_CTRL_GPIO_PORT, LCD_RGB_BL_CTRL_PIN, GPIO_PIN_SET); /* Assert LCD_BL_CTRL pin */ 01271 } 01272 01273 /** 01274 * @brief Disables the display. 01275 * @retval None 01276 */ 01277 void BSP_RGB_LCD_DisplayOff(void) 01278 { 01279 /* Display Off */ 01280 __HAL_LTDC_DISABLE(&hltdc_eval); 01281 BSP_IO_WritePin(LCD_RGB_DISP_PIN, GPIO_PIN_RESET); /* De-assert LCD_DISP pin */ 01282 HAL_GPIO_WritePin(LCD_RGB_BL_CTRL_GPIO_PORT, LCD_RGB_BL_CTRL_PIN, GPIO_PIN_RESET);/* De-assert LCD_BL_CTRL pin */ 01283 } 01284 01285 /** 01286 * @brief Initializes the LTDC MSP. 01287 * @param hltdc: LTDC handle 01288 * @param Params 01289 * @retval None 01290 */ 01291 __weak void BSP_RGB_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params) 01292 { 01293 GPIO_InitTypeDef gpio_init_structure; 01294 01295 /* IO expander init */ 01296 BSP_IO_Init(); 01297 01298 /* Enable the LTDC and DMA2D clocks */ 01299 __HAL_RCC_LTDC_CLK_ENABLE(); 01300 __HAL_RCC_DMA2D_CLK_ENABLE(); 01301 01302 /* Enable GPIOs clock */ 01303 __HAL_RCC_GPIOD_CLK_ENABLE(); 01304 __HAL_RCC_GPIOE_CLK_ENABLE(); 01305 __HAL_RCC_GPIOF_CLK_ENABLE(); 01306 LCD_RGB_BL_CTRL_GPIO_CLK_ENABLE(); 01307 01308 /*** LTDC Pins configuration ***/ 01309 /* GPIOD configuration */ 01310 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3 | GPIO_PIN_8 | GPIO_PIN_9 | \ 01311 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15; 01312 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01313 gpio_init_structure.Pull = GPIO_NOPULL; 01314 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01315 gpio_init_structure.Alternate = GPIO_AF11_LTDC; 01316 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 01317 01318 /* GPIOE configuration */ 01319 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | \ 01320 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01321 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01322 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01323 gpio_init_structure.Pull = GPIO_NOPULL; 01324 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01325 gpio_init_structure.Alternate = GPIO_AF11_LTDC; 01326 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 01327 01328 /* GPIOF configuration */ 01329 gpio_init_structure.Pin = GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01330 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01331 gpio_init_structure.Alternate = GPIO_AF11_LTDC; 01332 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 01333 01334 gpio_init_structure.Pin = GPIO_PIN_11; 01335 gpio_init_structure.Alternate = GPIO_AF9_LTDC; 01336 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 01337 01338 /* LCD_DISP & LCD_RST pins configuration (over MFX IO Expander) */ 01339 BSP_IO_ConfigPin(LCD_RGB_DISP_PIN | LCD_RGB_RST_PIN, IO_MODE_OUTPUT); 01340 01341 /* LCD_BL_CTRL GPIO configuration */ 01342 gpio_init_structure.Pin = LCD_RGB_BL_CTRL_PIN; /* LCD_BL_CTRL pin has to be manually controlled */ 01343 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 01344 HAL_GPIO_Init(LCD_RGB_BL_CTRL_GPIO_PORT, &gpio_init_structure); 01345 } 01346 01347 /** 01348 * @brief DeInitializes BSP_RGB_LCD_ MSP. 01349 * @param hltdc: LTDC handle 01350 * @param Params 01351 * @retval None 01352 */ 01353 __weak void BSP_RGB_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params) 01354 { 01355 GPIO_InitTypeDef gpio_init_structure; 01356 01357 /* Disable LTDC block */ 01358 __HAL_LTDC_DISABLE(hltdc); 01359 01360 /* LTDC Pins deactivation */ 01361 01362 /* GPIOD deactivation */ 01363 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3 | GPIO_PIN_8 | GPIO_PIN_9 | \ 01364 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15; 01365 HAL_GPIO_DeInit(GPIOD, gpio_init_structure.Pin); 01366 01367 /* GPIOE deactivation */ 01368 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | \ 01369 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01370 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01371 HAL_GPIO_DeInit(GPIOE, gpio_init_structure.Pin); 01372 01373 /* GPIOF configuration */ 01374 gpio_init_structure.Pin = GPIO_PIN_11 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01375 HAL_GPIO_DeInit(GPIOF, gpio_init_structure.Pin); 01376 01377 /* Disable LTDC clock */ 01378 __HAL_RCC_LTDC_CLK_DISABLE(); 01379 01380 /* GPIO pins clock can be shut down in the application 01381 by surcharging this __weak function */ 01382 } 01383 01384 /** 01385 * @brief Clock Config. 01386 * @param hltdc: LTDC handle 01387 * @param Params 01388 * @note This API is called by BSP_RGB_LCD_Init() 01389 * Being __weak it can be overwritten by the application 01390 * @retval None 01391 */ 01392 __weak void BSP_RGB_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params) 01393 { 01394 RCC_PeriphCLKInitTypeDef PeriphClkInit; 01395 01396 /* RK043FN48H LCD clock configuration */ 01397 /* PLLSAI2_VCO Input = MSI_VALUE/PLLSAI2_M = 4 Mhz */ 01398 /* PLLSAI2_VCO Output = PLLSAI2_VCO Input * PLLSAI2N = 192 Mhz */ 01399 /* PLLLCDCLK = PLLSAI2_VCO Output/PLLSAIR = 160/4 = 40 Mhz */ 01400 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_4 = 40/4 = 10Mhz */ 01401 PeriphClkInit.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01402 PeriphClkInit.PLLSAI2.PLLSAI2Source = RCC_PLLSOURCE_MSI; 01403 PeriphClkInit.PLLSAI2.PLLSAI2M = 1; 01404 PeriphClkInit.PLLSAI2.PLLSAI2N = 40; 01405 PeriphClkInit.PLLSAI2.PLLSAI2R = 4; 01406 PeriphClkInit.PLLSAI2.PLLSAI2ClockOut = RCC_PLLSAI2_LTDCCLK; 01407 PeriphClkInit.LtdcClockSelection = RCC_LTDCCLKSOURCE_PLLSAI2_DIV4; 01408 if(HAL_RCCEx_PeriphCLKConfig(&PeriphClkInit) != HAL_OK) 01409 { 01410 while(1); 01411 } 01412 } 01413 01414 /******************************************************************************* 01415 LTDC and DMA2D BSP Routines 01416 *******************************************************************************/ 01417 /** 01418 * @brief Handles LTDC interrupt request. 01419 * @note : Can be surcharged by application code implementation of the function. 01420 */ 01421 __weak void BSP_RGB_LCD_LTDC_IRQHandler(void) 01422 { 01423 HAL_LTDC_IRQHandler(&(hltdc_eval)); 01424 } 01425 01426 /** 01427 * @brief This function handles LTDC Error interrupt Handler. 01428 * @note : Can be surcharged by application code implementation of the function. 01429 */ 01430 01431 __weak void BSP_RGB_LCD_LTDC_ER_IRQHandler(void) 01432 { 01433 HAL_LTDC_IRQHandler(&(hltdc_eval)); 01434 } 01435 01436 /** 01437 * @brief Handles DMA2D interrupt request. 01438 * @note : Can be surcharged by application code implementation of the function. 01439 */ 01440 __weak void BSP_RGB_LCD_DMA2D_IRQHandler(void) 01441 { 01442 HAL_DMA2D_IRQHandler(&hdma2d_eval); 01443 } 01444 01445 /** 01446 * @} 01447 */ 01448 01449 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Private_Functions Private Functions 01450 * @{ 01451 */ 01452 01453 /******************************************************************************* 01454 Static Functions 01455 *******************************************************************************/ 01456 01457 /** 01458 * @brief Draws a character on LCD. 01459 * @param Xpos: Line where to display the character shape 01460 * @param Ypos: Start column address 01461 * @param c: Pointer to the character data 01462 * @retval None 01463 */ 01464 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01465 { 01466 uint32_t i = 0, j = 0; 01467 uint16_t height, width; 01468 uint8_t offset; 01469 uint8_t *pchar; 01470 uint32_t line; 01471 01472 height = DrawProp[ActiveLayer].pFont->Height; 01473 width = DrawProp[ActiveLayer].pFont->Width; 01474 01475 offset = 8 *((width + 7)/8) - width ; 01476 01477 for(i = 0; i < height; i++) 01478 { 01479 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01480 01481 switch(((width + 7)/8)) 01482 { 01483 01484 case 1: 01485 line = pchar[0]; 01486 break; 01487 01488 case 2: 01489 line = (pchar[0]<< 8) | pchar[1]; 01490 break; 01491 01492 case 3: 01493 default: 01494 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01495 break; 01496 } 01497 01498 for (j = 0; j < width; j++) 01499 { 01500 if(line & (1 << (width- j + offset- 1))) 01501 { 01502 BSP_RGB_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01503 } 01504 else 01505 { 01506 BSP_RGB_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01507 } 01508 } 01509 Ypos++; 01510 } 01511 } 01512 01513 /** 01514 * @brief Fills a triangle (between 3 points). 01515 * @param x1: Point 1 X position 01516 * @param y1: Point 1 Y position 01517 * @param x2: Point 2 X position 01518 * @param y2: Point 2 Y position 01519 * @param x3: Point 3 X position 01520 * @param y3: Point 3 Y position 01521 * @retval None 01522 */ 01523 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01524 { 01525 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01526 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 01527 curpixel = 0; 01528 01529 deltax = ABS(x2 - x1); /* The difference between the x's */ 01530 deltay = ABS(y2 - y1); /* The difference between the y's */ 01531 x = x1; /* Start x off at the first pixel */ 01532 y = y1; /* Start y off at the first pixel */ 01533 01534 if (x2 >= x1) /* The x-values are increasing */ 01535 { 01536 xinc1 = 1; 01537 xinc2 = 1; 01538 } 01539 else /* The x-values are decreasing */ 01540 { 01541 xinc1 = -1; 01542 xinc2 = -1; 01543 } 01544 01545 if (y2 >= y1) /* The y-values are increasing */ 01546 { 01547 yinc1 = 1; 01548 yinc2 = 1; 01549 } 01550 else /* The y-values are decreasing */ 01551 { 01552 yinc1 = -1; 01553 yinc2 = -1; 01554 } 01555 01556 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01557 { 01558 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01559 yinc2 = 0; /* Don't change the y for every iteration */ 01560 den = deltax; 01561 num = deltax / 2; 01562 num_add = deltay; 01563 num_pixels = deltax; /* There are more x-values than y-values */ 01564 } 01565 else /* There is at least one y-value for every x-value */ 01566 { 01567 xinc2 = 0; /* Don't change the x for every iteration */ 01568 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01569 den = deltay; 01570 num = deltay / 2; 01571 num_add = deltax; 01572 num_pixels = deltay; /* There are more y-values than x-values */ 01573 } 01574 01575 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 01576 { 01577 BSP_RGB_LCD_DrawLine(x, y, x3, y3); 01578 01579 num += num_add; /* Increase the numerator by the top of the fraction */ 01580 if (num >= den) /* Check if numerator >= denominator */ 01581 { 01582 num -= den; /* Calculate the new numerator value */ 01583 x += xinc1; /* Change the x as appropriate */ 01584 y += yinc1; /* Change the y as appropriate */ 01585 } 01586 x += xinc2; /* Change the x as appropriate */ 01587 y += yinc2; /* Change the y as appropriate */ 01588 } 01589 } 01590 01591 /** 01592 * @brief Fills a buffer. 01593 * @param LayerIndex: Layer index 01594 * @param pDst: Pointer to destination buffer 01595 * @param xSize: Buffer width 01596 * @param ySize: Buffer height 01597 * @param OffLine: Offset 01598 * @param ColorIndex: Color index 01599 * @retval None 01600 */ 01601 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01602 { 01603 /* Register to memory mode with ARGB8888 as color Mode */ 01604 hdma2d_eval.Init.Mode = DMA2D_R2M; 01605 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 01606 { /* RGB565 format */ 01607 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_RGB565; 01608 } 01609 else 01610 { /* ARGB8888 format */ 01611 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01612 } 01613 hdma2d_eval.Init.OutputOffset = OffLine; 01614 01615 hdma2d_eval.Instance = DMA2D; 01616 01617 /* DMA2D Initialization */ 01618 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01619 { 01620 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, LayerIndex) == HAL_OK) 01621 { 01622 if (HAL_DMA2D_Start(&hdma2d_eval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01623 { 01624 /* Polling For DMA transfer */ 01625 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01626 } 01627 } 01628 } 01629 } 01630 01631 /** 01632 * @brief Converts a line to an ARGB8888 pixel format. 01633 * @param pSrc: Pointer to source buffer 01634 * @param pDst: Output color 01635 * @param xSize: Buffer width 01636 * @param ColorMode: Input color mode 01637 * @retval None 01638 */ 01639 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01640 { 01641 /* Configure the DMA2D Mode, Color Mode and output offset */ 01642 hdma2d_eval.Init.Mode = DMA2D_M2M_PFC; 01643 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01644 hdma2d_eval.Init.OutputOffset = 0; 01645 01646 /* Foreground Configuration */ 01647 hdma2d_eval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01648 hdma2d_eval.LayerCfg[1].InputAlpha = 0xFF; 01649 hdma2d_eval.LayerCfg[1].InputColorMode = ColorMode; 01650 hdma2d_eval.LayerCfg[1].InputOffset = 0; 01651 01652 hdma2d_eval.Instance = DMA2D; 01653 01654 /* DMA2D Initialization */ 01655 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01656 { 01657 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, 1) == HAL_OK) 01658 { 01659 if (HAL_DMA2D_Start(&hdma2d_eval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01660 { 01661 /* Polling For DMA transfer */ 01662 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01663 } 01664 } 01665 } 01666 } 01667 01668 /** 01669 * @} 01670 */ 01671 01672 #endif /* USE_ROCKTECH_480x272 */ 01673 01674 /** 01675 * @} 01676 */ 01677 01678 /** 01679 * @} 01680 */ 01681 01682 /** 01683 * @} 01684 */ 01685 01686 /** 01687 * @} 01688 */ 01689 01690 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
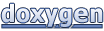