STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_rgb_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_rgb_lcd.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32l4r9i_eval_rgb_lcd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L4R9I_EVAL_RGB_LCD_H 00039 #define __STM32L4R9I_EVAL_RGB_LCD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32l4r9i_eval.h" 00047 /* Include LCD component Driver */ 00048 /* LCD RK043FN48H-CT672B 4,3" 480x272 pixels */ 00049 #include "../Components/rk043fn48h/rk043fn48h.h" 00050 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32L4R9I_EVAL 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32L4R9I_EVAL_LCD STM32L4R9I_EVAL LCD 00061 * @{ 00062 */ 00063 00064 /** @defgroup STM32L4R9I_EVAL_RGB_LCD STM32L4R9I_EVAL RGB LCD 00065 * @{ 00066 */ 00067 00068 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Exported_Constants Exported Constants 00069 * @{ 00070 */ 00071 /** 00072 * @brief LCD FB_StartAddress 00073 */ 00074 #define LCD_FB_START_ADDRESS (uint32_t)PhysFrameBuffer /* Internal SRAM used for LCD Frame buffer */ 00075 00076 /** 00077 * @brief LCD Reload Types 00078 */ 00079 #define LCD_RELOAD_IMMEDIATE ((uint32_t)LTDC_SRCR_IMR) 00080 #define LCD_RELOAD_VERTICAL_BLANKING ((uint32_t)LTDC_SRCR_VBR) 00081 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Exported_Variables Exported Variables 00087 * @{ 00088 */ 00089 extern LTDC_HandleTypeDef hltdc_eval; 00090 extern uint32_t PhysFrameBuffer[]; 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32L4R9I_EVAL_RGB_LCD_Exported_Functions Exported Functions 00097 * @{ 00098 */ 00099 uint8_t BSP_RGB_LCD_Init(void); 00100 uint8_t BSP_RGB_LCD_DeInit(void); 00101 uint32_t BSP_RGB_LCD_GetXSize(void); 00102 uint32_t BSP_RGB_LCD_GetYSize(void); 00103 void BSP_RGB_LCD_SetXSize(uint32_t imageWidthPixels); 00104 void BSP_RGB_LCD_SetYSize(uint32_t imageHeightPixels); 00105 00106 /* Functions using the LTDC controller */ 00107 void BSP_RGB_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FrameBuffer); 00108 void BSP_RGB_LCD_LayerRgb565Init(uint16_t LayerIndex, uint32_t FB_Address); 00109 void BSP_RGB_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00110 void BSP_RGB_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency); 00111 void BSP_RGB_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00112 void BSP_RGB_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address); 00113 void BSP_RGB_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00114 void BSP_RGB_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue); 00115 void BSP_RGB_LCD_ResetColorKeying(uint32_t LayerIndex); 00116 void BSP_RGB_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex); 00117 void BSP_RGB_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00118 void BSP_RGB_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00119 void BSP_RGB_LCD_SelectLayer(uint32_t LayerIndex); 00120 void BSP_RGB_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State); 00121 void BSP_RGB_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State); 00122 void BSP_RGB_LCD_Reload(uint32_t ReloadType); 00123 00124 void BSP_RGB_LCD_SetTextColor(uint32_t Color); 00125 uint32_t BSP_RGB_LCD_GetTextColor(void); 00126 void BSP_RGB_LCD_SetBackColor(uint32_t Color); 00127 uint32_t BSP_RGB_LCD_GetBackColor(void); 00128 void BSP_RGB_LCD_SetFont(sFONT *fonts); 00129 sFONT *BSP_RGB_LCD_GetFont(void); 00130 00131 uint32_t BSP_RGB_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00132 void BSP_RGB_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t pixel); 00133 void BSP_RGB_LCD_Clear(uint32_t Color); 00134 void BSP_RGB_LCD_ClearStringLine(uint32_t Line); 00135 void BSP_RGB_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00136 void BSP_RGB_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode); 00137 void BSP_RGB_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00138 00139 void BSP_RGB_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00140 void BSP_RGB_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00141 void BSP_RGB_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00142 void BSP_RGB_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00143 void BSP_RGB_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00144 void BSP_RGB_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00145 void BSP_RGB_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00146 void BSP_RGB_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp); 00147 00148 void BSP_RGB_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00149 void BSP_RGB_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00150 void BSP_RGB_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00151 void BSP_RGB_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00152 00153 void BSP_RGB_LCD_DisplayOff(void); 00154 void BSP_RGB_LCD_DisplayOn(void); 00155 00156 /* These __weak functions can be surcharged by application code for specific application needs */ 00157 void BSP_RGB_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params); 00158 void BSP_RGB_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params); 00159 void BSP_RGB_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params); 00160 void BSP_RGB_LCD_LTDC_IRQHandler(void); 00161 void BSP_RGB_LCD_LTDC_ER_IRQHandler(void); 00162 void BSP_RGB_LCD_DMA2D_IRQHandler(void); 00163 00164 /** 00165 * @} 00166 */ 00167 00168 /** 00169 * @} 00170 */ 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /** 00177 * @} 00178 */ 00179 00180 /** 00181 * @} 00182 */ 00183 00184 #ifdef __cplusplus 00185 } 00186 #endif 00187 00188 #endif /* __STM32L4R9I_EVAL_RGB_LCD_H */ 00189 00190 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
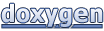