STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_ospi_ram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_ospi_ram.c 00004 * @author MCD Application Team 00005 * @brief This file includes a standard driver for the ISS66WVH8M8 HyperRAM 00006 * memory mounted on STM32L4R9I-EVAL board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive the ISS66WVH8M8 HyperRAM external 00013 memory mounted on STM32L4R9I-EVAL evaluation board. 00014 00015 (#) This driver need a specific component driver (ISS66WVH8M8) to be included with. 00016 00017 (#) Initialization steps: 00018 (++) Initialize the HyperRAM external memory using the BSP_OSPI_RAM_Init() function. This 00019 function includes the MSP layer hardware resources initialization and the 00020 OSPI interface with the external memory. 00021 00022 (#) HyperRAM memory operations 00023 (++) HyperRAM memory can be accessed with read/write operations once it is 00024 initialized. 00025 Read/write operation can be performed with AHB access using the functions 00026 BSP_OSPI_RAM_Read()/BSP_OSPI_RAM_Write(). 00027 (++) The function BSP_OSPI_RAM_GetInfo() returns the configuration of the HyperRAM memory. 00028 (see the HyperRAM memory data sheet) 00029 (++) Perform erase block operation using the function BSP_OSPI_RAM_Erase_Block() and by 00030 specifying the block address. You can perform an erase operation of the whole 00031 chip by calling the function BSP_OSPI_RAM_Erase_Chip(). 00032 (++) The function BSP_OSPI_RAM_GetStatus() returns the current status of the HyperRAM memory. 00033 (see the HyperRAM memory data sheet) 00034 @endverbatim 00035 ****************************************************************************** 00036 * @attention 00037 * 00038 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00039 * 00040 * Redistribution and use in source and binary forms, with or without modification, 00041 * are permitted provided that the following conditions are met: 00042 * 1. Redistributions of source code must retain the above copyright notice, 00043 * this list of conditions and the following disclaimer. 00044 * 2. Redistributions in binary form must reproduce the above copyright notice, 00045 * this list of conditions and the following disclaimer in the documentation 00046 * and/or other materials provided with the distribution. 00047 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00048 * may be used to endorse or promote products derived from this software 00049 * without specific prior written permission. 00050 * 00051 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00052 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00053 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00054 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00055 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00056 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00057 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00058 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00059 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00060 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00061 * 00062 ****************************************************************************** 00063 */ 00064 00065 /* Includes ------------------------------------------------------------------*/ 00066 #include "stm32l4r9i_eval_ospi_ram.h" 00067 00068 /** @addtogroup BSP 00069 * @{ 00070 */ 00071 00072 /** @addtogroup STM32L4R9I_EVAL 00073 * @{ 00074 */ 00075 00076 /** @defgroup STM32L4R9I_EVAL_OSPI_RAM STM32L4R9I_EVAL OSPI RAM 00077 * @{ 00078 */ 00079 00080 /* Private constants --------------------------------------------------------*/ 00081 /** @defgroup STM32L4R9I_EVAL_OSPI_RAM_Private_Constants Private Constants 00082 * @{ 00083 */ 00084 #define OSPI_RAM_QUAD_DISABLE 0x0 00085 #define OSPI_RAM_QUAD_ENABLE 0x1 00086 00087 #define OSPI_RAM_HIGH_PERF_DISABLE 0x0 00088 #define OSPI_RAM_HIGH_PERF_ENABLE 0x1 00089 /** 00090 * @} 00091 */ 00092 /* Private variables ---------------------------------------------------------*/ 00093 00094 /** @defgroup STM32L4R9I_EVAL_OSPI_RAM_Private_Variables Private Variables 00095 * @{ 00096 */ 00097 OSPI_HandleTypeDef OspiRamHandle; 00098 DMA_HandleTypeDef DmaOspiRamHandle; 00099 00100 /** 00101 * @} 00102 */ 00103 00104 00105 /* Private functions ---------------------------------------------------------*/ 00106 00107 /** @defgroup STM32L4R9I_EVAL_OSPI_RAM_Private_Functions Private Functions 00108 * @{ 00109 */ 00110 static void OSPI_RAM_MspInit (void); 00111 static void OSPI_RAM_MspDeInit(void); 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /* Exported functions ---------------------------------------------------------*/ 00118 00119 /** @addtogroup STM32L4R9I_EVAL_OSPI_RAM_Exported_Functions 00120 * @{ 00121 */ 00122 00123 /** 00124 * @brief Initializes the OSPI interface. 00125 * @retval HyperRAM memory status 00126 */ 00127 uint8_t BSP_OSPI_RAM_Init(void) 00128 { 00129 OSPI_HyperbusCfgTypeDef sHyperbusCfg; 00130 00131 OspiRamHandle.Instance = OCTOSPI2; 00132 00133 /* Call the DeInit function to reset the driver */ 00134 if (HAL_OSPI_DeInit(&OspiRamHandle) != HAL_OK) 00135 { 00136 return OSPI_RAM_ERROR; 00137 } 00138 00139 /* System level initialization */ 00140 OSPI_RAM_MspInit(); 00141 00142 /* OctoSPI initialization */ 00143 OspiRamHandle.Init.FifoThreshold = 4; 00144 OspiRamHandle.Init.DualQuad = HAL_OSPI_DUALQUAD_DISABLE; 00145 OspiRamHandle.Init.MemoryType = HAL_OSPI_MEMTYPE_HYPERBUS; 00146 OspiRamHandle.Init.DeviceSize = POSITION_VAL(ISS66WVH8M8_RAM_SIZE); /* 64 MBits */ 00147 OspiRamHandle.Init.ChipSelectHighTime = 2; 00148 OspiRamHandle.Init.FreeRunningClock = HAL_OSPI_FREERUNCLK_DISABLE; 00149 OspiRamHandle.Init.ClockMode = HAL_OSPI_CLOCK_MODE_0; 00150 OspiRamHandle.Init.WrapSize = HAL_OSPI_WRAP_NOT_SUPPORTED; 00151 OspiRamHandle.Init.ClockPrescaler = 3; /* OctoSPI clock = 110MHz / ClockPrescaler = 36.67 MHz */ 00152 OspiRamHandle.Init.SampleShifting = HAL_OSPI_SAMPLE_SHIFTING_NONE; 00153 OspiRamHandle.Init.DelayHoldQuarterCycle = HAL_OSPI_DHQC_ENABLE; 00154 OspiRamHandle.Init.ChipSelectBoundary = 0; 00155 00156 if (HAL_OSPI_Init(&OspiRamHandle) != HAL_OK) 00157 { 00158 return OSPI_RAM_ERROR; 00159 } 00160 00161 /* OctoSPI Hyperbus configuration to access memory space */ 00162 sHyperbusCfg.RWRecoveryTime = 3; /* 40 ns @ 60MHz */ 00163 sHyperbusCfg.AccessTime = ISS66WVH8M8_LATENCY_166M; 00164 sHyperbusCfg.WriteZeroLatency = HAL_OSPI_LATENCY_ON_WRITE; 00165 sHyperbusCfg.LatencyMode = HAL_OSPI_FIXED_LATENCY; 00166 00167 if (HAL_OSPI_HyperbusCfg(&OspiRamHandle, &sHyperbusCfg, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00168 { 00169 return OSPI_RAM_ERROR; 00170 } 00171 00172 return OSPI_RAM_OK; 00173 } 00174 00175 /** 00176 * @brief De-Initializes the OSPI interface. 00177 * @retval HyperRAM memory status 00178 */ 00179 uint8_t BSP_OSPI_RAM_DeInit(void) 00180 { 00181 OspiRamHandle.Instance = OCTOSPI1; 00182 00183 /* Call the DeInit function to reset the driver */ 00184 if (HAL_OSPI_DeInit(&OspiRamHandle) != HAL_OK) 00185 { 00186 return OSPI_RAM_ERROR; 00187 } 00188 00189 /* System level De-initialization */ 00190 OSPI_RAM_MspDeInit(); 00191 00192 return OSPI_RAM_OK; 00193 } 00194 00195 /** 00196 * @brief Reads an amount of data from the HyperRAM memory. 00197 * @param pData: Pointer to data to be read 00198 * @param ReadAddr: Read start address 00199 * @param Size: Size of data to read 00200 * @retval HyperRAM memory status 00201 */ 00202 uint8_t BSP_OSPI_RAM_Read(uint8_t* pData, uint32_t ReadAddr, uint32_t Size) 00203 { 00204 OSPI_HyperbusCmdTypeDef sCommand; 00205 00206 /* Initialize the read command */ 00207 sCommand.AddressSpace = HAL_OSPI_MEMORY_ADDRESS_SPACE; 00208 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00209 sCommand.Address = ReadAddr; 00210 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00211 sCommand.NbData = Size; 00212 00213 /* Configure the command */ 00214 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00215 { 00216 return OSPI_RAM_ERROR; 00217 } 00218 00219 /* Reception of the data */ 00220 if (HAL_OSPI_Receive(&OspiRamHandle, pData, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00221 { 00222 return OSPI_RAM_ERROR; 00223 } 00224 00225 return OSPI_RAM_OK; 00226 } 00227 00228 /** 00229 * @brief Reads an amount of data from the HyperRAM memory in DMA mode. 00230 * @param pData: Pointer to data to be read 00231 * @param ReadAddr: Read start address 00232 * @param Size: Size of data to read 00233 * @retval HyperRAM memory status 00234 */ 00235 uint8_t BSP_OSPI_RAM_Read_DMA(uint8_t* pData, uint32_t ReadAddr, uint32_t Size) 00236 { 00237 OSPI_HyperbusCmdTypeDef sCommand; 00238 00239 /* Initialize the read command */ 00240 sCommand.AddressSpace = HAL_OSPI_MEMORY_ADDRESS_SPACE; 00241 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00242 sCommand.Address = ReadAddr; 00243 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00244 sCommand.NbData = Size; 00245 00246 /* Configure the command */ 00247 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00248 { 00249 return OSPI_RAM_ERROR; 00250 } 00251 00252 /* Reception of the data */ 00253 if (HAL_OSPI_Receive_DMA(&OspiRamHandle, pData) != HAL_OK) 00254 { 00255 return OSPI_RAM_ERROR; 00256 } 00257 00258 return OSPI_RAM_OK; 00259 } 00260 00261 /** 00262 * @brief Writes an amount of data to the HyperRAM memory. 00263 * @param pData: Pointer to data to be written 00264 * @param WriteAddr: Write start address 00265 * @param Size: Size of data to write 00266 * @retval HyperRAM memory status 00267 */ 00268 uint8_t BSP_OSPI_RAM_Write(uint8_t* pData, uint32_t WriteAddr, uint32_t Size) 00269 { 00270 OSPI_HyperbusCmdTypeDef sCommand; 00271 00272 /* Initialize the write command */ 00273 sCommand.AddressSpace = HAL_OSPI_MEMORY_ADDRESS_SPACE; 00274 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00275 sCommand.Address = WriteAddr; 00276 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00277 sCommand.NbData = Size; 00278 00279 /* Configure the command */ 00280 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00281 { 00282 return OSPI_RAM_ERROR; 00283 } 00284 00285 /* Transmission of the data */ 00286 if (HAL_OSPI_Transmit(&OspiRamHandle, pData, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00287 { 00288 return OSPI_RAM_ERROR; 00289 } 00290 00291 return OSPI_RAM_OK; 00292 } 00293 00294 /** 00295 * @brief Writes an amount of data to the HyperRAM memory in DMA mode. 00296 * @param pData: Pointer to data to be written 00297 * @param WriteAddr: Write start address 00298 * @param Size: Size of data to write 00299 * @retval HyperRAM memory status 00300 */ 00301 uint8_t BSP_OSPI_RAM_Write_DMA(uint8_t* pData, uint32_t WriteAddr, uint32_t Size) 00302 { 00303 OSPI_HyperbusCmdTypeDef sCommand; 00304 00305 /* Initialize the write command */ 00306 sCommand.AddressSpace = HAL_OSPI_MEMORY_ADDRESS_SPACE; 00307 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00308 sCommand.Address = WriteAddr; 00309 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00310 sCommand.NbData = Size; 00311 00312 /* Configure the command */ 00313 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00314 { 00315 return OSPI_RAM_ERROR; 00316 } 00317 00318 /* Transmission of the data */ 00319 if (HAL_OSPI_Transmit_DMA(&OspiRamHandle, pData) != HAL_OK) 00320 { 00321 return OSPI_RAM_ERROR; 00322 } 00323 00324 return OSPI_RAM_OK; 00325 } 00326 00327 /** 00328 * @brief Configure the OSPI in memory-mapped mode 00329 * @retval HyperRAM memory status 00330 */ 00331 uint8_t BSP_OSPI_RAM_EnableMemoryMappedMode(void) 00332 { 00333 OSPI_HyperbusCmdTypeDef sCommand; 00334 OSPI_MemoryMappedTypeDef sMemMappedCfg; 00335 00336 /* OctoSPI Hyperbus command configuration */ 00337 sCommand.AddressSpace = HAL_OSPI_MEMORY_ADDRESS_SPACE; 00338 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00339 sCommand.Address = 0; 00340 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00341 sCommand.NbData = 1; 00342 00343 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00344 { 00345 return OSPI_RAM_ERROR; 00346 } 00347 00348 /* OctoSPI activation of memory-mapped mode */ 00349 sMemMappedCfg.TimeOutActivation = HAL_OSPI_TIMEOUT_COUNTER_DISABLE; 00350 00351 if (HAL_OSPI_MemoryMapped(&OspiRamHandle, &sMemMappedCfg) != HAL_OK) 00352 { 00353 return OSPI_RAM_ERROR; 00354 } 00355 00356 return OSPI_RAM_OK; 00357 } 00358 00359 /** 00360 * @brief This function enter the HyperRAM memory in deep power down mode. 00361 * @retval HyperRAM memory status 00362 */ 00363 uint8_t BSP_OSPI_RAM_EnterDeepPowerDown(void) 00364 { 00365 OSPI_HyperbusCfgTypeDef sHyperbusCfg; 00366 OSPI_HyperbusCmdTypeDef sCommand; 00367 uint16_t reg; 00368 00369 /* To write register, no latency is required. So a reconfiguration is performed */ 00370 sHyperbusCfg.RWRecoveryTime = 3; /* 40 ns @ 60MHz */ 00371 sHyperbusCfg.AccessTime = ISS66WVH8M8_LATENCY_166M; 00372 sHyperbusCfg.WriteZeroLatency = HAL_OSPI_NO_LATENCY_ON_WRITE; 00373 sHyperbusCfg.LatencyMode = HAL_OSPI_FIXED_LATENCY; 00374 00375 if (HAL_OSPI_HyperbusCfg(&OspiRamHandle, &sHyperbusCfg, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00376 { 00377 return OSPI_RAM_ERROR; 00378 } 00379 00380 /* Read the value of the CR0 register */ 00381 sCommand.AddressSpace = HAL_OSPI_REGISTER_ADDRESS_SPACE; 00382 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00383 sCommand.Address = ISS66WVH8M8_CR0_ADDRESS; 00384 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00385 sCommand.NbData = 2; 00386 00387 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00388 { 00389 return OSPI_RAM_ERROR; 00390 } 00391 00392 if (HAL_OSPI_Receive(&OspiRamHandle, (uint8_t *)(®), HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00393 { 00394 return OSPI_RAM_ERROR; 00395 } 00396 00397 /* Update the deep power down value of the CR0 register */ 00398 SET_BIT(reg, ISS66WVH8M8_CR0_DPDE); 00399 00400 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00401 { 00402 return OSPI_RAM_ERROR; 00403 } 00404 00405 if (HAL_OSPI_Transmit(&OspiRamHandle, (uint8_t *)(®), HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00406 { 00407 return OSPI_RAM_ERROR; 00408 } 00409 00410 /* --- Memory takes 10us min to enter deep power down --- */ 00411 /* --- At least 30us should be respected before leaving deep power down --- */ 00412 00413 /* A reconfiguration is performed to re-enable the latency on write access */ 00414 sHyperbusCfg.WriteZeroLatency = HAL_OSPI_LATENCY_ON_WRITE; 00415 00416 if (HAL_OSPI_HyperbusCfg(&OspiRamHandle, &sHyperbusCfg, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00417 { 00418 return OSPI_RAM_ERROR; 00419 } 00420 00421 return OSPI_RAM_OK; 00422 } 00423 00424 /** 00425 * @brief This function leave the HyperRAM memory from deep power down mode. 00426 * @retval HyperRAM memory status 00427 */ 00428 uint8_t BSP_OSPI_RAM_LeaveDeepPowerDown(void) 00429 { 00430 /* --- A dummy command is sent to the memory, as the nCS should be low for at least 200 ns --- */ 00431 /* --- Memory takes 150us max to leave deep power down --- */ 00432 00433 OSPI_HyperbusCmdTypeDef sCommand; 00434 uint32_t data; 00435 00436 /* Initialize the read command */ 00437 sCommand.AddressSpace = HAL_OSPI_MEMORY_ADDRESS_SPACE; 00438 sCommand.AddressSize = HAL_OSPI_ADDRESS_32_BITS; 00439 sCommand.Address = 0; 00440 sCommand.DQSMode = HAL_OSPI_DQS_ENABLE; 00441 sCommand.NbData = 4; 00442 00443 /* Configure the command */ 00444 if (HAL_OSPI_HyperbusCmd(&OspiRamHandle, &sCommand, HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00445 { 00446 return OSPI_RAM_ERROR; 00447 } 00448 00449 /* Reception of the data */ 00450 if (HAL_OSPI_Receive(&OspiRamHandle, (uint8_t*)(&data), HAL_OSPI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00451 { 00452 return OSPI_RAM_ERROR; 00453 } 00454 00455 return OSPI_RAM_OK; 00456 } 00457 00458 /** 00459 * @brief Handles OctoSPI HyperRAM DMA transfer interrupt request. 00460 * @retval None 00461 */ 00462 void BSP_OSPI_RAM_DMA_IRQHandler(void) 00463 { 00464 HAL_DMA_IRQHandler(OspiRamHandle.hdma); 00465 } 00466 00467 /** 00468 * @brief Handles OctoSPI HyperRAM interrupt request. 00469 * @retval None 00470 */ 00471 void BSP_OSPI_RAM_IRQHandler(void) 00472 { 00473 HAL_OSPI_IRQHandler(&OspiRamHandle); 00474 } 00475 /** 00476 * @} 00477 */ 00478 00479 /** @addtogroup STM32L4R9I_EVAL_OSPI_RAM_Private_Functions 00480 * @{ 00481 */ 00482 00483 /** 00484 * @brief Initializes the OSPI MSP. 00485 * @retval None 00486 */ 00487 static void OSPI_RAM_MspInit(void) 00488 { 00489 GPIO_InitTypeDef GPIO_InitStruct; 00490 00491 /* Enable the OctoSPI memory interface, DMA, DMAMUX and GPIO clocks */ 00492 __HAL_RCC_OSPIM_CLK_ENABLE(); 00493 __HAL_RCC_OSPI2_CLK_ENABLE(); 00494 __HAL_RCC_DMAMUX1_CLK_ENABLE(); 00495 __HAL_RCC_DMA1_CLK_ENABLE(); 00496 __HAL_RCC_GPIOG_CLK_ENABLE(); 00497 __HAL_RCC_GPIOH_CLK_ENABLE(); 00498 __HAL_RCC_GPIOI_CLK_ENABLE(); 00499 00500 /* Reset the OctoSPI memory interface */ 00501 __HAL_RCC_OSPI2_FORCE_RESET(); 00502 __HAL_RCC_OSPI2_RELEASE_RESET(); 00503 00504 /* IOSV bit MUST be set to access GPIO port G[2:15] */ 00505 __HAL_RCC_PWR_CLK_ENABLE(); 00506 SET_BIT(PWR->CR2, PWR_CR2_IOSV); 00507 00508 /* OctoSPI CS GPIO pin configuration */ 00509 GPIO_InitStruct.Pin = GPIO_PIN_5; 00510 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00511 GPIO_InitStruct.Pull = GPIO_PULLUP; 00512 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00513 GPIO_InitStruct.Alternate = GPIO_AF5_OCTOSPIM_P2; 00514 HAL_GPIO_Init(GPIOI, &GPIO_InitStruct); 00515 00516 /* OctoSPI DQS GPIO pins configuration */ 00517 GPIO_InitStruct.Pin = GPIO_PIN_15; 00518 GPIO_InitStruct.Pull = GPIO_NOPULL; 00519 HAL_GPIO_Init(GPIOG, &GPIO_InitStruct); 00520 00521 /* OctoSPI D3, D4, D5, D6 and D7 GPIO pins configuration */ 00522 GPIO_InitStruct.Pin = (GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12); 00523 HAL_GPIO_Init(GPIOH, &GPIO_InitStruct); 00524 00525 /* OctoSPI CK, nCK, D2, D1 and D0 GPIO pins configuration */ 00526 GPIO_InitStruct.Pin = (GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11); 00527 HAL_GPIO_Init(GPIOI, &GPIO_InitStruct); 00528 00529 /* Configure the OctoSPI DMA */ 00530 DmaOspiRamHandle.Instance = DMA1_Channel2; 00531 DmaOspiRamHandle.Init.Request = DMA_REQUEST_OCTOSPI2; 00532 DmaOspiRamHandle.Init.PeriphInc = DMA_PINC_DISABLE; 00533 DmaOspiRamHandle.Init.MemInc = DMA_MINC_ENABLE; 00534 DmaOspiRamHandle.Init.Mode = DMA_NORMAL; 00535 DmaOspiRamHandle.Init.Priority = DMA_PRIORITY_LOW; 00536 DmaOspiRamHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_BYTE; 00537 DmaOspiRamHandle.Init.MemDataAlignment = DMA_MDATAALIGN_BYTE; 00538 00539 __HAL_LINKDMA(&OspiRamHandle, hdma, DmaOspiRamHandle); 00540 HAL_DMA_Init(&DmaOspiRamHandle); 00541 00542 /* Enable and set priority of the OctoSPI and DMA interrupts */ 00543 HAL_NVIC_SetPriority(OCTOSPI2_IRQn, 0x0F, 0); 00544 HAL_NVIC_SetPriority(DMA1_Channel2_IRQn, 0x00, 0); 00545 00546 HAL_NVIC_EnableIRQ(OCTOSPI2_IRQn); 00547 HAL_NVIC_EnableIRQ(DMA1_Channel2_IRQn); 00548 } 00549 00550 /** 00551 * @brief De-Initializes the OSPI MSP. 00552 * @retval None 00553 */ 00554 static void OSPI_RAM_MspDeInit(void) 00555 { 00556 GPIO_InitTypeDef GPIO_InitStruct; 00557 00558 /* Disable DMA and OctoSPI interrupts */ 00559 HAL_NVIC_DisableIRQ(DMA1_Channel2_IRQn); 00560 HAL_NVIC_DisableIRQ(OCTOSPI2_IRQn); 00561 00562 /* De-configure the OctoSPI DMA */ 00563 DmaOspiRamHandle.Instance = DMA1_Channel2; 00564 HAL_DMA_DeInit(&DmaOspiRamHandle); 00565 00566 /* OctoSPI CLK, CS, D0-D7, DQS GPIO pins de-configuration */ 00567 HAL_GPIO_DeInit(GPIOG, GPIO_PIN_15); 00568 HAL_GPIO_DeInit(GPIOH, (GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12)); 00569 HAL_GPIO_DeInit(GPIOI, (GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11)); 00570 00571 /* Set GPIOI pin 5 in pull up mode (optimum default setting) */ 00572 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00573 GPIO_InitStruct.Pin = GPIO_PIN_5; 00574 GPIO_InitStruct.Pull = GPIO_NOPULL; 00575 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00576 HAL_GPIO_Init(GPIOI, &GPIO_InitStruct); 00577 00578 /* Set GPIOI pin 6 and 7 in no pull, low state (optimum default setting) */ 00579 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00580 GPIO_InitStruct.Pull = GPIO_NOPULL; 00581 GPIO_InitStruct.Pin = (GPIO_PIN_6 | GPIO_PIN_7); 00582 HAL_GPIO_Init(GPIOI, &GPIO_InitStruct); 00583 HAL_GPIO_WritePin(GPIOI, GPIO_PIN_6, GPIO_PIN_RESET); 00584 HAL_GPIO_WritePin(GPIOI, GPIO_PIN_7, GPIO_PIN_RESET); 00585 00586 /* Reset the OctoSPI memory interface */ 00587 __HAL_RCC_OSPI2_FORCE_RESET(); 00588 __HAL_RCC_OSPI2_RELEASE_RESET(); 00589 00590 /* Disable the OctoSPI memory interface clock */ 00591 __HAL_RCC_OSPI2_CLK_DISABLE(); 00592 } 00593 00594 /** 00595 * @} 00596 */ 00597 00598 /** 00599 * @} 00600 */ 00601 00602 /** 00603 * @} 00604 */ 00605 00606 /** 00607 * @} 00608 */ 00609 00610 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00611
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
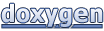