STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_nor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_nor.c 00004 * @author MCD Application Team 00005 * @brief This file includes a standard driver for the M29W128GL70ZA6E NOR 00006 * memories mounted on STM32L4R9I-EVAL board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive the M29W128GL70ZA6E NOR flash external 00013 memory mounted on STM32L4R9I-EVAL evaluation board. 00014 00015 (#) This driver does not need a specific component driver for the NOR device 00016 to be included with. 00017 00018 (#) Initialization steps: 00019 (++) Initialize the NOR external memory using the BSP_NOR_Init() function. This 00020 function includes the MSP layer hardware resources initialization and the 00021 FMC controller configuration to interface with the external NOR memory. 00022 00023 (#) NOR flash operations 00024 (++) NOR external memory can be accessed with read/write operations once it is 00025 initialized. 00026 Read/write operation can be performed with AHB access using the functions 00027 BSP_NOR_ReadData()/BSP_NOR_WriteData(). The BSP_NOR_WriteData() performs write operation 00028 of an amount of data by unit (halfword). You can also perform a program data 00029 operation of an amount of data using the function BSP_NOR_ProgramData(). 00030 (++) The function BSP_NOR_Read_ID() returns the chip IDs stored in the structure 00031 "NOR_IDTypeDef". (see the NOR IDs in the memory data sheet) 00032 (++) Perform erase block operation using the function BSP_NOR_Erase_Block() and by 00033 specifying the block address. You can perform an erase operation of the whole 00034 chip by calling the function BSP_NOR_Erase_Chip(). 00035 (++) After other operations, the function BSP_NOR_ReturnToReadMode() allows the NOR 00036 flash to return to read mode to perform read operations on it. 00037 @endverbatim 00038 ****************************************************************************** 00039 * @attention 00040 * 00041 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00042 * 00043 * Redistribution and use in source and binary forms, with or without modification, 00044 * are permitted provided that the following conditions are met: 00045 * 1. Redistributions of source code must retain the above copyright notice, 00046 * this list of conditions and the following disclaimer. 00047 * 2. Redistributions in binary form must reproduce the above copyright notice, 00048 * this list of conditions and the following disclaimer in the documentation 00049 * and/or other materials provided with the distribution. 00050 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00051 * may be used to endorse or promote products derived from this software 00052 * without specific prior written permission. 00053 * 00054 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00055 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00056 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00057 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00058 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00059 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00060 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00061 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00062 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00063 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00064 * 00065 ****************************************************************************** 00066 */ 00067 00068 /* Includes ------------------------------------------------------------------*/ 00069 #include "stm32l4r9i_eval_nor.h" 00070 00071 /** @addtogroup BSP 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32L4R9I_EVAL 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM32L4R9I_EVAL_NOR STM32L4R9I_EVAL NOR 00080 * @{ 00081 */ 00082 00083 /* Private constants ---------------------------------------------------------*/ 00084 00085 /** @defgroup STM32L4R9I_EVAL_NOR_Private_Constants Private Constants 00086 * @{ 00087 */ 00088 /* Timings for NOR M29W128GL70ZA6E */ 00089 #define NOR_ADDR_SETUP_TIME 9 /* 70ns with a clock at 120 MHz (period of 8.33 ns) */ 00090 #define NOR_DATA_SETUP_TIME 5 /* 45ns with a clock at 120 MHz (period of 8.33 ns) */ 00091 #define NOR_DATA_HOLD_TIME 0 00092 #define NOR_TURN_AROUND_TIME 4 /* 30ns with a clock at 120 MHz (perido of 8.33 ns) */ 00093 00094 #define NOR_MAX_BUFFER_SIZE 32 /* 16-bits bus so 32 words */ 00095 00096 /** 00097 * @} 00098 */ 00099 00100 /* Private variables ---------------------------------------------------------*/ 00101 00102 /** @defgroup STM32L4R9I_EVAL_NOR_Private_Variables Private Variables 00103 * @{ 00104 */ 00105 static NOR_HandleTypeDef norHandle; 00106 static FMC_NORSRAM_TimingTypeDef Timing; 00107 00108 /** 00109 * @} 00110 */ 00111 00112 /* Private functions ---------------------------------------------------------*/ 00113 00114 /** @defgroup STM32L4R9I_EVAL_NOR_Private_Functions Private Functions 00115 * @{ 00116 */ 00117 static void NOR_MspInit(void); 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32L4R9I_EVAL_NOR_Exported_Functions Exported Functions 00124 * @{ 00125 */ 00126 00127 /** 00128 * @brief Initializes the NOR device. 00129 * @retval NOR memory status 00130 */ 00131 uint8_t BSP_NOR_Init(void) 00132 { 00133 norHandle.Instance = FMC_NORSRAM_DEVICE; 00134 norHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00135 00136 /* NOR device configuration */ 00137 Timing.AddressSetupTime = NOR_ADDR_SETUP_TIME; 00138 Timing.AddressHoldTime = 1; /* Min value, Don't care on NOR Access mode B */ 00139 Timing.DataSetupTime = NOR_DATA_SETUP_TIME; 00140 Timing.DataHoldTime = NOR_DATA_HOLD_TIME; 00141 Timing.CLKDivision = 2; /* Min value, Don't care on NOR Access mode B */ 00142 Timing.DataLatency = 2; /* Min value, Don't care on NOR Access mode B */ 00143 Timing.BusTurnAroundDuration = NOR_TURN_AROUND_TIME; 00144 Timing.AccessMode = FMC_ACCESS_MODE_B; 00145 00146 norHandle.Init.NSBank = FMC_NORSRAM_BANK3; 00147 norHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00148 norHandle.Init.MemoryType = FMC_MEMORY_TYPE_NOR; 00149 norHandle.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00150 norHandle.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00151 norHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00152 norHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00153 norHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00154 norHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00155 norHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00156 norHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_ENABLE; 00157 norHandle.Init.WriteBurst = FMC_BURST_ACCESS_MODE_DISABLE; 00158 norHandle.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00159 norHandle.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00160 norHandle.Init.NBLSetupTime = 0; 00161 norHandle.Init.PageSize = FMC_PAGE_SIZE_NONE; 00162 00163 /* NOR controller initialization */ 00164 NOR_MspInit(); 00165 00166 if(HAL_NOR_Init(&norHandle, &Timing, &Timing) != HAL_OK) 00167 { 00168 return NOR_STATUS_ERROR; 00169 } 00170 else 00171 { 00172 return NOR_STATUS_OK; 00173 } 00174 } 00175 00176 /** 00177 * @brief Reads an amount of data from the NOR device. 00178 * @param uwStartAddress: Read start address 00179 * @param pData: Pointer to data to be read 00180 * @param uwDataSize: Size of data to read 00181 * @retval NOR memory status 00182 */ 00183 uint8_t BSP_NOR_ReadData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00184 { 00185 if(HAL_NOR_ReadBuffer(&norHandle, NOR_DEVICE_ADDR + uwStartAddress, pData, uwDataSize) != HAL_OK) 00186 { 00187 return NOR_STATUS_ERROR; 00188 } 00189 else 00190 { 00191 return NOR_STATUS_OK; 00192 } 00193 } 00194 00195 /** 00196 * @brief Returns the NOR memory to read mode. 00197 * @retval None 00198 */ 00199 void BSP_NOR_ReturnToReadMode(void) 00200 { 00201 HAL_NOR_ReturnToReadMode(&norHandle); 00202 } 00203 00204 /** 00205 * @brief Writes an amount of data to the NOR device. 00206 * @param uwStartAddress: Write start address 00207 * @param pData: Pointer to data to be written 00208 * @param uwDataSize: Size of data to write 00209 * @retval NOR memory status 00210 */ 00211 uint8_t BSP_NOR_WriteData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00212 { 00213 uint32_t index = uwDataSize; 00214 00215 while(index > 0) 00216 { 00217 /* Write data to NOR */ 00218 HAL_NOR_Program(&norHandle, (uint32_t *)(NOR_DEVICE_ADDR + uwStartAddress), pData); 00219 00220 /* Read NOR device status */ 00221 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00222 { 00223 return NOR_STATUS_ERROR; 00224 } 00225 00226 /* Update the counters */ 00227 index--; 00228 uwStartAddress += 2; 00229 pData++; 00230 } 00231 00232 return NOR_STATUS_OK; 00233 } 00234 00235 /** 00236 * @brief Programs an amount of data to the NOR device. 00237 * @param uwStartAddress: Write start address 00238 * @param pData: Pointer to data to be written 00239 * @param uwDataSize: Size of data to write 00240 * @retval NOR memory status 00241 */ 00242 uint8_t BSP_NOR_ProgramData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00243 { 00244 uint32_t buffer_size, data_nb; 00245 uint16_t *src_addr, *dest_addr; 00246 src_addr = pData; 00247 dest_addr = (uint16_t *)(NOR_DEVICE_ADDR + uwStartAddress); 00248 data_nb = uwDataSize; 00249 00250 do 00251 { 00252 if (uwDataSize < NOR_MAX_BUFFER_SIZE) 00253 { 00254 buffer_size = data_nb; 00255 } 00256 else 00257 { 00258 buffer_size = NOR_MAX_BUFFER_SIZE; 00259 } 00260 00261 /* Send NOR program buffer operation */ 00262 HAL_NOR_ProgramBuffer(&norHandle, (uint32_t)dest_addr, src_addr, buffer_size); 00263 00264 /* Return the NOR memory status */ 00265 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00266 { 00267 return NOR_STATUS_ERROR; 00268 } 00269 00270 data_nb -= buffer_size; 00271 src_addr += buffer_size; 00272 dest_addr += buffer_size; 00273 } while(data_nb > 0); 00274 00275 return NOR_STATUS_OK; 00276 } 00277 00278 /** 00279 * @brief Erases the specified block of the NOR device. 00280 * @param BlockAddress: Block address to erase 00281 * @retval NOR memory status 00282 */ 00283 uint8_t BSP_NOR_Erase_Block(uint32_t BlockAddress) 00284 { 00285 /* Send NOR erase block operation */ 00286 HAL_NOR_Erase_Block(&norHandle, BlockAddress, NOR_DEVICE_ADDR); 00287 00288 /* Return the NOR memory status */ 00289 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, BLOCKERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00290 { 00291 return NOR_STATUS_ERROR; 00292 } 00293 else 00294 { 00295 return NOR_STATUS_OK; 00296 } 00297 } 00298 00299 /** 00300 * @brief Erases the entire NOR chip. 00301 * @retval NOR memory status 00302 */ 00303 uint8_t BSP_NOR_Erase_Chip(void) 00304 { 00305 /* Send NOR Erase chip operation */ 00306 HAL_NOR_Erase_Chip(&norHandle, NOR_DEVICE_ADDR); 00307 00308 /* Return the NOR memory status */ 00309 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, CHIPERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00310 { 00311 return NOR_STATUS_ERROR; 00312 } 00313 else 00314 { 00315 return NOR_STATUS_OK; 00316 } 00317 } 00318 00319 /** 00320 * @brief Reads NOR flash IDs. 00321 * @param pNOR_ID : Pointer to NOR ID structure 00322 * @retval NOR memory status 00323 */ 00324 uint8_t BSP_NOR_Read_ID(NOR_IDTypeDef *pNOR_ID) 00325 { 00326 if(HAL_NOR_Read_ID(&norHandle, pNOR_ID) != HAL_OK) 00327 { 00328 return NOR_STATUS_ERROR; 00329 } 00330 else 00331 { 00332 return NOR_STATUS_OK; 00333 } 00334 } 00335 00336 /** 00337 * @} 00338 */ 00339 00340 /** @addtogroup STM32L4R9I_EVAL_NOR_Private_Functions 00341 * @{ 00342 */ 00343 00344 /** 00345 * @brief Initializes the NOR MSP. 00346 * @retval None 00347 */ 00348 static void NOR_MspInit(void) 00349 { 00350 GPIO_InitTypeDef gpioinitstruct = {0}; 00351 00352 /* Enable FMC clock */ 00353 __HAL_RCC_FMC_CLK_ENABLE(); 00354 00355 /* Enable GPIOs clock */ 00356 __HAL_RCC_GPIOD_CLK_ENABLE(); 00357 __HAL_RCC_GPIOE_CLK_ENABLE(); 00358 __HAL_RCC_GPIOF_CLK_ENABLE(); 00359 __HAL_RCC_GPIOG_CLK_ENABLE(); 00360 __HAL_RCC_PWR_CLK_ENABLE(); 00361 HAL_PWREx_EnableVddIO2(); 00362 00363 /* Common GPIO configuration */ 00364 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00365 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00366 gpioinitstruct.Alternate = GPIO_AF12_FMC; 00367 00368 /*## NE configuration #######*/ 00369 /* NE1 : SRAM */ 00370 gpioinitstruct.Pull = GPIO_PULLUP; 00371 gpioinitstruct.Pin = GPIO_PIN_7; 00372 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00373 /* NE3 : NOR */ 00374 /* NE4 : TFT LCD */ 00375 gpioinitstruct.Pin = GPIO_PIN_10 | GPIO_PIN_12; 00376 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00377 00378 /*## NOE and NWE configuration #######*/ 00379 gpioinitstruct.Pin = GPIO_PIN_4 | GPIO_PIN_5; 00380 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00381 00382 /*## Ready/Busy configuration #######*/ 00383 gpioinitstruct.Pin = NOR_READY_BUSY_PIN; 00384 HAL_GPIO_Init(NOR_READY_BUSY_GPIO, &gpioinitstruct); 00385 00386 /*## Address Bus #######*/ 00387 /* GPIOD configuration */ 00388 gpioinitstruct.Pull = GPIO_NOPULL; 00389 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00390 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00391 00392 /* GPIOE configuration */ 00393 gpioinitstruct.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | 00394 GPIO_PIN_5 | GPIO_PIN_6; 00395 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00396 00397 /* GPIOF configuration */ 00398 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00399 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00400 GPIO_PIN_14 | GPIO_PIN_15; 00401 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00402 00403 /* GPIOG configuration */ 00404 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00405 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00406 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00407 00408 /*## Data Bus #######*/ 00409 /* GPIOD configuration */ 00410 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00411 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00412 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00413 00414 /* GPIOE configuration */ 00415 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00416 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00417 GPIO_PIN_14 | GPIO_PIN_15; 00418 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00419 } 00420 00421 /** 00422 * @brief NOR BSP Wait for Ready/Busy signal. 00423 * @param hnor: Pointer to NOR handle 00424 * @param Timeout: Timeout duration 00425 * @retval None 00426 */ 00427 void HAL_NOR_MspWait(NOR_HandleTypeDef *hnor, uint32_t Timeout) 00428 { 00429 #if 0 00430 uint32_t timeout = Timeout; 00431 00432 /* Polling on Ready/Busy signal */ 00433 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_BUSY_STATE) && (timeout > 0)) 00434 { 00435 timeout--; 00436 } 00437 00438 timeout = Timeout; 00439 00440 /* Polling on Ready/Busy signal */ 00441 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_READY_STATE) && (timeout > 0)) 00442 { 00443 timeout--; 00444 } 00445 #endif 00446 } 00447 00448 /** 00449 * @} 00450 */ 00451 00452 /** 00453 * @} 00454 */ 00455 00456 /** 00457 * @} 00458 */ 00459 00460 /** 00461 * @} 00462 */ 00463 00464 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00465
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
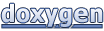