STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery_idd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_idd.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief Header file for stm32l476g_discovery_idd.c module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L476G_DISCOVERY_IDD_H 00040 #define __STM32L476G_DISCOVERY_IDD_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l476g_discovery.h" 00048 /* Include Idd measurement component driver */ 00049 #include "../Components/mfxstm32l152/mfxstm32l152.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32L476G_DISCOVERY 00056 * @{ 00057 */ 00058 00059 /** @addtogroup STM32L476G_DISCOVERY_IDD 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM32L476G_DISCOVERY_IDD_Exported_Types Exported Types 00064 * @{ 00065 */ 00066 00067 /** @defgroup IDD_Config IDD Config 00068 * @{ 00069 */ 00070 typedef enum 00071 { 00072 IDD_OK = 0, 00073 IDD_TIMEOUT = 1, 00074 IDD_ZERO_VALUE = 2, 00075 IDD_ERROR = 0xFF 00076 } 00077 IDD_StatusTypeDef; 00078 /** 00079 * @} 00080 */ 00081 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup STM32L476G_DISCOVERY_IDD_Exported_Defines Exported Defines 00087 * @{ 00088 */ 00089 /** 00090 * @brief Shunt values on discovery in milli ohms 00091 */ 00092 #define DISCOVERY_IDD_SHUNT0_VALUE ((uint16_t) 1000) /*!< value in milliohm */ 00093 #define DISCOVERY_IDD_SHUNT1_VALUE ((uint16_t) 24) /*!< value in ohm */ 00094 #define DISCOVERY_IDD_SHUNT2_VALUE ((uint16_t) 620) /*!< value in ohm */ 00095 #define DISCOVERY_IDD_SHUNT4_VALUE ((uint16_t) 10000) /*!< value in ohm */ 00096 00097 /** 00098 * @brief Shunt stabilization delay on discovery in milli ohms 00099 */ 00100 #define DISCOVERY_IDD_SHUNT0_STABDELAY ((uint8_t) 149) /*!< value in millisec */ 00101 #define DISCOVERY_IDD_SHUNT1_STABDELAY ((uint8_t) 149) /*!< value in millisec */ 00102 #define DISCOVERY_IDD_SHUNT2_STABDELAY ((uint8_t) 149) /*!< value in millisec */ 00103 #define DISCOVERY_IDD_SHUNT4_STABDELAY ((uint8_t) 255) /*!< value in millisec */ 00104 00105 /** 00106 * @brief IDD Ampli Gain on discovery 00107 */ 00108 #if defined(USE_STM32L476G_DISCO_REVC) 00109 #define DISCOVERY_IDD_AMPLI_GAIN ((uint16_t) 4967) /*!< value is gain * 100 */ 00110 #else 00111 #define DISCOVERY_IDD_AMPLI_GAIN ((uint16_t) 4990) /*!< value is gain * 100 */ 00112 #endif 00113 00114 /** 00115 * @brief IDD Vdd Min on discovery 00116 */ 00117 #define DISCOVERY_IDD_VDD_MIN ((uint16_t) 2000) /*!< value in millivolt */ 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /* Exported functions --------------------------------------------------------*/ 00124 /** @defgroup STM32L476G_DISCOVERY_IDD_Exported_Functions Exported Functions 00125 * @{ 00126 */ 00127 uint8_t BSP_IDD_Init(void); 00128 void BSP_IDD_DeInit(void); 00129 void BSP_IDD_Reset(void); 00130 void BSP_IDD_LowPower(void); 00131 void BSP_IDD_WakeUp(void); 00132 void BSP_IDD_StartMeasure(void); 00133 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig); 00134 void BSP_IDD_GetValue(uint32_t *IddValue); 00135 void BSP_IDD_EnableIT(void); 00136 void BSP_IDD_ClearIT(void); 00137 uint8_t BSP_IDD_GetITStatus(void); 00138 void BSP_IDD_DisableIT(void); 00139 uint8_t BSP_IDD_ErrorGetCode(void); 00140 void BSP_IDD_ErrorEnableIT(void); 00141 void BSP_IDD_ErrorClearIT(void); 00142 uint8_t BSP_IDD_ErrorGetITStatus(void); 00143 void BSP_IDD_ErrorDisableIT(void); 00144 00145 /** 00146 * @} 00147 */ 00148 00149 /** 00150 * @} 00151 */ 00152 00153 /** 00154 * @} 00155 */ 00156 00157 /** 00158 * @} 00159 */ 00160 00161 #ifdef __cplusplus 00162 } 00163 #endif 00164 00165 #endif /* __STM32L476G_DISCOVERY_IDD_H */ 00166 00167 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
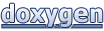