STM32L476G-Discovery BSP User Manual
|
This file provides a set of functions needed to manage the Audio driver for the STM32L476G-Discovery board. More...
Go to the source code of this file.
Data Structures | |
struct | AUDIO_OUT_TypeDef |
struct | AUDIO_IN_TypeDef |
Defines | |
#define | SAIClockDivider(__FREQUENCY__) |
#define | DFSDMOverSampling(__FREQUENCY__) |
#define | DFSDMClockDivider(__FREQUENCY__) |
#define | DFSDMFilterOrder(__FREQUENCY__) |
#define | DFSDMRightBitShift(__FREQUENCY__) |
#define | SaturaLH(N, L, H) (((N)<(L))?(L):(((N)>(H))?(H):(N))) |
Functions | |
static void | AUDIO_CODEC_Reset (void) |
Resets the audio codec. | |
static uint8_t | AUDIO_SAIx_Init (uint32_t AudioFreq) |
Initializes the Audio Codec audio interface (SAI). | |
static uint8_t | AUDIO_SAIx_DeInit (void) |
De-initializes the Audio Codec audio interface (SAI). | |
static uint8_t | AUDIO_DFSDMx_Init (uint32_t AudioFreq) |
Initializes the Digital Filter for Sigma-Delta Modulators interface (DFSDM). | |
static uint8_t | AUDIO_DFSDMx_DeInit (void) |
De-initializes the Digital Filter for Sigma-Delta Modulators interface (DFSDM). | |
static uint8_t | AUDIO_SAIPLLConfig (uint32_t Frequency) |
Configures the SAI PLL clock according to the required audio frequency. | |
uint8_t | BSP_AUDIO_OUT_Init (uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) |
Configures the audio codec related peripherals. | |
uint8_t | BSP_AUDIO_OUT_DeInit (void) |
De-Initializes audio codec related peripherals. | |
uint8_t | BSP_AUDIO_OUT_Play (uint16_t *pData, uint32_t Size) |
Starts playing audio stream from a data buffer for a determined size. | |
uint8_t | BSP_AUDIO_OUT_ChangeBuffer (uint16_t *pData, uint16_t Size) |
Sends n-Bytes on the SAI interface. | |
uint8_t | BSP_AUDIO_OUT_Pause (void) |
This function Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Resume (void) |
This function Resumes the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Stop (uint32_t Option) |
Stops audio playing and Power down the Audio Codec. | |
uint8_t | BSP_AUDIO_OUT_SetVolume (uint8_t Volume) |
Controls the current audio volume level. | |
uint8_t | BSP_AUDIO_OUT_SetMute (uint32_t Cmd) |
Enables or disables the MUTE mode by software. | |
uint8_t | BSP_AUDIO_OUT_SetOutputMode (uint8_t Output) |
Switch dynamically (while audio file is being played) the output target (speaker or headphone). | |
uint8_t | BSP_AUDIO_OUT_SetFrequency (uint32_t AudioFreq) |
Updates the audio frequency. | |
void | BSP_AUDIO_OUT_ChangeAudioConfig (uint32_t AudioOutOption) |
Changes the Audio Out Configuration. | |
void | BSP_AUDIO_OUT_RegisterCallbacks (Audio_CallbackTypeDef ErrorCallback, Audio_CallbackTypeDef HalfTransferCallback, Audio_CallbackTypeDef TransferCompleteCallback) |
register user callback functions | |
void | HAL_SAI_TxCpltCallback (SAI_HandleTypeDef *hsai) |
Tx Transfer completed callbacks. | |
void | HAL_SAI_TxHalfCpltCallback (SAI_HandleTypeDef *hsai) |
Tx Half Transfer completed callbacks. | |
void | HAL_SAI_ErrorCallback (SAI_HandleTypeDef *hsai) |
SAI error callbacks. | |
uint8_t | BSP_AUDIO_IN_Init (uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr) |
Initializes micropone related peripherals. | |
uint8_t | BSP_AUDIO_IN_DeInit (void) |
De-Initializes microphone related peripherals. | |
uint8_t | BSP_AUDIO_IN_Record (uint16_t *pbuf, uint32_t size) |
Starts audio recording. | |
uint8_t | BSP_AUDIO_IN_SetFrequency (uint32_t AudioFreq) |
Updates the audio frequency. | |
void | HAL_DFSDM_FilterRegConvCpltCallback (DFSDM_Filter_HandleTypeDef *hdfsdm_filter) |
Regular conversion complete callback. | |
void | HAL_DFSDM_FilterRegConvHalfCpltCallback (DFSDM_Filter_HandleTypeDef *hdfsdm_filter) |
Half regular conversion complete callback. | |
void | HAL_DFSDM_FilterErrorCallback (DFSDM_Filter_HandleTypeDef *hdfsdm_filter) |
Error callback. | |
uint8_t | BSP_AUDIO_IN_Stop (void) |
Stops audio recording. | |
uint8_t | BSP_AUDIO_IN_Pause (void) |
Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_IN_Resume (void) |
Resumes the audio file stream. | |
void | BSP_AUDIO_IN_RegisterCallbacks (Audio_CallbackTypeDef ErrorCallback, Audio_CallbackTypeDef HalfTransferCallback, Audio_CallbackTypeDef TransferCompleteCallback) |
register user callback functions | |
void | HAL_SAI_MspInit (SAI_HandleTypeDef *hsai) |
SAI MSP Init. | |
void | HAL_SAI_MspDeInit (SAI_HandleTypeDef *hsai) |
SAI MSP De-init. | |
void | HAL_DFSDM_ChannelMspInit (DFSDM_Channel_HandleTypeDef *hdfsdm_channel) |
Initializes the DFSDM channel MSP. | |
void | HAL_DFSDM_ChannelMspDeInit (DFSDM_Channel_HandleTypeDef *hdfsdm_channel) |
De-initializes the DFSDM channel MSP. | |
void | HAL_DFSDM_FilterMspInit (DFSDM_Filter_HandleTypeDef *hdfsdm_filter) |
Initializes the DFSDM filter MSP. | |
void | HAL_DFSDM_FilterMspDeInit (DFSDM_Filter_HandleTypeDef *hdfsdm_filter) |
De-initializes the DFSDM filter MSP. | |
Variables | |
static AUDIO_OUT_TypeDef | hAudioOut |
static AUDIO_IN_TypeDef | hAudioIn |
static DMA_HandleTypeDef | hDmaSai |
SAI_HandleTypeDef | BSP_AUDIO_hSai |
DFSDM_Filter_HandleTypeDef | BSP_AUDIO_hDfsdmLeftFilter |
Detailed Description
This file provides a set of functions needed to manage the Audio driver for the STM32L476G-Discovery board.
- Attention:
© COPYRIGHT(c) 2015 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm32l476g_discovery_audio.c.
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
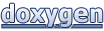