STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery_gyroscope.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_gyroscope.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the L3GD20 00008 * MEMS accelerometer available on STM32L476G-Discovery board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32l476g_discovery_gyroscope.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32L476G_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE STM32L476G-DISCOVERY GYROSCOPE 00051 * @{ 00052 */ 00053 00054 /* Private typedef -----------------------------------------------------------*/ 00055 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Types Private Types 00056 * @{ 00057 */ 00058 /** 00059 * @} 00060 */ 00061 00062 /* Private defines ------------------------------------------------------------*/ 00063 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Constants Private Constants 00064 * @{ 00065 */ 00066 /** 00067 * @} 00068 */ 00069 00070 /* Private macros ------------------------------------------------------------*/ 00071 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Macros Private Macros 00072 * @{ 00073 */ 00074 /** 00075 * @} 00076 */ 00077 00078 /* Private variables ---------------------------------------------------------*/ 00079 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Variables Private Variables 00080 * @{ 00081 */ 00082 static GYRO_DrvTypeDef *GyroscopeDrv; 00083 00084 /** 00085 * @} 00086 */ 00087 00088 /* Private function prototypes -----------------------------------------------*/ 00089 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_FunctionPrototypes Private Functions 00090 * @{ 00091 */ 00092 /** 00093 * @} 00094 */ 00095 00096 /* Exported functions --------------------------------------------------------*/ 00097 /** @addtogroup STM32L476G_DISCOVERY_GYROSCOPE_Exported_Functions 00098 * @{ 00099 */ 00100 00101 /** 00102 * @brief Initialize Gyroscope. 00103 * @retval GYRO_OK or GYRO_ERROR 00104 */ 00105 uint8_t BSP_GYRO_Init(void) 00106 { 00107 uint8_t ret = GYRO_ERROR; 00108 uint16_t ctrl = 0x0000; 00109 GYRO_InitTypeDef L3GD20_InitStructure; 00110 GYRO_FilterConfigTypeDef L3GD20_FilterStructure={0,0}; 00111 00112 if((L3gd20Drv.ReadID() == I_AM_L3GD20) || (L3gd20Drv.ReadID() == I_AM_L3GD20_TR)) 00113 { 00114 /* Initialize the gyroscope driver structure */ 00115 GyroscopeDrv = &L3gd20Drv; 00116 00117 /* Configure Mems : data rate, power mode, full scale and axes */ 00118 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_ACTIVE; 00119 L3GD20_InitStructure.Output_DataRate = L3GD20_OUTPUT_DATARATE_1; 00120 L3GD20_InitStructure.Axes_Enable = L3GD20_AXES_ENABLE; 00121 L3GD20_InitStructure.Band_Width = L3GD20_BANDWIDTH_4; 00122 L3GD20_InitStructure.BlockData_Update = L3GD20_BlockDataUpdate_Continous; 00123 L3GD20_InitStructure.Endianness = L3GD20_BLE_LSB; 00124 L3GD20_InitStructure.Full_Scale = L3GD20_FULLSCALE_500; 00125 00126 /* Configure MEMS: data rate, power mode, full scale and axes */ 00127 ctrl = (uint16_t) (L3GD20_InitStructure.Power_Mode | L3GD20_InitStructure.Output_DataRate | \ 00128 L3GD20_InitStructure.Axes_Enable | L3GD20_InitStructure.Band_Width); 00129 00130 ctrl |= (uint16_t) ((L3GD20_InitStructure.BlockData_Update | L3GD20_InitStructure.Endianness | \ 00131 L3GD20_InitStructure.Full_Scale) << 8); 00132 00133 /* Initialize component */ 00134 GyroscopeDrv->Init(ctrl); 00135 00136 L3GD20_FilterStructure.HighPassFilter_Mode_Selection =L3GD20_HPM_NORMAL_MODE_RES; 00137 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency = L3GD20_HPFCF_0; 00138 00139 ctrl = (uint8_t) ((L3GD20_FilterStructure.HighPassFilter_Mode_Selection |\ 00140 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency)); 00141 00142 /* Configure component filter */ 00143 GyroscopeDrv->FilterConfig(ctrl) ; 00144 00145 /* Enable component filter */ 00146 GyroscopeDrv->FilterCmd(L3GD20_HIGHPASSFILTER_ENABLE); 00147 00148 ret = GYRO_OK; 00149 } 00150 else 00151 { 00152 ret = GYRO_ERROR; 00153 } 00154 00155 return ret; 00156 } 00157 00158 00159 /** 00160 * @brief DeInitialize Gyroscope. 00161 * @retval None 00162 */ 00163 void BSP_GYRO_DeInit(void) 00164 { 00165 GYRO_IO_DeInit(); 00166 } 00167 00168 00169 /** 00170 * @brief Put Gyroscope in low power mode. 00171 * @retval None 00172 */ 00173 void BSP_GYRO_LowPower(void) 00174 { 00175 uint16_t ctrl = 0x0000; 00176 GYRO_InitTypeDef L3GD20_InitStructure; 00177 00178 /* configure only Power_Mode field */ 00179 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_POWERDOWN; 00180 00181 ctrl = (uint16_t) (L3GD20_InitStructure.Power_Mode); 00182 00183 /* Set component in low-power mode */ 00184 GyroscopeDrv->LowPower(ctrl); 00185 00186 00187 } 00188 00189 /** 00190 * @brief Read ID of Gyroscope component. 00191 * @retval ID 00192 */ 00193 uint8_t BSP_GYRO_ReadID(void) 00194 { 00195 uint8_t id = 0x00; 00196 00197 if(GyroscopeDrv->ReadID != NULL) 00198 { 00199 id = GyroscopeDrv->ReadID(); 00200 } 00201 return id; 00202 } 00203 00204 /** 00205 * @brief Reboot memory content of Gyroscope. 00206 * @retval None 00207 */ 00208 void BSP_GYRO_Reset(void) 00209 { 00210 if(GyroscopeDrv->Reset != NULL) 00211 { 00212 GyroscopeDrv->Reset(); 00213 } 00214 } 00215 00216 /** 00217 * @brief Configure Gyroscope interrupts (INT1 or INT2). 00218 * @param pIntConfig: pointer to a GYRO_InterruptConfigTypeDef 00219 * structure that contains the configuration setting for the L3GD20 Interrupt. 00220 * @retval None 00221 */ 00222 void BSP_GYRO_ITConfig(GYRO_InterruptConfigTypeDef *pIntConfig) 00223 { 00224 uint16_t interruptconfig = 0x0000; 00225 00226 if(GyroscopeDrv->ConfigIT != NULL) 00227 { 00228 /* Configure latch Interrupt request and axe interrupts */ 00229 interruptconfig |= ((uint8_t)(pIntConfig->Latch_Request| \ 00230 pIntConfig->Interrupt_Axes) << 8); 00231 00232 interruptconfig |= (uint8_t)(pIntConfig->Interrupt_ActiveEdge); 00233 00234 GyroscopeDrv->ConfigIT(interruptconfig); 00235 } 00236 } 00237 00238 /** 00239 * @brief Enable Gyroscope interrupts (INT1 or INT2). 00240 * @param IntPin: Interrupt pin 00241 * This parameter can be: 00242 * @arg L3GD20_INT1 00243 * @arg L3GD20_INT2 00244 * @retval None 00245 */ 00246 void BSP_GYRO_EnableIT(uint8_t IntPin) 00247 { 00248 if(GyroscopeDrv->EnableIT != NULL) 00249 { 00250 GyroscopeDrv->EnableIT(IntPin); 00251 } 00252 } 00253 00254 /** 00255 * @brief Disable Gyroscope interrupts (INT1 or INT2). 00256 * @param IntPin: Interrupt pin 00257 * This parameter can be: 00258 * @arg L3GD20_INT1 00259 * @arg L3GD20_INT2 00260 * @retval None 00261 */ 00262 void BSP_GYRO_DisableIT(uint8_t IntPin) 00263 { 00264 if(GyroscopeDrv->DisableIT != NULL) 00265 { 00266 GyroscopeDrv->DisableIT(IntPin); 00267 } 00268 } 00269 00270 /** 00271 * @brief Get XYZ angular acceleration from the Gyroscope. 00272 * @param pfData: pointer on floating array 00273 * @retval None 00274 */ 00275 void BSP_GYRO_GetXYZ(float* pfData) 00276 { 00277 if(GyroscopeDrv->GetXYZ!= NULL) 00278 { 00279 GyroscopeDrv->GetXYZ(pfData); 00280 } 00281 } 00282 00283 /** 00284 * @} 00285 */ 00286 00287 /** 00288 * @} 00289 */ 00290 00291 /** 00292 * @} 00293 */ 00294 00295 /** 00296 * @} 00297 */ 00298 00299 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
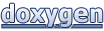