STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery_idd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_idd.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of firmware functions to manage the 00008 * Idd measurement driver for STM32L476G-Discovery board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32l476g_discovery_idd.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32L476G_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L476G_DISCOVERY_IDD STM32L476G-DISCOVERY IDD 00051 * @brief This file includes the Idd driver for STM32L476G-DISCOVERY board. 00052 * It allows user to measure MCU Idd current on board, especially in 00053 * different low power modes. 00054 * @{ 00055 */ 00056 00057 /** @defgroup STM32L476G_DISCOVERY_IDD_Private_Defines Private Defines 00058 * @{ 00059 */ 00060 00061 /** 00062 * @} 00063 */ 00064 00065 00066 /** @defgroup STM32L476G_DISCOVERY_IDD_Private_Variables Private Variables 00067 * @{ 00068 */ 00069 static IDD_DrvTypeDef *IddDrv; 00070 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup STM32L476G_DISCOVERY_IDD_Private_Functions Private Functions 00076 * @{ 00077 */ 00078 00079 /** 00080 * @} 00081 */ 00082 00083 /** @defgroup STM32L476G_DISCOVERY_IDD_Exported_Functions Exported Functions 00084 * @{ 00085 */ 00086 00087 /** 00088 * @brief Configures IDD measurement component. 00089 * @retval IDD_OK if no problem during initialization 00090 */ 00091 uint8_t BSP_IDD_Init(void) 00092 { 00093 IDD_ConfigTypeDef iddconfig = {0}; 00094 uint8_t mfxstm32l152_id = 0; 00095 uint8_t ret = 0; 00096 00097 /* wake up mfx component in case it went to standby mode */ 00098 mfxstm32l152_idd_drv.WakeUp(IDD_I2C_ADDRESS); 00099 HAL_Delay(5); 00100 00101 /* Read ID and verify if the MFX is ready */ 00102 mfxstm32l152_id = mfxstm32l152_idd_drv.ReadID(IDD_I2C_ADDRESS); 00103 00104 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00105 { 00106 /* Initialize the Idd driver structure */ 00107 IddDrv = &mfxstm32l152_idd_drv; 00108 00109 /* Initialize the Idd driver */ 00110 if(IddDrv->Init != NULL) 00111 { 00112 IddDrv->Init(IDD_I2C_ADDRESS); 00113 } 00114 00115 /* Configure Idd component with default values */ 00116 iddconfig.AmpliGain = DISCOVERY_IDD_AMPLI_GAIN; 00117 iddconfig.VddMin = DISCOVERY_IDD_VDD_MIN; 00118 iddconfig.Shunt0Value = DISCOVERY_IDD_SHUNT0_VALUE; 00119 iddconfig.Shunt1Value = DISCOVERY_IDD_SHUNT1_VALUE; 00120 iddconfig.Shunt2Value = DISCOVERY_IDD_SHUNT2_VALUE; 00121 iddconfig.Shunt3Value = 0; 00122 iddconfig.Shunt4Value = DISCOVERY_IDD_SHUNT4_VALUE; 00123 iddconfig.Sh0StabDelay = DISCOVERY_IDD_SHUNT0_STABDELAY; 00124 iddconfig.Sh1StabDelay = DISCOVERY_IDD_SHUNT1_STABDELAY; 00125 iddconfig.Sh2StabDelay = DISCOVERY_IDD_SHUNT2_STABDELAY; 00126 iddconfig.Sh3StabDelay = 0; 00127 iddconfig.Sh4StabDelay = DISCOVERY_IDD_SHUNT4_STABDELAY; 00128 iddconfig.ShuntNbOnBoard = MFXSTM32L152_IDD_SHUNT_NB_4; 00129 iddconfig.ShuntNbUsed = MFXSTM32L152_IDD_SHUNT_NB_4; 00130 iddconfig.VrefMeasurement = MFXSTM32L152_IDD_VREF_AUTO_MEASUREMENT_ENABLE; 00131 iddconfig.Calibration = MFXSTM32L152_IDD_AUTO_CALIBRATION_ENABLE; 00132 iddconfig.PreDelayUnit = MFXSTM32L152_IDD_PREDELAY_20_MS; 00133 iddconfig.PreDelayValue = 0x7F; 00134 iddconfig.MeasureNb = 100; 00135 iddconfig.DeltaDelayUnit= MFXSTM32L152_IDD_DELTADELAY_0_5_MS; 00136 iddconfig.DeltaDelayValue = 10; 00137 BSP_IDD_Config(iddconfig); 00138 00139 ret = IDD_OK; 00140 } 00141 else 00142 { 00143 ret = IDD_ERROR; 00144 } 00145 00146 return ret; 00147 } 00148 00149 /** 00150 * @brief Unconfigures IDD measurement component. 00151 * @retval IDD_OK if no problem during deinitialization 00152 */ 00153 void BSP_IDD_DeInit(void) 00154 { 00155 if(IddDrv->DeInit!= NULL) 00156 { 00157 IddDrv->DeInit(IDD_I2C_ADDRESS); 00158 } 00159 } 00160 00161 /** 00162 * @brief Reset Idd measurement component. 00163 * @retval None 00164 */ 00165 void BSP_IDD_Reset(void) 00166 { 00167 if(IddDrv->Reset != NULL) 00168 { 00169 IddDrv->Reset(IDD_I2C_ADDRESS); 00170 } 00171 } 00172 00173 /** 00174 * @brief Turn Idd measurement component in low power (standby/sleep) mode 00175 * @retval None 00176 */ 00177 void BSP_IDD_LowPower(void) 00178 { 00179 if(IddDrv->LowPower != NULL) 00180 { 00181 IddDrv->LowPower(IDD_I2C_ADDRESS); 00182 } 00183 } 00184 00185 /** 00186 * @brief Start Measurement campaign 00187 * @retval None 00188 */ 00189 void BSP_IDD_StartMeasure(void) 00190 { 00191 if(IddDrv->Start != NULL) 00192 { 00193 IddDrv->Start(IDD_I2C_ADDRESS); 00194 } 00195 } 00196 00197 /** 00198 * @brief Configure Idd component 00199 * @param IddConfig: structure of idd parameters 00200 * @retval None 00201 */ 00202 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig) 00203 { 00204 if(IddDrv->Config != NULL) 00205 { 00206 IddDrv->Config(IDD_I2C_ADDRESS, IddConfig); 00207 } 00208 } 00209 00210 /** 00211 * @brief Get Idd current value. 00212 * @param IddValue: Pointer on u32 to store Idd. Value unit is 10 nA. 00213 * @retval None 00214 */ 00215 void BSP_IDD_GetValue(uint32_t *IddValue) 00216 { 00217 if(IddDrv->GetValue != NULL) 00218 { 00219 IddDrv->GetValue(IDD_I2C_ADDRESS, IddValue); 00220 } 00221 } 00222 00223 /** 00224 * @brief Enable Idd interrupt that warn end of measurement 00225 * @retval None 00226 */ 00227 void BSP_IDD_EnableIT(void) 00228 { 00229 if(IddDrv->EnableIT != NULL) 00230 { 00231 IddDrv->EnableIT(IDD_I2C_ADDRESS); 00232 } 00233 } 00234 00235 /** 00236 * @brief Clear Idd interrupt that warn end of measurement 00237 * @retval None 00238 */ 00239 void BSP_IDD_ClearIT(void) 00240 { 00241 if(IddDrv->ClearIT != NULL) 00242 { 00243 IddDrv->ClearIT(IDD_I2C_ADDRESS); 00244 } 00245 } 00246 00247 /** 00248 * @brief Get Idd interrupt status 00249 * @retval status 00250 */ 00251 uint8_t BSP_IDD_GetITStatus(void) 00252 { 00253 if(IddDrv->GetITStatus != NULL) 00254 { 00255 return (IddDrv->GetITStatus(IDD_I2C_ADDRESS)); 00256 } 00257 else 00258 { 00259 return IDD_ERROR; 00260 } 00261 } 00262 00263 /** 00264 * @brief Disable Idd interrupt that warn end of measurement 00265 * @retval None 00266 */ 00267 void BSP_IDD_DisableIT(void) 00268 { 00269 if(IddDrv->DisableIT != NULL) 00270 { 00271 IddDrv->DisableIT(IDD_I2C_ADDRESS); 00272 } 00273 } 00274 00275 /** 00276 * @brief Get Error Code . 00277 * @retval Error code or error status 00278 */ 00279 uint8_t BSP_IDD_ErrorGetCode(void) 00280 { 00281 if(IddDrv->ErrorGetSrc != NULL) 00282 { 00283 if((IddDrv->ErrorGetSrc(IDD_I2C_ADDRESS) & MFXSTM32L152_IDD_ERROR_SRC) != RESET) 00284 { 00285 if(IddDrv->ErrorGetCode != NULL) 00286 { 00287 return IddDrv->ErrorGetCode(IDD_I2C_ADDRESS); 00288 } 00289 else 00290 { 00291 return IDD_ERROR; 00292 } 00293 } 00294 else 00295 { 00296 return IDD_ERROR; 00297 } 00298 } 00299 else 00300 { 00301 return IDD_ERROR; 00302 } 00303 } 00304 00305 00306 /** 00307 * @brief Enable error interrupt that warn end of measurement 00308 * @retval None 00309 */ 00310 void BSP_IDD_ErrorEnableIT(void) 00311 { 00312 if(IddDrv->ErrorEnableIT != NULL) 00313 { 00314 IddDrv->ErrorEnableIT(IDD_I2C_ADDRESS); 00315 } 00316 } 00317 00318 /** 00319 * @brief Clear Error interrupt that warn end of measurement 00320 * @retval None 00321 */ 00322 void BSP_IDD_ErrorClearIT(void) 00323 { 00324 if(IddDrv->ErrorClearIT != NULL) 00325 { 00326 IddDrv->ErrorClearIT(IDD_I2C_ADDRESS); 00327 } 00328 } 00329 00330 /** 00331 * @brief Get Error interrupt status 00332 * @retval Status 00333 */ 00334 uint8_t BSP_IDD_ErrorGetITStatus(void) 00335 { 00336 if(IddDrv->ErrorGetITStatus != NULL) 00337 { 00338 return (IddDrv->ErrorGetITStatus(IDD_I2C_ADDRESS)); 00339 } 00340 else 00341 { 00342 return 0; 00343 } 00344 } 00345 00346 /** 00347 * @brief Disable Error interrupt 00348 * @retval None 00349 */ 00350 void BSP_IDD_ErrorDisableIT(void) 00351 { 00352 if(IddDrv->ErrorDisableIT != NULL) 00353 { 00354 IddDrv->ErrorDisableIT(IDD_I2C_ADDRESS); 00355 } 00356 } 00357 00358 /** 00359 * @brief Wake up Idd measurement component. 00360 * @retval None 00361 */ 00362 void BSP_IDD_WakeUp(void) 00363 { 00364 if(IddDrv->WakeUp != NULL) 00365 { 00366 IddDrv->WakeUp(IDD_I2C_ADDRESS); 00367 } 00368 } 00369 00370 /** 00371 * @} 00372 */ 00373 00374 /** 00375 * @} 00376 */ 00377 00378 /** 00379 * @} 00380 */ 00381 00382 /** 00383 * @} 00384 */ 00385 00386 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00387
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
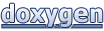