STM32303C_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_AUDIO_OUT_Init (uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) |
Configure the audio peripherals. | |
uint8_t | BSP_AUDIO_OUT_Play (uint16_t *pBuffer, uint32_t Size) |
Starts playing audio stream from a data buffer for a determined size. | |
uint8_t | BSP_AUDIO_OUT_ChangeBuffer (uint16_t *pData, uint16_t Size) |
Sends n-Bytes on the I2S interface. | |
uint8_t | BSP_AUDIO_OUT_Pause (void) |
This function Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Resume (void) |
This function Resumes the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Stop (uint32_t Option) |
Stops audio playing and Power down the Audio Codec. | |
uint8_t | BSP_AUDIO_OUT_SetVolume (uint8_t Volume) |
Controls the current audio volume level. | |
uint8_t | BSP_AUDIO_OUT_SetMute (uint32_t Cmd) |
Enables or disables the MUTE mode by software. | |
uint8_t | BSP_AUDIO_OUT_SetOutputMode (uint8_t Output) |
Switch dynamically (while audio file is played) the output target (speaker or headphone). | |
uint8_t | BSP_AUDIO_OUT_SetFrequency (uint32_t AudioFreq) |
Update the audio frequency. | |
void | HAL_I2S_TxCpltCallback (I2S_HandleTypeDef *hi2s) |
Tx Transfer completed callbacks. | |
void | HAL_I2S_TxHalfCpltCallback (I2S_HandleTypeDef *hi2s) |
Tx Transfer Half completed callbacks. | |
void | HAL_I2S_ErrorCallback (I2S_HandleTypeDef *hi2s) |
I2S error callbacks. | |
__weak void | BSP_AUDIO_OUT_TransferComplete_CallBack (void) |
Manages the DMA full Transfer complete event. | |
__weak void | BSP_AUDIO_OUT_HalfTransfer_CallBack (void) |
Manages the DMA Half Transfer complete event. | |
__weak void | BSP_AUDIO_OUT_Error_CallBack (void) |
Audio OUT Error callback function. |
Function Documentation
uint8_t BSP_AUDIO_OUT_ChangeBuffer | ( | uint16_t * | pData, |
uint16_t | Size | ||
) |
Sends n-Bytes on the I2S interface.
- Parameters:
-
pData pointer on data address Size number of data to be written
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 247 of file stm32303c_eval_audio.c.
References hAudioOutI2s.
void BSP_AUDIO_OUT_Error_CallBack | ( | void | ) |
Audio OUT Error callback function.
- Return values:
-
None
Definition at line 462 of file stm32303c_eval_audio.c.
Referenced by HAL_I2S_ErrorCallback().
void BSP_AUDIO_OUT_HalfTransfer_CallBack | ( | void | ) |
Manages the DMA Half Transfer complete event.
- Return values:
-
None
Definition at line 454 of file stm32303c_eval_audio.c.
Referenced by HAL_I2S_TxHalfCpltCallback().
uint8_t BSP_AUDIO_OUT_Init | ( | uint16_t | OutputDevice, |
uint8_t | Volume, | ||
uint32_t | AudioFreq | ||
) |
Configure the audio peripherals.
- Parameters:
-
OutputDevice OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO . Volume Initial volume level (from 0 (Mute) to 100 (Max)) AudioFreq Audio frequency used to play the audio stream.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 182 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, I2Sx_Init(), and pAudioDrv.
uint8_t BSP_AUDIO_OUT_Pause | ( | void | ) |
This function Pauses the audio file stream.
In case of using DMA, the DMA Pause feature is used.
- Note:
- When calling BSP_AUDIO_OUT_Pause() function for pause, only BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() function for resume could lead to unexpected behavior).
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 259 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, hAudioOutI2s, and pAudioDrv.
uint8_t BSP_AUDIO_OUT_Play | ( | uint16_t * | pBuffer, |
uint32_t | Size | ||
) |
Starts playing audio stream from a data buffer for a determined size.
- Parameters:
-
pBuffer Pointer to the buffer Size Number of audio data BYTES.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 227 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, DMA_MAX, hAudioOutI2s, and pAudioDrv.
uint8_t BSP_AUDIO_OUT_Resume | ( | void | ) |
This function Resumes the audio file stream.
- Note:
- When calling BSP_AUDIO_OUT_Pause() function for pause, only BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() function for resume could lead to unexpected behavior).
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 280 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, hAudioOutI2s, and pAudioDrv.
uint8_t BSP_AUDIO_OUT_SetFrequency | ( | uint32_t | AudioFreq | ) |
Update the audio frequency.
- Parameters:
-
AudioFreq Audio frequency used to play the audio stream.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 392 of file stm32303c_eval_audio.c.
References I2Sx_Init().
uint8_t BSP_AUDIO_OUT_SetMute | ( | uint32_t | Cmd | ) |
Enables or disables the MUTE mode by software.
- Parameters:
-
Cmd could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to unmute the codec and restore previous volume level.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 351 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, and pAudioDrv.
uint8_t BSP_AUDIO_OUT_SetOutputMode | ( | uint8_t | Output | ) |
Switch dynamically (while audio file is played) the output target (speaker or headphone).
- Note:
- This function modifies a global variable of the audio codec driver: OutputDev.
- Parameters:
-
Output specifies the audio output target: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 373 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, and pAudioDrv.
uint8_t BSP_AUDIO_OUT_SetVolume | ( | uint8_t | Volume | ) |
Controls the current audio volume level.
- Parameters:
-
Volume Volume level to be set in percentage from 0% to 100% (0 for Mute and 100 for Max volume level).
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 331 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, and pAudioDrv.
uint8_t BSP_AUDIO_OUT_Stop | ( | uint32_t | Option | ) |
Stops audio playing and Power down the Audio Codec.
- Parameters:
-
Option could be one of the following parameters - CODEC_PDWN_SW: for software power off (by writing registers). Then no need to reconfigure the Codec after power on.
- CODEC_PDWN_HW: completely shut down the codec (physically). Then need to reconfigure the Codec after power on.
- Return values:
-
AUDIO_OK if correct communication, else wrong communication
Definition at line 303 of file stm32303c_eval_audio.c.
References AUDIO_ERROR, AUDIO_I2C_ADDRESS, AUDIO_OK, hAudioOutI2s, and pAudioDrv.
void BSP_AUDIO_OUT_TransferComplete_CallBack | ( | void | ) |
Manages the DMA full Transfer complete event.
- Return values:
-
None
Definition at line 446 of file stm32303c_eval_audio.c.
Referenced by HAL_I2S_TxCpltCallback().
void HAL_I2S_ErrorCallback | ( | I2S_HandleTypeDef * | hi2s | ) |
I2S error callbacks.
- Parameters:
-
hi2s I2S handle
- Return values:
-
None
Definition at line 432 of file stm32303c_eval_audio.c.
References BSP_AUDIO_OUT_Error_CallBack(), and I2Sx.
void HAL_I2S_TxCpltCallback | ( | I2S_HandleTypeDef * | hi2s | ) |
Tx Transfer completed callbacks.
- Parameters:
-
hi2s I2S handle
- Return values:
-
None
Definition at line 403 of file stm32303c_eval_audio.c.
References BSP_AUDIO_OUT_TransferComplete_CallBack(), and I2Sx.
void HAL_I2S_TxHalfCpltCallback | ( | I2S_HandleTypeDef * | hi2s | ) |
Tx Transfer Half completed callbacks.
- Parameters:
-
hi2s I2S handle
- Return values:
-
None
Definition at line 417 of file stm32303c_eval_audio.c.
References BSP_AUDIO_OUT_HalfTransfer_CallBack(), and I2Sx.
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
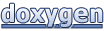