STM32303C_EVAL BSP User Manual
|
Functions | |
void | LCD_IO_Init (void) |
Configures the LCD_SPI interface. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
register address. | |
uint16_t | LCD_IO_ReadData (uint16_t Reg) |
Read register value. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_WriteByte (uint8_t Data) |
Write a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
Read a byte from the SD. | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Send 5 bytes command to the SD card and get response. | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Wait response from the SD card. | |
void | SD_IO_WriteDummy (void) |
Send dummy byte with CS High. | |
void | EEPROM_SPI_IO_Init (void) |
Initializes the EEPROM SPI and put it into StandBy State (Ready for data transfer). | |
void | EEPROM_SPI_IO_WriteByte (uint8_t Data) |
Write a byte on the EEPROM. | |
uint8_t | EEPROM_SPI_IO_ReadByte (void) |
Read a byte from the EEPROM. | |
HAL_StatusTypeDef | EEPROM_SPI_IO_WriteData (uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to SPI EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_SPI_IO_ReadData (uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from SPI EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_SPI_IO_WaitEepromStandbyState (void) |
Wait response from the SPI EEPROM. | |
void | EEPROM_I2C_IO_Init (void) |
Initializes peripherals used by the I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_I2C_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_I2C_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_I2C_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
void | TSENSOR_IO_Init (void) |
Initializes peripherals used by the I2C Temperature Sensor driver. | |
void | TSENSOR_IO_Write (uint16_t DevAddress, uint8_t *pBuffer, uint8_t WriteAddr, uint16_t Length) |
Writes one byte to the TSENSOR. | |
void | TSENSOR_IO_Read (uint16_t DevAddress, uint8_t *pBuffer, uint8_t ReadAddr, uint16_t Length) |
Reads one byte from the TSENSOR. | |
uint16_t | TSENSOR_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if Temperature Sensor is ready for communication. | |
void | AUDIO_IO_Init (void) |
Initializes peripherals used by the Audio Codec driver. | |
void | AUDIO_IO_DeInit (void) |
DeInitializes Audio low level. | |
void | AUDIO_IO_Write (uint16_t DevAddress, uint8_t Reg, uint8_t Value) |
Writes a single data on the Audio Codec. | |
uint8_t | AUDIO_IO_Read (uint16_t DevAddress, uint8_t Reg) |
Reads a single data from the Audio Codec. | |
void | AUDIO_IO_Delay (uint32_t Delay) |
Wait for loop in ms. |
Function Documentation
void AUDIO_IO_DeInit | ( | void | ) |
DeInitializes Audio low level.
- Note:
- This function is intentionally kept empty, user should define it.
Definition at line 1452 of file stm32303c_eval.c.
void AUDIO_IO_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
- Parameters:
-
Delay in ms.
- Return values:
-
None
Definition at line 1489 of file stm32303c_eval.c.
void AUDIO_IO_Init | ( | void | ) |
Initializes peripherals used by the Audio Codec driver.
- Return values:
-
None
Definition at line 1443 of file stm32303c_eval.c.
References I2Cx_Init().
uint8_t AUDIO_IO_Read | ( | uint16_t | DevAddress, |
uint8_t | Reg | ||
) |
Reads a single data from the Audio Codec.
- Parameters:
-
DevAddress Target device address Reg Target Register address
- Return values:
-
Data to be read
Definition at line 1475 of file stm32303c_eval.c.
References I2Cx_ReadData().
void AUDIO_IO_Write | ( | uint16_t | DevAddress, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
Writes a single data on the Audio Codec.
- Parameters:
-
DevAddress Target device address Reg Target Register address Value Data to be written
- Return values:
-
None
Definition at line 1464 of file stm32303c_eval.c.
References I2Cx_WriteData().
void EEPROM_I2C_IO_Init | ( | void | ) |
Initializes peripherals used by the I2C EEPROM driver.
- Return values:
-
None
Definition at line 1347 of file stm32303c_eval.c.
References I2Cx_Init().
Referenced by EEPROM_I2C_Init().
HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress Target device address Trials Number of trials
- Return values:
-
HAL status
Definition at line 1385 of file stm32303c_eval.c.
References I2Cx_IsDeviceReady().
Referenced by EEPROM_I2C_Init(), and EEPROM_I2C_WaitEepromStandbyState().
HAL_StatusTypeDef EEPROM_I2C_IO_ReadData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from I2C EEPROM driver.
- Parameters:
-
DevAddress Target device address MemAddress Internal memory address pBuffer Pointer to data buffer BufferSize Amount of data to be read
- Return values:
-
HAL status
Definition at line 1373 of file stm32303c_eval.c.
References I2Cx_ReadBuffer().
Referenced by EEPROM_I2C_ReadBuffer().
HAL_StatusTypeDef EEPROM_I2C_IO_WriteData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Write data to I2C EEPROM driver.
- Parameters:
-
DevAddress Target device address MemAddress Internal memory address pBuffer Pointer to data buffer BufferSize Amount of data to be sent
- Return values:
-
HAL status
Definition at line 1360 of file stm32303c_eval.c.
References I2Cx_WriteBuffer().
Referenced by EEPROM_I2C_WritePage().
void EEPROM_SPI_IO_Init | ( | void | ) |
Initializes the EEPROM SPI and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 1152 of file stm32303c_eval.c.
References EEPROM_CS_GPIO_CLK_ENABLE, EEPROM_CS_GPIO_PORT, EEPROM_CS_HIGH, EEPROM_CS_PIN, and SPIx_Init().
Referenced by EEPROM_SPI_Init().
uint8_t EEPROM_SPI_IO_ReadByte | ( | void | ) |
Read a byte from the EEPROM.
- Return values:
-
uint8_t (The received byte).
Definition at line 1189 of file stm32303c_eval.c.
References SPIx_Read().
HAL_StatusTypeDef EEPROM_SPI_IO_ReadData | ( | uint16_t | MemAddress, |
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from SPI EEPROM driver.
- Parameters:
-
MemAddress Internal memory address pBuffer Pointer to data buffer BufferSize Amount of data to be read
- Return values:
-
HAL_StatusTypeDef HAL Status
< Select the EEPROM: Chip Select low
< Send "Write to Memory " instruction
< Send MemAddress high nibble address byte to write to
< Send WriteAddr medium nibble address byte to write to
< Send WriteAddr low nibble address byte to write to
< while there is data to be read
< Read a byte from the EEPROM
< Point to the next location where the byte read will be saved
Definition at line 1269 of file stm32303c_eval.c.
References EEPROM_CMD_READ, EEPROM_CS_HIGH, EEPROM_CS_LOW, SPIx_Read(), and SPIx_Write().
Referenced by EEPROM_SPI_ReadBuffer().
HAL_StatusTypeDef EEPROM_SPI_IO_WaitEepromStandbyState | ( | void | ) |
Wait response from the SPI EEPROM.
- Return values:
-
HAL_StatusTypeDef HAL Status
< Select the EEPROM: Chip Select low
< Send "Read Status Register" instruction
< Loop as long as the memory is busy with a write cycle
< Send a dummy byte to generate the clock needed by the EEPROM and put the value of the status register in EEPROM Status variable
< Deselect the EEPROM: Chip Select high
Definition at line 1304 of file stm32303c_eval.c.
References EEPROM_CMD_RDSR, EEPROM_CS_HIGH, EEPROM_CS_LOW, EEPROM_WIP_FLAG, SPIx_Read(), and SPIx_Write().
Referenced by EEPROM_SPI_IO_WriteData(), and EEPROM_SPI_WaitEepromStandbyState().
void EEPROM_SPI_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the EEPROM.
- Parameters:
-
Data byte to send.
- Return values:
-
None
Definition at line 1179 of file stm32303c_eval.c.
References SPIx_Write().
HAL_StatusTypeDef EEPROM_SPI_IO_WriteData | ( | uint16_t | MemAddress, |
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Write data to SPI EEPROM driver.
- Parameters:
-
MemAddress Internal memory address pBuffer Pointer to data buffer BufferSize Amount of data to be read
- Return values:
-
HAL_StatusTypeDef HAL Status
< Enable the write access to the EEPROM
< Select the EEPROM: Chip Select low
< Send "Write Enable" instruction
< Deselect the EEPROM: Chip Select high
< Select the EEPROM: Chip Select low
< Send "Write to Memory " instruction
< Send MemAddress high nibble address byte to write to
< Send MemAddress medium nibble address byte to write to
< Send MemAddress low nibble address byte to write to
< while there is data to be written on the EEPROM
< Send the current byte
< Point on the next byte to be written
< Wait the end of EEPROM writing
< Disable the write access to the EEROM
< Send "Write Disable" instruction
< Deselect the EEPROM: Chip Select high
Definition at line 1207 of file stm32303c_eval.c.
References EEPROM_CMD_WRDI, EEPROM_CMD_WREN, EEPROM_CMD_WRITE, EEPROM_CS_HIGH, EEPROM_CS_LOW, EEPROM_SPI_IO_WaitEepromStandbyState(), and SPIx_Write().
Referenced by EEPROM_SPI_WritePage().
void LCD_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
- Parameters:
-
Delay in ms.
- Return values:
-
None
Definition at line 987 of file stm32303c_eval.c.
void LCD_IO_Init | ( | void | ) |
Configures the LCD_SPI interface.
- Return values:
-
None
Definition at line 871 of file stm32303c_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_NCS_GPIO_CLK_ENABLE, LCD_NCS_GPIO_PORT, LCD_NCS_PIN, and SPIx_Init().
uint16_t LCD_IO_ReadData | ( | uint16_t | Reg | ) |
Read register value.
- Parameters:
-
Reg
- Return values:
-
None
Definition at line 947 of file stm32303c_eval.c.
References heval_Spi, LCD_CS_HIGH, LCD_CS_LOW, LCD_IO_WriteReg(), LCD_READ_REG, SPIx_Read(), SPIx_Write(), and START_BYTE.
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Write register value.
- Parameters:
-
pData Pointer on the register value Size Size of byte to transmit to the register
- Return values:
-
None
Definition at line 898 of file stm32303c_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_WRITE_REG, SPIx_Write(), and START_BYTE.
void LCD_IO_WriteReg | ( | uint8_t | Reg | ) |
register address.
- Parameters:
-
Reg
- Return values:
-
None
Definition at line 926 of file stm32303c_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, SET_INDEX, SPIx_Write(), and START_BYTE.
Referenced by LCD_IO_ReadData().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 999 of file stm32303c_eval.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_EXTI_IRQn, SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
uint8_t SD_IO_ReadByte | ( | void | ) |
Read a byte from the SD.
- Return values:
-
The received byte.
Definition at line 1056 of file stm32303c_eval.c.
References SPIx_Read().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), and SD_IO_WaitResponse().
HAL_StatusTypeDef SD_IO_WaitResponse | ( | uint8_t | Response | ) |
Wait response from the SD card.
- Parameters:
-
Response Expected response from the SD card
- Return values:
-
HAL_StatusTypeDef HAL Status
Definition at line 1110 of file stm32303c_eval.c.
References SD_IO_ReadByte().
Referenced by BSP_SD_Erase(), BSP_SD_ReadBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_IO_WriteCmd().
void SD_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the SD.
- Parameters:
-
Data byte to send.
- Return values:
-
None
Definition at line 1046 of file stm32303c_eval.c.
References SPIx_Write().
Referenced by BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_IO_Init(), SD_IO_WriteCmd(), and SD_IO_WriteDummy().
HAL_StatusTypeDef SD_IO_WriteCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) |
Send 5 bytes command to the SD card and get response.
- Parameters:
-
Cmd The user expected command to send to SD card. Arg The command argument. Crc The CRC. Response Expected response from the SD card
- Return values:
-
HAL_StatusTypeDef HAL Status
Definition at line 1075 of file stm32303c_eval.c.
References SD_CS_LOW, SD_IO_WaitResponse(), SD_IO_WriteByte(), and SD_NO_RESPONSE_EXPECTED.
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
void SD_IO_WriteDummy | ( | void | ) |
Send dummy byte with CS High.
- Return values:
-
None
Definition at line 1136 of file stm32303c_eval.c.
References SD_CS_HIGH, SD_DUMMY_BYTE, and SD_IO_WriteByte().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
void TSENSOR_IO_Init | ( | void | ) |
Initializes peripherals used by the I2C Temperature Sensor driver.
- Return values:
-
None
Definition at line 1395 of file stm32303c_eval.c.
References I2Cx_Init().
uint16_t TSENSOR_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if Temperature Sensor is ready for communication.
- Parameters:
-
DevAddress Target device address Trials Number of trials
- Return values:
-
HAL status
Definition at line 1432 of file stm32303c_eval.c.
References I2Cx_IsDeviceReady().
void TSENSOR_IO_Read | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | ReadAddr, | ||
uint16_t | Length | ||
) |
Reads one byte from the TSENSOR.
- Parameters:
-
DevAddress Target device address pBuffer pointer to the buffer that receives the data read from the TSENSOR. ReadAddr TSENSOR's internal address to read from. Length Number of data to read
- Return values:
-
None
Definition at line 1421 of file stm32303c_eval.c.
References I2Cx_ReadBuffer().
void TSENSOR_IO_Write | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | WriteAddr, | ||
uint16_t | Length | ||
) |
Writes one byte to the TSENSOR.
- Parameters:
-
DevAddress Target device address pBuffer Pointer to data buffer WriteAddr TSENSOR's internal address to write to. Length Number of data to write
- Return values:
-
None
Definition at line 1408 of file stm32303c_eval.c.
References I2Cx_WriteBuffer().
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
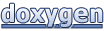