STM32303C_EVAL BSP User Manual
|
This file contains the common defines and functions prototypes for the stm32303c_eval_sd.c driver. More...
#include "stm32303c_eval.h"
Go to the source code of this file.
Data Structures | |
struct | SD_CSD |
Card Specific Data: CSD Register. More... | |
struct | SD_CID |
Card Identification Data: CID Register. More... | |
struct | SD_CardInfo |
SD Card information. More... | |
Defines | |
#define | MSD_OK 0x00 |
SD status structure definition. | |
#define | MSD_ERROR 0x01 |
#define | SD_START_DATA_SINGLE_BLOCK_READ 0xFE /* Data token start byte, Start Single Block Read */ |
Start Data tokens: Tokens (necessary because at nop/idle (and CS active) only 0xff is on the data/command line) | |
#define | SD_START_DATA_MULTIPLE_BLOCK_READ 0xFE /* Data token start byte, Start Multiple Block Read */ |
#define | SD_START_DATA_SINGLE_BLOCK_WRITE 0xFE /* Data token start byte, Start Single Block Write */ |
#define | SD_START_DATA_MULTIPLE_BLOCK_WRITE 0xFD /* Data token start byte, Start Multiple Block Write */ |
#define | SD_STOP_DATA_MULTIPLE_BLOCK_WRITE 0xFD /* Data toke stop byte, Stop Multiple Block Write */ |
#define | SD_PRESENT ((uint8_t)0x01) |
SD detection on its memory slot. | |
#define | SD_NOT_PRESENT ((uint8_t)0x00) |
#define | SD_CMD_GO_IDLE_STATE 0 /* CMD0 = 0x40 */ |
Commands: CMDxx = CMD-number | 0x40. | |
#define | SD_CMD_SEND_OP_COND 1 /* CMD1 = 0x41 */ |
#define | SD_CMD_SEND_CSD 9 /* CMD9 = 0x49 */ |
#define | SD_CMD_SEND_CID 10 /* CMD10 = 0x4A */ |
#define | SD_CMD_STOP_TRANSMISSION 12 /* CMD12 = 0x4C */ |
#define | SD_CMD_SEND_STATUS 13 /* CMD13 = 0x4D */ |
#define | SD_CMD_SET_BLOCKLEN 16 /* CMD16 = 0x50 */ |
#define | SD_CMD_READ_SINGLE_BLOCK 17 /* CMD17 = 0x51 */ |
#define | SD_CMD_READ_MULT_BLOCK 18 /* CMD18 = 0x52 */ |
#define | SD_CMD_SET_BLOCK_COUNT 23 /* CMD23 = 0x57 */ |
#define | SD_CMD_WRITE_SINGLE_BLOCK 24 /* CMD24 = 0x58 */ |
#define | SD_CMD_WRITE_MULT_BLOCK 25 /* CMD25 = 0x59 */ |
#define | SD_CMD_PROG_CSD 27 /* CMD27 = 0x5B */ |
#define | SD_CMD_SET_WRITE_PROT 28 /* CMD28 = 0x5C */ |
#define | SD_CMD_CLR_WRITE_PROT 29 /* CMD29 = 0x5D */ |
#define | SD_CMD_SEND_WRITE_PROT 30 /* CMD30 = 0x5E */ |
#define | SD_CMD_SD_ERASE_GRP_START 32 /* CMD32 = 0x60 */ |
#define | SD_CMD_SD_ERASE_GRP_END 33 /* CMD33 = 0x61 */ |
#define | SD_CMD_UNTAG_SECTOR 34 /* CMD34 = 0x62 */ |
#define | SD_CMD_ERASE_GRP_START 35 /* CMD35 = 0x63 */ |
#define | SD_CMD_ERASE_GRP_END 36 /* CMD36 = 0x64 */ |
#define | SD_CMD_UNTAG_ERASE_GROUP 37 /* CMD37 = 0x65 */ |
#define | SD_CMD_ERASE 38 /* CMD38 = 0x66 */ |
Enumerations | |
enum | SD_Info { SD_RESPONSE_NO_ERROR = (0x00), SD_IN_IDLE_STATE = (0x01), SD_ERASE_RESET = (0x02), SD_ILLEGAL_COMMAND = (0x04), SD_COM_CRC_ERROR = (0x08), SD_ERASE_SEQUENCE_ERROR = (0x10), SD_ADDRESS_ERROR = (0x20), SD_PARAMETER_ERROR = (0x40), SD_RESPONSE_FAILURE = (0xFF), SD_DATA_OK = (0x05), SD_DATA_CRC_ERROR = (0x0B), SD_DATA_WRITE_ERROR = (0x0D), SD_DATA_OTHER_ERROR = (0xFF) } |
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD/SD communication. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *p32Data, uint64_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *p32Data, uint64_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
uint8_t | BSP_SD_GetStatus (void) |
Returns the SD status. | |
uint8_t | BSP_SD_GetCardInfo (SD_CardInfo *pCardInfo) |
Returns information about specific card. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_WriteByte (uint8_t Data) |
Write a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
Read a byte from the SD. | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Send 5 bytes command to the SD card and get response. | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Wait response from the SD card. | |
void | SD_IO_WriteDummy (void) |
Send dummy byte with CS High. |
Detailed Description
This file contains the common defines and functions prototypes for the stm32303c_eval_sd.c driver.
- Attention:
© COPYRIGHT(c) 2016 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm32303c_eval_sd.h.
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
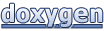