STM32303C_EVAL BSP User Manual
|
stm32303c_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_audio.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the 00006 * stm32303c_eval_audio.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32303C_EVAL_AUDIO_H 00039 #define __STM32303C_EVAL_AUDIO_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 /* Include AUDIO component driver */ 00047 #include "../Components/cs42l52/cs42l52.h" 00048 #include "stm32303c_eval.h" 00049 /** @addtogroup BSP 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM32303C_EVAL 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32303C_EVAL_AUDIO 00058 * @{ 00059 */ 00060 00061 /** @defgroup STM32303C_EVAL_AUDIO_Exported_Types Exported Types 00062 * @{ 00063 */ 00064 typedef enum 00065 { 00066 AUDIO_OK = 0x00, 00067 AUDIO_ERROR = 0x01, 00068 AUDIO_TIMEOUT = 0x02 00069 00070 }AUDIO_StatusTypeDef; 00071 00072 /** 00073 * @} 00074 */ 00075 00076 /** @defgroup STM32303C_EVAL_AUDIO_Exported_Constants Exported Constants 00077 * @{ 00078 */ 00079 00080 /* Audio Codec hardware I2C address */ 00081 #define AUDIO_I2C_ADDRESS 0x94 00082 00083 /*---------------------------------------------------------------------------- 00084 AUDIO OUT CONFIGURATION 00085 ----------------------------------------------------------------------------*/ 00086 00087 /* I2S peripheral configuration defines */ 00088 #define I2Sx SPI3 00089 #define I2Sx_CLK_ENABLE() __HAL_RCC_SPI3_CLK_ENABLE() 00090 #define I2Sx_CLK_DISABLE() __HAL_RCC_SPI3_CLK_DISABLE() 00091 #define I2Sx_FORCE_RESET() __HAL_RCC_SPI3_FORCE_RESET() 00092 #define I2Sx_RELEASE_RESET() __HAL_RCC_SPI3_RELEASE_RESET() 00093 00094 #define I2Sx_WS_PIN GPIO_PIN_4 00095 #define I2Sx_MCK_PIN GPIO_PIN_9 00096 #define I2Sx_SCK_PIN GPIO_PIN_10 00097 #define I2Sx_DIN_PIN GPIO_PIN_12 00098 00099 #define I2Sx_WS_GPIO_PORT GPIOA 00100 #define I2Sx_MCK_GPIO_PORT GPIOA 00101 #define I2Sx_SCK_DIN_GPIO_PORT GPIOC 00102 #define I2Sx_MCK_WS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00103 #define I2Sx_MCK_WS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00104 #define I2Sx_WS_AF GPIO_AF6_SPI3 00105 #define I2Sx_MCK_AF GPIO_AF5_SPI3 00106 #define I2Sx_SCK_DIN_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00107 #define I2Sx_SCK_DIN_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00108 #define I2Sx_SCK_DIN_AF GPIO_AF6_SPI3 00109 00110 /* I2S DMA Stream definitions */ 00111 #define I2Sx_DMAx_CLK_ENABLE() __HAL_RCC_DMA2_CLK_ENABLE() 00112 #define I2Sx_DMAx_CLK_DISABLE() __HAL_RCC_DMA2_CLK_DISABLE() 00113 #define I2Sx_DMAx_CHANNEL DMA2_Channel2 00114 #define I2Sx_DMAx_IRQ DMA2_Channel2_IRQn 00115 #define I2Sx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00116 #define I2Sx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00117 #define DMA_MAX_SZE 0xFFFF 00118 00119 /* Select the interrupt preemption priority and subpriority for the DMA interrupt */ 00120 #define AUDIO_OUT_IRQ_PREPRIO ((uint32_t)0x0E) /* Select the preemption priority level(0 is the highest) */ 00121 #define AUDIO_OUT_IRQ_SUBPRIO 0 /* Select the sub-priority level (0 is the highest) */ 00122 /*----------------------------------------------------------------------------*/ 00123 00124 /*------------------------------------------------------------------------------ 00125 OPTIONAL Configuration defines parameters 00126 ------------------------------------------------------------------------------*/ 00127 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00128 00129 #define DMA_MAX(_X_) (((_X_) <= DMA_MAX_SZE)? (_X_):DMA_MAX_SZE) 00130 /** 00131 * @} 00132 */ 00133 00134 /** @defgroup STM32303C_EVAL_AUDIO_Exported_Variables Exported Variables 00135 * @{ 00136 */ 00137 00138 /** 00139 * @} 00140 */ 00141 00142 /** @defgroup STM32303C_EVAL_AUDIO_Exported_Macros Exported Macros 00143 * @{ 00144 */ 00145 /** 00146 * @} 00147 */ 00148 00149 /* Exported functions --------------------------------------------------------*/ 00150 /** @defgroup STM32303C_EVAL_AUDIO_Exported_Functions Exported Functions 00151 * @{ 00152 */ 00153 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00154 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00155 uint8_t BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00156 uint8_t BSP_AUDIO_OUT_Pause(void); 00157 uint8_t BSP_AUDIO_OUT_Resume(void); 00158 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00159 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00160 uint8_t BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00161 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Command); 00162 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00163 00164 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00165 /* This function is called when the requested data has been completely transferred.*/ 00166 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00167 00168 /* This function is called when half of the requested buffer has been transferred. */ 00169 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00170 00171 /* This function is called when an Interrupt due to transfer error on or peripheral 00172 error occurs. */ 00173 void BSP_AUDIO_OUT_Error_CallBack(void); 00174 /** 00175 * @} 00176 */ 00177 00178 /** 00179 * @} 00180 */ 00181 00182 /** 00183 * @} 00184 */ 00185 00186 /** 00187 * @} 00188 */ 00189 00190 #ifdef __cplusplus 00191 } 00192 #endif 00193 00194 #endif /* __STM32303C_EVAL_AUDIO_H */ 00195 00196 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
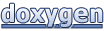