STM32303C_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD/SD communication. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *p32Data, uint64_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *p32Data, uint64_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
uint8_t | BSP_SD_GetStatus (void) |
Returns the SD status. | |
uint8_t | BSP_SD_GetCardInfo (SD_CardInfo *pCardInfo) |
Returns information about specific card. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_WriteByte (uint8_t Data) |
Write a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
Read a byte from the SD. | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Send 5 bytes command to the SD card and get response. | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Wait response from the SD card. | |
void | SD_IO_WriteDummy (void) |
Send dummy byte with CS High. |
Function Documentation
uint8_t BSP_SD_Erase | ( | uint32_t | StartAddr, |
uint32_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
- Parameters:
-
StartAddr Start byte address EndAddr End byte address
- Return values:
-
SD status
Definition at line 664 of file stm32303c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_ERASE, SD_CMD_SD_ERASE_GRP_END, SD_CMD_SD_ERASE_GRP_START, SD_IO_WaitResponse(), SD_RESPONSE_NO_ERROR, and SD_SendCmd().
uint8_t BSP_SD_GetCardInfo | ( | SD_CardInfo * | pCardInfo | ) |
Returns information about specific card.
- Parameters:
-
pCardInfo pointer to a SD_CardInfo structure that contains all SD card information.
- Return values:
-
The SD Response: - MSD_ERROR : Sequence failed
- MSD_OK : Sequence succeed
Definition at line 197 of file stm32303c_eval_sd.c.
References SD_CardInfo::CardBlockSize, SD_CardInfo::CardCapacity, SD_CardInfo::Cid, SD_CardInfo::Csd, SD_CSD::DeviceSize, SD_CSD::DeviceSizeMul, MSD_ERROR, SD_CSD::RdBlockLen, SD_GetCIDRegister(), and SD_GetCSDRegister().
uint8_t BSP_SD_GetStatus | ( | void | ) |
Returns the SD status.
- Return values:
-
The SD status.
Definition at line 632 of file stm32303c_eval_sd.c.
References MSD_OK.
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD/SD communication.
- Return values:
-
The SD Response: - MSD_ERROR : Sequence failed
- MSD_OK : Sequence succeed
Definition at line 152 of file stm32303c_eval_sd.c.
References BSP_SD_IsDetected(), MSD_ERROR, SD_GoIdleState(), SD_IO_Init(), SD_NOT_PRESENT, SD_PRESENT, and SdStatus.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
- Return values:
-
Returns if SD is detected or not
Definition at line 176 of file stm32303c_eval_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_NOT_PRESENT, and SD_PRESENT.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | p32Data, |
uint64_t | ReadAddr, | ||
uint16_t | BlockSize, | ||
uint32_t | NumberOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in polling mode.
- Parameters:
-
p32Data Pointer to the buffer that will contain the data to transmit ReadAddr Address from where data is to be read BlockSize SD card data block size, that should be 512 NumberOfBlocks Number of SD blocks to read
- Return values:
-
SD status
Definition at line 220 of file stm32303c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_READ_SINGLE_BLOCK, SD_CMD_SET_BLOCKLEN, SD_IO_ReadByte(), SD_IO_WaitResponse(), SD_IO_WriteCmd(), SD_IO_WriteDummy(), SD_RESPONSE_NO_ERROR, and SD_START_DATA_SINGLE_BLOCK_READ.
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | p32Data, |
uint64_t | WriteAddr, | ||
uint16_t | BlockSize, | ||
uint32_t | NumberOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in polling mode.
- Parameters:
-
p32Data Pointer to the buffer that will contain the data to transmit WriteAddr Address from where data is to be written BlockSize SD card data block size, that should be 512 NumberOfBlocks Number of SD blocks to write
- Return values:
-
SD status
Definition at line 286 of file stm32303c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_WRITE_SINGLE_BLOCK, SD_DATA_OK, SD_DUMMY_BYTE, SD_GetDataResponse(), SD_IO_ReadByte(), SD_IO_WriteByte(), SD_IO_WriteCmd(), SD_IO_WriteDummy(), SD_RESPONSE_NO_ERROR, and SD_START_DATA_SINGLE_BLOCK_WRITE.
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 999 of file stm32303c_eval.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_EXTI_IRQn, SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
uint8_t SD_IO_ReadByte | ( | void | ) |
Read a byte from the SD.
- Return values:
-
The received byte.
Definition at line 1056 of file stm32303c_eval.c.
References SPIx_Read().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), and SD_IO_WaitResponse().
HAL_StatusTypeDef SD_IO_WaitResponse | ( | uint8_t | Response | ) |
Wait response from the SD card.
- Parameters:
-
Response Expected response from the SD card
- Return values:
-
HAL_StatusTypeDef HAL Status
Definition at line 1110 of file stm32303c_eval.c.
References SD_IO_ReadByte().
Referenced by BSP_SD_Erase(), BSP_SD_ReadBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_IO_WriteCmd().
void SD_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the SD.
- Parameters:
-
Data byte to send.
- Return values:
-
None
Definition at line 1046 of file stm32303c_eval.c.
References SPIx_Write().
Referenced by BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_IO_Init(), SD_IO_WriteCmd(), and SD_IO_WriteDummy().
HAL_StatusTypeDef SD_IO_WriteCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) |
Send 5 bytes command to the SD card and get response.
- Parameters:
-
Cmd The user expected command to send to SD card. Arg The command argument. Crc The CRC. Response Expected response from the SD card
- Return values:
-
HAL_StatusTypeDef HAL Status
Definition at line 1075 of file stm32303c_eval.c.
References SD_CS_LOW, SD_IO_WaitResponse(), SD_IO_WriteByte(), and SD_NO_RESPONSE_EXPECTED.
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
void SD_IO_WriteDummy | ( | void | ) |
Send dummy byte with CS High.
- Return values:
-
None
Definition at line 1136 of file stm32303c_eval.c.
References SD_CS_HIGH, SD_DUMMY_BYTE, and SD_IO_WriteByte().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
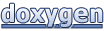