STM32303C_EVAL BSP User Manual
|
stm32303c_eval_audio.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_audio.c 00004 * @author MCD Application Team 00005 * @brief This file provides the Audio driver for the STM32303C_EVAL 00006 * evaluation board(MB1019). 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /*============================================================================== 00038 User NOTES 00039 How To use this driver: 00040 ----------------------- 00041 + This driver supports STM32F30x devices on STM32303C_EVAL Evaluation boards: 00042 + Call the function BSP_AUDIO_OUT_Init( 00043 OutputDevice: physical output mode (OUTPUT_DEVICE_SPEAKER, 00044 OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_AUTO or 00045 OUTPUT_DEVICE_BOTH) 00046 Volume: initial volume to be set (0 is min (mute), 100 is max (100%) 00047 AudioFreq: Audio frequency in Hz (8000, 16000, 22500, 32000 ...) 00048 this parameter is relative to the audio file/stream type. 00049 ) 00050 This function configures all the hardware required for the audio application (codec, I2C, I2S, 00051 GPIOs, DMA and interrupt if needed). This function returns 0 if configuration is OK. 00052 if the returned value is different from 0 or the function is stuck then the communication with 00053 the codec has failed (try to un-plug the power or reset device in this case). 00054 - OUTPUT_DEVICE_SPEAKER: only speaker will be set as output for the audio stream. 00055 - OUTPUT_DEVICE_HEADPHONE: only headphones will be set as output for the audio stream. 00056 - OUTPUT_DEVICE_AUTO: Selection of output device is made through external switch (implemented 00057 into the audio jack on the evaluation board). When the Headphone is connected it is used 00058 as output. When the headphone is disconnected from the audio jack, the output is 00059 automatically switched to Speaker. 00060 - OUTPUT_DEVICE_BOTH: both Speaker and Headphone are used as outputs for the audio stream 00061 at the same time. 00062 + Call the function BSP_AUDIO_OUT_Play( 00063 pBuffer: pointer to the audio data file address 00064 Size: size of the buffer to be sent in Bytes 00065 ) 00066 to start playing (for the first time) from the audio file/stream. 00067 + Call the function BSP_AUDIO_OUT_Pause() to pause playing 00068 + Call the function BSP_AUDIO_OUT_Resume() to resume playing. 00069 Note. After calling BSP_AUDIO_OUT_Pause() function for pause, only BSP_AUDIO_OUT_Resume() should be called 00070 for resume (it is not allowed to call BSP_AUDIO_OUT_Play() in this case). 00071 Note. This function should be called only when the audio file is played or paused (not stopped). 00072 + For each mode, you may need to implement the relative callback functions into your code. 00073 The Callback functions are named BSP_AUDIO_OUT_XXX_CallBack() and only their prototypes are declared in 00074 the stm32303c_eval_audio.h file. (refer to the example for more details on the callbacks implementations) 00075 + To Stop playing, to modify the volume level or to mute, use the functions 00076 BSP_AUDIO_OUT_Stop(), BSP_AUDIO_OUT_SetVolume(), BSP_AUDIO_OUT_SetFrequency(), BSP_AUDIO_OUT_SetOutputMode() and BSP_AUDIO_OUT_SetMute(). 00077 + The driver API and the callback functions are at the end of the stm32303c_eval_audio.h file. 00078 00079 Driver architecture: 00080 ------------------- 00081 + This driver provide the High Audio Layer: consists of the function API exported in the stm32303c_eval_audio.h file 00082 (BSP_AUDIO_OUT_Init(), BSP_AUDIO_OUT_Play() ...) 00083 + This driver provide also the Media Access Layer (MAL): which consists of functions allowing to access the media containing/ 00084 providing the audio file/stream. These functions are also included as local functions into 00085 the stm32303c_eval_audio.c file (I2Sx_MspInit() and I2Sx_Init()) 00086 00087 Known Limitations: 00088 ------------------- 00089 1- When using the Speaker, if the audio file quality is not high enough, the speaker output 00090 may produce high and uncomfortable noise level. To avoid this issue, to use speaker 00091 output properly, try to increase audio file sampling rate (typically higher than 48KHz). 00092 This operation will lead to larger file size. 00093 2- Communication with the audio codec (through I2C) may be corrupted if it is interrupted by some 00094 user interrupt routines (in this case, interrupts could be disabled just before the start of 00095 communication then re-enabled when it is over). Note that this communication is only done at 00096 the configuration phase (BSP_AUDIO_OUT_Init() or BSP_AUDIO_OUT_Stop()) and when Volume control modification is 00097 performed (BSP_AUDIO_OUT_SetVolume() or AUDIO_OUT_Mute() or BSP_AUDIO_OUT_SetOutputMode()). 00098 When the audio data is played, no communication is required with the audio codec. 00099 3- Parsing of audio file is not implemented (in order to determine audio file properties: Mono/Stereo, Data size, 00100 File size, Audio Frequency, Audio Data header size ...). The configuration is fixed for the given audio file. 00101 4- Mono audio streaming is not supported (in order to play mono audio streams, each data should be sent twice 00102 on the I2S or should be duplicated on the source buffer. Or convert the stream in stereo before playing). 00103 5- Supports only 16-bits audio data size. 00104 ==============================================================================*/ 00105 00106 /* Includes ------------------------------------------------------------------*/ 00107 #include "stm32303c_eval_audio.h" 00108 00109 /** @addtogroup BSP 00110 * @{ 00111 */ 00112 00113 /** @addtogroup STM32303C_EVAL 00114 * @{ 00115 */ 00116 00117 /** @defgroup STM32303C_EVAL_AUDIO STM32303C_EVAL AUDIO 00118 * @brief This file includes the low layer audio driver available on STM32303C_EVAL 00119 * evaluation board(MB1019). 00120 * @{ 00121 */ 00122 00123 /** @defgroup STM32303C_EVAL_AUDIO_Private_Types Private Types 00124 * @{ 00125 */ 00126 /** 00127 * @} 00128 */ 00129 00130 /* Private defines ------------------------------------------------------------*/ 00131 /** @defgroup STM32303C_EVAL_AUDIO_Private_Constants Private Constants 00132 * @{ 00133 */ 00134 /** 00135 * @} 00136 */ 00137 00138 /* Private macros ------------------------------------------------------------*/ 00139 /** @defgroup STM32303C_EVAL_AUDIO_Private_Macros Private Macros 00140 * @{ 00141 */ 00142 /** 00143 * @} 00144 */ 00145 00146 /* Private variables ---------------------------------------------------------*/ 00147 /** @defgroup STM32303C_EVAL_AUDIO_Private_Variables Private Variables 00148 * @{ 00149 */ 00150 00151 /*### PLAY ###*/ 00152 static AUDIO_DrvTypeDef *pAudioDrv = NULL; 00153 I2S_HandleTypeDef hAudioOutI2s; 00154 00155 /** 00156 * @} 00157 */ 00158 00159 /* Private function prototypes -----------------------------------------------*/ 00160 /** @defgroup STM32303C_EVAL_AUDIO_Private_Functions Private Functions 00161 * @{ 00162 */ 00163 static void I2Sx_MspInit(void); 00164 static AUDIO_StatusTypeDef I2Sx_Init(uint32_t AudioFreq); 00165 /** 00166 * @} 00167 */ 00168 00169 /* Exported functions --------------------------------------------------------*/ 00170 /** @addtogroup STM32303C_EVAL_AUDIO_Exported_Functions 00171 * @{ 00172 */ 00173 00174 /** 00175 * @brief Configure the audio peripherals. 00176 * @param OutputDevice OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00177 * OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO . 00178 * @param Volume Initial volume level (from 0 (Mute) to 100 (Max)) 00179 * @param AudioFreq Audio frequency used to play the audio stream. 00180 * @retval AUDIO_OK if correct communication, else wrong communication 00181 */ 00182 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00183 { 00184 uint8_t ret = AUDIO_OK; 00185 uint32_t deviceid = 0x00; 00186 00187 if(pAudioDrv == NULL) 00188 { 00189 deviceid = cs42l52_drv.ReadID(AUDIO_I2C_ADDRESS); 00190 00191 if((deviceid & CS42L52_ID_MASK) == CS42L52_ID) 00192 { 00193 /* Initialize the audio driver structure */ 00194 pAudioDrv = &cs42l52_drv; 00195 ret = AUDIO_OK; 00196 } 00197 else 00198 { 00199 ret = AUDIO_ERROR; 00200 } 00201 } 00202 00203 if(ret == AUDIO_OK) 00204 { 00205 if(pAudioDrv->Init(AUDIO_I2C_ADDRESS, OutputDevice, Volume, AudioFreq) != 0) 00206 { 00207 ret = AUDIO_ERROR; 00208 } 00209 else 00210 { 00211 /* I2S data transfer preparation: 00212 Prepare the Media to be used for the audio transfer from memory to I2S peripheral */ 00213 /* Configure the I2S peripheral */ 00214 ret = I2Sx_Init(AudioFreq); 00215 } 00216 } 00217 00218 return ret; 00219 } 00220 00221 /** 00222 * @brief Starts playing audio stream from a data buffer for a determined size. 00223 * @param pBuffer Pointer to the buffer 00224 * @param Size Number of audio data BYTES. 00225 * @retval AUDIO_OK if correct communication, else wrong communication 00226 */ 00227 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size) 00228 { 00229 /* Call the audio Codec Play function */ 00230 if (pAudioDrv->Play(AUDIO_I2C_ADDRESS, pBuffer, Size) != 0) 00231 { 00232 return AUDIO_ERROR; 00233 } 00234 else 00235 { 00236 /* Update the Media layer and enable it for play */ 00237 return (HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size))); 00238 } 00239 } 00240 00241 /** 00242 * @brief Sends n-Bytes on the I2S interface. 00243 * @param pData pointer on data address 00244 * @param Size number of data to be written 00245 * @retval AUDIO_OK if correct communication, else wrong communication 00246 */ 00247 uint8_t BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00248 { 00249 return (HAL_I2S_Transmit_DMA(&hAudioOutI2s, pData, Size)); 00250 } 00251 00252 /** 00253 * @brief This function Pauses the audio file stream. In case of using DMA, the DMA Pause feature is used. 00254 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00255 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00256 * function for resume could lead to unexpected behavior). 00257 * @retval AUDIO_OK if correct communication, else wrong communication 00258 */ 00259 uint8_t BSP_AUDIO_OUT_Pause(void) 00260 { 00261 /* Call the Audio Codec Pause/Resume function */ 00262 if (pAudioDrv->Pause(AUDIO_I2C_ADDRESS) != 0) 00263 { 00264 return AUDIO_ERROR; 00265 } 00266 else 00267 { 00268 /* Call the Media layer pause function */ 00269 return (HAL_I2S_DMAPause(&hAudioOutI2s)); 00270 } 00271 } 00272 00273 /** 00274 * @brief This function Resumes the audio file stream. 00275 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00276 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00277 * function for resume could lead to unexpected behavior). 00278 * @retval AUDIO_OK if correct communication, else wrong communication 00279 */ 00280 uint8_t BSP_AUDIO_OUT_Resume(void) 00281 { 00282 /* Call the Audio Codec Pause/Resume function */ 00283 if(pAudioDrv->Resume(AUDIO_I2C_ADDRESS) != 0) 00284 { 00285 return AUDIO_ERROR; 00286 } 00287 else 00288 { 00289 /* Call the Media layer resume function */ 00290 return (HAL_I2S_DMAResume(&hAudioOutI2s)); 00291 } 00292 } 00293 00294 /** 00295 * @brief Stops audio playing and Power down the Audio Codec. 00296 * @param Option could be one of the following parameters 00297 * - CODEC_PDWN_SW: for software power off (by writing registers). 00298 * Then no need to reconfigure the Codec after power on. 00299 * - CODEC_PDWN_HW: completely shut down the codec (physically). 00300 * Then need to reconfigure the Codec after power on. 00301 * @retval AUDIO_OK if correct communication, else wrong communication 00302 */ 00303 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option) 00304 { 00305 /* Call DMA Stop to disable DMA stream before stopping codec */ 00306 HAL_I2S_DMAStop(&hAudioOutI2s); 00307 00308 /* Call Audio Codec Stop function */ 00309 if(pAudioDrv->Stop(AUDIO_I2C_ADDRESS, Option) != 0) 00310 { 00311 return AUDIO_ERROR; 00312 } 00313 else 00314 { 00315 if(Option == CODEC_PDWN_HW) 00316 { 00317 /* Wait at least 100us */ 00318 HAL_Delay(1); 00319 } 00320 /* Return AUDIO_OK when all operations are correctly done */ 00321 return AUDIO_OK; 00322 } 00323 } 00324 00325 /** 00326 * @brief Controls the current audio volume level. 00327 * @param Volume Volume level to be set in percentage from 0% to 100% (0 for 00328 * Mute and 100 for Max volume level). 00329 * @retval AUDIO_OK if correct communication, else wrong communication 00330 */ 00331 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume) 00332 { 00333 /* Call the codec volume control function with converted volume value */ 00334 if(pAudioDrv->SetVolume(AUDIO_I2C_ADDRESS, Volume) != 0) 00335 { 00336 return AUDIO_ERROR; 00337 } 00338 else 00339 { 00340 /* Return AUDIO_OK when all operations are correctly done */ 00341 return AUDIO_OK; 00342 } 00343 } 00344 00345 /** 00346 * @brief Enables or disables the MUTE mode by software 00347 * @param Cmd could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00348 * unmute the codec and restore previous volume level. 00349 * @retval AUDIO_OK if correct communication, else wrong communication 00350 */ 00351 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd) 00352 { 00353 /* Call the Codec Mute function */ 00354 if(pAudioDrv->SetMute(AUDIO_I2C_ADDRESS, Cmd) != 0) 00355 { 00356 return AUDIO_ERROR; 00357 } 00358 else 00359 { 00360 /* Return AUDIO_OK when all operations are correctly done */ 00361 return AUDIO_OK; 00362 } 00363 } 00364 00365 /** 00366 * @brief Switch dynamically (while audio file is played) the output target 00367 * (speaker or headphone). 00368 * @note This function modifies a global variable of the audio codec driver: OutputDev. 00369 * @param Output specifies the audio output target: OUTPUT_DEVICE_SPEAKER, 00370 * OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO 00371 * @retval AUDIO_OK if correct communication, else wrong communication 00372 */ 00373 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output) 00374 { 00375 /* Call the Codec output Device function */ 00376 if(pAudioDrv->SetOutputMode(AUDIO_I2C_ADDRESS, Output) != 0) 00377 { 00378 return AUDIO_ERROR; 00379 } 00380 else 00381 { 00382 /* Return AUDIO_OK when all operations are correctly done */ 00383 return AUDIO_OK; 00384 } 00385 } 00386 00387 /** 00388 * @brief Update the audio frequency. 00389 * @param AudioFreq Audio frequency used to play the audio stream. 00390 * @retval AUDIO_OK if correct communication, else wrong communication 00391 */ 00392 uint8_t BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq) 00393 { 00394 /* Update the I2S audio frequency configuration */ 00395 return (I2Sx_Init(AudioFreq)); 00396 } 00397 00398 /** 00399 * @brief Tx Transfer completed callbacks 00400 * @param hi2s I2S handle 00401 * @retval None 00402 */ 00403 void HAL_I2S_TxCpltCallback(I2S_HandleTypeDef *hi2s) 00404 { 00405 if(hi2s->Instance == I2Sx) 00406 { 00407 /* Call the user function which will manage directly transfer complete*/ 00408 BSP_AUDIO_OUT_TransferComplete_CallBack(); 00409 } 00410 } 00411 00412 /** 00413 * @brief Tx Transfer Half completed callbacks 00414 * @param hi2s I2S handle 00415 * @retval None 00416 */ 00417 void HAL_I2S_TxHalfCpltCallback(I2S_HandleTypeDef *hi2s) 00418 { 00419 if(hi2s->Instance == I2Sx) 00420 { 00421 /* Manage the remaining file size and new address offset: This function 00422 should be coded by user (its prototype is already declared in stm32303c_eval_audio.h) */ 00423 BSP_AUDIO_OUT_HalfTransfer_CallBack(); 00424 } 00425 } 00426 00427 /** 00428 * @brief I2S error callbacks 00429 * @param hi2s I2S handle 00430 * @retval None 00431 */ 00432 void HAL_I2S_ErrorCallback(I2S_HandleTypeDef *hi2s) 00433 { 00434 /* Manage the error generated on DMA: This function 00435 should be coded by user (its prototype is already declared in stm32303c_eval_audio.h) */ 00436 if(hi2s->Instance == I2Sx) 00437 { 00438 BSP_AUDIO_OUT_Error_CallBack(); 00439 } 00440 } 00441 00442 /** 00443 * @brief Manages the DMA full Transfer complete event. 00444 * @retval None 00445 */ 00446 __weak void BSP_AUDIO_OUT_TransferComplete_CallBack(void) 00447 { 00448 } 00449 00450 /** 00451 * @brief Manages the DMA Half Transfer complete event. 00452 * @retval None 00453 */ 00454 __weak void BSP_AUDIO_OUT_HalfTransfer_CallBack(void) 00455 { 00456 } 00457 00458 /** 00459 * @brief Audio OUT Error callback function 00460 * @retval None 00461 */ 00462 __weak void BSP_AUDIO_OUT_Error_CallBack(void) 00463 { 00464 } 00465 00466 /** 00467 * @} 00468 */ 00469 00470 /** @addtogroup STM32303C_EVAL_AUDIO_Private_Functions 00471 * @{ 00472 */ 00473 00474 /****************************************************************************** 00475 Static Function 00476 *******************************************************************************/ 00477 00478 /** 00479 * @brief AUDIO OUT I2S MSP Init 00480 * @retval None 00481 */ 00482 static void I2Sx_MspInit(void) 00483 { 00484 static DMA_HandleTypeDef hdma_i2sTx; 00485 GPIO_InitTypeDef GPIO_InitStruct; 00486 I2S_HandleTypeDef *hi2s = &hAudioOutI2s; 00487 00488 /* Enable I2S GPIO clocks */ 00489 I2Sx_MCK_WS_GPIO_CLK_ENABLE(); 00490 I2Sx_SCK_DIN_GPIO_CLK_ENABLE(); 00491 00492 /* I2S pins configuration: SCK and DIN pins -----------------------------*/ 00493 GPIO_InitStruct.Pin = (I2Sx_SCK_PIN | I2Sx_DIN_PIN); 00494 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00495 GPIO_InitStruct.Pull = GPIO_NOPULL; 00496 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00497 GPIO_InitStruct.Alternate = I2Sx_SCK_DIN_AF; 00498 HAL_GPIO_Init(I2Sx_SCK_DIN_GPIO_PORT, &GPIO_InitStruct); 00499 00500 /* I2S pins configuration: WS pin -----------------------------*/ 00501 GPIO_InitStruct.Pin = I2Sx_WS_PIN; 00502 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00503 GPIO_InitStruct.Pull = GPIO_NOPULL; 00504 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00505 GPIO_InitStruct.Alternate = I2Sx_WS_AF; 00506 HAL_GPIO_Init(I2Sx_WS_GPIO_PORT, &GPIO_InitStruct); 00507 00508 /* I2S pins configuration: MCK pin -----------------------------*/ 00509 GPIO_InitStruct.Pin = I2Sx_MCK_PIN; 00510 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00511 GPIO_InitStruct.Pull = GPIO_NOPULL; 00512 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00513 GPIO_InitStruct.Alternate = I2Sx_MCK_AF; 00514 HAL_GPIO_Init(I2Sx_MCK_GPIO_PORT, &GPIO_InitStruct); 00515 00516 /* Enable I2S clock */ 00517 I2Sx_CLK_ENABLE(); 00518 00519 /* Force the I2S peripheral clock reset */ 00520 I2Sx_FORCE_RESET(); 00521 00522 /* Release the I2S peripheral clock reset */ 00523 I2Sx_RELEASE_RESET(); 00524 00525 /* Enable the I2S DMA clock */ 00526 I2Sx_DMAx_CLK_ENABLE(); 00527 00528 /* Configure the hdma_i2sTx handle parameters */ 00529 hdma_i2sTx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00530 hdma_i2sTx.Init.PeriphInc = DMA_PINC_DISABLE; 00531 hdma_i2sTx.Init.MemInc = DMA_MINC_ENABLE; 00532 hdma_i2sTx.Init.PeriphDataAlignment = I2Sx_DMAx_PERIPH_DATA_SIZE; 00533 hdma_i2sTx.Init.MemDataAlignment = I2Sx_DMAx_MEM_DATA_SIZE; 00534 hdma_i2sTx.Init.Mode = DMA_NORMAL; 00535 hdma_i2sTx.Init.Priority = DMA_PRIORITY_HIGH; 00536 00537 hdma_i2sTx.Instance = I2Sx_DMAx_CHANNEL; 00538 00539 /* Associate the DMA handle */ 00540 __HAL_LINKDMA(hi2s, hdmatx, hdma_i2sTx); 00541 00542 /* Configure the DMA Stream */ 00543 HAL_DMA_Init(&hdma_i2sTx); 00544 00545 /* I2S DMA IRQ Channel configuration */ 00546 HAL_NVIC_SetPriority((IRQn_Type)I2Sx_DMAx_IRQ, AUDIO_OUT_IRQ_PREPRIO, AUDIO_OUT_IRQ_SUBPRIO); 00547 HAL_NVIC_EnableIRQ((IRQn_Type)I2Sx_DMAx_IRQ); 00548 } 00549 00550 /** 00551 * @brief Initializes the Audio Codec audio interface (I2S) 00552 * @param AudioFreq Audio frequency to be configured for the I2S peripheral. 00553 * @retval AUDIO_StatusTypeDef AUDIO Status 00554 */ 00555 static AUDIO_StatusTypeDef I2Sx_Init(uint32_t AudioFreq) 00556 { 00557 /* I2S peripheral configuration */ 00558 hAudioOutI2s.Init.AudioFreq = AudioFreq; 00559 hAudioOutI2s.Init.ClockSource = I2S_CLOCK_SYSCLK; 00560 hAudioOutI2s.Init.CPOL = I2S_CPOL_LOW; 00561 hAudioOutI2s.Init.DataFormat = I2S_DATAFORMAT_16B; 00562 hAudioOutI2s.Init.MCLKOutput = I2S_MCLKOUTPUT_ENABLE; 00563 hAudioOutI2s.Init.Mode = I2S_MODE_MASTER_TX; 00564 hAudioOutI2s.Init.Standard = I2S_STANDARD; 00565 hAudioOutI2s.Instance = I2Sx; 00566 00567 /* Disable I2S block */ 00568 __HAL_I2S_DISABLE(&hAudioOutI2s); 00569 00570 /* Initialize the I2S peripheral with the structure above */ 00571 if(HAL_I2S_GetState(&hAudioOutI2s) == HAL_I2S_STATE_RESET) 00572 { 00573 I2Sx_MspInit(); 00574 } 00575 00576 if (HAL_I2S_Init(&hAudioOutI2s) != HAL_OK) 00577 { 00578 return AUDIO_ERROR; 00579 } 00580 00581 return AUDIO_OK; 00582 } 00583 /** 00584 * @} 00585 */ 00586 00587 /** 00588 * @} 00589 */ 00590 00591 /** 00592 * @} 00593 */ 00594 00595 /** 00596 * @} 00597 */ 00598 00599 /** 00600 * @} 00601 */ 00602 00603 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
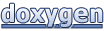