STM3210E_EVAL BSP User Manual
|
Functions | |
void | LCD_IO_WriteData (uint16_t RegValue) |
Writes data on LCD data register. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Writes multiple data on LCD data register. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
Writes register on LCD register. | |
uint16_t | LCD_IO_ReadData (uint16_t Reg) |
Reads data from LCD data register. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
uint8_t | FLASH_SPI_IO_WriteByte (uint8_t Data) |
Write a byte on the FLASH SPI. | |
uint8_t | FLASH_SPI_IO_ReadByte (void) |
Read a byte from the FLASH SPI. | |
HAL_StatusTypeDef | FLASH_SPI_IO_ReadData (uint32_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from FLASH SPI driver. | |
HAL_StatusTypeDef | FLASH_SPI_IO_WaitForWriteEnd (void) |
Wait response from the FLASH SPI and Deselect the device. | |
uint32_t | FLASH_SPI_IO_ReadID (void) |
Reads FLASH SPI identification. | |
void | TSENSOR_IO_Write (uint16_t DevAddress, uint8_t *pBuffer, uint8_t WriteAddr, uint16_t Length) |
Writes one byte to the TSENSOR. | |
void | TSENSOR_IO_Read (uint16_t DevAddress, uint8_t *pBuffer, uint8_t ReadAddr, uint16_t Length) |
Reads one byte from the TSENSOR. | |
uint16_t | TSENSOR_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if Temperature Sensor is ready for communication. | |
void | AUDIO_IO_DeInit (void) |
DeInitializes Audio low level. | |
void | AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
uint8_t | AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. |
Function Documentation
void AUDIO_IO_DeInit | ( | void | ) |
DeInitializes Audio low level.
- Note:
- This function is intentionally kept empty, user should define it.
Definition at line 1315 of file stm3210e_eval.c.
uint8_t AUDIO_IO_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address
- Return values:
-
Data to be read
Definition at line 1337 of file stm3210e_eval.c.
References I2Cx_ReadData().
void AUDIO_IO_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address Value,: Data to be written
Definition at line 1326 of file stm3210e_eval.c.
References I2Cx_WriteData().
uint8_t FLASH_SPI_IO_ReadByte | ( | void | ) |
Read a byte from the FLASH SPI.
- Return values:
-
uint8_t (The received byte).
Definition at line 1102 of file stm3210e_eval.c.
References SPIx_Read().
HAL_StatusTypeDef FLASH_SPI_IO_ReadData | ( | uint32_t | MemAddress, |
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from FLASH SPI driver.
- Parameters:
-
MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be read
- Return values:
-
HAL_StatusTypeDef HAL Status
< Select the FLASH: Chip Select low
< Send "Read from Memory " instruction
< Send ReadAddr high nibble address byte to read from
< Send ReadAddr medium nibble address byte to read from
< Send ReadAddr low nibble address byte to read from
< while there is data to be read
< Read a byte from the FLASH
< Point to the next location where the byte read will be saved
Definition at line 1120 of file stm3210e_eval.c.
References FLASH_SPI_CMD_READ, FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, FLASH_SPI_DUMMY_BYTE, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_ReadData().
uint32_t FLASH_SPI_IO_ReadID | ( | void | ) |
Reads FLASH SPI identification.
- Return values:
-
FLASH identification
< Select the FLASH: Chip Select low
< Send "RDID " instruction
< Read a byte from the FLASH
< Read a byte from the FLASH
< Read a byte from the FLASH
< Deselect the FLASH: Chip Select high
Definition at line 1204 of file stm3210e_eval.c.
References FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, FLASH_SPI_DUMMY_BYTE, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_ReadID().
HAL_StatusTypeDef FLASH_SPI_IO_WaitForWriteEnd | ( | void | ) |
Wait response from the FLASH SPI and Deselect the device.
- Return values:
-
HAL_StatusTypeDef HAL Status
< Select the FLASH: Chip Select low
< Select the FLASH: Chip Select low
< Send "Read Status Register" instruction
< Loop as long as the memory is busy with a write cycle
< Send a dummy byte to generate the clock needed by the FLASH and put the value of the status register in FLASH_Status variable
< Deselect the FLASH: Chip Select high
Definition at line 1171 of file stm3210e_eval.c.
References FLASH_SPI_CMD_RDSR, FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, FLASH_SPI_DUMMY_BYTE, FLASH_SPI_WIP_FLAG, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_EraseBulk(), BSP_SERIAL_FLASH_EraseSector(), and BSP_SERIAL_FLASH_WritePage().
uint8_t FLASH_SPI_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the FLASH SPI.
- Parameters:
-
Data,: byte to send.
Definition at line 1092 of file stm3210e_eval.c.
References SPIx_Write().
Referenced by BSP_SERIAL_FLASH_EraseBulk(), BSP_SERIAL_FLASH_EraseSector(), and BSP_SERIAL_FLASH_WritePage().
void LCD_Delay | ( | uint32_t | Delay | ) |
uint16_t LCD_IO_ReadData | ( | uint16_t | Reg | ) |
Reads data from LCD data register.
- Parameters:
-
Reg,: Register to be read
- Return values:
-
Read data.
Definition at line 1040 of file stm3210e_eval.c.
References FSMC_BANK1NORSRAM4_ReadData().
void LCD_IO_WriteData | ( | uint16_t | RegValue | ) |
Writes data on LCD data register.
- Parameters:
-
RegValue,: Data to be written
- Return values:
-
None
Definition at line 999 of file stm3210e_eval.c.
References FSMC_BANK1NORSRAM4_WriteData().
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Writes multiple data on LCD data register.
- Parameters:
-
pData,: Data to be written Size,: number of data to write
Definition at line 1009 of file stm3210e_eval.c.
References FSMC_BANK1NORSRAM4_WriteData().
void LCD_IO_WriteReg | ( | uint8_t | Reg | ) |
Writes register on LCD register.
- Parameters:
-
Reg,: Register to be written
Definition at line 1030 of file stm3210e_eval.c.
References FSMC_BANK1NORSRAM4_WriteReg().
uint16_t TSENSOR_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if Temperature Sensor is ready for communication.
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 1272 of file stm3210e_eval.c.
References I2Cx_IsDeviceReady().
void TSENSOR_IO_Read | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | ReadAddr, | ||
uint16_t | Length | ||
) |
Reads one byte from the TSENSOR.
- Parameters:
-
DevAddress,: Target device address pBuffer : pointer to the buffer that receives the data read from the TSENSOR. ReadAddr : TSENSOR's internal address to read from. Length,: Number of data to read
Definition at line 1261 of file stm3210e_eval.c.
References I2Cx_ReadBuffer().
void TSENSOR_IO_Write | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | WriteAddr, | ||
uint16_t | Length | ||
) |
Writes one byte to the TSENSOR.
- Parameters:
-
DevAddress,: Target device address pBuffer,: Pointer to data buffer WriteAddr,: TSENSOR's internal address to write to. Length,: Number of data to write
Definition at line 1249 of file stm3210e_eval.c.
References I2Cx_WriteBuffer().
Generated on Fri Feb 24 2017 17:15:12 for STM3210E_EVAL BSP User Manual by
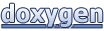