STM3210E_EVAL BSP User Manual
|
stm3210e_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval.c 00004 * @author MCD Application Team 00005 * @version V6.0.2 00006 * @date 29-April-2016 00007 * @brief This file provides a set of firmware functions to manage Leds, 00008 * push-button and COM ports for STM3210E_EVAL 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm3210e_eval.h" 00041 00042 /** @defgroup BSP BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM3210E_EVAL STM3210E EVAL 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM3210E_EVAL_Common STM3210E EVAL Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM3210E_EVAL_Private_TypesDefinitions STM3210E EVAL Private TypesDefinitions 00055 * @{ 00056 */ 00057 00058 typedef struct 00059 { 00060 __IO uint16_t REG; 00061 __IO uint16_t RAM; 00062 00063 }LCD_CONTROLLER_TypeDef; 00064 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM3210E_EVAL_Private_Defines STM3210E EVAL Private Defines 00070 * @{ 00071 */ 00072 00073 /** 00074 * @brief STM3210E EVAL BSP Driver version number 00075 */ 00076 #define __STM3210E_EVAL_BSP_VERSION_MAIN (0x06) /*!< [31:24] main version */ 00077 #define __STM3210E_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00078 #define __STM3210E_EVAL_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00079 #define __STM3210E_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00080 #define __STM3210E_EVAL_BSP_VERSION ((__STM3210E_EVAL_BSP_VERSION_MAIN << 24)\ 00081 |(__STM3210E_EVAL_BSP_VERSION_SUB1 << 16)\ 00082 |(__STM3210E_EVAL_BSP_VERSION_SUB2 << 8 )\ 00083 |(__STM3210E_EVAL_BSP_VERSION_RC)) 00084 00085 00086 /* Note: LCD /CS is CE4 - Bank 4 of NOR/SRAM Bank 1~4 */ 00087 #define TFT_LCD_BASE FSMC_BANK1_4 00088 #define TFT_LCD ((LCD_CONTROLLER_TypeDef *) TFT_LCD_BASE) 00089 00090 /** 00091 * @} 00092 */ 00093 00094 00095 /** @defgroup STM3210E_EVAL_Private_Variables STM3210E EVAL Private Variables 00096 * @{ 00097 */ 00098 /** 00099 * @brief LED variables 00100 */ 00101 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00102 LED2_GPIO_PORT, 00103 LED3_GPIO_PORT, 00104 LED4_GPIO_PORT}; 00105 00106 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00107 LED2_PIN, 00108 LED3_PIN, 00109 LED4_PIN}; 00110 00111 /** 00112 * @brief BUTTON variables 00113 */ 00114 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00115 TAMPER_BUTTON_GPIO_PORT, 00116 KEY_BUTTON_GPIO_PORT, 00117 SEL_JOY_GPIO_PORT, 00118 LEFT_JOY_GPIO_PORT, 00119 RIGHT_JOY_GPIO_PORT, 00120 DOWN_JOY_GPIO_PORT, 00121 UP_JOY_GPIO_PORT}; 00122 00123 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00124 TAMPER_BUTTON_PIN, 00125 KEY_BUTTON_PIN, 00126 SEL_JOY_PIN, 00127 LEFT_JOY_PIN, 00128 RIGHT_JOY_PIN, 00129 DOWN_JOY_PIN, 00130 UP_JOY_PIN}; 00131 00132 const uint8_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00133 TAMPER_BUTTON_EXTI_IRQn, 00134 KEY_BUTTON_EXTI_IRQn, 00135 SEL_JOY_EXTI_IRQn, 00136 LEFT_JOY_EXTI_IRQn, 00137 RIGHT_JOY_EXTI_IRQn, 00138 DOWN_JOY_EXTI_IRQn, 00139 UP_JOY_EXTI_IRQn}; 00140 00141 /** 00142 * @brief JOYSTICK variables 00143 */ 00144 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00145 LEFT_JOY_GPIO_PORT, 00146 RIGHT_JOY_GPIO_PORT, 00147 DOWN_JOY_GPIO_PORT, 00148 UP_JOY_GPIO_PORT}; 00149 00150 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00151 LEFT_JOY_PIN, 00152 RIGHT_JOY_PIN, 00153 DOWN_JOY_PIN, 00154 UP_JOY_PIN}; 00155 00156 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00157 LEFT_JOY_EXTI_IRQn, 00158 RIGHT_JOY_EXTI_IRQn, 00159 DOWN_JOY_EXTI_IRQn, 00160 UP_JOY_EXTI_IRQn}; 00161 00162 /** 00163 * @brief COM variables 00164 */ 00165 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1, EVAL_COM2}; 00166 00167 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT, EVAL_COM2_TX_GPIO_PORT}; 00168 00169 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT, EVAL_COM2_RX_GPIO_PORT}; 00170 00171 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN, EVAL_COM2_TX_PIN}; 00172 00173 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN, EVAL_COM2_RX_PIN}; 00174 00175 /** 00176 * @brief BUS variables 00177 */ 00178 #ifdef HAL_SPI_MODULE_ENABLED 00179 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00180 static SPI_HandleTypeDef heval_Spi; 00181 #endif /* HAL_SPI_MODULE_ENABLED */ 00182 00183 #ifdef HAL_I2C_MODULE_ENABLED 00184 uint32_t I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00185 I2C_HandleTypeDef heval_I2c; 00186 #endif /* HAL_I2C_MODULE_ENABLED */ 00187 00188 /** 00189 * @} 00190 */ 00191 00192 #if defined(HAL_SRAM_MODULE_ENABLED) 00193 00194 static void FSMC_BANK1NORSRAM4_WriteData(uint16_t Data); 00195 static void FSMC_BANK1NORSRAM4_WriteReg(uint8_t Reg); 00196 static uint16_t FSMC_BANK1NORSRAM4_ReadData(uint8_t Reg); 00197 static void FSMC_BANK1NORSRAM4_Init(void); 00198 static void FSMC_BANK1NORSRAM4_MspInit(void); 00199 00200 /* LCD IO functions */ 00201 void LCD_IO_Init(void); 00202 void LCD_IO_WriteData(uint16_t RegValue); 00203 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00204 void LCD_IO_WriteReg(uint8_t Reg); 00205 uint16_t LCD_IO_ReadData(uint16_t Reg); 00206 void LCD_Delay (uint32_t delay); 00207 #endif /*HAL_SRAM_MODULE_ENABLED*/ 00208 00209 /* I2Cx bus function */ 00210 #ifdef HAL_I2C_MODULE_ENABLED 00211 /* Link function for I2C EEPROM peripheral */ 00212 static void I2Cx_Init(void); 00213 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00214 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00215 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00216 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00217 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00218 static void I2Cx_Error (void); 00219 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00220 00221 /* Link functions for Temperature Sensor peripheral */ 00222 void TSENSOR_IO_Init(void); 00223 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00224 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00225 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00226 00227 /* Link function for Audio peripheral */ 00228 void AUDIO_IO_Init(void); 00229 void AUDIO_IO_DeInit(void); 00230 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00231 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00232 00233 #endif /* HAL_I2C_MODULE_ENABLED */ 00234 00235 #ifdef HAL_SPI_MODULE_ENABLED 00236 /* SPIx bus function */ 00237 static HAL_StatusTypeDef SPIx_Init(void); 00238 static uint8_t SPIx_Write(uint8_t Value); 00239 static uint8_t SPIx_Read(void); 00240 static void SPIx_Error (void); 00241 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00242 00243 /* Link function for EEPROM peripheral over SPI */ 00244 HAL_StatusTypeDef FLASH_SPI_IO_Init(void); 00245 uint8_t FLASH_SPI_IO_WriteByte(uint8_t Data); 00246 uint8_t FLASH_SPI_IO_ReadByte(void); 00247 HAL_StatusTypeDef FLASH_SPI_IO_ReadData(uint32_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00248 void FLASH_SPI_IO_WriteEnable(void); 00249 HAL_StatusTypeDef FLASH_SPI_IO_WaitForWriteEnd(void); 00250 uint32_t FLASH_SPI_IO_ReadID(void); 00251 #endif /* HAL_SPI_MODULE_ENABLED */ 00252 00253 /** @defgroup STM3210E_EVAL_Exported_Functions STM3210E EVAL Exported Functions 00254 * @{ 00255 */ 00256 00257 /** 00258 * @brief This method returns the STM3210E EVAL BSP Driver revision 00259 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00260 */ 00261 uint32_t BSP_GetVersion(void) 00262 { 00263 return __STM3210E_EVAL_BSP_VERSION; 00264 } 00265 00266 /** 00267 * @brief Configures LED GPIO. 00268 * @param Led: Specifies the Led to be configured. 00269 * This parameter can be one of following parameters: 00270 * @arg LED1 00271 * @arg LED2 00272 * @arg LED3 00273 * @arg LED4 00274 */ 00275 void BSP_LED_Init(Led_TypeDef Led) 00276 { 00277 GPIO_InitTypeDef gpioinitstruct = {0}; 00278 00279 /* Enable the GPIO_LED clock */ 00280 LEDx_GPIO_CLK_ENABLE(Led); 00281 00282 /* Configure the GPIO_LED pin */ 00283 gpioinitstruct.Pin = LED_PIN[Led]; 00284 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00285 gpioinitstruct.Pull = GPIO_NOPULL; 00286 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00287 00288 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00289 00290 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00291 } 00292 00293 /** 00294 * @brief Turns selected LED On. 00295 * @param Led: Specifies the Led to be set on. 00296 * This parameter can be one of following parameters: 00297 * @arg LED1 00298 * @arg LED2 00299 * @arg LED3 00300 * @arg LED4 00301 */ 00302 void BSP_LED_On(Led_TypeDef Led) 00303 { 00304 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00305 } 00306 00307 /** 00308 * @brief Turns selected LED Off. 00309 * @param Led: Specifies the Led to be set off. 00310 * This parameter can be one of following parameters: 00311 * @arg LED1 00312 * @arg LED2 00313 * @arg LED3 00314 * @arg LED4 00315 */ 00316 void BSP_LED_Off(Led_TypeDef Led) 00317 { 00318 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00319 } 00320 00321 /** 00322 * @brief Toggles the selected LED. 00323 * @param Led: Specifies the Led to be toggled. 00324 * This parameter can be one of following parameters: 00325 * @arg LED1 00326 * @arg LED2 00327 * @arg LED3 00328 * @arg LED4 00329 */ 00330 void BSP_LED_Toggle(Led_TypeDef Led) 00331 { 00332 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00333 } 00334 00335 /** 00336 * @brief Configures push button GPIO and EXTI Line. 00337 * @param Button: Button to be configured. 00338 * This parameter can be one of the following values: 00339 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00340 * @arg BUTTON_SEL : Sel Push Button on Joystick 00341 * @arg BUTTON_LEFT : Left Push Button on Joystick 00342 * @arg BUTTON_RIGHT : Right Push Button on Joystick 00343 * @arg BUTTON_DOWN : Down Push Button on Joystick 00344 * @arg BUTTON_UP : Up Push Button on Joystick 00345 * @param Button_Mode: Button mode requested. 00346 * This parameter can be one of the following values: 00347 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00348 * @arg BUTTON_MODE_EVT : Button will be connected to EXTI line 00349 * with event generation capability 00350 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00351 * with interrupt generation capability 00352 */ 00353 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00354 { 00355 GPIO_InitTypeDef gpioinitstruct = {0}; 00356 00357 /* Enable the corresponding Push Button clock */ 00358 BUTTONx_GPIO_CLK_ENABLE(Button); 00359 00360 /* Configure Push Button pin as input */ 00361 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00362 gpioinitstruct.Pull = GPIO_PULLDOWN; 00363 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00364 00365 if (Button_Mode == BUTTON_MODE_GPIO) 00366 { 00367 /* Configure Button pin as input */ 00368 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00369 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00370 } 00371 else if (Button_Mode == BUTTON_MODE_EXTI) 00372 { 00373 if(Button != BUTTON_WAKEUP) 00374 { 00375 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00376 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00377 } 00378 else 00379 { 00380 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00381 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00382 } 00383 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00384 00385 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00386 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00387 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00388 } 00389 else if (Button_Mode == BUTTON_MODE_EVT) 00390 { 00391 if(Button != BUTTON_WAKEUP) 00392 { 00393 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00394 gpioinitstruct.Mode = GPIO_MODE_EVT_FALLING; 00395 } 00396 else 00397 { 00398 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00399 gpioinitstruct.Mode = GPIO_MODE_EVT_RISING; 00400 } 00401 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00402 } 00403 } 00404 00405 /** 00406 * @brief Returns the selected button state. 00407 * @param Button: Button to be checked. 00408 * This parameter can be one of the following values: 00409 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00410 * @retval Button state 00411 */ 00412 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00413 { 00414 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00415 } 00416 00417 /** 00418 * @brief Configures all button of the joystick in GPIO or EXTI modes. 00419 * @param Joy_Mode: Joystick mode. 00420 * This parameter can be one of the following values: 00421 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00422 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00423 * with interrupt generation capability 00424 * @retval HAL_OK: if all initializations are OK. Other value if error. 00425 */ 00426 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00427 { 00428 JOYState_TypeDef joykey = JOY_NONE; 00429 GPIO_InitTypeDef gpioinitstruct = {0}; 00430 00431 /* Initialized the Joystick. */ 00432 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00433 { 00434 /* Enable the JOY clock */ 00435 JOYx_GPIO_CLK_ENABLE(joykey); 00436 00437 gpioinitstruct.Pin = JOY_PIN[joykey]; 00438 gpioinitstruct.Pull = GPIO_NOPULL; 00439 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00440 00441 if (Joy_Mode == JOY_MODE_GPIO) 00442 { 00443 /* Configure Joy pin as input */ 00444 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00445 HAL_GPIO_Init(JOY_PORT[joykey], &gpioinitstruct); 00446 } 00447 00448 if (Joy_Mode == JOY_MODE_EXTI) 00449 { 00450 /* Configure Joy pin as input with External interrupt */ 00451 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00452 HAL_GPIO_Init(JOY_PORT[joykey], &gpioinitstruct); 00453 00454 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00455 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x0F, 0); 00456 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00457 } 00458 } 00459 00460 return HAL_OK; 00461 } 00462 00463 /** 00464 * @brief Returns the current joystick status. 00465 * @retval Code of the joystick key pressed 00466 * This code can be one of the following values: 00467 * @arg JOY_SEL 00468 * @arg JOY_DOWN 00469 * @arg JOY_LEFT 00470 * @arg JOY_RIGHT 00471 * @arg JOY_UP 00472 * @arg JOY_NONE 00473 */ 00474 JOYState_TypeDef BSP_JOY_GetState(void) 00475 { 00476 JOYState_TypeDef joykey = JOY_NONE; 00477 00478 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00479 { 00480 if(HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_RESET) 00481 { 00482 /* Return Code Joystick key pressed */ 00483 return joykey; 00484 } 00485 } 00486 00487 /* No Joystick key pressed */ 00488 return JOY_NONE; 00489 } 00490 00491 #ifdef HAL_UART_MODULE_ENABLED 00492 /** 00493 * @brief Configures COM port. 00494 * @param COM: Specifies the COM port to be configured. 00495 * This parameter can be one of following parameters: 00496 * @arg COM1 00497 * @param huart: pointer to a UART_HandleTypeDef structure that 00498 * contains the configuration information for the specified UART peripheral. 00499 */ 00500 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00501 { 00502 GPIO_InitTypeDef gpioinitstruct = {0}; 00503 00504 /* Enable GPIO clock */ 00505 COMx_TX_GPIO_CLK_ENABLE(COM); 00506 COMx_RX_GPIO_CLK_ENABLE(COM); 00507 00508 /* Enable USART clock */ 00509 COMx_CLK_ENABLE(COM); 00510 00511 /* Configure USART Tx as alternate function push-pull */ 00512 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00513 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00514 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00515 gpioinitstruct.Pull = GPIO_PULLUP; 00516 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00517 00518 /* Configure USART Rx as alternate function push-pull */ 00519 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00520 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00521 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00522 00523 /* USART configuration */ 00524 huart->Instance = COM_USART[COM]; 00525 HAL_UART_Init(huart); 00526 } 00527 #endif /* HAL_UART_MODULE_ENABLED */ 00528 00529 /** 00530 * @} 00531 */ 00532 00533 /** @defgroup STM3210E_EVAL_BusOperations_Functions STM3210E EVAL BusOperations Functions 00534 * @{ 00535 */ 00536 00537 /******************************************************************************* 00538 BUS OPERATIONS 00539 *******************************************************************************/ 00540 00541 /*************************** FSMC Routines ************************************/ 00542 #if defined(HAL_SRAM_MODULE_ENABLED) 00543 /** 00544 * @brief Initializes FSMC_BANK4 MSP. 00545 */ 00546 static void FSMC_BANK1NORSRAM4_MspInit(void) 00547 { 00548 GPIO_InitTypeDef gpioinitstruct = {0}; 00549 00550 /* Enable FMC clock */ 00551 __HAL_RCC_FSMC_CLK_ENABLE(); 00552 00553 /* Enable GPIOs clock */ 00554 __HAL_RCC_GPIOD_CLK_ENABLE(); 00555 __HAL_RCC_GPIOE_CLK_ENABLE(); 00556 __HAL_RCC_GPIOF_CLK_ENABLE(); 00557 __HAL_RCC_GPIOG_CLK_ENABLE(); 00558 __HAL_RCC_AFIO_CLK_ENABLE(); 00559 00560 /* Common GPIO configuration */ 00561 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00562 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00563 00564 /* Set PD.00(D2), PD.01(D3), PD.04(NOE), PD.05(NWE), PD.08(D13), PD.09(D14), 00565 PD.10(D15), PD.14(D0), PD.15(D1) as alternate function push pull */ 00566 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | 00567 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | 00568 GPIO_PIN_15; 00569 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00570 00571 /* Set PE.07(D4), PE.08(D5), PE.09(D6), PE.10(D7), PE.11(D8), PE.12(D9), PE.13(D10), 00572 PE.14(D11), PE.15(D12) as alternate function push pull */ 00573 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00574 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | 00575 GPIO_PIN_15; 00576 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00577 00578 /* Set PF.00(A0 (RS)) as alternate function push pull */ 00579 gpioinitstruct.Pin = GPIO_PIN_0; 00580 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00581 00582 /* Set PG.12(NE4 (LCD/CS)) as alternate function push pull - CE3(LCD /CS) */ 00583 gpioinitstruct.Pin = GPIO_PIN_12; 00584 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00585 } 00586 00587 /** 00588 * @brief Initializes LCD IO. 00589 */ 00590 static void FSMC_BANK1NORSRAM4_Init(void) 00591 { 00592 SRAM_HandleTypeDef hsram; 00593 FSMC_NORSRAM_TimingTypeDef sramtiming = {0}; 00594 00595 /*** Configure the SRAM Bank 4 ***/ 00596 /* Configure IPs */ 00597 hsram.Instance = FSMC_NORSRAM_DEVICE; 00598 hsram.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00599 00600 sramtiming.AddressSetupTime = 1; 00601 sramtiming.AddressHoldTime = 1; 00602 sramtiming.DataSetupTime = 2; 00603 sramtiming.BusTurnAroundDuration = 1; 00604 sramtiming.CLKDivision = 2; 00605 sramtiming.DataLatency = 2; 00606 sramtiming.AccessMode = FSMC_ACCESS_MODE_A; 00607 00608 /* Color LCD configuration 00609 LCD configured as follow: 00610 - Data/Address MUX = Disable 00611 - Memory Type = SRAM 00612 - Data Width = 16bit 00613 - Write Operation = Enable 00614 - Extended Mode = Enable 00615 - Asynchronous Wait = Disable */ 00616 hsram.Init.NSBank = FSMC_NORSRAM_BANK4; 00617 hsram.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00618 hsram.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00619 hsram.Init.MemoryDataWidth = FSMC_NORSRAM_MEM_BUS_WIDTH_16; 00620 hsram.Init.BurstAccessMode = FSMC_BURST_ACCESS_MODE_DISABLE; 00621 hsram.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00622 hsram.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00623 hsram.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00624 hsram.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00625 hsram.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00626 hsram.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00627 hsram.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00628 hsram.Init.WriteBurst = FSMC_WRITE_BURST_DISABLE; 00629 00630 /* Initialize the SRAM controller */ 00631 FSMC_BANK1NORSRAM4_MspInit(); 00632 HAL_SRAM_Init(&hsram, &sramtiming, &sramtiming); 00633 } 00634 00635 /** 00636 * @brief Writes register value. 00637 */ 00638 static void FSMC_BANK1NORSRAM4_WriteData(uint16_t Data) 00639 { 00640 /* Write 16-bit Data */ 00641 TFT_LCD->RAM = Data; 00642 } 00643 00644 /** 00645 * @brief Writes register address. 00646 * @param Reg: 00647 * @retval None 00648 */ 00649 static void FSMC_BANK1NORSRAM4_WriteReg(uint8_t Reg) 00650 { 00651 /* Write 16-bit Index, then Write Reg */ 00652 TFT_LCD->REG = Reg; 00653 } 00654 00655 /** 00656 * @brief Reads register value. 00657 * @retval Read value 00658 */ 00659 static uint16_t FSMC_BANK1NORSRAM4_ReadData(uint8_t Reg) 00660 { 00661 /* Write 16-bit Index (then Read Reg) */ 00662 TFT_LCD->REG = Reg; 00663 /* Read 16-bit Reg */ 00664 return (TFT_LCD->RAM); 00665 } 00666 #endif /*HAL_SRAM_MODULE_ENABLED*/ 00667 00668 #ifdef HAL_I2C_MODULE_ENABLED 00669 /******************************* I2C Routines**********************************/ 00670 00671 /** 00672 * @brief Eval I2Cx MSP Initialization 00673 * @param hi2c: I2C handle 00674 */ 00675 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00676 { 00677 GPIO_InitTypeDef gpioinitstruct = {0}; 00678 00679 if (hi2c->Instance == EVAL_I2Cx) 00680 { 00681 /*## Configure the GPIOs ################################################*/ 00682 00683 /* Enable GPIO clock */ 00684 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00685 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00686 00687 /* Configure I2C Tx as alternate function */ 00688 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN; 00689 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00690 gpioinitstruct.Pull = GPIO_NOPULL; 00691 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00692 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00693 00694 /* Configure I2C Rx as alternate function */ 00695 gpioinitstruct.Pin = EVAL_I2Cx_SDA_PIN; 00696 HAL_GPIO_Init(EVAL_I2Cx_SDA_GPIO_PORT, &gpioinitstruct); 00697 00698 00699 /*## Configure the Eval I2Cx peripheral #######################################*/ 00700 /* Enable Eval_I2Cx clock */ 00701 EVAL_I2Cx_CLK_ENABLE(); 00702 00703 /* Add delay related to RCC workaround */ 00704 while (READ_BIT(RCC->APB1ENR, RCC_APB1ENR_I2C1EN) != RCC_APB1ENR_I2C1EN) {}; 00705 00706 /* Force the I2C Periheral Clock Reset */ 00707 EVAL_I2Cx_FORCE_RESET(); 00708 00709 /* Release the I2C Periheral Clock Reset */ 00710 EVAL_I2Cx_RELEASE_RESET(); 00711 00712 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00713 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0xE, 0); 00714 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00715 00716 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00717 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0xE, 0); 00718 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00719 } 00720 } 00721 00722 /** 00723 * @brief Eval I2Cx Bus initialization 00724 */ 00725 static void I2Cx_Init(void) 00726 { 00727 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00728 { 00729 heval_I2c.Instance = EVAL_I2Cx; 00730 heval_I2c.Init.ClockSpeed = BSP_I2C_SPEED; 00731 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00732 heval_I2c.Init.OwnAddress1 = 0; 00733 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00734 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00735 heval_I2c.Init.OwnAddress2 = 0; 00736 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00737 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00738 00739 /* Init the I2C */ 00740 I2Cx_MspInit(&heval_I2c); 00741 HAL_I2C_Init(&heval_I2c); 00742 } 00743 } 00744 00745 /** 00746 * @brief Write a value in a register of the device through BUS. 00747 * @param Addr: Device address on BUS Bus. 00748 * @param Reg: The target register address to write 00749 * @param Value: The target register value to be written 00750 */ 00751 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00752 { 00753 HAL_StatusTypeDef status = HAL_OK; 00754 00755 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00756 00757 /* Check the communication status */ 00758 if(status != HAL_OK) 00759 { 00760 /* Execute user timeout callback */ 00761 I2Cx_Error(); 00762 } 00763 } 00764 00765 /** 00766 * @brief Write a value in a register of the device through BUS. 00767 * @param Addr: Device address on BUS Bus. 00768 * @param Reg: The target register address to write 00769 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00770 * @param pBuffer: The target register value to be written 00771 * @param Length: buffer size to be written 00772 */ 00773 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00774 { 00775 HAL_StatusTypeDef status = HAL_OK; 00776 00777 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00778 00779 /* Check the communication status */ 00780 if(status != HAL_OK) 00781 { 00782 /* Re-Initiaize the BUS */ 00783 I2Cx_Error(); 00784 } 00785 return status; 00786 } 00787 00788 /** 00789 * @brief Read a value in a register of the device through BUS. 00790 * @param Addr: Device address on BUS Bus. 00791 * @param Reg: The target register address to write 00792 * @retval Data read at register @ 00793 */ 00794 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00795 { 00796 HAL_StatusTypeDef status = HAL_OK; 00797 uint8_t value = 0; 00798 00799 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00800 00801 /* Check the communication status */ 00802 if(status != HAL_OK) 00803 { 00804 /* Execute user timeout callback */ 00805 I2Cx_Error(); 00806 00807 } 00808 return value; 00809 } 00810 00811 /** 00812 * @brief Reads multiple data on the BUS. 00813 * @param Addr: I2C Address 00814 * @param Reg: Reg Address 00815 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00816 * @param pBuffer: pointer to read data buffer 00817 * @param Length: length of the data 00818 * @retval 0 if no problems to read multiple data 00819 */ 00820 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00821 { 00822 HAL_StatusTypeDef status = HAL_OK; 00823 00824 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00825 00826 /* Check the communication status */ 00827 if(status != HAL_OK) 00828 { 00829 /* Re-Initiaize the BUS */ 00830 I2Cx_Error(); 00831 } 00832 return status; 00833 } 00834 00835 /** 00836 * @brief Checks if target device is ready for communication. 00837 * @note This function is used with Memory devices 00838 * @param DevAddress: Target device address 00839 * @param Trials: Number of trials 00840 * @retval HAL status 00841 */ 00842 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00843 { 00844 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2cxTimeout)); 00845 } 00846 00847 00848 /** 00849 * @brief Eval I2Cx error treatment function 00850 * @retval None 00851 */ 00852 static void I2Cx_Error (void) 00853 { 00854 /* De-initialize the I2C communication BUS */ 00855 HAL_I2C_DeInit(&heval_I2c); 00856 00857 /* Re- Initiaize the I2C communication BUS */ 00858 I2Cx_Init(); 00859 } 00860 00861 #endif /* HAL_I2C_MODULE_ENABLED */ 00862 00863 /******************************* SPI Routines**********************************/ 00864 #ifdef HAL_SPI_MODULE_ENABLED 00865 /** 00866 * @brief Initializes SPI MSP. 00867 */ 00868 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00869 { 00870 GPIO_InitTypeDef gpioinitstruct = {0}; 00871 00872 /*** Configure the GPIOs ***/ 00873 /* Enable GPIO clock */ 00874 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00875 EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00876 00877 /* configure SPI SCK */ 00878 gpioinitstruct.Pin = EVAL_SPIx_SCK_PIN; 00879 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00880 gpioinitstruct.Pull = GPIO_NOPULL; 00881 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00882 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00883 00884 /* configure SPI MISO and MOSI */ 00885 gpioinitstruct.Pin = (EVAL_SPIx_MISO_PIN | EVAL_SPIx_MOSI_PIN); 00886 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00887 gpioinitstruct.Pull = GPIO_NOPULL; 00888 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00889 HAL_GPIO_Init(EVAL_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00890 00891 /*** Configure the SPI peripheral ***/ 00892 /* Enable SPI clock */ 00893 EVAL_SPIx_CLK_ENABLE(); 00894 } 00895 00896 /** 00897 * @brief Initializes SPI HAL. 00898 */ 00899 HAL_StatusTypeDef SPIx_Init(void) 00900 { 00901 /* DeInitializes the SPI peripheral */ 00902 heval_Spi.Instance = EVAL_SPIx; 00903 HAL_SPI_DeInit(&heval_Spi); 00904 00905 /* SPI Config */ 00906 /* SPI baudrate is set to 36 MHz (PCLK2/SPI_BaudRatePrescaler = 72/2 = 36 MHz) */ 00907 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_2; 00908 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00909 heval_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00910 heval_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00911 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00912 heval_Spi.Init.CRCPolynomial = 7; 00913 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00914 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00915 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00916 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00917 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00918 00919 SPIx_MspInit(&heval_Spi); 00920 00921 return (HAL_SPI_Init(&heval_Spi)); 00922 } 00923 00924 00925 /** 00926 * @brief SPI Write a byte to device 00927 * @param WriteValue to be written 00928 * @retval The value of the received byte. 00929 */ 00930 static uint8_t SPIx_Write(uint8_t WriteValue) 00931 { 00932 HAL_StatusTypeDef status = HAL_OK; 00933 uint8_t ReadValue = 0; 00934 00935 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &WriteValue, (uint8_t*) &ReadValue, 1, SpixTimeout); 00936 00937 /* Check the communication status */ 00938 if(status != HAL_OK) 00939 { 00940 /* Execute user timeout callback */ 00941 SPIx_Error(); 00942 } 00943 00944 return ReadValue; 00945 } 00946 00947 00948 /** 00949 * @brief SPI Read 1 byte from device 00950 * @retval Read data 00951 */ 00952 static uint8_t SPIx_Read(void) 00953 { 00954 return (SPIx_Write(FLASH_SPI_DUMMY_BYTE)); 00955 } 00956 00957 00958 /** 00959 * @brief SPI error treatment function 00960 */ 00961 static void SPIx_Error (void) 00962 { 00963 /* De-initialize the SPI communication BUS */ 00964 HAL_SPI_DeInit(&heval_Spi); 00965 00966 /* Re- Initiaize the SPI communication BUS */ 00967 SPIx_Init(); 00968 } 00969 #endif /* HAL_SPI_MODULE_ENABLED */ 00970 00971 /** 00972 * @} 00973 */ 00974 00975 /** @defgroup STM3210E_EVAL_LinkOperations_Functions STM3210E EVAL LinkOperations Functions 00976 * @{ 00977 */ 00978 00979 /******************************************************************************* 00980 LINK OPERATIONS 00981 *******************************************************************************/ 00982 00983 #if defined(HAL_SRAM_MODULE_ENABLED) 00984 /********************************* LINK LCD ***********************************/ 00985 00986 /** 00987 * @brief Initializes LCD low level. 00988 */ 00989 void LCD_IO_Init(void) 00990 { 00991 FSMC_BANK1NORSRAM4_Init(); 00992 } 00993 00994 /** 00995 * @brief Writes data on LCD data register. 00996 * @param RegValue: Data to be written 00997 * @retval None 00998 */ 00999 void LCD_IO_WriteData(uint16_t RegValue) 01000 { 01001 FSMC_BANK1NORSRAM4_WriteData(RegValue); 01002 } 01003 01004 /** 01005 * @brief Writes multiple data on LCD data register. 01006 * @param pData: Data to be written 01007 * @param Size: number of data to write 01008 */ 01009 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 01010 { 01011 uint32_t counter = 0; 01012 uint16_t regvalue; 01013 01014 regvalue = *pData | (*(pData+1) << 8); 01015 01016 for (counter = Size; counter != 0; counter--) 01017 { 01018 /* Write 16-bit Reg */ 01019 FSMC_BANK1NORSRAM4_WriteData(regvalue); 01020 counter--; 01021 pData += 2; 01022 regvalue = *pData | (*(pData+1) << 8); 01023 } 01024 } 01025 01026 /** 01027 * @brief Writes register on LCD register. 01028 * @param Reg: Register to be written 01029 */ 01030 void LCD_IO_WriteReg(uint8_t Reg) 01031 { 01032 FSMC_BANK1NORSRAM4_WriteReg(Reg); 01033 } 01034 01035 /** 01036 * @brief Reads data from LCD data register. 01037 * @param Reg: Register to be read 01038 * @retval Read data. 01039 */ 01040 uint16_t LCD_IO_ReadData(uint16_t Reg) 01041 { 01042 /* Read 16-bit Reg */ 01043 return (FSMC_BANK1NORSRAM4_ReadData(Reg)); 01044 } 01045 01046 /** 01047 * @brief Wait for loop in ms. 01048 * @param Delay in ms. 01049 */ 01050 void LCD_Delay (uint32_t Delay) 01051 { 01052 HAL_Delay(Delay); 01053 } 01054 01055 #endif /*HAL_SRAM_MODULE_ENABLED*/ 01056 01057 #ifdef HAL_SPI_MODULE_ENABLED 01058 /******************************** LINK FLASH SPI ********************************/ 01059 /** 01060 * @brief Initializes the FLASH SPI and put it into StandBy State (Ready for 01061 * data transfer). 01062 */ 01063 HAL_StatusTypeDef FLASH_SPI_IO_Init(void) 01064 { 01065 HAL_StatusTypeDef Status = HAL_OK; 01066 01067 GPIO_InitTypeDef gpioinitstruct = {0}; 01068 01069 /* EEPROM_CS_GPIO Periph clock enable */ 01070 FLASH_SPI_CS_GPIO_CLK_ENABLE(); 01071 01072 /* Configure EEPROM_CS_PIN pin: EEPROM SPI CS pin */ 01073 gpioinitstruct.Pin = FLASH_SPI_CS_PIN; 01074 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01075 gpioinitstruct.Pull = GPIO_PULLUP; 01076 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 01077 HAL_GPIO_Init(FLASH_SPI_CS_GPIO_PORT, &gpioinitstruct); 01078 01079 /* SPI FLASH Config */ 01080 Status = SPIx_Init(); 01081 01082 /* EEPROM chip select high */ 01083 FLASH_SPI_CS_HIGH(); 01084 01085 return Status; 01086 } 01087 01088 /** 01089 * @brief Write a byte on the FLASH SPI. 01090 * @param Data: byte to send. 01091 */ 01092 uint8_t FLASH_SPI_IO_WriteByte(uint8_t Data) 01093 { 01094 /* Send the byte */ 01095 return (SPIx_Write(Data)); 01096 } 01097 01098 /** 01099 * @brief Read a byte from the FLASH SPI. 01100 * @retval uint8_t (The received byte). 01101 */ 01102 uint8_t FLASH_SPI_IO_ReadByte(void) 01103 { 01104 uint8_t data = 0; 01105 01106 /* Get the received data */ 01107 data = SPIx_Read(); 01108 01109 /* Return the shifted data */ 01110 return data; 01111 } 01112 01113 /** 01114 * @brief Read data from FLASH SPI driver 01115 * @param MemAddress: Internal memory address 01116 * @param pBuffer: Pointer to data buffer 01117 * @param BufferSize: Amount of data to be read 01118 * @retval HAL_StatusTypeDef HAL Status 01119 */ 01120 HAL_StatusTypeDef FLASH_SPI_IO_ReadData(uint32_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01121 { 01122 /*!< Select the FLASH: Chip Select low */ 01123 FLASH_SPI_CS_LOW(); 01124 01125 /*!< Send "Read from Memory " instruction */ 01126 SPIx_Write(FLASH_SPI_CMD_READ); 01127 01128 /*!< Send ReadAddr high nibble address byte to read from */ 01129 SPIx_Write((MemAddress & 0xFF0000) >> 16); 01130 /*!< Send ReadAddr medium nibble address byte to read from */ 01131 SPIx_Write((MemAddress& 0xFF00) >> 8); 01132 /*!< Send ReadAddr low nibble address byte to read from */ 01133 SPIx_Write(MemAddress & 0xFF); 01134 01135 while (BufferSize--) /*!< while there is data to be read */ 01136 { 01137 /*!< Read a byte from the FLASH */ 01138 *pBuffer = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01139 /*!< Point to the next location where the byte read will be saved */ 01140 pBuffer++; 01141 } 01142 01143 /*!< Deselect the FLASH: Chip Select high */ 01144 FLASH_SPI_CS_HIGH(); 01145 01146 return HAL_OK; 01147 } 01148 01149 /** 01150 * @brief Select the FLASH SPI and send "Write Enable" instruction 01151 */ 01152 void FLASH_SPI_IO_WriteEnable(void) 01153 { 01154 /*!< Select the FLASH: Chip Select low */ 01155 FLASH_SPI_CS_LOW(); 01156 01157 /*!< Send "Write Enable" instruction */ 01158 SPIx_Write(FLASH_SPI_CMD_WREN); 01159 01160 /*!< Select the FLASH: Chip Select low */ 01161 FLASH_SPI_CS_HIGH(); 01162 01163 /*!< Select the FLASH: Chip Select low */ 01164 FLASH_SPI_CS_LOW(); 01165 } 01166 01167 /** 01168 * @brief Wait response from the FLASH SPI and Deselect the device 01169 * @retval HAL_StatusTypeDef HAL Status 01170 */ 01171 HAL_StatusTypeDef FLASH_SPI_IO_WaitForWriteEnd(void) 01172 { 01173 /*!< Select the FLASH: Chip Select low */ 01174 FLASH_SPI_CS_HIGH(); 01175 01176 /*!< Select the FLASH: Chip Select low */ 01177 FLASH_SPI_CS_LOW(); 01178 01179 uint8_t flashstatus = 0; 01180 01181 /*!< Send "Read Status Register" instruction */ 01182 SPIx_Write(FLASH_SPI_CMD_RDSR); 01183 01184 /*!< Loop as long as the memory is busy with a write cycle */ 01185 do 01186 { 01187 /*!< Send a dummy byte to generate the clock needed by the FLASH 01188 and put the value of the status register in FLASH_Status variable */ 01189 flashstatus = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01190 01191 } 01192 while ((flashstatus & FLASH_SPI_WIP_FLAG) == SET); /* Write in progress */ 01193 01194 /*!< Deselect the FLASH: Chip Select high */ 01195 FLASH_SPI_CS_HIGH(); 01196 01197 return HAL_OK; 01198 } 01199 01200 /** 01201 * @brief Reads FLASH SPI identification. 01202 * @retval FLASH identification 01203 */ 01204 uint32_t FLASH_SPI_IO_ReadID(void) 01205 { 01206 uint32_t Temp = 0, Temp0 = 0, Temp1 = 0, Temp2 = 0; 01207 01208 /*!< Select the FLASH: Chip Select low */ 01209 FLASH_SPI_CS_LOW(); 01210 01211 /*!< Send "RDID " instruction */ 01212 SPIx_Write(0x9F); 01213 01214 /*!< Read a byte from the FLASH */ 01215 Temp0 = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01216 01217 /*!< Read a byte from the FLASH */ 01218 Temp1 = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01219 01220 /*!< Read a byte from the FLASH */ 01221 Temp2 = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01222 01223 /*!< Deselect the FLASH: Chip Select high */ 01224 FLASH_SPI_CS_HIGH(); 01225 01226 Temp = (Temp0 << 16) | (Temp1 << 8) | Temp2; 01227 01228 return Temp; 01229 } 01230 #endif /* HAL_SPI_MODULE_ENABLED */ 01231 01232 #ifdef HAL_I2C_MODULE_ENABLED 01233 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01234 /** 01235 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01236 */ 01237 void TSENSOR_IO_Init(void) 01238 { 01239 I2Cx_Init(); 01240 } 01241 01242 /** 01243 * @brief Writes one byte to the TSENSOR. 01244 * @param DevAddress: Target device address 01245 * @param pBuffer: Pointer to data buffer 01246 * @param WriteAddr: TSENSOR's internal address to write to. 01247 * @param Length: Number of data to write 01248 */ 01249 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01250 { 01251 I2Cx_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01252 } 01253 01254 /** 01255 * @brief Reads one byte from the TSENSOR. 01256 * @param DevAddress: Target device address 01257 * @param pBuffer : pointer to the buffer that receives the data read from the TSENSOR. 01258 * @param ReadAddr : TSENSOR's internal address to read from. 01259 * @param Length: Number of data to read 01260 */ 01261 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01262 { 01263 I2Cx_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01264 } 01265 01266 /** 01267 * @brief Checks if Temperature Sensor is ready for communication. 01268 * @param DevAddress: Target device address 01269 * @param Trials: Number of trials 01270 * @retval HAL status 01271 */ 01272 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01273 { 01274 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01275 } 01276 01277 /********************************* LINK AUDIO ***********************************/ 01278 01279 /** 01280 * @brief Initializes Audio low level. 01281 */ 01282 void AUDIO_IO_Init (void) 01283 { 01284 GPIO_InitTypeDef gpioinitstruct = {0}; 01285 01286 /* Enable Reset GPIO Clock */ 01287 AUDIO_RESET_GPIO_CLK_ENABLE(); 01288 01289 /* Audio reset pin configuration -------------------------------------------------*/ 01290 gpioinitstruct.Pin = AUDIO_RESET_PIN; 01291 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01292 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 01293 gpioinitstruct.Pull = GPIO_NOPULL; 01294 HAL_GPIO_Init(AUDIO_RESET_GPIO, &gpioinitstruct); 01295 01296 I2Cx_Init(); 01297 01298 /* Power Down the codec */ 01299 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_RESET); 01300 01301 /* wait for a delay to insure registers erasing */ 01302 HAL_Delay(5); 01303 01304 /* Power on the codec */ 01305 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_SET); 01306 01307 /* wait for a delay to insure registers erasing */ 01308 HAL_Delay(5); 01309 } 01310 01311 /** 01312 * @brief DeInitializes Audio low level. 01313 * @note This function is intentionally kept empty, user should define it. 01314 */ 01315 void AUDIO_IO_DeInit(void) 01316 { 01317 01318 } 01319 01320 /** 01321 * @brief Writes a single data. 01322 * @param Addr: I2C address 01323 * @param Reg: Reg address 01324 * @param Value: Data to be written 01325 */ 01326 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 01327 { 01328 I2Cx_WriteData(Addr, Reg, Value); 01329 } 01330 01331 /** 01332 * @brief Reads a single data. 01333 * @param Addr: I2C address 01334 * @param Reg: Reg address 01335 * @retval Data to be read 01336 */ 01337 uint8_t AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) 01338 { 01339 return I2Cx_ReadData(Addr, Reg); 01340 } 01341 01342 #endif /* HAL_I2C_MODULE_ENABLED */ 01343 01344 /** 01345 * @} 01346 */ 01347 01348 /** 01349 * @} 01350 */ 01351 01352 /** 01353 * @} 01354 */ 01355 01356 /** 01357 * @} 01358 */ 01359 01360 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01361
Generated on Fri Feb 24 2017 17:15:11 for STM3210E_EVAL BSP User Manual by
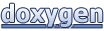