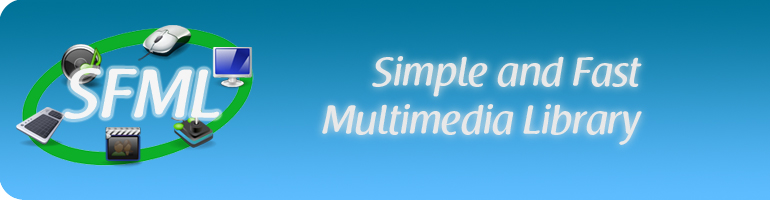
WindowImpl.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Window/WindowImpl.hpp> 00029 #include <SFML/Window/Event.hpp> 00030 #include <SFML/Window/WindowListener.hpp> 00031 #include <algorithm> 00032 #include <cmath> 00033 00034 #if defined(SFML_SYSTEM_WINDOWS) 00035 00036 #include <SFML/Window/Win32/WindowImplWin32.hpp> 00037 typedef sf::priv::WindowImplWin32 WindowImplType; 00038 00039 #elif defined(SFML_SYSTEM_LINUX) || defined(SFML_SYSTEM_FREEBSD) 00040 00041 #include <SFML/Window/Linux/WindowImplX11.hpp> 00042 typedef sf::priv::WindowImplX11 WindowImplType; 00043 00044 #elif defined(SFML_SYSTEM_MACOS) 00045 00046 #include <SFML/Window/Cocoa/WindowImplCocoa.hpp> 00047 typedef sf::priv::WindowImplCocoa WindowImplType; 00048 00049 #endif 00050 00051 00052 namespace sf 00053 { 00054 namespace priv 00055 { 00059 WindowImpl* WindowImpl::New() 00060 { 00061 return new WindowImplType(); 00062 } 00063 00064 00068 WindowImpl* WindowImpl::New(VideoMode Mode, const std::string& Title, unsigned long WindowStyle, WindowSettings& Params) 00069 { 00070 return new WindowImplType(Mode, Title, WindowStyle, Params); 00071 } 00072 00073 00077 WindowImpl* WindowImpl::New(WindowHandle Handle, WindowSettings& Params) 00078 { 00079 return new WindowImplType(Handle, Params); 00080 } 00081 00082 00086 WindowImpl::WindowImpl() : 00087 myWidth (0), 00088 myHeight (0), 00089 myJoyThreshold(0.1f) 00090 { 00091 } 00092 00093 00097 WindowImpl::~WindowImpl() 00098 { 00099 // Nothing to do 00100 } 00101 00102 00106 void WindowImpl::AddListener(WindowListener* Listener) 00107 { 00108 if (Listener) 00109 myListeners.insert(Listener); 00110 } 00111 00112 00116 void WindowImpl::RemoveListener(WindowListener* Listener) 00117 { 00118 myListeners.erase(Listener); 00119 } 00120 00121 00125 void WindowImpl::Initialize() 00126 { 00127 // Initialize the joysticks 00128 for (unsigned int i = 0; i < Joy::Count; ++i) 00129 { 00130 myJoysticks[i].Initialize(i); 00131 myJoyStates[i] = myJoysticks[i].UpdateState(); 00132 } 00133 } 00134 00135 00139 unsigned int WindowImpl::GetWidth() const 00140 { 00141 return myWidth; 00142 } 00143 00144 00148 unsigned int WindowImpl::GetHeight() const 00149 { 00150 return myHeight; 00151 } 00152 00153 00158 void WindowImpl::SetJoystickThreshold(float Threshold) 00159 { 00160 myJoyThreshold = Threshold; 00161 } 00162 00163 00167 void WindowImpl::DoEvents() 00168 { 00169 // Read the joysticks state and generate the appropriate events 00170 ProcessJoystickEvents(); 00171 00172 // Let the derived class process other events 00173 ProcessEvents(); 00174 } 00175 00176 00180 bool WindowImpl::IsContextActive() 00181 { 00182 return WindowImplType::IsContextActive(); 00183 } 00184 00185 00189 void WindowImpl::SendEvent(const Event& EventToSend) 00190 { 00191 for (std::set<WindowListener*>::iterator i = myListeners.begin(); i != myListeners.end(); ++i) 00192 { 00193 (*i)->OnEvent(EventToSend); 00194 } 00195 } 00196 00197 00203 int WindowImpl::EvaluateConfig(const VideoMode& Mode, const WindowSettings& Settings, int ColorBits, int DepthBits, int StencilBits, int Antialiasing) 00204 { 00205 return abs(static_cast<int>(Mode.BitsPerPixel - ColorBits)) + 00206 abs(static_cast<int>(Settings.DepthBits - DepthBits)) + 00207 abs(static_cast<int>(Settings.StencilBits - StencilBits)) + 00208 abs(static_cast<int>(Settings.AntialiasingLevel - Antialiasing)); 00209 } 00210 00211 00215 void WindowImpl::ProcessJoystickEvents() 00216 { 00217 for (unsigned int i = 0; i < Joy::Count; ++i) 00218 { 00219 // Copy the previous state of the joystick and get the new one 00220 JoystickState PreviousState = myJoyStates[i]; 00221 myJoyStates[i] = myJoysticks[i].UpdateState(); 00222 00223 // Axis 00224 for (unsigned int j = 0; j < Joy::AxisCount; ++j) 00225 { 00226 Joy::Axis Axis = static_cast<Joy::Axis>(j); 00227 if (myJoysticks[i].HasAxis(Axis)) 00228 { 00229 float PrevPos = PreviousState.Axis[j]; 00230 float CurrPos = myJoyStates[i].Axis[j]; 00231 if (fabs(CurrPos - PrevPos) >= myJoyThreshold) 00232 { 00233 Event Event; 00234 Event.Type = Event::JoyMoved; 00235 Event.JoyMove.JoystickId = i; 00236 Event.JoyMove.Axis = Axis; 00237 Event.JoyMove.Position = CurrPos; 00238 SendEvent(Event); 00239 } 00240 } 00241 } 00242 00243 // Buttons 00244 for (unsigned int j = 0; j < myJoysticks[i].GetButtonsCount(); ++j) 00245 { 00246 bool PrevPressed = PreviousState.Buttons[j]; 00247 bool CurrPressed = myJoyStates[i].Buttons[j]; 00248 00249 if ((!PrevPressed && CurrPressed) || (PrevPressed && !CurrPressed)) 00250 { 00251 Event Event; 00252 Event.Type = CurrPressed ? Event::JoyButtonPressed : Event::JoyButtonReleased; 00253 Event.JoyButton.JoystickId = i; 00254 Event.JoyButton.Button = j; 00255 SendEvent(Event); 00256 } 00257 } 00258 } 00259 } 00260 00261 00262 } // namespace priv 00263 00264 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::