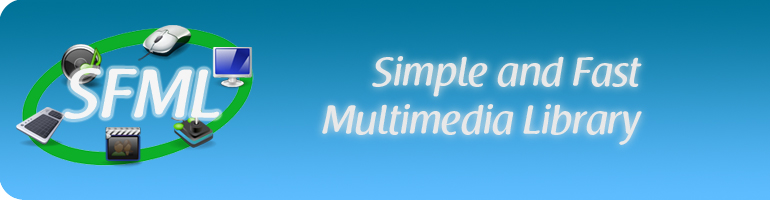
WindowImplXXX.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Window/XXX/WindowImplXXX.hpp> 00029 #include <SFML/Window/WindowStyle.hpp> 00030 #include <GL/gl.h> 00031 00032 00033 namespace sf 00034 { 00035 namespace priv 00036 { 00041 WindowImplXXX::WindowImplXXX() 00042 { 00043 // Create a dummy window (with the fewest attributes -- it's just to have a valid support for an OpenGL context) 00044 00045 // Initialize myWidth and myHeight members from base class with the window size 00046 00047 // Create an OpenGL context in this window and DO NOT make it active 00048 } 00049 00050 00054 WindowImplXXX::WindowImplXXX(WindowHandle Handle, WindowSettings& Params) 00055 { 00056 // Make sure we'll be able to catch all the events of the given window 00057 00058 // Initialize myWidth and myHeight members from base class with the window size 00059 00060 // Create an OpenGL context in this window and make it active 00061 } 00062 00063 00067 WindowImplXXX::WindowImplXXX(VideoMode Mode, const std::string& Title, unsigned long WindowStyle, WindowSettings& Params) : 00068 { 00069 // Create a new window with given size, title and style 00070 00071 // Initialize myWidth and myHeight members from base class with the window size 00072 00073 // Create an OpenGL context in this window and make it active 00074 } 00075 00076 00080 WindowImplXXX::~WindowImplXXX() 00081 { 00082 // Destroy the OpenGL context, the window and every resource allocated by this class 00083 } 00084 00085 00089 bool WindowImplXXX::IsContextActive() 00090 { 00091 // Should return whether xxxGetCurrentContext() is NULL or not; 00092 } 00093 00094 00098 void WindowImplXXX::Display() 00099 { 00100 // Swap OpenGL buffers (should be a call to xxxSwapBuffers) 00101 } 00102 00103 00107 void WindowImplXXX::ProcessEvents() 00108 { 00109 // Process every event for this window 00110 00111 // Generate a sf::Event and call SendEvent(Evt) for each event 00112 } 00113 00114 00118 void WindowImplXXX::SetActive(bool Active) const 00119 { 00120 // Bind / unbind OpenGL context (should be a call to xxxMakeCurrent) 00121 } 00122 00123 00127 void WindowImplXXX::UseVerticalSync(bool Enabled) 00128 { 00129 // Activate / deactivate vertical synchronization 00130 // usually using an OpenGL extension (should be a call to xxxSwapInterval) 00131 } 00132 00133 00137 void WindowImplXXX::ShowMouseCursor(bool Show) 00138 { 00139 // Show or hide the system cursor in this window 00140 } 00141 00142 00146 void WindowImplXXX::SetCursorPosition(unsigned int Left, unsigned int Top) 00147 { 00148 // Change the cursor position (Left and Top are relative to this window) 00149 } 00150 00151 00155 void WindowImplXXX::SetPosition(int Left, int Top) 00156 { 00157 // Change the window position 00158 } 00159 00160 00164 void WindowImplWin32::SetSize(unsigned int Width, unsigned int Height) 00165 { 00166 // Change the window size 00167 } 00168 00169 00173 void WindowImplXXX::Show(bool State) 00174 { 00175 // Show or hide the window 00176 } 00177 00181 void WindowImplXXX::EnableKeyRepeat(bool Enabled) 00182 { 00183 // Enable or disable automatic key-repeat for keydown events 00184 } 00185 00186 00190 void WindowImplXXX::SetIcon(unsigned int Width, unsigned int Height, const Uint8* Pixels) 00191 { 00192 // Change all the necessary icons of the window (titlebar, task bar, ...) with the 00193 // provided array of 32 bits RGBA pixels 00194 } 00195 00196 00197 /*=========================================================== 00198 STRATEGY FOR OPENGL CONTEXT CREATION 00199 00200 - If the requested level of anti-aliasing is not supported and is greater than 2, try with 2 00201 --> if level 2 fails, disable anti-aliasing 00202 --> it's important not to generate an error if anti-aliasing is not supported 00203 00204 - Use a matching pixel mode, or the best of all available pixel modes if no perfect match ; 00205 You should use the function EvaluateConfig to get a score for a given configuration 00206 00207 - Don't forget to fill Params (see constructors) back with the actual parameters we got from the chosen pixel format 00208 00209 - IMPORTANT : all OpenGL contexts must be shared (usually a call to xxxShareLists) 00210 00211 ===========================================================*/ 00212 00213 00214 /*=========================================================== 00215 STRATEGY FOR EVENT HANDLING 00216 00217 - Process any event matching with the ones in sf::Event::EventType 00218 --> Create a sf::Event, fill the members corresponding to the event type 00219 --> No need to handle joystick events, they are handled by WindowImpl::ProcessJoystickEvents 00220 --> Event::TextEntered must provide UTF-16 characters 00221 (see http://www.unicode.org/Public/PROGRAMS/CVTUTF/ for unicode conversions) 00222 --> Don't forget to process any destroy-like event (ie. when the window is destroyed externally) 00223 00224 - Use SendEvent function from base class to propagate the created events 00225 00226 ===========================================================*/ 00227 00228 00229 } // namespace priv 00230 00231 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::