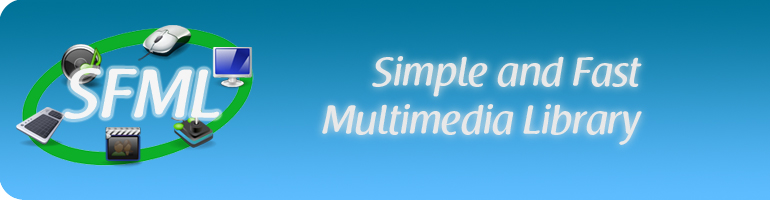
VideoMode.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Window/VideoMode.hpp> 00029 #include <SFML/Window/VideoModeSupport.hpp> 00030 #include <algorithm> 00031 #include <vector> 00032 00033 00037 namespace 00038 { 00039 // Global array of supported video modes 00040 std::vector<sf::VideoMode> SupportedModes; 00041 00042 // Functor for sorting modes from highest to lowest 00043 struct CompareModes 00044 { 00045 bool operator ()(const sf::VideoMode& v1, const sf::VideoMode& v2) const 00046 { 00047 if (v1.BitsPerPixel > v2.BitsPerPixel) 00048 return true; 00049 else if (v1.BitsPerPixel < v2.BitsPerPixel) 00050 return false; 00051 else if (v1.Width > v2.Width) 00052 return true; 00053 else if (v1.Width < v2.Width) 00054 return false; 00055 else 00056 return (v1.Height > v2.Height); 00057 } 00058 }; 00059 } 00060 00061 00062 namespace sf 00063 { 00067 VideoMode::VideoMode() : 00068 Width (0), 00069 Height (0), 00070 BitsPerPixel(0) 00071 { 00072 00073 } 00074 00075 00079 VideoMode::VideoMode(unsigned int ModeWidth, unsigned int ModeHeight, unsigned int ModeBpp) : 00080 Width (ModeWidth), 00081 Height (ModeHeight), 00082 BitsPerPixel(ModeBpp) 00083 { 00084 00085 } 00086 00087 00091 VideoMode VideoMode::GetDesktopMode() 00092 { 00093 // Directly forward to the video mode support 00094 return priv::VideoModeSupport::GetDesktopVideoMode(); 00095 } 00096 00097 00102 VideoMode VideoMode::GetMode(std::size_t Index) 00103 { 00104 if (SupportedModes.empty()) 00105 InitializeModes(); 00106 00107 if (Index < GetModesCount()) 00108 return SupportedModes[Index]; 00109 else 00110 return VideoMode(); 00111 } 00112 00113 00117 std::size_t VideoMode::GetModesCount() 00118 { 00119 if (SupportedModes.empty()) 00120 InitializeModes(); 00121 00122 return SupportedModes.size(); 00123 } 00124 00125 00129 bool VideoMode::IsValid() const 00130 { 00131 if (SupportedModes.empty()) 00132 InitializeModes(); 00133 00134 return std::find(SupportedModes.begin(), SupportedModes.end(), *this) != SupportedModes.end(); 00135 } 00136 00137 00141 bool VideoMode::operator ==(const VideoMode& Other) const 00142 { 00143 return (Width == Other.Width) && 00144 (Height == Other.Height) && 00145 (BitsPerPixel == Other.BitsPerPixel); 00146 } 00147 00148 00152 bool VideoMode::operator !=(const VideoMode& Other) const 00153 { 00154 return !(*this == Other); 00155 } 00156 00157 00161 void VideoMode::InitializeModes() 00162 { 00163 // We request the array of valid modes 00164 priv::VideoModeSupport::GetSupportedVideoModes(SupportedModes); 00165 00166 // And we sort them from highest to lowest (so that number 0 is the best) 00167 std::sort(SupportedModes.begin(), SupportedModes.end(), CompareModes()); 00168 } 00169 00170 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::