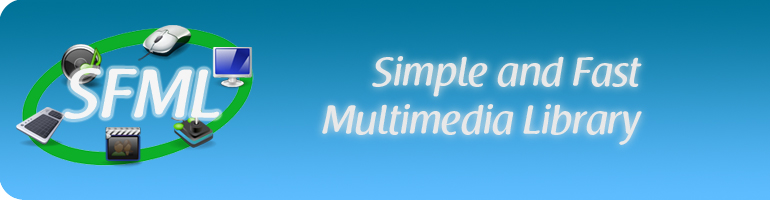
Unicode.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_UNICODE_HPP 00026 #define SFML_UNICODE_HPP 00027 00029 // Headers 00031 #include <SFML/Config.hpp> 00032 #include <iterator> 00033 #include <locale> 00034 #include <string> 00035 #include <stdlib.h> 00036 00037 00038 namespace sf 00039 { 00044 class SFML_API Unicode 00045 { 00046 public : 00047 00054 typedef std::basic_string<Uint8> UTF8String; 00055 typedef std::basic_string<Uint16> UTF16String; 00056 typedef std::basic_string<Uint32> UTF32String; 00057 00063 class SFML_API Text 00064 { 00065 public : 00066 00071 Text(); 00072 00079 Text(const char* Str); 00080 Text(const wchar_t* Str); 00081 Text(const Uint8* Str); 00082 Text(const Uint16* Str); 00083 Text(const Uint32* Str); 00084 Text(const std::string& Str); 00085 Text(const std::wstring& Str); 00086 Text(const Unicode::UTF8String& Str); 00087 Text(const Unicode::UTF16String& Str); 00088 Text(const Unicode::UTF32String& Str); 00089 00096 operator std::string () const; 00097 operator std::wstring () const; 00098 operator Unicode::UTF8String () const; 00099 operator Unicode::UTF16String () const; 00100 operator const Unicode::UTF32String&() const; 00101 00102 private : 00103 00105 // Data member 00107 sf::Unicode::UTF32String myUTF32String; 00108 }; 00109 00123 template <typename In, typename Out> 00124 static Out UTF32ToANSI(In Begin, In End, Out Output, char Replacement = '?', const std::locale& Locale = GetDefaultLocale()); 00125 00138 template <typename In, typename Out> 00139 static Out ANSIToUTF32(In Begin, In End, Out Output, const std::locale& Locale = GetDefaultLocale()); 00140 00153 template <typename In, typename Out> 00154 static Out UTF8ToUTF16(In Begin, In End, Out Output, Uint16 Replacement = '?'); 00155 00168 template <typename In, typename Out> 00169 static Out UTF8ToUTF32(In Begin, In End, Out Output, Uint32 Replacement = '?'); 00170 00183 template <typename In, typename Out> 00184 static Out UTF16ToUTF8(In Begin, In End, Out Output, Uint8 Replacement = '?'); 00185 00198 template <typename In, typename Out> 00199 static Out UTF16ToUTF32(In Begin, In End, Out Output, Uint32 Replacement = '?'); 00200 00213 template <typename In, typename Out> 00214 static Out UTF32ToUTF8(In Begin, In End, Out Output, Uint8 Replacement = '?'); 00215 00228 template <typename In, typename Out> 00229 static Out UTF32ToUTF16(In Begin, In End, Out Output, Uint16 Replacement = '?'); 00230 00240 template <typename In> 00241 static std::size_t GetUTF8Length(In Begin, In End); 00242 00252 template <typename In> 00253 static std::size_t GetUTF16Length(In Begin, In End); 00254 00264 template <typename In> 00265 static std::size_t GetUTF32Length(In Begin, In End); 00266 00267 private : 00268 00275 static const std::locale& GetDefaultLocale(); 00276 00278 // Static member data 00280 static const int UTF8TrailingBytes[256]; 00281 static const Uint32 UTF8Offsets[6]; 00282 static const Uint8 UTF8FirstBytes[7]; 00283 }; 00284 00285 #include <SFML/System/Unicode.inl> 00286 00287 } // namespace sf 00288 00289 00290 #endif // SFML_UNICODE_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::