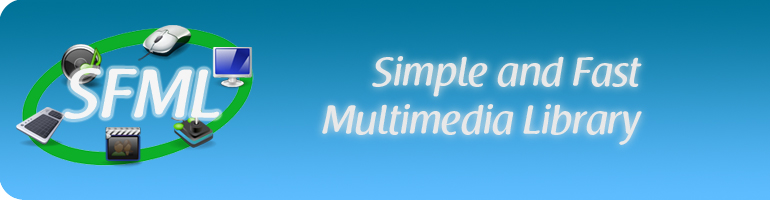
SoundFileOgg.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Audio/SoundFileOgg.hpp> 00029 #include <SFML/Audio/stb_vorbis/stb_vorbis.h> 00030 #include <iostream> 00031 00032 00033 namespace sf 00034 { 00035 namespace priv 00036 { 00040 SoundFileOgg::SoundFileOgg() : 00041 myStream (NULL), 00042 myChannelsCount(0) 00043 { 00044 00045 } 00046 00047 00051 SoundFileOgg::~SoundFileOgg() 00052 { 00053 if (myStream) 00054 stb_vorbis_close(myStream); 00055 } 00056 00057 00061 bool SoundFileOgg::IsFileSupported(const std::string& Filename, bool Read) 00062 { 00063 if (Read) 00064 { 00065 // Open the vorbis stream 00066 stb_vorbis* Stream = stb_vorbis_open_filename(const_cast<char*>(Filename.c_str()), NULL, NULL); 00067 00068 if (Stream) 00069 { 00070 stb_vorbis_close(Stream); 00071 return true; 00072 } 00073 else 00074 { 00075 return false; 00076 } 00077 } 00078 else 00079 { 00080 // No support for writing ogg files yet... 00081 return false; 00082 } 00083 } 00084 00085 00089 bool SoundFileOgg::IsFileSupported(const char* Data, std::size_t SizeInBytes) 00090 { 00091 // Open the vorbis stream 00092 unsigned char* Buffer = reinterpret_cast<unsigned char*>(const_cast<char*>(Data)); 00093 int Length = static_cast<int>(SizeInBytes); 00094 stb_vorbis* Stream = stb_vorbis_open_memory(Buffer, Length, NULL, NULL); 00095 00096 if (Stream) 00097 { 00098 stb_vorbis_close(Stream); 00099 return true; 00100 } 00101 else 00102 { 00103 return false; 00104 } 00105 } 00106 00107 00111 bool SoundFileOgg::OpenRead(const std::string& Filename, std::size_t& NbSamples, unsigned int& ChannelsCount, unsigned int& SampleRate) 00112 { 00113 // Close the file if already opened 00114 if (myStream) 00115 stb_vorbis_close(myStream); 00116 00117 // Open the vorbis stream 00118 myStream = stb_vorbis_open_filename(const_cast<char*>(Filename.c_str()), NULL, NULL); 00119 if (myStream == NULL) 00120 { 00121 std::cerr << "Failed to read sound file \"" << Filename << "\" (cannot open the file)" << std::endl; 00122 return false; 00123 } 00124 00125 // Get the music parameters 00126 stb_vorbis_info Infos = stb_vorbis_get_info(myStream); 00127 ChannelsCount = myChannelsCount = Infos.channels; 00128 SampleRate = Infos.sample_rate; 00129 NbSamples = static_cast<std::size_t>(stb_vorbis_stream_length_in_samples(myStream) * ChannelsCount); 00130 00131 return true; 00132 } 00133 00134 00138 bool SoundFileOgg::OpenRead(const char* Data, std::size_t SizeInBytes, std::size_t& NbSamples, unsigned int& ChannelsCount, unsigned int& SampleRate) 00139 { 00140 // Close the file if already opened 00141 if (myStream) 00142 stb_vorbis_close(myStream); 00143 00144 // Open the vorbis stream 00145 unsigned char* Buffer = reinterpret_cast<unsigned char*>(const_cast<char*>(Data)); 00146 int Length = static_cast<int>(SizeInBytes); 00147 myStream = stb_vorbis_open_memory(Buffer, Length, NULL, NULL); 00148 if (myStream == NULL) 00149 { 00150 std::cerr << "Failed to read sound file from memory (cannot open the file)" << std::endl; 00151 return false; 00152 } 00153 00154 // Get the music parameters 00155 stb_vorbis_info Infos = stb_vorbis_get_info(myStream); 00156 ChannelsCount = myChannelsCount = Infos.channels; 00157 SampleRate = Infos.sample_rate; 00158 NbSamples = static_cast<std::size_t>(stb_vorbis_stream_length_in_samples(myStream) * ChannelsCount); 00159 00160 return true; 00161 } 00162 00163 00167 std::size_t SoundFileOgg::Read(Int16* Data, std::size_t NbSamples) 00168 { 00169 if (myStream && Data && NbSamples) 00170 { 00171 int Read = stb_vorbis_get_samples_short_interleaved(myStream, myChannelsCount, Data, static_cast<int>(NbSamples)); 00172 return static_cast<std::size_t>(Read * myChannelsCount); 00173 } 00174 else 00175 { 00176 return 0; 00177 } 00178 } 00179 00180 } // namespace priv 00181 00182 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::