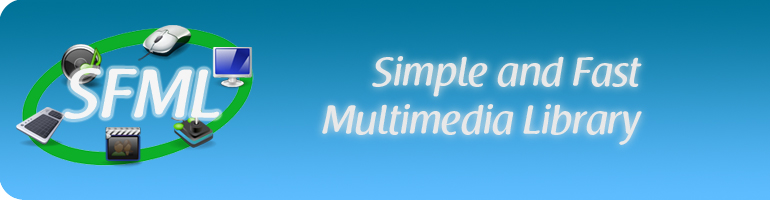
SelectorBase.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifdef _MSC_VER 00026 #pragma warning(disable : 4127) // "conditional expression is constant" generated by the FD_SET macro 00027 #endif 00028 00030 // Headers 00032 #include <SFML/Network/SelectorBase.hpp> 00033 00034 00035 namespace sf 00036 { 00040 SelectorBase::SelectorBase() : 00041 myMaxSocket(0) 00042 { 00043 Clear(); 00044 } 00045 00046 00050 void SelectorBase::Add(SocketHelper::SocketType Socket) 00051 { 00052 FD_SET(Socket, &mySet); 00053 00054 int Size = static_cast<int>(Socket); 00055 if (Size > myMaxSocket) 00056 myMaxSocket = Size; 00057 } 00058 00059 00063 void SelectorBase::Remove(SocketHelper::SocketType Socket) 00064 { 00065 FD_CLR(Socket, &mySet); 00066 } 00067 00068 00072 void SelectorBase::Clear() 00073 { 00074 FD_ZERO(&mySet); 00075 FD_ZERO(&mySetReady); 00076 00077 myMaxSocket = 0; 00078 } 00079 00080 00086 unsigned int SelectorBase::Wait(float Timeout) 00087 { 00088 // Setup the timeout structure 00089 timeval Time; 00090 Time.tv_sec = static_cast<long>(Timeout); 00091 Time.tv_usec = (static_cast<long>(Timeout * 1000) % 1000) * 1000; 00092 00093 // Prepare the set of sockets to return 00094 mySetReady = mySet; 00095 00096 // Wait until one of the sockets is ready for reading, or timeout is reached 00097 int NbSockets = select(myMaxSocket + 1, &mySetReady, NULL, NULL, Timeout > 0 ? &Time : NULL); 00098 00099 return NbSockets >= 0 ? static_cast<unsigned int>(NbSockets) : 0; 00100 } 00101 00102 00108 SocketHelper::SocketType SelectorBase::GetSocketReady(unsigned int Index) 00109 { 00110 // The standard FD_xxx interface doesn't define a direct access, 00111 // so we must go through the whole set to find the socket we're looking for 00112 for (int i = 0; i < myMaxSocket + 1; ++i) 00113 { 00114 if (FD_ISSET(i, &mySetReady)) 00115 { 00116 // Current socket is ready, but is it the Index-th one ? 00117 if (Index > 0) 00118 { 00119 Index--; 00120 } 00121 else 00122 { 00123 return static_cast<SocketHelper::SocketType>(i); 00124 } 00125 } 00126 } 00127 00128 // Invalid index : return an invalid socket 00129 return SocketHelper::InvalidSocket(); 00130 } 00131 00132 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::