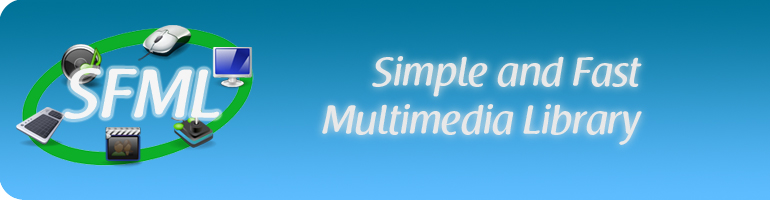
Packet.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_PACKET_HPP 00026 #define SFML_PACKET_HPP 00027 00029 // Headers 00031 #include <SFML/Config.hpp> 00032 #include <string> 00033 #include <vector> 00034 00035 00036 namespace sf 00037 { 00041 class SFML_API Packet 00042 { 00043 public : 00044 00049 Packet(); 00050 00055 virtual ~Packet(); 00056 00064 void Append(const void* Data, std::size_t SizeInBytes); 00065 00070 void Clear(); 00071 00080 const char* GetData() const; 00081 00088 std::size_t GetDataSize() const; 00089 00096 bool EndOfPacket() const; 00097 00104 operator bool() const; 00105 00110 Packet& operator >>(bool& Data); 00111 Packet& operator >>(Int8& Data); 00112 Packet& operator >>(Uint8& Data); 00113 Packet& operator >>(Int16& Data); 00114 Packet& operator >>(Uint16& Data); 00115 Packet& operator >>(Int32& Data); 00116 Packet& operator >>(Uint32& Data); 00117 Packet& operator >>(float& Data); 00118 Packet& operator >>(double& Data); 00119 Packet& operator >>(char* Data); 00120 Packet& operator >>(std::string& Data); 00121 Packet& operator >>(wchar_t* Data); 00122 Packet& operator >>(std::wstring& Data); 00123 00128 Packet& operator <<(bool Data); 00129 Packet& operator <<(Int8 Data); 00130 Packet& operator <<(Uint8 Data); 00131 Packet& operator <<(Int16 Data); 00132 Packet& operator <<(Uint16 Data); 00133 Packet& operator <<(Int32 Data); 00134 Packet& operator <<(Uint32 Data); 00135 Packet& operator <<(float Data); 00136 Packet& operator <<(double Data); 00137 Packet& operator <<(const char* Data); 00138 Packet& operator <<(const std::string& Data); 00139 Packet& operator <<(const wchar_t* Data); 00140 Packet& operator <<(const std::wstring& Data); 00141 00142 private : 00143 00144 friend class SocketTCP; 00145 friend class SocketUDP; 00146 00155 bool CheckSize(std::size_t Size); 00156 00165 virtual const char* OnSend(std::size_t& DataSize); 00166 00174 virtual void OnReceive(const char* Data, std::size_t DataSize); 00175 00177 // Member data 00179 std::vector<char> myData; 00180 std::size_t myReadPos; 00181 bool myIsValid; 00182 }; 00183 00184 } // namespace sf 00185 00186 00187 #endif // SFML_PACKET_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::