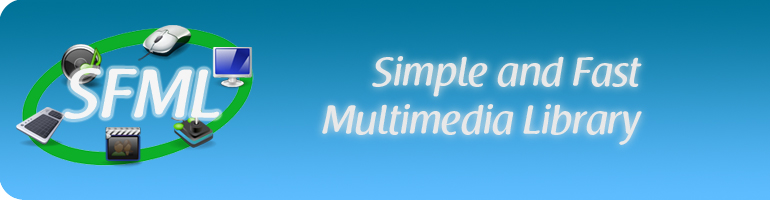
Packet.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Network/Packet.hpp> 00029 #include <SFML/Network/SocketHelper.hpp> 00030 #include <string.h> 00031 00032 00033 namespace sf 00034 { 00038 Packet::Packet() : 00039 myReadPos(0), 00040 myIsValid(true) 00041 { 00042 00043 } 00044 00045 00049 Packet::~Packet() 00050 { 00051 00052 } 00053 00054 00058 void Packet::Append(const void* Data, std::size_t SizeInBytes) 00059 { 00060 if (Data && (SizeInBytes > 0)) 00061 { 00062 std::size_t Start = myData.size(); 00063 myData.resize(Start + SizeInBytes); 00064 memcpy(&myData[Start], Data, SizeInBytes); 00065 } 00066 } 00067 00068 00072 void Packet::Clear() 00073 { 00074 myData.clear(); 00075 myReadPos = 0; 00076 myIsValid = true; 00077 } 00078 00079 00085 const char* Packet::GetData() const 00086 { 00087 return !myData.empty() ? &myData[0] : NULL; 00088 } 00089 00090 00094 std::size_t Packet::GetDataSize() const 00095 { 00096 return myData.size(); 00097 } 00098 00099 00103 bool Packet::EndOfPacket() const 00104 { 00105 return myReadPos >= myData.size(); 00106 } 00107 00108 00112 Packet::operator bool() const 00113 { 00114 return myIsValid; 00115 } 00116 00117 00121 Packet& Packet::operator >>(bool& Data) 00122 { 00123 Uint8 Value; 00124 if (*this >> Value) 00125 Data = (Value != 0); 00126 00127 return *this; 00128 } 00129 Packet& Packet::operator >>(Int8& Data) 00130 { 00131 if (CheckSize(sizeof(Data))) 00132 { 00133 Data = *reinterpret_cast<const Int8*>(GetData() + myReadPos); 00134 myReadPos += sizeof(Data); 00135 } 00136 00137 return *this; 00138 } 00139 Packet& Packet::operator >>(Uint8& Data) 00140 { 00141 if (CheckSize(sizeof(Data))) 00142 { 00143 Data = *reinterpret_cast<const Uint8*>(GetData() + myReadPos); 00144 myReadPos += sizeof(Data); 00145 } 00146 00147 return *this; 00148 } 00149 Packet& Packet::operator >>(Int16& Data) 00150 { 00151 if (CheckSize(sizeof(Data))) 00152 { 00153 Data = ntohs(*reinterpret_cast<const Int16*>(GetData() + myReadPos)); 00154 myReadPos += sizeof(Data); 00155 } 00156 00157 return *this; 00158 } 00159 Packet& Packet::operator >>(Uint16& Data) 00160 { 00161 if (CheckSize(sizeof(Data))) 00162 { 00163 Data = ntohs(*reinterpret_cast<const Uint16*>(GetData() + myReadPos)); 00164 myReadPos += sizeof(Data); 00165 } 00166 00167 return *this; 00168 } 00169 Packet& Packet::operator >>(Int32& Data) 00170 { 00171 if (CheckSize(sizeof(Data))) 00172 { 00173 Data = ntohl(*reinterpret_cast<const Int32*>(GetData() + myReadPos)); 00174 myReadPos += sizeof(Data); 00175 } 00176 00177 return *this; 00178 } 00179 Packet& Packet::operator >>(Uint32& Data) 00180 { 00181 if (CheckSize(sizeof(Data))) 00182 { 00183 Data = ntohl(*reinterpret_cast<const Uint32*>(GetData() + myReadPos)); 00184 myReadPos += sizeof(Data); 00185 } 00186 00187 return *this; 00188 } 00189 Packet& Packet::operator >>(float& Data) 00190 { 00191 if (CheckSize(sizeof(Data))) 00192 { 00193 Data = *reinterpret_cast<const float*>(GetData() + myReadPos); 00194 myReadPos += sizeof(Data); 00195 } 00196 00197 return *this; 00198 } 00199 Packet& Packet::operator >>(double& Data) 00200 { 00201 if (CheckSize(sizeof(Data))) 00202 { 00203 Data = *reinterpret_cast<const double*>(GetData() + myReadPos); 00204 myReadPos += sizeof(Data); 00205 } 00206 00207 return *this; 00208 } 00209 Packet& Packet::operator >>(char* Data) 00210 { 00211 // First extract string length 00212 Uint32 Length; 00213 *this >> Length; 00214 00215 if ((Length > 0) && CheckSize(Length)) 00216 { 00217 // Then extract characters 00218 memcpy(Data, GetData() + myReadPos, Length); 00219 Data[Length] = '\0'; 00220 00221 // Update reading position 00222 myReadPos += Length; 00223 } 00224 00225 return *this; 00226 } 00227 Packet& Packet::operator >>(std::string& Data) 00228 { 00229 // First extract string length 00230 Uint32 Length; 00231 *this >> Length; 00232 00233 Data.clear(); 00234 if ((Length > 0) && CheckSize(Length)) 00235 { 00236 // Then extract characters 00237 Data.assign(GetData() + myReadPos, Length); 00238 00239 // Update reading position 00240 myReadPos += Length; 00241 } 00242 00243 return *this; 00244 } 00245 Packet& Packet::operator >>(wchar_t* Data) 00246 { 00247 // First extract string length 00248 Uint32 Length; 00249 *this >> Length; 00250 00251 if ((Length > 0) && CheckSize(Length * sizeof(Int32))) 00252 { 00253 // Then extract characters 00254 for (Uint32 i = 0; i < Length; ++i) 00255 { 00256 Uint32 c; 00257 *this >> c; 00258 Data[i] = static_cast<wchar_t>(c); 00259 } 00260 Data[Length] = L'\0'; 00261 } 00262 00263 return *this; 00264 } 00265 Packet& Packet::operator >>(std::wstring& Data) 00266 { 00267 // First extract string length 00268 Uint32 Length; 00269 *this >> Length; 00270 00271 Data.clear(); 00272 if ((Length > 0) && CheckSize(Length * sizeof(Int32))) 00273 { 00274 // Then extract characters 00275 for (Uint32 i = 0; i < Length; ++i) 00276 { 00277 Uint32 c; 00278 *this >> c; 00279 Data += static_cast<wchar_t>(c); 00280 } 00281 } 00282 00283 return *this; 00284 } 00285 00286 00290 Packet& Packet::operator <<(bool Data) 00291 { 00292 *this << static_cast<Uint8>(Data); 00293 return *this; 00294 } 00295 Packet& Packet::operator <<(Int8 Data) 00296 { 00297 Append(&Data, sizeof(Data)); 00298 return *this; 00299 } 00300 Packet& Packet::operator <<(Uint8 Data) 00301 { 00302 Append(&Data, sizeof(Data)); 00303 return *this; 00304 } 00305 Packet& Packet::operator <<(Int16 Data) 00306 { 00307 Int16 ToWrite = htons(Data); 00308 Append(&ToWrite, sizeof(ToWrite)); 00309 return *this; 00310 } 00311 Packet& Packet::operator <<(Uint16 Data) 00312 { 00313 Uint16 ToWrite = htons(Data); 00314 Append(&ToWrite, sizeof(ToWrite)); 00315 return *this; 00316 } 00317 Packet& Packet::operator <<(Int32 Data) 00318 { 00319 Int32 ToWrite = htonl(Data); 00320 Append(&ToWrite, sizeof(ToWrite)); 00321 return *this; 00322 } 00323 Packet& Packet::operator <<(Uint32 Data) 00324 { 00325 Uint32 ToWrite = htonl(Data); 00326 Append(&ToWrite, sizeof(ToWrite)); 00327 return *this; 00328 } 00329 Packet& Packet::operator <<(float Data) 00330 { 00331 Append(&Data, sizeof(Data)); 00332 return *this; 00333 } 00334 Packet& Packet::operator <<(double Data) 00335 { 00336 Append(&Data, sizeof(Data)); 00337 return *this; 00338 } 00339 Packet& Packet::operator <<(const char* Data) 00340 { 00341 // First insert string length 00342 Uint32 Length = 0; 00343 for (const char* c = Data; *c != '\0'; ++c) 00344 ++Length; 00345 *this << Length; 00346 00347 // Then insert characters 00348 Append(Data, Length * sizeof(char)); 00349 00350 return *this; 00351 } 00352 Packet& Packet::operator <<(const std::string& Data) 00353 { 00354 // First insert string length 00355 Uint32 Length = static_cast<Uint32>(Data.size()); 00356 *this << Length; 00357 00358 // Then insert characters 00359 if (Length > 0) 00360 { 00361 Append(Data.c_str(), Length * sizeof(std::string::value_type)); 00362 } 00363 00364 return *this; 00365 } 00366 Packet& Packet::operator <<(const wchar_t* Data) 00367 { 00368 // First insert string length 00369 Uint32 Length = 0; 00370 for (const wchar_t* c = Data; *c != L'\0'; ++c) 00371 ++Length; 00372 *this << Length; 00373 00374 // Then insert characters 00375 for (const wchar_t* c = Data; *c != L'\0'; ++c) 00376 *this << static_cast<Int32>(*c); 00377 00378 return *this; 00379 } 00380 Packet& Packet::operator <<(const std::wstring& Data) 00381 { 00382 // First insert string length 00383 Uint32 Length = static_cast<Uint32>(Data.size()); 00384 *this << Length; 00385 00386 // Then insert characters 00387 if (Length > 0) 00388 { 00389 for (std::wstring::const_iterator c = Data.begin(); c != Data.end(); ++c) 00390 *this << static_cast<Int32>(*c); 00391 } 00392 00393 return *this; 00394 } 00395 00396 00400 bool Packet::CheckSize(std::size_t Size) 00401 { 00402 myIsValid = myIsValid && (myReadPos + Size <= myData.size()); 00403 00404 return myIsValid; 00405 } 00406 00407 00411 const char* Packet::OnSend(std::size_t& DataSize) 00412 { 00413 DataSize = GetDataSize(); 00414 return GetData(); 00415 } 00416 00417 00421 void Packet::OnReceive(const char* Data, std::size_t DataSize) 00422 { 00423 Append(Data, DataSize); 00424 } 00425 00426 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::