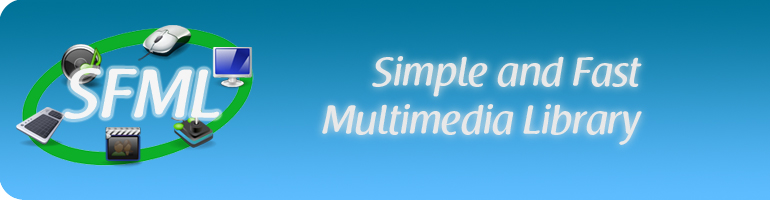
OpenAL.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_OPENAL_HPP 00026 #define SFML_OPENAL_HPP 00027 00029 // Headers 00031 #include <SFML/Config.hpp> 00032 00033 #if defined(SFML_SYSTEM_MACOS) 00034 #include <OpenAL/al.h> 00035 #include <OpenAL/alc.h> 00036 #else 00037 #include <AL/al.h> 00038 #include <AL/alc.h> 00039 #endif 00040 00041 #include <iostream> 00042 #include <string> 00043 00044 00045 namespace sf 00046 { 00047 namespace priv 00048 { 00054 #ifdef SFML_DEBUG 00055 00056 // If in debug mode, perform a test on every call 00057 #define ALCheck(Func) ((Func), priv::ALCheckError(__FILE__, __LINE__)) 00058 00059 #else 00060 00061 // Else, we don't add any overhead 00062 #define ALCheck(Func) (Func) 00063 00064 #endif 00065 00066 00071 inline void ALCheckError(const std::string& File, unsigned int Line) 00072 { 00073 // Get the last error 00074 ALenum ErrorCode = alGetError(); 00075 00076 if (ErrorCode != AL_NO_ERROR) 00077 { 00078 std::string Error, Desc; 00079 00080 // Decode the error code 00081 switch (ErrorCode) 00082 { 00083 case AL_INVALID_NAME : 00084 { 00085 Error = "AL_INVALID_NAME"; 00086 Desc = "an unacceptable name has been specified"; 00087 break; 00088 } 00089 00090 case AL_INVALID_ENUM : 00091 { 00092 Error = "AL_INVALID_ENUM"; 00093 Desc = "an unacceptable value has been specified for an enumerated argument"; 00094 break; 00095 } 00096 00097 case AL_INVALID_VALUE : 00098 { 00099 Error = "AL_INVALID_VALUE"; 00100 Desc = "a numeric argument is out of range"; 00101 break; 00102 } 00103 00104 case AL_INVALID_OPERATION : 00105 { 00106 Error = "AL_INVALID_OPERATION"; 00107 Desc = "the specified operation is not allowed in the current state"; 00108 break; 00109 } 00110 00111 case AL_OUT_OF_MEMORY : 00112 { 00113 Error = "AL_OUT_OF_MEMORY"; 00114 Desc = "there is not enough memory left to execute the command"; 00115 break; 00116 } 00117 } 00118 00119 // Log the error 00120 std::cerr << "An internal OpenAL call failed in " 00121 << File.substr(File.find_last_of("\\/") + 1) << " (" << Line << ") : " 00122 << Error << ", " << Desc 00123 << std::endl; 00124 } 00125 } 00126 00127 } // namespace priv 00128 00129 } // namespace sf 00130 00131 00132 #endif // SFML_OPENAL_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::