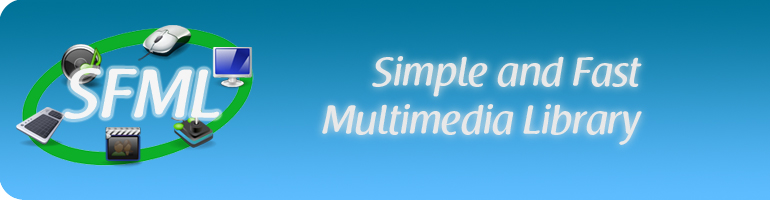
Input.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_INPUT_HPP 00026 #define SFML_INPUT_HPP 00027 00029 // Headers 00031 #include <SFML/Config.hpp> 00032 #include <SFML/System/NonCopyable.hpp> 00033 #include <SFML/Window/Event.hpp> 00034 #include <SFML/Window/WindowListener.hpp> 00035 00036 00037 namespace sf 00038 { 00044 class SFML_API Input : public WindowListener, NonCopyable 00045 { 00046 public : 00047 00052 Input(); 00053 00062 bool IsKeyDown(Key::Code KeyCode) const; 00063 00072 bool IsMouseButtonDown(Mouse::Button Button) const; 00073 00083 bool IsJoystickButtonDown(unsigned int JoyId, unsigned int Button) const; 00084 00091 int GetMouseX() const; 00092 00099 int GetMouseY() const; 00100 00110 float GetJoystickAxis(unsigned int JoyId, Joy::Axis Axis) const; 00111 00112 private : 00113 00118 virtual void OnEvent(const Event& EventReceived); 00119 00124 void ResetStates(); 00125 00127 // Member data 00129 bool myKeys[Key::Count]; 00130 bool myMouseButtons[Mouse::ButtonCount]; 00131 int myMouseX; 00132 int myMouseY; 00133 bool myJoystickButtons[Joy::Count][Joy::ButtonCount]; 00134 float myJoystickAxis[Joy::Count][Joy::AxisCount]; 00135 }; 00136 00137 } // namespace sf 00138 00139 00140 #endif // SFML_INPUT_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::