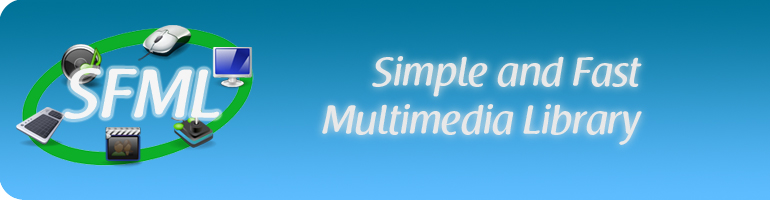
Input.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Window/Input.hpp> 00029 00030 00031 namespace sf 00032 { 00036 Input::Input() : 00037 myMouseX(0), 00038 myMouseY(0) 00039 { 00040 ResetStates(); 00041 } 00042 00043 00047 bool Input::IsKeyDown(Key::Code KeyCode) const 00048 { 00049 return myKeys[KeyCode]; 00050 } 00051 00052 00056 bool Input::IsMouseButtonDown(Mouse::Button Button) const 00057 { 00058 return myMouseButtons[Button]; 00059 } 00060 00061 00065 bool Input::IsJoystickButtonDown(unsigned int JoyId, unsigned int Button) const 00066 { 00067 if ((JoyId < Joy::Count) && (Button < Joy::ButtonCount)) 00068 return myJoystickButtons[JoyId][Button]; 00069 else 00070 return false; 00071 } 00072 00073 00077 int Input::GetMouseX() const 00078 { 00079 return myMouseX; 00080 } 00081 00082 00086 int Input::GetMouseY() const 00087 { 00088 return myMouseY; 00089 } 00090 00091 00095 float Input::GetJoystickAxis(unsigned int JoyId, Joy::Axis Axis) const 00096 { 00097 if (JoyId < Joy::Count) 00098 return myJoystickAxis[JoyId][Axis]; 00099 else 00100 return 0.f; 00101 } 00102 00103 00107 void Input::OnEvent(const Event& EventReceived) 00108 { 00109 switch (EventReceived.Type) 00110 { 00111 // Key events 00112 case Event::KeyPressed : myKeys[EventReceived.Key.Code] = true; break; 00113 case Event::KeyReleased : myKeys[EventReceived.Key.Code] = false; break; 00114 00115 // Mouse event 00116 case Event::MouseButtonPressed : myMouseButtons[EventReceived.MouseButton.Button] = true; break; 00117 case Event::MouseButtonReleased : myMouseButtons[EventReceived.MouseButton.Button] = false; break; 00118 00119 // Mouse move event 00120 case Event::MouseMoved : 00121 myMouseX = EventReceived.MouseMove.X; 00122 myMouseY = EventReceived.MouseMove.Y; 00123 break; 00124 00125 // Joystick button events 00126 case Event::JoyButtonPressed : myJoystickButtons[EventReceived.JoyButton.JoystickId][EventReceived.JoyButton.Button] = true; break; 00127 case Event::JoyButtonReleased : myJoystickButtons[EventReceived.JoyButton.JoystickId][EventReceived.JoyButton.Button] = false; break; 00128 00129 // Joystick move event 00130 case Event::JoyMoved : 00131 myJoystickAxis[EventReceived.JoyMove.JoystickId][EventReceived.JoyMove.Axis] = EventReceived.JoyMove.Position; 00132 break; 00133 00134 // Lost focus event : we must reset all persistent states 00135 case Event::LostFocus : 00136 { 00137 ResetStates(); 00138 break; 00139 } 00140 00141 default : 00142 break; 00143 } 00144 } 00145 00146 00150 void Input::ResetStates() 00151 { 00152 for (int i = 0; i < Key::Count; ++i) 00153 myKeys[i] = false; 00154 00155 for (int i = 0; i < Mouse::ButtonCount; ++i) 00156 myMouseButtons[i] = false; 00157 00158 for (int i = 0; i < Joy::Count; ++i) 00159 { 00160 for (int j = 0; j < Joy::ButtonCount; ++j) 00161 myJoystickButtons[i][j] = false; 00162 00163 for (int j = 0; j < Joy::AxisCount; ++j) 00164 myJoystickAxis[i][j] = 0.f; 00165 myJoystickAxis[i][Joy::AxisPOV] = -1.f; 00166 } 00167 } 00168 00169 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::