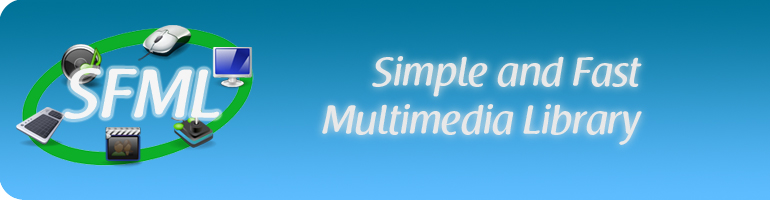
Http.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_HTTP_HPP 00026 #define SFML_HTTP_HPP 00027 00029 // Headers 00031 #include <SFML/System/NonCopyable.hpp> 00032 #include <SFML/Network/IPAddress.hpp> 00033 #include <SFML/Network/SocketTCP.hpp> 00034 #include <map> 00035 #include <string> 00036 00037 00038 namespace sf 00039 { 00045 class SFML_API Http : NonCopyable 00046 { 00047 public : 00048 00054 class SFML_API Request 00055 { 00056 public : 00057 00061 enum Method 00062 { 00063 Get, 00064 Post, 00065 Head 00066 }; 00067 00076 Request(Method RequestMethod = Get, const std::string& URI = "/", const std::string& Body = ""); 00077 00085 void SetField(const std::string& Field, const std::string& Value); 00086 00094 void SetMethod(Method RequestMethod); 00095 00103 void SetURI(const std::string& URI); 00104 00113 void SetHttpVersion(unsigned int Major, unsigned int Minor); 00114 00123 void SetBody(const std::string& Body); 00124 00125 private : 00126 00127 friend class Http; 00128 00135 std::string ToString() const; 00136 00145 bool HasField(const std::string& Field) const; 00146 00148 // Types 00150 typedef std::map<std::string, std::string> FieldTable; 00151 00153 // Member data 00155 FieldTable myFields; 00156 Method myMethod; 00157 std::string myURI; 00158 unsigned int myMajorVersion; 00159 unsigned int myMinorVersion; 00160 std::string myBody; 00161 }; 00162 00168 class SFML_API Response 00169 { 00170 public : 00171 00176 enum Status 00177 { 00178 // 2xx: success 00179 Ok = 200, 00180 Created = 201, 00181 Accepted = 202, 00182 NoContent = 204, 00183 00184 // 3xx: redirection 00185 MultipleChoices = 300, 00186 MovedPermanently = 301, 00187 MovedTemporarily = 302, 00188 NotModified = 304, 00189 00190 // 4xx: client error 00191 BadRequest = 400, 00192 Unauthorized = 401, 00193 Forbidden = 403, 00194 NotFound = 404, 00195 00196 // 5xx: server error 00197 InternalServerError = 500, 00198 NotImplemented = 501, 00199 BadGateway = 502, 00200 ServiceNotAvailable = 503, 00201 00202 // 10xx: SFML custom codes 00203 InvalidResponse = 1000, 00204 ConnectionFailed = 1001 00205 }; 00206 00211 Response(); 00212 00221 const std::string& GetField(const std::string& Field) const; 00222 00229 Status GetStatus() const; 00230 00237 unsigned int GetMajorHttpVersion() const; 00238 00245 unsigned int GetMinorHttpVersion() const; 00246 00257 const std::string& GetBody() const; 00258 00259 private : 00260 00261 friend class Http; 00262 00269 void FromString(const std::string& Data); 00270 00272 // Types 00274 typedef std::map<std::string, std::string> FieldTable; 00275 00277 // Member data 00279 FieldTable myFields; 00280 Status myStatus; 00281 unsigned int myMajorVersion; 00282 unsigned int myMinorVersion; 00283 std::string myBody; 00284 }; 00285 00290 Http(); 00291 00299 Http(const std::string& Host, unsigned short Port = 0); 00300 00308 void SetHost(const std::string& Host, unsigned short Port = 0); 00309 00324 Response SendRequest(const Request& Req, float Timeout = 0.f); 00325 00326 private : 00327 00329 // Member data 00331 SocketTCP myConnection; 00332 IPAddress myHost; 00333 std::string myHostName; 00334 unsigned short myPort; 00335 }; 00336 00337 } // namespace sf 00338 00339 00340 #endif // SFML_HTTP_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::