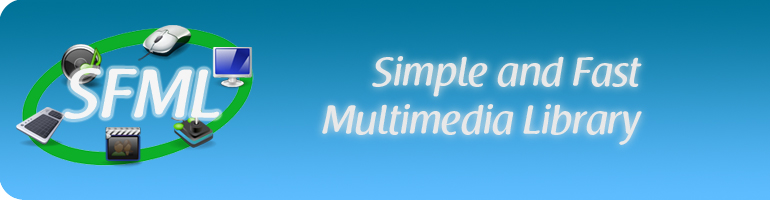
Drawable.hpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00025 #ifndef SFML_DRAWABLE_HPP 00026 #define SFML_DRAWABLE_HPP 00027 00029 // Headers 00031 #include <SFML/System/Vector2.hpp> 00032 #include <SFML/Graphics/Color.hpp> 00033 #include <SFML/Graphics/Matrix3.hpp> 00034 00035 00036 namespace sf 00037 { 00038 class RenderTarget; 00039 00043 namespace Blend 00044 { 00045 enum Mode 00046 { 00047 Alpha, 00048 Add, 00049 Multiply, 00050 None 00051 }; 00052 } 00053 00058 class SFML_API Drawable 00059 { 00060 public : 00061 00071 Drawable(const Vector2f& Position = Vector2f(0, 0), const Vector2f& Scale = Vector2f(1, 1), float Rotation = 0.f, const Color& Col = Color(255, 255, 255, 255)); 00072 00077 virtual ~Drawable(); 00078 00086 void SetPosition(float X, float Y); 00087 00094 void SetPosition(const Vector2f& Position); 00095 00102 void SetX(float X); 00103 00110 void SetY(float Y); 00111 00119 void SetScale(float ScaleX, float ScaleY); 00120 00127 void SetScale(const Vector2f& Scale); 00128 00135 void SetScaleX(float FactorX); 00136 00143 void SetScaleY(float FactorY); 00144 00154 void SetCenter(float CenterX, float CenterY); 00155 00164 void SetCenter(const Vector2f& Center); 00165 00172 void SetRotation(float Rotation); 00173 00181 void SetColor(const Color& Col); 00182 00190 void SetBlendMode(Blend::Mode Mode); 00191 00198 const Vector2f& GetPosition() const; 00199 00206 const Vector2f& GetScale() const; 00207 00214 const Vector2f& GetCenter() const; 00215 00223 float GetRotation() const; 00224 00231 const Color& GetColor() const; 00232 00239 Blend::Mode GetBlendMode() const; 00240 00248 void Move(float OffsetX, float OffsetY); 00249 00256 void Move(const Vector2f& Offset); 00257 00265 void Scale(float FactorX, float FactorY); 00266 00273 void Scale(const Vector2f& Factor); 00274 00281 void Rotate(float Angle); 00282 00292 sf::Vector2f TransformToLocal(const sf::Vector2f& Point) const; 00293 00303 sf::Vector2f TransformToGlobal(const sf::Vector2f& Point) const; 00304 00305 protected : 00306 00313 const Matrix3& GetMatrix() const; 00314 00321 const Matrix3& GetInverseMatrix() const; 00322 00323 private : 00324 00325 friend class RenderTarget; 00326 00333 void Draw(RenderTarget& Target) const; 00334 00341 virtual void Render(RenderTarget& Target) const = 0; 00342 00344 // Member data 00346 Vector2f myPosition; 00347 Vector2f myScale; 00348 Vector2f myCenter; 00349 float myRotation; 00350 Color myColor; 00351 Blend::Mode myBlendMode; 00352 mutable bool myNeedUpdate; 00353 mutable bool myInvNeedUpdate; 00354 mutable Matrix3 myMatrix; 00355 mutable Matrix3 myInvMatrix; 00356 }; 00357 00358 } // namespace sf 00359 00360 00361 #endif // SFML_DRAWABLE_HPP
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::