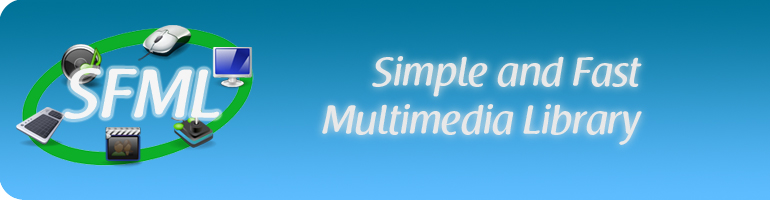
Color.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Graphics/Color.hpp> 00029 #include <algorithm> 00030 00031 00032 namespace sf 00033 { 00035 // Static member data 00037 const Color Color::Black(0, 0, 0); 00038 const Color Color::White(255, 255, 255); 00039 const Color Color::Red(255, 0, 0); 00040 const Color Color::Green(0, 255, 0); 00041 const Color Color::Blue(0, 0, 255); 00042 const Color Color::Yellow(255, 255, 0); 00043 const Color Color::Magenta(255, 0, 255); 00044 const Color Color::Cyan(0, 255, 255); 00045 00046 00050 Color::Color() : 00051 r(0), 00052 g(0), 00053 b(0), 00054 a(255) 00055 { 00056 00057 } 00058 00059 00063 Color::Color(Uint8 R, Uint8 G, Uint8 B, Uint8 A) : 00064 r(R), 00065 g(G), 00066 b(B), 00067 a(A) 00068 { 00069 00070 } 00071 00072 00076 Color& Color::operator +=(const Color& Other) 00077 { 00078 r = static_cast<Uint8>(std::min(r + Other.r, 255)); 00079 g = static_cast<Uint8>(std::min(g + Other.g, 255)); 00080 b = static_cast<Uint8>(std::min(b + Other.b, 255)); 00081 a = static_cast<Uint8>(std::min(a + Other.a, 255)); 00082 00083 return *this; 00084 } 00085 00086 00090 Color& Color::operator *=(const Color& Other) 00091 { 00092 r = static_cast<Uint8>(r * Other.r / 255); 00093 g = static_cast<Uint8>(g * Other.g / 255); 00094 b = static_cast<Uint8>(b * Other.b / 255); 00095 a = static_cast<Uint8>(a * Other.a / 255); 00096 00097 return *this; 00098 } 00099 00100 00104 bool Color::operator ==(const Color& Other) const 00105 { 00106 return (r == Other.r) && (g == Other.g) && (b == Other.b) && (a == Other.a); 00107 } 00108 00109 00113 bool Color::operator !=(const Color& Other) const 00114 { 00115 return (r != Other.r) || (g != Other.g) || (b != Other.b) || (a != Other.a); 00116 } 00117 00118 00122 Color operator +(const Color& Color1, const Color& Color2) 00123 { 00124 Color c = Color1; 00125 c += Color2; 00126 00127 return c; 00128 } 00129 00130 00134 Color operator *(const Color& Color1, const Color& Color2) 00135 { 00136 Color c = Color1; 00137 c *= Color2; 00138 00139 return c; 00140 } 00141 00142 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::