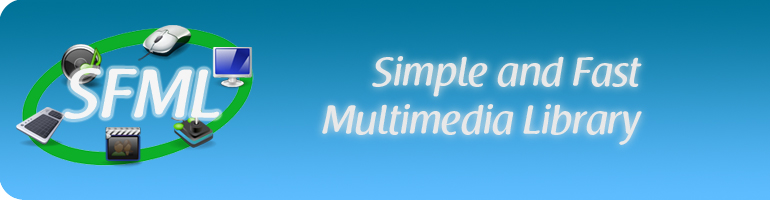
AudioDevice.cpp
00001 00002 // 00003 // SFML - Simple and Fast Multimedia Library 00004 // Copyright (C) 2007-2009 Laurent Gomila ([email protected]) 00005 // 00006 // This software is provided 'as-is', without any express or implied warranty. 00007 // In no event will the authors be held liable for any damages arising from the use of this software. 00008 // 00009 // Permission is granted to anyone to use this software for any purpose, 00010 // including commercial applications, and to alter it and redistribute it freely, 00011 // subject to the following restrictions: 00012 // 00013 // 1. The origin of this software must not be misrepresented; 00014 // you must not claim that you wrote the original software. 00015 // If you use this software in a product, an acknowledgment 00016 // in the product documentation would be appreciated but is not required. 00017 // 00018 // 2. Altered source versions must be plainly marked as such, 00019 // and must not be misrepresented as being the original software. 00020 // 00021 // 3. This notice may not be removed or altered from any source distribution. 00022 // 00024 00026 // Headers 00028 #include <SFML/Audio/AudioDevice.hpp> 00029 #include <SFML/Audio/AudioResource.hpp> 00030 #include <SFML/Audio/Listener.hpp> 00031 #include <algorithm> 00032 #include <iostream> 00033 00034 00035 namespace sf 00036 { 00037 namespace priv 00038 { 00040 // Static member data 00042 AudioDevice* AudioDevice::ourInstance; 00043 00044 00048 AudioDevice::AudioDevice() : 00049 myRefCount(0) 00050 { 00051 // Create the device 00052 myDevice = alcOpenDevice(NULL); 00053 00054 if (myDevice) 00055 { 00056 // Create the context 00057 myContext = alcCreateContext(myDevice, NULL); 00058 00059 if (myContext) 00060 { 00061 // Set the context as the current one (we'll only need one) 00062 alcMakeContextCurrent(myContext); 00063 00064 // Initialize the listener, located at the origin and looking along the Z axis 00065 Listener::SetPosition(0.f, 0.f, 0.f); 00066 Listener::SetTarget(0.f, 0.f, -1.f); 00067 } 00068 else 00069 { 00070 std::cerr << "Failed to create the audio context" << std::endl; 00071 } 00072 } 00073 else 00074 { 00075 std::cerr << "Failed to open the audio device" << std::endl; 00076 } 00077 } 00078 00079 00083 AudioDevice::~AudioDevice() 00084 { 00085 // Destroy the context 00086 alcMakeContextCurrent(NULL); 00087 if (myContext) 00088 alcDestroyContext(myContext); 00089 00090 // Destroy the device 00091 if (myDevice) 00092 alcCloseDevice(myDevice); 00093 } 00094 00095 00099 AudioDevice& AudioDevice::GetInstance() 00100 { 00101 // Create the audio device if it doesn't exist 00102 if (!ourInstance) 00103 ourInstance = new AudioDevice; 00104 00105 return *ourInstance; 00106 } 00107 00108 00112 void AudioDevice::AddReference() 00113 { 00114 // Create the audio device if it doesn't exist 00115 if (!ourInstance) 00116 ourInstance = new AudioDevice; 00117 00118 // Increase the references count 00119 ourInstance->myRefCount++; 00120 } 00121 00122 00126 void AudioDevice::RemoveReference() 00127 { 00128 // Decrease the references count 00129 ourInstance->myRefCount--; 00130 00131 // Destroy the audio device if the references count reaches 0 00132 if (ourInstance->myRefCount == 0) 00133 { 00134 delete ourInstance; 00135 ourInstance = NULL; 00136 } 00137 } 00138 00139 00143 ALCdevice* AudioDevice::GetDevice() const 00144 { 00145 return myDevice; 00146 } 00147 00148 00152 ALenum AudioDevice::GetFormatFromChannelsCount(unsigned int ChannelsCount) const 00153 { 00154 // Find the good format according to the number of channels 00155 switch (ChannelsCount) 00156 { 00157 case 1 : return AL_FORMAT_MONO16; 00158 case 2 : return AL_FORMAT_STEREO16; 00159 case 4 : return alGetEnumValue("AL_FORMAT_QUAD16"); 00160 case 6 : return alGetEnumValue("AL_FORMAT_51CHN16"); 00161 case 7 : return alGetEnumValue("AL_FORMAT_61CHN16"); 00162 case 8 : return alGetEnumValue("AL_FORMAT_71CHN16"); 00163 } 00164 00165 return 0; 00166 } 00167 00168 } // namespace priv 00169 00170 } // namespace sf
:: Copyright © 2007-2008 Laurent Gomila, all rights reserved :: Documentation generated by doxygen 1.5.2 ::