GameShield V5 SDK Programming Guide
v5.2
|
GameShield name space. More...
Classes | |
class | TGSObject |
Base of GS5 Objects. More... | |
class | TGSVariable |
User defined variables or parameters of action / licenses. More... | |
class | TGSAction |
GS5 Action Object. More... | |
class | TGSLicense |
GS License Object. More... | |
class | TGSRequest |
Request Object. More... | |
class | TGSEntity |
Entity Object. More... | |
class | TGSCore |
GS5 Core Object. More... | |
class | TLM_Inspector |
class | TLM_Period |
LM_Expire_Period Inspector. More... | |
class | TGSApp |
GS5 Application. More... | |
class | TGSDynamicLM |
Base class of Dynamic License Model. More... | |
Typedefs | |
typedef void * | gs_handle_t |
GS5 Object handle. | |
typedef gs_handle_t | TEntityHandle |
Entity Object handle. | |
typedef gs_handle_t | TLicenseHandle |
License Object Handle. | |
typedef gs_handle_t | TVarHandle |
Variable / Parameter Object Handle. | |
typedef gs_handle_t | TMonitorHandle |
GS5 event monitor object handle. | |
typedef gs_handle_t | TActionHandle |
Action Object Handle. | |
typedef gs_handle_t | TRequestHandle |
Request Object Handle. | |
typedef gs_handle_t | TEventHandle |
Event Object Handle. | |
typedef gs_handle_t | TEventSourceHandle |
Event Source Object Handle. | |
typedef const char * | entity_id_t |
Entity Id is a string. | |
typedef const char * | license_id_t |
License Id is a string. | |
typedef unsigned char | action_id_t |
Action Id is a unsigned byte. | |
typedef int | var_type_t |
Variable TypeId is an int. | |
Enumerations | |
enum | { GS_ERROR_GENERIC = -1, GS_ERROR_INVALID_HANDLE = 1, GS_ERROR_INVALID_INDEX = 2, GS_ERROR_INVALID_NAME = 3, GS_ERROR_INVALID_ACTION = 4, GS_ERROR_INVALID_LICENSE = 5, GS_ERROR_INVALID_ENTITY = 6, GS_ERROR_INVALID_VALUE = 7 } |
GS5 error code. More... | |
enum | TLicensePolicy { POLICY_INVALID, POLICY_ANY, POLICY_ALL } |
License Policy. More... | |
enum | TLicenseStatus { STATUS_INVALID = -1, STATUS_LOCKED = 0, STATUS_UNLOCKED = 1, STATUS_ACTIVE = 2 } |
License Status. More... | |
enum | TEventType { EVENT_TYPE_APP = 0, EVENT_TYPE_LICENSE = 100, EVENT_TYPE_ENTITY = 200, EVENT_TYPE_USER = 0x10000000 } |
Defines Event Type Base. More... | |
User Defined Variable | |
enum | TVarType { VAR_TYPE_INT = 7, VAR_TYPE_INT64 = 8, VAR_TYPE_FLOAT = 9, VAR_TYPE_DOUBLE = 10 , VAR_TYPE_STRING = 20, VAR_TYPE_TIME = 30 } |
User Defined Variable TypeId. More... | |
Functions | |
template<> | |
void | TGSVariable::get< std::string > (std::string &v) |
gets value as string | |
template<> | |
void | TGSVariable::set< std::string > (std::string v) |
sets value from string | |
int | gsInit (const char *productId, const char *origLic, const char *password, void *reserved) |
One-time Initialization of gsCore. More... | |
int | gsLoadFromLocalStorage (const char *productId, const char *password) |
Loads from Local Storage (* Read Only*) More... | |
int | gsLoadFromLicenseFile (const char *productLic, const char *productId, const char *password) |
Loads from external license file (* Read Only*) More... | |
int | gsCleanUp () |
Finalize the gsCore. More... | |
const char * | gsGetVersion () |
Get the current SDK version. | |
void | gsCloseHandle (gs_handle_t handle) |
Close GS5 Object Handle. More... | |
void | gsFlush () |
Save pending license changes in memory to local storage. More... | |
const char * | gsGetLastErrorMessage () |
Get the last error message. More... | |
int | gsGetLastErrorCode () |
Get the last error code. More... | |
int | gsGetBuildId () |
Returns the Build Id of the running binary release. More... | |
const char * | gsGetProductName () |
Get Product Name See: ProductName. | |
const char * | gsGetProductId () |
Get Product Id See: ProductId. | |
bool | gsRunInWrappedMode () |
Test if the current process is running inside GS5 Ironwrapper runtime. More... | |
const char * | gsGetAppRootPath () |
Gets the root path of the application. | |
const char * | gsGetAppCommandLine () |
Gets the startup game exe commandline. | |
const char * | gsGetAppMainExe () |
Gets the full path to the startup game exe. | |
const char * | gsRevoke () |
Revokes local license. More... | |
bool | gsIsNodeLocked () |
Node Lock ( Copy Protection ) | |
Entity APIs | |
int | gsGetEntityCount () |
Get the total number of entities defined in the application's license file. More... | |
TEntityHandle | gsOpenEntityByIndex (int index) |
Get the entity object by index. More... | |
TEntityHandle | gsOpenEntityById (entity_id_t entityId) |
Get the entity object by entity's unique id. More... | |
unsigned int | gsGetEntityAttributes (TEntityHandle hEntity) |
Get the entity's current license status. More... | |
entity_id_t | gsGetEntityId (TEntityHandle hEntity) |
Get the entity's unique id. More... | |
const char * | gsGetEntityName (TEntityHandle hEntity) |
Get the entity's name. More... | |
const char * | gsGetEntityDescription (TEntityHandle hEntity) |
Get the entity's description. More... | |
TLicensePolicy | gsGetEntityLicensePolicy (TEntityHandle hEntity) |
Get the entity's license policy (ref: LicensePolicy) More... | |
bool | gsBeginAccessEntity (TEntityHandle hEntity) |
Try start accessing an entity. More... | |
bool | gsEndAccessEntity (TEntityHandle hEntity) |
Try end accessing an entity. More... | |
License APIs | |
int | gsGetLicenseCount (TEntityHandle hEntity) |
Gets the total number of licenses currently attached to an entity. | |
TLicenseHandle | gsOpenLicenseByIndex (TEntityHandle hEntity, int index) |
Gets license object handle by its index. More... | |
TLicenseHandle | gsOpenLicenseById (TEntityHandle hEntity, license_id_t licenseId) |
Gets license object handle by its id. More... | |
license_id_t | gsGetLicenseId (TLicenseHandle hLicense) |
Gets the license object's license id. | |
const char * | gsGetLicenseName (TLicenseHandle hLicense) |
Gets the license object's license name. | |
const char * | gsGetLicenseDescription (TLicenseHandle hLicense) |
Gets the license object's license description. | |
TLicenseStatus | gsGetLicenseStatus (TLicenseHandle hLicense) |
Gets the license object's license status (ref: LicenseStatus) | |
bool | gsIsLicenseValid (TLicenseHandle hLicense) |
Is license currently valid? | |
TEntityHandle | gsGetLicensedEntity (TLicenseHandle hLicense) |
Get the entity object to which the license is attached. | |
int | gsGetLicenseParamCount (TLicenseHandle hLicense) |
Get total number of parameters in a license. | |
TVarHandle | gsGetLicenseParamByIndex (TLicenseHandle hLicense, int index) |
Get the license parameter by its index, ranges [0, gsGetLicenseParamCount()-1 ]. | |
TVarHandle | gsGetLicenseParamByName (TLicenseHandle hLicense, const char *name) |
Get the license parameter by its name. | |
Action APIs | |
int | gsGetActionInfoCount (TLicenseHandle hLicense) |
Gets total number of actions appliable to a license Ref: ActionInfo. | |
const char * | gsGetActionInfoByIndex (TLicenseHandle hLicense, int index, action_id_t *actionId) |
Gets action information by index (ref: ActionInfo) More... | |
const char * | gsGetActionName (TActionHandle hAct) |
Gets action name. | |
action_id_t | gsGetActionId (TActionHandle hAct) |
Gets action unique id. | |
const char * | gsGetActionDescription (TActionHandle hAct) |
Gets action description. | |
const char * | gsGetActionString (TActionHandle hAct) |
Gets action what-to-do string (ref: What to do action string) | |
int | gsGetActionParamCount (TActionHandle hAct) |
Gets the total number of action parameters. | |
TVarHandle | gsGetActionParamByName (TActionHandle hAct, const char *paramName) |
Gets action parameter by its name. More... | |
TVarHandle | gsGetActionParamByIndex (TActionHandle hAct, int index) |
Gets action parameter by its index. More... | |
Variable / Parameter APIs | |
TVarHandle | gsAddVariable (const char *varName, TVarType varType, int attr, const char *initValStr) |
Adds a user defined variable. More... | |
bool | gsRemoveVariable (const char *varName) |
Remove a user defined variable. More... | |
TVarHandle | gsGetVariable (const char *varName) |
Gets a user defined variable. More... | |
int | gsGetTotalVariables () |
Get total number of user defined variables. | |
TVarHandle | gsGetVariableByIndex (int index) |
Get user defined variable by its index. | |
const char * | gsGetVariableName (TVarHandle hVar) |
Get variable's name. | |
TVarType | gsGetVariableType (TVarHandle hVar) |
Get variable's type id (ref: varType) | |
bool | gsIsVariableValid (TVarHandle hVar) |
Is variable holds a valid value? | |
const char * | gsVariableTypeToString (var_type_t paramType) |
Convert variable's type id to type string (ref: varType) | |
int | gsGetVariableAttr (TVarHandle hVar) |
Gets variable's attribute (ref: varAttr) | |
const char * | gsVariableAttrToString (int attr, char *buf, int bufSize) |
Converts from variable's attribute value to its string format (ref: varAttr) | |
int | gsVariableAttrFromString (const char *attrStr) |
Converts from variable's attribute string to attribute value (ref: varAttr) | |
const char * | gsGetVariableValueAsString (TVarHandle hVar) |
Returns param value as value string. More... | |
bool | gsSetVariableValueFromString (TVarHandle hVar, const char *valStr) |
Sets the variable's value from a string. More... | |
bool | gsGetVariableValueAsInt (TVarHandle hVar, int &val) |
Returns param value as a 32 bit integer. More... | |
bool | gsSetVariableValueFromInt (TVarHandle hVar, int val) |
Sets the variable's value from an integer. | |
bool | gsGetVariableValueAsInt64 (TVarHandle hVar, int64_t &val) |
Returns param value as 64 bit integer. More... | |
bool | gsSetVariableValueFromInt64 (TVarHandle hVar, int64_t val) |
Sets the variable's value from a 64 bit integer. | |
bool | gsGetVariableValueAsFloat (TVarHandle hVar, float &val) |
Returns param value as float. More... | |
bool | gsSetVariableValueFromFloat (TVarHandle hVar, float val) |
Sets the variable's value from a float. | |
bool | gsGetVariableValueAsDouble (TVarHandle hVar, double &val) |
Returns param value as double. More... | |
bool | gsSetVariableValueFromDouble (TVarHandle hVar, double val) |
Sets the variable's value from a double. | |
bool | gsGetVariableValueAsTime (TVarHandle hVar, time_t &val) |
Gets the variable's value as a time_t value. More... | |
bool | gsSetVariableValueFromTime (TVarHandle hVar, time_t val) |
Sets the variable's value from a time_t value. | |
Request APIs | |
TRequestHandle | gsCreateRequest () |
Create a request object. | |
TActionHandle | gsAddRequestAction (TRequestHandle hReq, action_id_t actId, TLicenseHandle hLic) |
Create and add an action to the request. More... | |
TActionHandle | gsAddRequestActionEx (TRequestHandle hReq, action_id_t actId, const char *entityId, const char *licenseId) |
Create and add an action to the request. More... | |
const char * | gsGetRequestCode (TRequestHandle hReq) |
Generates the request code from the request object. | |
bool | gsApplyLicenseCode (const char *activationCode) |
Applys license code (aka. Activation Code) | |
HTML Render APIs | |
bool | gsRenderHTML (const char *url, const char *title, int width, int height) |
Rendering HTML page in process. More... | |
bool | gsRenderHTMLEx (const char *url, const char *title, int width, int height, bool resizable, bool exitAppWhenUIClosed, bool cleanUpAfterRendering) |
Rendering HTML with more control (Since SDK 5.0.7) More... | |
Debug Helpers | |
bool | gsIsDebugVersion () |
Is the SDK binary a DEBUG version? | |
void | gsTrace (const char *msg) |
Output debug message. More... | |
Application Control APIs | |
void | gsExitApp (int rc) |
Exit application gracefully. | |
void | gsTerminateApp (int rc) |
Exit application forcefully. | |
void | gsPlayApp () |
Continue running the application. | |
void | gsPauseApp () |
Pauses the application. | |
void | gsResumeAndExitApp () |
Resume a paused application and quit immediately. | |
void | gsRestartApp () |
Restart the current application. | |
bool | gsIsRestartedApp () |
Is the current application a restarted session? | |
Session Variables APIs | |
void | gsSetAppVar (const char *name, const char *val) |
Sets the value of an application session variable. | |
const char * | gsGetAppVar (const char *name) |
Gets the value of an application session variable. | |
User Defined Event APIs | |
void | gsPostUserEvent (unsigned int eventId, bool bSync, void *eventData, unsigned int eventDataSize) |
Post User Event. More... | |
void * | gsGetUserEventData (TEventHandle hEvent, unsigned int *evtDataSize) |
Gets user defined event data information. More... | |
Virtual Machine Support | |
typedef unsigned int | vm_mask_t |
Virtual Machine Id Mask. | |
bool | gsRunInsideVM (vm_mask_t vmask) |
Detailed Description
GameShield name space.
Enumeration Type Documentation
anonymous enum |
GS5 error code.
enum gs::TEventType |
enum gs::TLicensePolicy |
License Policy.
Defines the combination policy of the multiple licenses attached to a single entity
enum gs::TLicenseStatus |
enum gs::TVarType |
User Defined Variable TypeId.
Ref: varType gs::gsAddVariable()
Enumerator | |
---|---|
VAR_TYPE_INT |
32-bit integer |
VAR_TYPE_INT64 |
64-bit integer |
VAR_TYPE_FLOAT |
float |
VAR_TYPE_DOUBLE |
double |
VAR_TYPE_STRING |
Boolean. |
VAR_TYPE_TIME |
ansi-string |
Function Documentation
TActionHandle gs::gsAddRequestAction | ( | TRequestHandle | hReq, |
action_id_t | actId, | ||
TLicenseHandle | hLic | ||
) |
Create and add an action to the request.
- Parameters
-
hReq The request handle the action is added to actId The action type id hLic The target license the action is apply to, NULL if a global action (apply to all entities/licenses)
- Returns
- The action handle, 0 if the action type is not supported.
TActionHandle gs::gsAddRequestActionEx | ( | TRequestHandle | hReq, |
action_id_t | actId, | ||
const char * | entityId, | ||
const char * | licenseId | ||
) |
Create and add an action to the request.
- Parameters
-
hReq The request handle the action is added to actId The action type id (entityId,licenseId) The target license(s) the action is apply to, (NULL, NULL) for a global action, (entityId, NULL) specify action is applied to all licenses associated to the entity
- Returns
- The action handle, 0 if the action type is not supported.
TVarHandle gs::gsAddVariable | ( | const char * | varName, |
TVarType | varType, | ||
int | attr, | ||
const char * | initValStr | ||
) |
Adds a user defined variable.
- Parameters
-
varName variable name varType TVarType, ref: Variable Type attr variable attribute, ref: Variable Attribute It is a combination of Variable Attribute Mask. initValStr string representation of initial variable value
- Returns
- the handle to the created variable, or INVALID_GS_HANDLE on error.
bool gs::gsBeginAccessEntity | ( | TEntityHandle | hEntity | ) |
Try start accessing an entity.
If an entity is accessible, all of the associated resources (files, keys, codes, etc.) can be legally used, otherwise they cannot be accessed by the application.
The api can be called recursively, and each call must be paired with a gsEndAccessEntity(). When the api is called for the first time the event EVENT_ENTITY_TRY_ACCESS and EVENT_ENTITY_ACCESS_STARTED are posted.
- Parameters
-
hEntity The handle to entity to be accessed
- Returns
- returns true if the entity is accessed successfully. returns false if:
- Cannot access any entity when your game is wrapped by a DEMO version of GS5/IDE and the its demo license has expired;
- Entity cannot be accessed due to its negative license feedback;
int gs::gsCleanUp | ( | ) |
Finalize the gsCore.
When the application is terminating, call this api to cleanup the internal data resources.
void gs::gsCloseHandle | ( | gs_handle_t | handle | ) |
Close GS5 Object Handle.
All handles from gsCore apis must be closed to release internal resources.
bool gs::gsEndAccessEntity | ( | TEntityHandle | hEntity | ) |
Try end accessing an entity.
- Parameters
-
hEntity The handle to entity being accessed
- Returns
- true on success, false if there is unexpected error occurs.
This api must be paired with gsBeginAccessEntity(), if it is the last calling then event EVENT_ENTITY_ACCESS_ENDING and EVENT_ENTITY_ACCESS_ENDED will be posted.
void gs::gsFlush | ( | ) |
Save pending license changes in memory to local storage.
Usually the changes in memory is saved to local storage periodically when game running, this api focce a saving immediately.
const char* gs::gsGetActionInfoByIndex | ( | TLicenseHandle | hLicense, |
int | index, | ||
action_id_t * | actionId | ||
) |
Gets action information by index (ref: ActionInfo)
- Parameters
-
hLicense License handle to be inspected index index of action information, range [0, getActionInfoCount()-1 ] [out] actionId output buffer to receive action id
- Returns
- the action name
TVarHandle gs::gsGetActionParamByIndex | ( | TActionHandle | hAct, |
int | index | ||
) |
Gets action parameter by its index.
- Parameters
-
hAct handle to action being inspected; index the index of parameter, range [0, gsGetActionParamCount()-1 ]
- Returns
- Variable handle on success, INVALID_GS_HANDLE if paramer not found
TVarHandle gs::gsGetActionParamByName | ( | TActionHandle | hAct, |
const char * | paramName | ||
) |
Gets action parameter by its name.
- Parameters
-
hAct handle to action being inspected; paramName the string name of a action parameter.
- Returns
- Variable handle on success, INVALID_GS_HANDLE if paramer not found
int gs::gsGetBuildId | ( | ) |
Returns the Build Id of the running binary release.
See: BuildId
unsigned int gs::gsGetEntityAttributes | ( | TEntityHandle | hEntity | ) |
Get the entity's current license status.
- Parameters
-
hEntity Handle to the entity object
- Returns
- EntityAttr
int gs::gsGetEntityCount | ( | ) |
Get the total number of entities defined in the application's license file.
- Returns
- -1 if error occurs.
const char* gs::gsGetEntityDescription | ( | TEntityHandle | hEntity | ) |
Get the entity's description.
- Parameters
-
hEntity Handle to the entity object
- Returns
- entity description
entity_id_t gs::gsGetEntityId | ( | TEntityHandle | hEntity | ) |
Get the entity's unique id.
- Parameters
-
hEntity Handle to the entity object
- Returns
- string entity unique id
TLicensePolicy gs::gsGetEntityLicensePolicy | ( | TEntityHandle | hEntity | ) |
Get the entity's license policy (ref: LicensePolicy)
- Parameters
-
hEntity Handle to the entity object
- Returns
- entity license policy
const char* gs::gsGetEntityName | ( | TEntityHandle | hEntity | ) |
Get the entity's name.
- Parameters
-
hEntity Handle to the entity object
- Returns
- entity name
int gs::gsGetLastErrorCode | ( | ) |
Get the last error code.
When gsCore API returns, you can always retrieve the last error code for details if on error.
const char* gs::gsGetLastErrorMessage | ( | ) |
Get the last error message.
When gsCore API returns, you can always retrieve the last error message for details if on error.
void* gs::gsGetUserEventData | ( | TEventHandle | hEvent, |
unsigned int * | evtDataSize | ||
) |
Gets user defined event data information.
- Parameters
-
hEvent The handle to user event [out] evtDataSize output inter receiving the length of event data
- Returns
- Pointer to user defined event data
TVarHandle gs::gsGetVariable | ( | const char * | varName | ) |
Gets a user defined variable.
- Parameters
-
varName the name of variable to retrieve
- Returns
- the handle to variable on success, INVALID_GS_HANDLE if variable not found.
bool gs::gsGetVariableValueAsDouble | ( | TVarHandle | hVar, |
double & | val | ||
) |
Returns param value as double.
- Parameters
-
hVar The param handle [out] val Reference to integer receiving the result
- Returns
- True on success, False if the variable is not readable, or there is a value conversion error.
bool gs::gsGetVariableValueAsFloat | ( | TVarHandle | hVar, |
float & | val | ||
) |
Returns param value as float.
- Parameters
-
hVar The param handle [out] val Reference to integer receiving the result
- Returns
- True on success, False if the variable is not readable, or there is a value conversion error.
bool gs::gsGetVariableValueAsInt | ( | TVarHandle | hVar, |
int & | val | ||
) |
Returns param value as a 32 bit integer.
- Parameters
-
hVar The param handle [out] val Reference to integer receiving the result
- Returns
- True on success, False if the variable is not readable, or there is a value conversion error.
bool gs::gsGetVariableValueAsInt64 | ( | TVarHandle | hVar, |
int64_t & | val | ||
) |
Returns param value as 64 bit integer.
- Parameters
-
hVar The param handle [out] val Reference to integer receiving the result
- Returns
- True on success, False if the variable is not readable, or there is a value conversion error.
const char* gs::gsGetVariableValueAsString | ( | TVarHandle | hVar | ) |
Returns param value as value string.
- Parameters
-
hVar The param handle
- Returns
- If the function succeeds, the return value is the pointer to the value string representation. If the variable is not readable, this function returns empty string ("").
bool gs::gsGetVariableValueAsTime | ( | TVarHandle | hVar, |
time_t & | val | ||
) |
Gets the variable's value as a time_t value.
- Parameters
-
hVar handle to variable object [out] val reference to time_t data structure receiving the result
- Returns
- true if on success, val holds the number of seconds since 00:00 hours, Jan 1, 1970 UTC (i.e., the current unix timestamp). return false if the variable does not hold a valid date time value. (
- See Also
- gsIsVariableValid())
int gs::gsInit | ( | const char * | productId, |
const char * | origLic, | ||
const char * | password, | ||
void * | reserved | ||
) |
One-time Initialization of gsCore.
Runtime Initializer, always update local storage as needed. [Read & Write]
It tries to search and load license in the following order:
- Loads from local storage first;
- Loads from embedded license data;
- Loads from input license file;
- Parameters
-
productId The Product Unique Id. Each product has an unique Id that can be used to identity the application on the remote server. origLic The full path to the original license document. This license file is compiled from the GameShield project IDE and defines the initial license status of the product. The file will not be altered and can be deployed in any folder. password The string key to decrypt the license document. reserved Reserved parameter
- Returns
- Returns 0 on success, otherwise non-zero, gsGetLastError() to get the error message.
int gs::gsLoadFromLicenseFile | ( | const char * | productLic, |
const char * | productId, | ||
const char * | password | ||
) |
Loads from external license file (* Read Only*)
As a read-only viewer, it tries to load from license file and won't update its content.
- Parameters
-
productLic The full path to the external license file. productId The Product Unique Id. Each product has an unique Id that can be used to identity the application on the remote server. password The string key to decrypt the license document.
- Returns
- Returns 0 on success, otherwise non-zero, gsGetLastError() to get the error message.
int gs::gsLoadFromLocalStorage | ( | const char * | productId, |
const char * | password | ||
) |
Loads from Local Storage (* Read Only*)
As a read-only viewer, it tries to load from local storage only and won't update local storage.
- Parameters
-
productId The Product Unique Id. Each product has an unique Id that can be used to identity the application on the remote server. password The string key to decrypt the license document.
- Returns
- Returns 0 on success, otherwise non-zero, gsGetLastError() to get the error message.
TEntityHandle gs::gsOpenEntityById | ( | entity_id_t | entityId | ) |
Get the entity object by entity's unique id.
- Parameters
-
entityId unique id of the entity, entityId
- Returns
- INVALID_GS_HANDLE if on error, otherwise the non-zero handle to the entity
Returned handle must be closed by gsCloseHandle() when not needed.
TEntityHandle gs::gsOpenEntityByIndex | ( | int | index | ) |
Get the entity object by index.
- Parameters
-
index index to the entity, range from 0 to gsGetEntityCount()-1
- Returns
- INVALID_GS_HANDLE if on error, otherwise the non-zero handle to the entity
Returned handle must be closed by gsCloseHandle() when not needed.
TLicenseHandle gs::gsOpenLicenseById | ( | TEntityHandle | hEntity, |
license_id_t | licenseId | ||
) |
Gets license object handle by its id.
- Parameters
-
hEntity the entity to be checked licenseId the license unique id
- Returns
- the license object handle
TLicenseHandle gs::gsOpenLicenseByIndex | ( | TEntityHandle | hEntity, |
int | index | ||
) |
Gets license object handle by its index.
- Parameters
-
hEntity the entity to be checked index the license index of all attached licenses, ranges :[ 0, gsGetLicenseCount()-1 ]
- Returns
- the license object handle
void gs::gsPostUserEvent | ( | unsigned int | eventId, |
bool | bSync, | ||
void * | eventData, | ||
unsigned int | eventDataSize | ||
) |
Post User Event.
- Parameters
-
eventId User defined event id ( must >= GS_USER_EVENT ) bSync true if event is posted synchronized, the api returns after the event has been parsed by all event handlers. otherwise the api returns immediately. eventData [Optional] data buffer pointer associated with the event, NULL if no event data eventDataSize size of event data buffer, ignored if eventData is NULL
- Returns
- none
bool gs::gsRemoveVariable | ( | const char * | varName | ) |
Remove a user defined variable.
- Parameters
-
varName the name of variable to remove
- Returns
- true if the variable is removed, false if variable not found.
bool gs::gsRenderHTML | ( | const char * | url, |
const char * | title, | ||
int | width, | ||
int | height | ||
) |
Rendering HTML page in process.
- Parameters
-
url URL to html local file or web site page to render; title The caption of form window rendering the HTML page; width Pixel width of HTML page; height Pixel height of HTML page;
It can be called before gsInit() to render generic HTML pages. However, gsInit() must be called before to render LMApp HTML pages.
The default behavior is:
- Windows Resizable = True;
- ExitAppAfterUI = False;
- CleanUpAfterRender = False;
- See Also
- gsRenderHTMLEx
bool gs::gsRenderHTMLEx | ( | const char * | url, |
const char * | title, | ||
int | width, | ||
int | height, | ||
bool | resizable, | ||
bool | exitAppWhenUIClosed, | ||
bool | cleanUpAfterRendering | ||
) |
Rendering HTML with more control (Since SDK 5.0.7)
- Parameters
-
url URL to html local file or web site page to render; title The caption of form window rendering the HTML page; width Pixel width of HTML page; height Pixel height of HTML page; resizable,: The HTML windows is resizable. exitAppWhenUIClosed,: Terminate current process when the HTML main windows is manually closed (clicking [x] button on right title for Windows). if false, the UI is just closed. cleanUpAfterRendering,: Clean up all of the internal rendering facilities before this API returns. If you cleanup rendering, then the possible conflicts with game are minimized, however, next time the render engine has to be re-created again. WARNING: If set to true, the Qt rendering engine might CRASH for the second time api calling due to Qt internal issue!!! So the best practice is that: Only set cleanUpAfterRendering to true if it is the last time rendering.
If you do not cleanup rendering, the render engine stays active in memory and is quick for next rendering. However, since the Qt/Win stuffs is still alive, it might conflict with game in unexpected way.(Mac: The top main menu bar, about, etc.)
const char* gs::gsRevoke | ( | ) |
Revokes local license.
This api invalidates current application licenses and returns a string as a receipt.
After this api calling, the application should be terminated because all licenses have been locked down.
- Returns
- empty ("") if fails, non-empty string as receipt on success.
The api might fail because:
- Project license setting is not node-locked, hence no reason to lock down local license before transferring license between different machines;
- There is no already unlocked (fully pruchased) license in application, so there is no reason to lock down a demo version;
- Local license storage updating error;
bool gs::gsRunInsideVM | ( | vm_mask_t | vmask | ) |
Test if the current process is runing inside a virtual machine.
- Parameters
-
vmask mask of VM types VM Supported : VM_VMware (0x01), VM_VirtualPC (0x02),VM_VirtualBox (0x04), VM_Fusion (0x08), VM_Parallel (0x10), VM_QEMU (0x20)
- Returns
- true if any of the VM types (or'ed in the mask) is detected.
It can be called before gsInit().
bool gs::gsRunInWrappedMode | ( | ) |
Test if the current process is running inside GS5 Ironwrapper runtime.
It can be called before gsInit().
bool gs::gsSetVariableValueFromString | ( | TVarHandle | hVar, |
const char * | valStr | ||
) |
Sets the variable's value from a string.
- Parameters
-
hVar The param handle valStr The param value in string format
- Returns
- true on success, false if the variable is not writable, or there is a value conversion error.
void gs::gsTrace | ( | const char * | msg | ) |
Output debug message.
For SDK/Debug version, the message is appended to the current debug log file. For SDK/Release version, the message is displayed in: (1) DebugViewer (Windows via OutputDebugString); (2) Console (Unix via printf)
Generated on Mon Feb 3 2014 13:15:29 for GameShield V5 SDK Programming Guide by
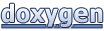