GameShield V5 SDK Programming Guide
v5.2
|
gsCore C API Interface More...
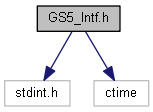
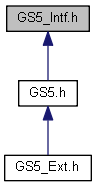
Namespaces | |
gs | |
GameShield name space. | |
Macros | |
#define | INVALID_GS_HANDLE NULL |
Invalid GS5 object handle is NULL. | |
#define | GS_USER_EVENT 0x10000000 |
User defined event id must >= GS_USER_EVENT. | |
Entity Status Attributes | |
#define | ENTITY_ATTRIBUTE_ACCESSIBLE 1 |
Entity is currently accessible. | |
#define | ENTITY_ATTRIBUTE_UNLOCKED 2 |
Entity's license is fully activated, no expire /trial limits at all. | |
#define | ENTITY_ATTRIBUTE_ACCESSING 4 |
Entity is active (being accessed via gsBeginAccessEntity()) | |
#define | ENTITY_ATTRIBUTE_LOCKED 8 |
Entity is locked. | |
#define | ENTITY_ATTRIBUTE_AUTOSTART 16 |
Entity is auto-start. | |
License Model Property Permission | |
#define | LM_PARAM_HIDDEN 1 |
the param is invisible from SDK apis | |
#define | LM_PARAM_TEMP 2 |
the param is not persistent (not saved in local license storage) | |
#define | LM_PARAM_READ 4 |
the param can read via SDK apis | |
#define | LM_PARAM_WRITE 8 |
the param can write via SDK apis | |
#define | LM_PARAM_INHERIT 16 |
the param is inheritable (new build will inherit value from old build when license upgrading) | |
Application Events | |
#define | EVENT_IDBASE_APPLICATION 0 |
#define | EVENT_APP_BEGIN 1 |
Application just gets started, please initialize. More... | |
#define | EVENT_APP_END 2 |
Application is going to terminate, last signal before game exits. | |
#define | EVENT_APP_CLOCK_ROLLBACK 3 |
Alarm: Application detects the clock is rolled back. | |
#define | EVENT_APP_INTEGRITY_CORRUPT 4 |
Fatal Error: Application integrity is corrupted. | |
#define | EVENT_APP_RUN 5 |
Application starts to run, last signal before game code is executing. | |
License Events | |
#define | EVENT_IDBASE_LICENSE 100 |
License event id range: [100, 199]. | |
#define | EVENT_LICENSE_NEWINSTALL 101 |
Original license is uploaded to license store for the first time. | |
#define | EVENT_LICENSE_READY 102 |
The application's license store is connected /initialized successfully (gsCore::gsInit() == 0) | |
#define | EVENT_LICENSE_FAIL 103 |
The application's license store cannot be connected /initialized! (gsCore::gsInit() != 0) | |
#define | EVENT_LICENSE_LOADING 105 |
License is loading... | |
Entity Events | |
#define | EVENT_IDBASE_ENTITY 200 |
Entity event id range [200, 299]. | |
#define | EVENT_ENTITY_TRY_ACCESS 201 |
#define | EVENT_ENTITY_ACCESS_STARTED 202 |
#define | EVENT_ENTITY_ACCESS_ENDING 203 |
#define | EVENT_ENTITY_ACCESS_ENDED 204 |
#define | EVENT_ENTITY_ACCESS_INVALID 205 |
Alarm: Entity access invalid (due to expiration, etc) | |
#define | EVENT_ENTITY_ACCESS_HEARTBEAT 206 |
Internal ping event indicating entity is still alive. | |
#define | EVENT_ENTITY_ACTION_APPLIED 208 |
Action Applied to Entity The status of attached licenses have been modified by applying license action. More... | |
Generic actions | |
Action IDs | |
#define | ACT_UNLOCK 1 |
Unlock entity / license. | |
#define | ACT_LOCK 2 |
Lock down entity / license. | |
#define | ACT_SET_PARAM 3 |
#define | ACT_ENABLE_PARAM 4 |
#define | ACT_DISABLE_PARAM 5 |
#define | ACT_ENABLE_COPYPROTECTION 6 |
Enable Copy protection feature (NodeLock) | |
#define | ACT_DISABLE_COPYPROTECTION 7 |
Disable Copy protection feature (NodeLock) | |
#define | ACT_ENABLE_ALLEXPIRATION 8 |
#define | ACT_DISABLE_ALLEXPIRATION 9 |
#define | ACT_RESET_ALLEXPIRATION 10 |
#define | ACT_CLEAN 11 |
Clean up local license storage. | |
#define | ACT_DUMMY 12 |
Dummy action, carry only client id. | |
#define | ACT_PUSH 13 |
#define | ACT_PULL 14 |
#define | ACT_NAG_ON 15 |
Enable Demo Nag UI. | |
#define | ACT_NAG_OFF 16 |
Disable Demo Nag UI. | |
#define | ACT_ONE_SHOT 17 |
Activation Code can be used only once. | |
#define | ACT_SHELFTIME 18 |
Activation Code has a shelf time. | |
#define | ACT_FP_FIX 19 |
FingerPrint Mismatch Fix. | |
#define | ACT_REVOKE 20 |
Revoke local license. | |
LM-specific actions | |
#define | ACT_ADD_ACCESSTIME 100 |
Increase /Decrease access time (LM.expire.accessTime) | |
#define | ACT_SET_ACCESSTIME 101 |
Sets access time (LM.expire.accessTime) | |
#define | ACT_SET_STARTDATE 102 |
Sets start date (LM.expire.hardDate) | |
#define | ACT_SET_ENDDATE 103 |
Sets end date (LM.expire.hardDate) | |
#define | ACT_SET_SESSIONTIME 104 |
Sets maximum execution session time (LM.expire.sessionTime) | |
#define | ACT_SET_EXPIRE_PERIOD 105 |
Sets expire period (LM.expire.period) | |
#define | ACT_ADD_EXPIRE_PERIOD 106 |
Increases / Decreases expire period (LM.expire.period) | |
#define | ACT_SET_EXPIRE_DURATION 107 |
Sets expire duration (LM.expire.duration) | |
#define | ACT_ADD_EXPIRE_DURATION 108 |
Increases / Decreases expire duration (LM.expire.duration) | |
Typedefs | |
typedef void * | gs::gs_handle_t |
GS5 Object handle. | |
typedef gs_handle_t | gs::TEntityHandle |
Entity Object handle. | |
typedef gs_handle_t | gs::TLicenseHandle |
License Object Handle. | |
typedef gs_handle_t | gs::TVarHandle |
Variable / Parameter Object Handle. | |
typedef gs_handle_t | gs::TMonitorHandle |
GS5 event monitor object handle. | |
typedef gs_handle_t | gs::TActionHandle |
Action Object Handle. | |
typedef gs_handle_t | gs::TRequestHandle |
Request Object Handle. | |
typedef gs_handle_t | gs::TEventHandle |
Event Object Handle. | |
typedef gs_handle_t | gs::TEventSourceHandle |
Event Source Object Handle. | |
typedef const char * | gs::entity_id_t |
Entity Id is a string. | |
typedef const char * | gs::license_id_t |
License Id is a string. | |
typedef unsigned char | gs::action_id_t |
Action Id is a unsigned byte. | |
typedef int | gs::var_type_t |
Variable TypeId is an int. | |
Enumerations | |
enum | gs::TLicensePolicy { gs::POLICY_INVALID, gs::POLICY_ANY, gs::POLICY_ALL } |
License Policy. More... | |
enum | gs::TLicenseStatus { gs::STATUS_INVALID = -1, gs::STATUS_LOCKED = 0, gs::STATUS_UNLOCKED = 1, gs::STATUS_ACTIVE = 2 } |
License Status. More... | |
enum | gs::TEventType { gs::EVENT_TYPE_APP = 0, gs::EVENT_TYPE_LICENSE = 100, gs::EVENT_TYPE_ENTITY = 200, gs::EVENT_TYPE_USER = 0x10000000 } |
Defines Event Type Base. More... | |
Functions | |
int | gs::gsInit (const char *productId, const char *origLic, const char *password, void *reserved) |
One-time Initialization of gsCore. More... | |
int | gs::gsLoadFromLocalStorage (const char *productId, const char *password) |
Loads from Local Storage (* Read Only*) More... | |
int | gs::gsLoadFromLicenseFile (const char *productLic, const char *productId, const char *password) |
Loads from external license file (* Read Only*) More... | |
int | gs::gsCleanUp () |
Finalize the gsCore. More... | |
const char * | gs::gsGetVersion () |
Get the current SDK version. | |
void | gs::gsCloseHandle (gs_handle_t handle) |
Close GS5 Object Handle. More... | |
void | gs::gsFlush () |
Save pending license changes in memory to local storage. More... | |
const char * | gs::gsGetLastErrorMessage () |
Get the last error message. More... | |
int | gs::gsGetLastErrorCode () |
Get the last error code. More... | |
int | gs::gsGetBuildId () |
Returns the Build Id of the running binary release. More... | |
const char * | gs::gsGetProductName () |
Get Product Name See: ProductName. | |
const char * | gs::gsGetProductId () |
Get Product Id See: ProductId. | |
bool | gs::gsRunInWrappedMode () |
Test if the current process is running inside GS5 Ironwrapper runtime. More... | |
const char * | gs::gsGetAppRootPath () |
Gets the root path of the application. | |
const char * | gs::gsGetAppCommandLine () |
Gets the startup game exe commandline. | |
const char * | gs::gsGetAppMainExe () |
Gets the full path to the startup game exe. | |
const char * | gs::gsRevoke () |
Revokes local license. More... | |
bool | gs::gsIsNodeLocked () |
Node Lock ( Copy Protection ) | |
Entity APIs | |
int | gs::gsGetEntityCount () |
Get the total number of entities defined in the application's license file. More... | |
TEntityHandle | gs::gsOpenEntityByIndex (int index) |
Get the entity object by index. More... | |
TEntityHandle | gs::gsOpenEntityById (entity_id_t entityId) |
Get the entity object by entity's unique id. More... | |
unsigned int | gs::gsGetEntityAttributes (TEntityHandle hEntity) |
Get the entity's current license status. More... | |
entity_id_t | gs::gsGetEntityId (TEntityHandle hEntity) |
Get the entity's unique id. More... | |
const char * | gs::gsGetEntityName (TEntityHandle hEntity) |
Get the entity's name. More... | |
const char * | gs::gsGetEntityDescription (TEntityHandle hEntity) |
Get the entity's description. More... | |
TLicensePolicy | gs::gsGetEntityLicensePolicy (TEntityHandle hEntity) |
Get the entity's license policy (ref: LicensePolicy) More... | |
bool | gs::gsBeginAccessEntity (TEntityHandle hEntity) |
Try start accessing an entity. More... | |
bool | gs::gsEndAccessEntity (TEntityHandle hEntity) |
Try end accessing an entity. More... | |
License APIs | |
int | gs::gsGetLicenseCount (TEntityHandle hEntity) |
Gets the total number of licenses currently attached to an entity. | |
TLicenseHandle | gs::gsOpenLicenseByIndex (TEntityHandle hEntity, int index) |
Gets license object handle by its index. More... | |
TLicenseHandle | gs::gsOpenLicenseById (TEntityHandle hEntity, license_id_t licenseId) |
Gets license object handle by its id. More... | |
license_id_t | gs::gsGetLicenseId (TLicenseHandle hLicense) |
Gets the license object's license id. | |
const char * | gs::gsGetLicenseName (TLicenseHandle hLicense) |
Gets the license object's license name. | |
const char * | gs::gsGetLicenseDescription (TLicenseHandle hLicense) |
Gets the license object's license description. | |
TLicenseStatus | gs::gsGetLicenseStatus (TLicenseHandle hLicense) |
Gets the license object's license status (ref: LicenseStatus) | |
bool | gs::gsIsLicenseValid (TLicenseHandle hLicense) |
Is license currently valid? | |
TEntityHandle | gs::gsGetLicensedEntity (TLicenseHandle hLicense) |
Get the entity object to which the license is attached. | |
int | gs::gsGetLicenseParamCount (TLicenseHandle hLicense) |
Get total number of parameters in a license. | |
TVarHandle | gs::gsGetLicenseParamByIndex (TLicenseHandle hLicense, int index) |
Get the license parameter by its index, ranges [0, gsGetLicenseParamCount()-1 ]. | |
TVarHandle | gs::gsGetLicenseParamByName (TLicenseHandle hLicense, const char *name) |
Get the license parameter by its name. | |
Action APIs | |
int | gs::gsGetActionInfoCount (TLicenseHandle hLicense) |
Gets total number of actions appliable to a license Ref: ActionInfo. | |
const char * | gs::gsGetActionInfoByIndex (TLicenseHandle hLicense, int index, action_id_t *actionId) |
Gets action information by index (ref: ActionInfo) More... | |
const char * | gs::gsGetActionName (TActionHandle hAct) |
Gets action name. | |
action_id_t | gs::gsGetActionId (TActionHandle hAct) |
Gets action unique id. | |
const char * | gs::gsGetActionDescription (TActionHandle hAct) |
Gets action description. | |
const char * | gs::gsGetActionString (TActionHandle hAct) |
Gets action what-to-do string (ref: What to do action string) | |
int | gs::gsGetActionParamCount (TActionHandle hAct) |
Gets the total number of action parameters. | |
TVarHandle | gs::gsGetActionParamByName (TActionHandle hAct, const char *paramName) |
Gets action parameter by its name. More... | |
TVarHandle | gs::gsGetActionParamByIndex (TActionHandle hAct, int index) |
Gets action parameter by its index. More... | |
Variable / Parameter APIs | |
TVarHandle | gs::gsAddVariable (const char *varName, TVarType varType, int attr, const char *initValStr) |
Adds a user defined variable. More... | |
bool | gs::gsRemoveVariable (const char *varName) |
Remove a user defined variable. More... | |
TVarHandle | gs::gsGetVariable (const char *varName) |
Gets a user defined variable. More... | |
int | gs::gsGetTotalVariables () |
Get total number of user defined variables. | |
TVarHandle | gs::gsGetVariableByIndex (int index) |
Get user defined variable by its index. | |
const char * | gs::gsGetVariableName (TVarHandle hVar) |
Get variable's name. | |
TVarType | gs::gsGetVariableType (TVarHandle hVar) |
Get variable's type id (ref: varType) | |
bool | gs::gsIsVariableValid (TVarHandle hVar) |
Is variable holds a valid value? | |
const char * | gs::gsVariableTypeToString (var_type_t paramType) |
Convert variable's type id to type string (ref: varType) | |
int | gs::gsGetVariableAttr (TVarHandle hVar) |
Gets variable's attribute (ref: varAttr) | |
const char * | gs::gsVariableAttrToString (int attr, char *buf, int bufSize) |
Converts from variable's attribute value to its string format (ref: varAttr) | |
int | gs::gsVariableAttrFromString (const char *attrStr) |
Converts from variable's attribute string to attribute value (ref: varAttr) | |
const char * | gs::gsGetVariableValueAsString (TVarHandle hVar) |
Returns param value as value string. More... | |
bool | gs::gsSetVariableValueFromString (TVarHandle hVar, const char *valStr) |
Sets the variable's value from a string. More... | |
bool | gs::gsGetVariableValueAsInt (TVarHandle hVar, int &val) |
Returns param value as a 32 bit integer. More... | |
bool | gs::gsSetVariableValueFromInt (TVarHandle hVar, int val) |
Sets the variable's value from an integer. | |
bool | gs::gsGetVariableValueAsInt64 (TVarHandle hVar, int64_t &val) |
Returns param value as 64 bit integer. More... | |
bool | gs::gsSetVariableValueFromInt64 (TVarHandle hVar, int64_t val) |
Sets the variable's value from a 64 bit integer. | |
bool | gs::gsGetVariableValueAsFloat (TVarHandle hVar, float &val) |
Returns param value as float. More... | |
bool | gs::gsSetVariableValueFromFloat (TVarHandle hVar, float val) |
Sets the variable's value from a float. | |
bool | gs::gsGetVariableValueAsDouble (TVarHandle hVar, double &val) |
Returns param value as double. More... | |
bool | gs::gsSetVariableValueFromDouble (TVarHandle hVar, double val) |
Sets the variable's value from a double. | |
bool | gs::gsGetVariableValueAsTime (TVarHandle hVar, time_t &val) |
Gets the variable's value as a time_t value. More... | |
bool | gs::gsSetVariableValueFromTime (TVarHandle hVar, time_t val) |
Sets the variable's value from a time_t value. | |
Request APIs | |
TRequestHandle | gs::gsCreateRequest () |
Create a request object. | |
TActionHandle | gs::gsAddRequestAction (TRequestHandle hReq, action_id_t actId, TLicenseHandle hLic) |
Create and add an action to the request. More... | |
TActionHandle | gs::gsAddRequestActionEx (TRequestHandle hReq, action_id_t actId, const char *entityId, const char *licenseId) |
Create and add an action to the request. More... | |
const char * | gs::gsGetRequestCode (TRequestHandle hReq) |
Generates the request code from the request object. | |
bool | gs::gsApplyLicenseCode (const char *activationCode) |
Applys license code (aka. Activation Code) | |
HTML Render APIs | |
bool | gs::gsRenderHTML (const char *url, const char *title, int width, int height) |
Rendering HTML page in process. More... | |
bool | gs::gsRenderHTMLEx (const char *url, const char *title, int width, int height, bool resizable, bool exitAppWhenUIClosed, bool cleanUpAfterRendering) |
Rendering HTML with more control (Since SDK 5.0.7) More... | |
Debug Helpers | |
bool | gs::gsIsDebugVersion () |
Is the SDK binary a DEBUG version? | |
void | gs::gsTrace (const char *msg) |
Output debug message. More... | |
Application Control APIs | |
void | gs::gsExitApp (int rc) |
Exit application gracefully. | |
void | gs::gsTerminateApp (int rc) |
Exit application forcefully. | |
void | gs::gsPlayApp () |
Continue running the application. | |
void | gs::gsPauseApp () |
Pauses the application. | |
void | gs::gsResumeAndExitApp () |
Resume a paused application and quit immediately. | |
void | gs::gsRestartApp () |
Restart the current application. | |
bool | gs::gsIsRestartedApp () |
Is the current application a restarted session? | |
Session Variables APIs | |
void | gs::gsSetAppVar (const char *name, const char *val) |
Sets the value of an application session variable. | |
const char * | gs::gsGetAppVar (const char *name) |
Gets the value of an application session variable. | |
User Defined Event APIs | |
void | gs::gsPostUserEvent (unsigned int eventId, bool bSync, void *eventData, unsigned int eventDataSize) |
Post User Event. More... | |
void * | gs::gsGetUserEventData (TEventHandle hEvent, unsigned int *evtDataSize) |
Gets user defined event data information. More... | |
Virtual Machine Support | |
#define | VM_VMware 0x01 |
VMware (http://www.vmware.com/) | |
#define | VM_VirtualPC 0x02 |
Virtual PC (http://www.microsoft.com/windows/virtual-pc/) | |
#define | VM_VirtualBox 0x04 |
VirtualBox (https://www.virtualbox.org/) | |
#define | VM_Fusion 0x08 |
VMWARE Fusion. | |
#define | VM_Parallel 0x10 |
Parallels (http://www.parallels.com) | |
#define | VM_QEMU 0x20 |
QEMU (http://www.qemu.org) | |
typedef unsigned int | gs::vm_mask_t |
Virtual Machine Id Mask. | |
bool | gs::gsRunInsideVM (vm_mask_t vmask) |
User Defined Variable | |
#define | VAR_ATTR_READ 0x01 |
Variable is readable. More... | |
#define | VAR_ATTR_WRITE 0x02 |
Variable is writable. | |
#define | VAR_ATTR_PERSISTENT 0x04 |
Variable is persisted to local storage. | |
#define | VAR_ATTR_SECURE 0x08 |
Variable is secured in memory. | |
#define | VAR_ATTR_REMOTE 0x10 |
Variable is persisted at server side. | |
#define | VAR_ATTR_HIDDEN 0x20 |
Variable cannot be enumerted via apis. | |
#define | VAR_ATTR_SYSTEM 0x40 |
Variable is reserved for internal system usage. | |
enum | gs::TVarType { gs::VAR_TYPE_INT = 7, gs::VAR_TYPE_INT64 = 8, gs::VAR_TYPE_FLOAT = 9, gs::VAR_TYPE_DOUBLE = 10 , gs::VAR_TYPE_STRING = 20, gs::VAR_TYPE_TIME = 30 } |
User Defined Variable TypeId. More... | |
Detailed Description
gsCore C API Interface
These apis are flat-C style handle-based apis, OOP classes are recommended in GS5 extension development.
Macro Definition Documentation
#define EVENT_APP_BEGIN 1 |
Application just gets started, please initialize.
When this event triggers, the local license has been initialized via gsInit().
#define EVENT_ENTITY_ACCESS_ENDED 204 |
The entity is deactivated now.
The listeners can revoke any protected resources here. (remove injected decrypting keys, etc.) Licenses are kept in inactive mode.
#define EVENT_ENTITY_ACCESS_ENDING 203 |
The entity is leaving now.
The listeners can revoke any protected resources here. (remove injected decrypting keys, etc.) Licenses are still in active mode.
#define EVENT_ENTITY_ACCESS_STARTED 202 |
The entity is being accessed.
The listeners can enable any protected resources here. (inject decrypting keys, etc.) The internal licenses status have changed to active mode.
#define EVENT_ENTITY_ACTION_APPLIED 208 |
Action Applied to Entity The status of attached licenses have been modified by applying license action.
It is called after the change has been made.
#define EVENT_ENTITY_TRY_ACCESS 201 |
The entity is to be accessed.
The listeners might be able to modify the license store here. The internal licenses status are untouched. (inactive if not accessed before)
Generated on Mon Feb 3 2014 13:15:29 for GameShield V5 SDK Programming Guide by
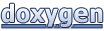