GameShield V5 SDK Programming Guide
v5.2
|
GS5 Application. More...
Public Member Functions | |
const char * | getAppRootPath () |
Gets application root directory. | |
const char * | getAppCommandLine () |
Gets application startup commandline. More... | |
const char * | getAppMainExe () |
Gets full path to the application startup Exe. More... | |
const char * | getGameTitle () |
Gets Application Title. More... | |
void | sendUserEvent (unsigned int eventId, void *eventData=NULL, unsigned int eventDataSize=0) |
Send User Defined Event (Synchronized event posting) More... | |
TGSCore * | core () |
Gets pointer to TGSCore instance. More... | |
Application Control | |
void | exitApp (int rc) |
Exits application gracefully. More... | |
void | terminateApp (int rc) |
Terminates application forcefully. More... | |
void | playApp () |
Allow the game code starts to play. More... | |
void | restartApp () |
Replay the game. More... | |
void | pauseApp () |
Pause the game [ Experimental, Windows Only ]. More... | |
void | resumeAndExitApp () |
Resume a paused game and terminate. [ Experimental, Windows Only ]. More... | |
Application Running Context Query | |
bool | isFirstPass () |
Is First Game Pass? More... | |
bool | isGamePass () |
Is First Game Pass? More... | |
bool | isLastPass () |
Is Last Pass? More... | |
bool | isFirstGameExe () |
Is the current process exe is the first game exe? More... | |
bool | isLastGameExe () |
Is the current process exe is the last game exe? More... | |
bool | isMainThread () |
Is the current thread the main thread? More... | |
bool | isRestartedApp () |
Is the current process a restarted one? More... | |
App Session Variables | |
void | setSessionVar (const char *name, const char *val) |
Write session variable. More... | |
const char * | getSessionVar (const char *name) |
Read session variable. More... | |
Protected Member Functions | |
virtual bool | OnAppInit () |
Application Initialization Callback to let the application initialize itself. More... | |
TGSApp () | |
Constructor Protected constructor to avoid creating TGSApp instance from user code directly. | |
Generic Event Handlers | |
virtual void | OnAppEvent (unsigned int evtId) |
Generic Application Events Handler. More... | |
virtual void | OnLicenseEvent (unsigned int evtId) |
Generic License Events Handler. More... | |
virtual void | OnEntityEvent (unsigned int evtId, TGSEntity *entity) |
Generic Entity Events Handler. More... | |
virtual void | OnUserEvent (unsigned int eventId, void *eventData, unsigned int eventDataSize) |
User Event Handker. More... | |
Application Event Handlers | |
virtual void | OnAppBegin () |
Application Event Handler: Game Starts (EVENT_APP_BEGIN) More... | |
virtual void | OnAppRun () |
Application Event Handler: Game Runs (EVENT_APP_RUN) More... | |
virtual void | OnAppEnd () |
Application Event Handler: Game Exits (EVENT_APP_END) More... | |
virtual void | OnClockRolledBack () |
Application Event Handler: Clock Rollback Detected (EVENT_APP_CLOCK_ROLLBACK) More... | |
virtual void | OnIntegrityCorrupted () |
Application Event Handler: Clock Rollback Detected (EVENT_APP_INTEGRITY_CORRUPT) More... | |
License Event Handlers | |
virtual void | OnNewInstall () |
License Event Handler: (EVENT_LICENSE_NEWINSTALL) More... | |
virtual void | OnLicenseLoading () |
License Event Handler: (EVENT_LICENSE_LOADING) More... | |
virtual void | OnLicenseLoaded () |
License Event Handler: (EVENT_LICENSE_READY) More... | |
virtual void | OnLicenseFail () |
License Event Handler: (EVENT_LICENSE_FAIL) More... | |
Entity Event Handlers | |
virtual void | OnEntityAccessStarting (TGSEntity *entity) |
Entity Event Handler: (EVENT_ENTITY_TRY_ACCESS) More... | |
virtual void | OnEntityAccessStarted (TGSEntity *entity) |
Entity Event Handler: (EVENT_ENTITY_ACCESS_STARTED) More... | |
virtual void | OnEntityAccessEnding (TGSEntity *entity) |
Entity Event Handler: (EVENT_ENTITY_ACCESS_ENDING) More... | |
virtual void | OnEntityAccessEnded (TGSEntity *entity) |
Entity Event Handler: (EVENT_ENTITY_ACCESS_ENDED) More... | |
virtual void | OnEntityAccessInvalid (TGSEntity *entity, bool inGame) |
Entity Event Handler: (EVENT_ENTITY_ACCESS_INVALID) More... | |
virtual void | OnEntityHeartBeat (TGSEntity *entity) |
Entity Event Handler: (EVENT_ENTITY_ACCESS_HEARTBEAT) More... | |
virtual void | OnEntityActionApplied (TGSEntity *entity) |
Entity Event Handler: (EVENT_ENTITY_ACTION_APPLIED) More... | |
Detailed Description
GS5 Application.
The base class to:
- monitor GS events;
- query and control application execution;
To monitor GS events, a subclass must override interested event handlers.
uses macro DECLARE_APP in class declaration and IMPLEMENT_APP in cpp file to register the subclass. uses macro GET_APP to retrieve the single application instance.
Member Function Documentation
|
inline |
Gets pointer to TGSCore instance.
It is a helper function retrieving the private class member _core, it is the same as TGSCore::getInstance()
void gs::TGSApp::exitApp | ( | int | rc | ) |
Exits application gracefully.
- Parameters
-
rc Exit Code
Stops the game gracefully, the LMApp will get a chance (optionally in the last different game pass for P1S2, P1S3 mode) to render its Exit-UI later.
const char * gs::TGSApp::getAppCommandLine | ( | ) |
Gets application startup commandline.
The startup exe of a Exe-Hopping game might be different from the current exe; this function returns the original command line launching the game.
const char * gs::TGSApp::getAppMainExe | ( | ) |
Gets full path to the application startup Exe.
The startup exe of a Exe-Hopping game might be different from the current exe. it is the first exe started when game launching.
Instead of hard-coding an icon, your custom LMApp may want to extract icon from game's main exe and display it in UI, which make your LM more reusable.
const char * gs::TGSApp::getGameTitle | ( | ) |
Gets Application Title.
- Returns
- The game title defined in license project file.
This is a simple helper to return TGSCore::productName(). The game title can be used in LMApp UI.
const char * gs::TGSApp::getSessionVar | ( | const char * | name | ) |
Read session variable.
Ref: AppVar
bool gs::TGSApp::isFirstGameExe | ( | ) |
Is the current process exe is the first game exe?
OnAppBegin() is called only when isFirstGameExe() returns true.
Ref: Exe-Hopping
bool gs::TGSApp::isFirstPass | ( | ) |
bool gs::TGSApp::isGamePass | ( | ) |
bool gs::TGSApp::isLastGameExe | ( | ) |
Is the current process exe is the last game exe?
OnAppEnd() is called only when isLastGameExe() returns true.
Ref: Exe-Hopping
bool gs::TGSApp::isLastPass | ( | ) |
bool gs::TGSApp::isMainThread | ( | ) |
Is the current thread the main thread?
- See Also
- OnEntityAccessInvalid
bool gs::TGSApp::isRestartedApp | ( | ) |
Is the current process a restarted one?
- See Also
- restartApp()
|
protectedvirtual |
Application Event Handler: Game Starts (EVENT_APP_BEGIN)
Called when the game starts and the license has been initialized. The default method does nothing.
LMApp can check the current license status and pop up UI if necessary. If the product has been fully activated, you might simply bypass the startup LMApp UI.
|
protectedvirtual |
Application Event Handler: Game Exits (EVENT_APP_END)
Called when the game is terminating. The default method does nothing.
The local license storage is not closed (gsCleanUp is not called) yet and it is a good chance to pops up LMApp exit UI if game not activated.
|
protectedvirtual |
Generic Application Events Handler.
- Parameters
-
evtId Application Event Identifier
The method parses the event id and invokes the corresponding event handlers. It is recommended that subclass override individual event handlers instead of this one.
- See Also
- OnAppBegin
- OnAppRun
- OnAppEnd
|
protectedvirtual |
Application Initialization Callback to let the application initialize itself.
- Returns
- TRUE if application is initialized correctly, FALSE if something fatal happens and the game will terminate immediately.
It is called after TGSCore has been initialized.
|
protectedvirtual |
Application Event Handler: Game Runs (EVENT_APP_RUN)
Called when the game's original code starts to run. The default method does nothing.
You can start timing or initialize whatever logic needed while game is running.
|
protectedvirtual |
Application Event Handler: Clock Rollback Detected (EVENT_APP_CLOCK_ROLLBACK)
Called when a clock rollback behavior has been detected. The default method does nothing.
A clock rollback might be detected if the local machine date time is modified backward manually. Some built-in License Models (LM_Expire_HardDate, LM_Expire_Period) use the local clock time, for them there is a built-in LM parameter called "rollbackTolerance" to define the maximum time difference tolerable without trigger the clock rollback event.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_ACCESS_ENDED)
Called when an entity accessing is over. (
- See Also
- gsEndAccess)
- Parameters
-
entity The entity to be accessed
If an entity will not be used any more, the gsEndAccess() will destroy its internal data structures, and before api returning it triggers this event telling you that the entity has left the "Active" status and becomes "In-Active" now.
The default method does nothing.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_ACCESS_ENDING)
Called when an entity accessing is going to an end. (
- See Also
- gsEndAccess)
- Parameters
-
entity The entity to be accessed
If an entity will not be used any more, the gsEndAccess() will destroy its internal data structures, and before api returning it triggers this event telling you that the entity is leaving the "Active" status.
It is a great chance to release any resources used by the entity. For example, the encryption keys are destroyed so that the files associated with the entity cannot be accessed by game.
The default method does nothing.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_ACCESS_INVALID)
Called when an entity accessing becomes invalid.
- Parameters
-
entity The entity to be accessed inGame If the event is triggered while game running.
For some reason, if the license attached to an active entity (entity being accessed) becomes invalid (TGSLicense::isValid() returns false), the entity triggers this error event telling you that the entity should not be accessed any more.
It is just like the car driving, the green traffic light stands for the positive license status, when license status changes to invalid at run time, you will see a red traffic light keeps flashing ahead, so the correct response is braking the car and stops as quick as possible.
The default GS5 behavior is terminating the game immediately and pops up the LMApp exit UI optionally in the last game pass (Execution mode: P1S2, P1S3). You can change the default behavior by setting one of the built-in LM's parameter "exitAppOnExpire" to false, as a result the game won't be stopped automatically by GS5 kernel.
This event handler gives you a chance to prompt the user to activate the game. However, be aware that when game running the event might be triggered from a non-main thread, rendering a UI in non-main thread is not a easy job, that is why the method has a inGame parameter, if you want to activate an entity inside the game, just do it properly, if it is ok for you to activate an entity after game terminates, inGame can make your job easier:
The default method does nothing.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_ACCESS_STARTED)
Called when an entity is accessed successfully. (
- See Also
- gsBeginAccess)
- Parameters
-
entity The entity to be accessed
If an entity is accessible, the gsBeginAccess() will initialize internal data structures for this entity, and before returning triggers this event telling you that the entity enters "Active" status.
The default method does nothing.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_TRY_ACCESS)
Called when an entity is going to be accessed. (
- See Also
- gsBeginAccess)
- Parameters
-
entity The entity to be accessed
It is a great chance to prepare all resources needed by the entity logic. If currently the entity is not activated yet, you can pop up reminder UI to prompt user activate it.
The default method does nothing.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_ACTION_APPLIED)
Called when an entity's license status is modified by an action.
- Parameters
-
entity The entity to be modified
When applying activation code, the gsApplyLicenseCode() triggers this event after the action is applied to an entity.
You can use this event handler to update the LMApp UI to reflect the latest license status.
The default method does nothing.
|
protectedvirtual |
Generic Entity Events Handler.
- Parameters
-
evtId Entity Event Identifier entity The source entity triggering this event
The method parses the event id and invokes the corresponding event handlers. It is recommended that subclass override individual event handlers instead of this one.
|
protectedvirtual |
Entity Event Handler: (EVENT_ENTITY_ACCESS_HEARTBEAT)
Called periodically while an entity is being accessed (in Active status).
- Parameters
-
entity The entity to be accessed
This event can be used as a timer.
The default method does nothing.
|
protectedvirtual |
Application Event Handler: Clock Rollback Detected (EVENT_APP_INTEGRITY_CORRUPT)
Called when a game integrity corruption has been detected. The default method does nothing.
Integrity corruption might be:
- Content of external files are modified (compared to the version when project wrapping);
- Game binaries has been modified in memory;
|
protectedvirtual |
Generic License Events Handler.
- Parameters
-
evtId License Event Identifier
The method parses the event id and invokes the corresponding event handlers. It is recommended that subclass override individual event handlers instead of this one.
|
protectedvirtual |
License Event Handler: (EVENT_LICENSE_FAIL)
Called when the game's license cannot be loaded from local storage.
It is a fatal error, and the most of gsCore apis are not usable at this point.
The reason of a license loading failure might be:
- License data corruption due to media error or logic error;
- Hard disk permission error;
GS5 has tried hard to minimize the chances of license loading failure by using redundunt storage and implementing transaction algorithm.
The default method does nothing.
|
protectedvirtual |
License Event Handler: (EVENT_LICENSE_READY)
Called when the game's license has been loaded successfully from local storage.
The default method does nothing.
|
protectedvirtual |
License Event Handler: (EVENT_LICENSE_LOADING)
Called when the game's license is being loaded from local storage.
It gives you a chance to register any used custom license models from external dlls, because the license data might contain custom LM status and the internal license model factory has to create a LM instance to parse/deserialize the custom LM data. The default method does nothing.
|
protectedvirtual |
License Event Handler: (EVENT_LICENSE_NEWINSTALL)
Called when a game launches for the first time on the local machine.
This event is triggered before OnAppBegin() when the local license storage is being initialized by gsCore::init(), It happens only once for the very first launching of the game. The default method does nothing.
|
inlineprotectedvirtual |
User Event Handker.
- Parameters
-
eventId User defined event id ( >= GS_USER_EVENT) eventData Pointer to event data buffer, NULL if no event data assoicated; eventDataSize Length of event data buffer
The subclass can override this method to handle user defined event
void gs::TGSApp::pauseApp | ( | ) |
Pause the game [ Experimental, Windows Only ].
Pauses game's thread and hide all its top windows.
It provides the License model developer a chance to pop up UI while game is running / terminating.
- See Also
- resumeAndExitApp()
void gs::TGSApp::playApp | ( | ) |
Allow the game code starts to play.
Indicates to the GS5 kernel that the game code can be executed right now.
void gs::TGSApp::restartApp | ( | ) |
Replay the game.
Restarts the game manually from your code, usually after the game has been activated in your LMApp Exit-UI.
When game restarting, you can improve game player's user experience by bypassing LMApp startup UI and goes directly to the game pass.
- See Also
- isRestartedApp()
void gs::TGSApp::resumeAndExitApp | ( | ) |
Resume a paused game and terminate. [ Experimental, Windows Only ].
- See Also
- pauseApp()
void gs::TGSApp::sendUserEvent | ( | unsigned int | eventId, |
void * | eventData = NULL , |
||
unsigned int | eventDataSize = 0 |
||
) |
Send User Defined Event (Synchronized event posting)
- Parameters
-
eventId User defined event id ( must >= GS_USER_EVENT ) eventData [Optional] data buffer pointer associated with the event, NULL if no event data eventDataSize size of event data buffer, ignored if eventData is NULL
- Returns
- none
void gs::TGSApp::setSessionVar | ( | const char * | name, |
const char * | val | ||
) |
Write session variable.
Ref: AppVar
void gs::TGSApp::terminateApp | ( | int | rc | ) |
Terminates application forcefully.
- Parameters
-
rc Exit Code
Stops the game forcefully; the game terminates immediately without giving a chance to pop up LMApp's Exit-UI,
Generated on Mon Feb 3 2014 13:15:30 for GameShield V5 SDK Programming Guide by
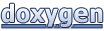