GameShield V5 SDK Programming Guide
v5.2
|
GS5 Core Object. More...
Public Member Functions | |
int | cleanUp () |
Clean up the core. More... | |
void | flush () |
Save license immediately if dirty. | |
TGSRequest * | createRequest () |
Create a request object. | |
bool | applyLicenseCode (const char *code) |
Apply license code. | |
const char * | lastErrorMessage () |
Get the last error message. | |
int | lastErrorCode () |
Get the last error code. | |
const char * | productName () |
Get product name. | |
const char * | productId () |
Get product unique id. | |
int | buildId () |
Get product Build Id (ref: Build Id) | |
void | trace (const char *msg) |
Output debug message. More... | |
const char * | revoke () |
Revokes local license. More... | |
License Initialization / Load APIs | |
bool | init (const char *productId, const char *productLic, const char *licPassword) |
One-time Initialization of gsCore. More... | |
int | loadFromLocalStorage (const char *productId, const char *password) |
Loads from Local Storage (* Read Only*) More... | |
int | loadFromLicenseFile (const char *productLic, const char *productId, const char *password) |
Loads from external license file (* Read Only*) More... | |
Entity Enumeration | |
int | getTotalEntities () |
Get total entities. | |
TGSEntity * | getEntityByIndex (int index) |
Get entity by index ( 0 <= index < getTotalEntities()-1 ) | |
TGSEntity * | getEntityById (entity_id_t entityId) |
Get entity by its unique entity id. | |
"User Defined Variables" | |
TGSVariable * | addVariable (const char *varName, TVarType varType, unsigned int attr, const char *initValStr) |
Adds a user defined variable. More... | |
bool | removeVariable (const char *varName) |
remove user defined variable by its name More... | |
int | getTotalVariables () |
Get total user defined variables. | |
TGSVariable * | getVariableByName (const char *name) |
Get user defined variable by its name. | |
TGSVariable * | getVariableByIndex (int index) |
Get user defined variable by index ( 0 <= index < getTotalVariables() ) | |
Time Engine Service | |
void | turnOnInternalTimer () |
Turn on internal timer. More... | |
void | turnOffInternalTimer () |
Turn off internaltimer. More... | |
bool | isInternalTimerActive () |
Is internal timer turned on? | |
void | tickFromExternalTimer () |
tick the core time engine from external timer More... | |
void | pauseTimeEngine () |
Pause the GS5 core time engine. More... | |
void | resumeTimeEngine () |
Resume time engine if it is previously paused. | |
bool | isTimeEngineActive () |
Is time engine currently active? ( firing events ) | |
Commonly used request code helpers | |
std::string | getUnlockRequestCode () |
Get request code to unlock the whole application ( all entities/licenses ) | |
std::string | getCleanRequestCode () |
Get request code to clean up the local license. | |
std::string | getDummyRequestCode () |
Get request code to send client information (fingerprint) to server. | |
Static Public Member Functions | |
static TGSCore * | getInstance () |
Get the single core object instance. | |
static const char * | getEventName (int eventId) |
Convert event id to human readable string, for debug purpose. More... | |
static const char * | SDKVersion () |
Get the current SDK binary version. | |
static bool | runInWrappedMode () |
Test if the current process is running inside GS5 Ironwrapper runtime. More... | |
static bool | runInVM () |
Test if the current process is runing inside any virtual machine. | |
static bool | isDebugVersion () |
Is current SDK binary a debugger version? | |
HTML Render | |
static bool | renderHTML (const char *url, const char *title, int width, int height) |
Rendering HTML page in process. More... | |
static bool | renderHTML (const char *url, const char *title, int width, int height, bool resizable, bool exitAppWhenUIClosed, bool cleanUpAfterRendering) |
Rendering HTML with more control (Since SDK 5.0.7) More... | |
Detailed Description
GS5 Core Object.
TGSCore is a singleton class to interact with GS5 runtime.
It can be retrieved by TGSCore::getInstance(), or, if you are working on a subclass of TGSApp, just refer to it via TGSApp::core() member function.
Why Core must be initialized before API calling?
The core has to be initialized ( TGSCore::init() ) successfully before most core apis can be used, except those apis that do not need access to license information therefore do not need a previous initialization, in which case they are tagged as static member functions.
However, TGSCore::renderHTML() is a special case, if the Web page is just a generic one ( normal web pages) it can render without calling TGSCore::init() first, otherwise if the Web page has content releated to current license status (via javascript gs5.js, etc.) then the TGSCore must be initialized before page rendering begins.
When Core should be initialized in my source code?
When developing GS5 extension with SDK, where should I put gsInit() or TGSCore::init() calling in my source code?
Depending on the usage of SDK, there are three senerios:
Your extension module is not wrapped at all: If you are developing a GS5 extension dll (or simply compiled in the game exe) that is not delivered in a wrapped mode, it is the developer's reponsibility to initialize the core before its apis are called.
Usually it happens if you are trying to develop simple test cases to play with the SDK apis, or develop internal utility to test or view license information, etc.
- Your extension module is wrapped but in Ironwrapper Only mode: In this case, the GS5 kernel won't get a chance to initialize itself so the developer also has to initialize the core first before most api callings are mode.
Your extension module is wrapped in GS5 IDE's Built-In or Custom license model mode:
When game loading, a GS5 system component ( gsLoader.dll on Windows ) will be embedded to orchestra the workflow of application execution mode, event broadcasting, etc. The GS5 kernel can listen to application startup events and initialize itself properly before EVENT_APP_BEGIN is fired.
If your code follows the SDK design pattern that is completely event-driven, there is no need to initialize GS5 core manually since it is already done by the system. You can call gsInit() manually in your event handlers but is totally useless and bypassed directly in GS5 core.
Member Function Documentation
TGSVariable * gs::TGSCore::addVariable | ( | const char * | varName, |
TVarType | varType, | ||
unsigned int | attr, | ||
const char * | initValStr | ||
) |
Adds a user defined variable.
- Parameters
-
varName variable name varType TVarType, ref: Variable Type attr variable attribute, ref: Variable Attribute initValStr string representation of initial variable value
- Returns
- if the variable already exists, returns the variable object, otherwise returns the the created new variable
int gs::TGSCore::cleanUp | ( | ) |
Clean up the core.
When the application is terminating, call this api to cleanup the internal data resources.
When game runs in wrapped mode, GS5 kernel will detect game exiting and flush all pending data to local license storage automatically on terminating, so this api is optional and GS5 extension developer do not have to call it manually.
For a simple non-wrapping SDK usage, this api must be called before game terminates to make sure the in-memory pending license changes are saved to local storage.
- See Also
- gsCleanUp()
|
static |
Convert event id to human readable string, for debug purpose.
It can be used to display user-friendly debug message to log view.
ref: Event Id
bool gs::TGSCore::init | ( | const char * | productId, |
const char * | productLic, | ||
const char * | licPassword | ||
) |
One-time Initialization of gsCore.
One time GS5 kernel initialize, always update local storage as needed. [Read & Write]
It tries to search and load license in the following order:
- Loads from local storage first;
- Loads from embedded license data;
- Loads from input license file;
- Parameters
-
productId The Product Unique Id. Each product has an unique Id that can be used to identity the application on the remote server. productLic The full path to the original license document. This license file is compiled from the GameShield project IDE and defines the initial license status of the product. The file will not be altered and can be deployed in any folder. licPassword The string key to decrypt the license document.
- Returns
- Returns true on success, false on error, uses lastErrorCode()/lastErrorMessage() to get the error information.
Ref:
|
inline |
Loads from external license file (* Read Only*)
As a read-only viewer, it tries to load from license file and won't update its content.
- Parameters
-
productLic The full path to the external license file. productId The Product Unique Id. Each product has an unique Id that can be used to identity the application on the remote server. password The string key to decrypt the license document.
- Returns
- Returns 0 on success, otherwise non-zero error code, calls lastErrorMessage() to get the error message.
|
inline |
Loads from Local Storage (* Read Only*)
As a read-only viewer, it tries to load from local storage only and won't update local storage.
- Parameters
-
productId The Product Unique Id. Each product has an unique Id that can be used to identity the application on the remote server. password The string key to decrypt the license document.
- Returns
- Returns 0 on success, otherwise non-zero error code, lastErrorMessage() to get the error message.
void gs::TGSCore::pauseTimeEngine | ( | ) |
Pause the GS5 core time engine.
When GS5 time engine is paused, no event can be fired.
bool gs::TGSCore::removeVariable | ( | const char * | varName | ) |
remove user defined variable by its name
- Parameters
-
varName variable name
- Returns
- true if success, false if variable not found
|
static |
Rendering HTML page in process.
- Parameters
-
url URL to html local file or web site page to render; title The caption of form window rendering the HTML page; width Pixel width of HTML page; height Pixel height of HTML page;
It can be called before init() to render generic HTML pages. However, init() must be called before to render LMApp HTML pages.
The default behavior is:
- Windows Resizable = True;
- ExitAppAfterUI = False;
- CleanUpAfterRender = False;
|
static |
Rendering HTML with more control (Since SDK 5.0.7)
- Parameters
-
url URL to html local file or web site page to render; title The caption of form window rendering the HTML page; width Pixel width of HTML page; height Pixel height of HTML page; resizable,: The HTML windows is resizable. exitAppWhenUIClosed,: Terminate current process when the HTML main windows is manually closed (clicking [x] button on right title for Windows). if false, the UI is just closed. cleanUpAfterRendering,: Clean up all of the internal rendering facilities before this API returns. If you cleanup rendering, then the possible conflicts with game are minimized, however, next time the render engine has to be re-created again. WARNING: If set to true, the Qt rendering engine might CRASH for the second time api calling due to Qt internal issue!!! So the best practice is that: Only set cleanUpAfterRendering to true if it is the last time rendering.
If you do not cleanup rendering, the render engine stays active in memory and is quick for next rendering. However, since the Qt/Win stuffs is still alive, it might conflict with game in unexpected way.(Mac: The top main menu bar, about, etc.)
const char * gs::TGSCore::revoke | ( | ) |
Revokes local license.
This api invalidates current application licenses and returns a string as a receipt.
After this api calling, the application should be terminated because all licenses have been locked down.
- Returns
- NULL if fails, non-empty string as receipt on success.
The api might fail because:
- Project license setting is not node-locked, hence no reason to lock down local license before transferring license between different machines;
- There is no already unlocked (fully pruchased) license in application, so there is no reason to lock down a demo version;
- Local license storage updating error;
|
inlinestatic |
Test if the current process is running inside GS5 Ironwrapper runtime.
It can be called before gsInit().
void gs::TGSCore::tickFromExternalTimer | ( | ) |
tick the core time engine from external timer
The external timer frequency is not necessarily 1 HZ. Using an external timer you can control the thread context in which GS events are fired.
void gs::TGSCore::trace | ( | const char * | msg | ) |
Output debug message.
For SDK/Debug version, the message is appended to the current debug log file. For SDK/Release version, the message is displayed in: (1) DebugViewer (Windows via OutputDebugString); (2) Console (Unix via printf)
void gs::TGSCore::turnOffInternalTimer | ( | ) |
Turn off internaltimer.
After TGSCore::init() is called, the internal timer is automatically turned on. if you want to use your own timer, you should turn off the internal timer first, then call tickFromExteralTimer() manually from your timer routine.
void gs::TGSCore::turnOnInternalTimer | ( | ) |
Turn on internal timer.
After TGSCore::init() is called, the internal timer is automatically turned on.
Generated on Mon Feb 3 2014 13:15:29 for GameShield V5 SDK Programming Guide by
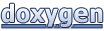