![]() |
NiTE 2.0
|
#include <NiTE.h>
Classes | |
class | NewFrameListener |
Public Member Functions | |
UserTracker () | |
~UserTracker () | |
void | addNewFrameListener (NewFrameListener *pListener) |
Status | convertDepthCoordinatesToJoint (int x, int y, int z, float *pOutX, float *pOutY) const |
Status | convertJointCoordinatesToDepth (float x, float y, float z, float *pOutX, float *pOutY) const |
Status | create (openni::Device *pDevice=NULL) |
void | destroy () |
float | getSkeletonSmoothingFactor () const |
bool | isValid () const |
Status | readFrame (UserTrackerFrameRef *pFrame) |
void | removeNewFrameListener (NewFrameListener *pListener) |
Status | setSkeletonSmoothingFactor (float factor) |
Status | startPoseDetection (UserId user, PoseType type) |
Status | startSkeletonTracking (UserId id) |
void | stopPoseDetection (UserId user, PoseType type) |
void | stopSkeletonTracking (UserId id) |
Detailed Description
This is the main object of the User Tracker algorithm. It provides access to one half of the algorithms provided by NiTE. Scene segmentation, skeleton, floor plane detection, and pose detection are all provided by this class.
The first purpose of the User Tracker algorithm is to find all of the active users in a specific scene. It individually tracks each human it finds, and provides the means to seperate their outline from each other and from the background. Once the scene has been segmented, the User Tracker is also used to initiate Skeleton Tracking and Pose Detection algorithms.
Each user is provided an ID as they are detected. The user ID remains constant as long as the user remains in the frame. If a user leaves the field of view of the camera, or tracking of that user is otherwise lost, the user may have a different ID when he is detected again. There is currently no mechanism that provides persistant recognition of individuals when they are not being actively tracking. If this functionality is desired, it will need to be implimented at the application level.
A listener class is provided to allow event based interaction with this algorithm.
- See also:
- UserMap for the output format of the User Tracker algorithm
- UserData for additional data output by this format
- Skeleton if you are also interested in tracking a user's skeleton
- NiTE for a couple of static functions that must be run before User Tracker can be used
- HandTracker for Gesture and Hand tracking algorithms.
Constructor & Destructor Documentation
nite::UserTracker::UserTracker | ( | ) | [inline] |
Default constructor. Creates an empty UserTracker with a NULL handle. This object will not be useful until the create() function is called.
- See also:
- UserTracker::create() for a function to create and activate the algorithm.
- UserTracker::isValid() to determine whether create() has already been called.
nite::UserTracker::~UserTracker | ( | ) | [inline] |
Destructor. Automatically calls the provided destroy() function.
Member Function Documentation
void nite::UserTracker::addNewFrameListener | ( | NewFrameListener * | pListener | ) | [inline] |
Adds a NewFrameListner object to this UserTracker so that it will respond when a new frame is generated.
- Parameters:
-
[in] pListener Pointer to a listener to add.
- See also:
- UserTracker::NewFrameListener for more information on using event based interaction with UserTracker
Status nite::UserTracker::convertDepthCoordinatesToJoint | ( | int | x, |
int | y, | ||
int | z, | ||
float * | pOutX, | ||
float * | pOutY | ||
) | const [inline] |
In general, two coordinate systems are used in OpenNI 2.0. These conventions are also followed in NiTE 2.0.
Skeleton joint positions are provided in "Real World" coordinates, while the native coordinate system of depth maps is the "projective" system. In short, "Real World" coordinates locate objects using a Cartesian coordinate system with the origin at the sensor. "Projective" coordinates measure straight line distance from the sensor, and indicate x/y coordinates using pixels in the image (which is mathematically equivalent to specifying angles). See the OpenNI 2.0 documentation online for more information.
This function allows you to convert the native depth map coordinates to the system used by the joints. This might be useful for performing certain types of measurements (eg distance between a joint and an object identified only in the depth map).
Note that no output is given for the Z coordinate. Z coordinates remain the same when performing the conversion. An input value is still required for Z, since this can affect the x/y output.
- Parameters:
-
[in] x The input X coordinate using the "projective" coordinate system. [in] y The input Y coordinate using the "projective" coordinate system. [in] z The input Z coordinate using the "projective" coordinate system. [out] pOutX Pointer to a location to store the output X coordinate in the "real world" system. [out] pOutY Pointer to a location to store the output Y coordinate in the "real world" system.
- Returns:
- Status indicating success or failure of this operation. This is needed because the ability to convert between coordinate systems requires a properly initalized Device from OpenNI 2.0.
Status nite::UserTracker::convertJointCoordinatesToDepth | ( | float | x, |
float | y, | ||
float | z, | ||
float * | pOutX, | ||
float * | pOutY | ||
) | const [inline] |
In general, two coordinate systems are used in OpenNI 2.0. These conventions are also followed in NiTE 2.0.
Skeleton joint positions are provided in "Real World" coordinates, while the native coordinate system of depth maps is the "projective" system. In short, "Real World" coordinates locate objects using a Cartesian coordinate system with the origin at the sensor. "Projective" coordinates measure straight line distance from the sensor (perpendicular to the sensor face), and indicate x/y coordinates using pixels in the image (which is mathematically equivalent to specifying angles). See the OpenNI 2.0 documentation online for more information.
Note that no output is given for the Z coordinate. Z coordinates remain the same when performing the conversion. An input value is still required for Z, since this can affect the x/y output.
This function allows you to convert the coordinates of a SkeletonJoint to the native coordinates of a depth map. This is useful if you need to find the joint position on the raw depth map.
- Parameters:
-
[in] x The input X coordinate using the "real world" coordinate system. [in] y The input Y coordinate using the "real world" coordinate system. [in] z The input Z coordinate using the "real world" coordinate system. [out] pOutX Pointer to a location to store the output X coordinate in the "projective" system. [out] pOutY Pointer to a location to store the output Y coordinate in the "projective" system.
- Returns:
- Status indicating success or failure of this operation. This is needed because the ability to convert between coordinate systems requires a properly initalized Device from OpenNI 2.0.
Status nite::UserTracker::create | ( | openni::Device * | pDevice = NULL | ) | [inline] |
Creates and initializes an empty User Tracker. This function should be the first one called when a new UserTracker object is constructed.
An OpenNI device with depth capabilities is required for this algorithm to work. See the OpenNI 2.0 documentation for more information about using an OpenNI 2.0 compliant hardware device and creating a Device object.
- Parameters:
-
[in] pDevice A pointer to an initalized OpenNI 2.0 Device object that provides depth streams.
- Returns:
- A status code to indicate success/failure. Since this relies on an external hardware device, it is important for applications to check this value.
- See also:
- Status enumeration for a list of all possible status values generated by this call.
void nite::UserTracker::destroy | ( | ) | [inline] |
Shuts down the user tracker and releases all resources used by it.
This is the opposite of create(). This function is called automatically by the destructor in the current implimentation, but it is good practice to run it manually when the algorithm is no longer required. Running this function more than once is safe -- it simply exits if called on a non-valid UserTracker.
float nite::UserTracker::getSkeletonSmoothingFactor | ( | ) | const [inline] |
Queries the current skeleton smoothing factor.
- Returns:
- Current skeleton smoothing factor.
- See also:
- setSkeletonSmoothingFactor for more information on the smoothing factor, and the means to change it.
bool nite::UserTracker::isValid | ( | ) | const [inline] |
Indicates whether the UserTracker is valid.
When a new UserTracker is first constructed, this function will indicate that it is invalid (ie return False). Once the create() function has been successfully called, then this function will return True. If the destroy() function is called, this function will again indicate invalid.
It is safe to run create() and destroy() without calling this function -- both of those functions already check this value and return without doing anything if no action is required.
- Returns:
- True if the UserTracker object is correctly initialized, False otherwise.
- See also:
- create() function -- causes the UserTracker to become initialized.
- destroy() function -- causes the UserTracker to become uninitialized.
Status nite::UserTracker::readFrame | ( | UserTrackerFrameRef * | pFrame | ) | [inline] |
Gets the next snapshot of the algorithm. This causes all data to be generated for the next frame of the algorithm -- algorithm frames correspond to the input depth frames used to generate them.
- Parameters:
-
pFrame [out] A pointer that will be set to point to the next frame of data.
- Returns:
- Status code indicating whether this operation was successful.
void nite::UserTracker::removeNewFrameListener | ( | NewFrameListener * | pListener | ) | [inline] |
Removes a NewFrameListener object from this UserTracker's list of listeners. The listener will no longer respond when a new frame is generated.
- Parameters:
-
[in] pListener Pointer to a listener to remove.
- See also:
- UserTracker::NewFrameListener for more information on using event based interaction with UserTracker.
Status nite::UserTracker::setSkeletonSmoothingFactor | ( | float | factor | ) | [inline] |
Control the smoothing factor of the skeleton joints. Factor should be between 0 (no smoothing at all) and 1 (no movement at all).
Experimenting with this factor should allow you to fine tune the skeleton performance. Higher values will produce smoother operation of the skeleton, but may make the skeleton feel less responsive to the user.
- Parameters:
-
[in] factor The smoothing factor.
- Returns:
- Status code indicating success or failure of this operation.
This function commands the UserTracker to start detecting specific poses for a specific user.
- Parameters:
-
[in] user The UserID of the user that you would like to detect a pose for. [in] type The type of pose you would like to detect.
- Returns:
- Status code indicating success or failure of this operation.
Requests that the Skeleton algorithm starts tracking a specific user. Once started, the skeleton will provide information on the joint position and orientation for that user during each new frame of the UserTracker.
Note that the computational requirements of calculating a skeleton increase linearly with the number of users tracked. Tracking too many users may result in poor performance and high CPU utilization. If performance slows to the point where the skeleton is not calculated at the full frame rate of the depth data used to generate it, the algorithm tends to perform poorly.
- Parameters:
-
[in] UserID The ID number of the user to calculate a skeleton for.
- Returns:
- Status code indicating success or failure of this operation.
- See also:
- nite::Skeleton for more information on the skeleton algorithm.
This function commands the pose detection algorithm to stop detecting a specific pose for a specific user. Since it is possible to detect multiple poses from multiple users, it is possible that detection of a different pose on the same user (or the same pose on a different user) may continue after this function is called.
- Parameters:
-
[in] user The UserID of the user to stop detecting a specific pose for. [in] type The PoseType of the pose to stop detecting.
void nite::UserTracker::stopSkeletonTracking | ( | UserId | id | ) | [inline] |
Stops skeleton tracking for a specific user. If multiple users are being tracked, this will only stop tracking for the user specified -- skeleton calculation will continue for remaining users.
- Parameters:
-
[in] UserID of the person to stop tracking.
- See also:
- nite::Skeleton for more information on the skeleton algorithm.
The documentation for this class was generated from the following file:
Generated on Thu Jun 6 2013 17:48:15 for NiTE 2.0 by
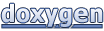