![]() |
NiTE 2.0
|
NiTE.h
Go to the documentation of this file.
00001 /******************************************************************************* 00002 * * 00003 * PrimeSense NiTE 2.0 * 00004 * Copyright (C) 2012 PrimeSense Ltd. * 00005 * * 00006 *******************************************************************************/ 00007 00008 #ifndef _NITE_H_ 00009 #define _NITE_H_ 00010 00011 #include "NiteCAPI.h" 00012 #include <OpenNI.h> 00013 00014 // Summary of use cases, modules, facades 00015 00016 namespace nite { 00017 #include "NiteEnums.h" 00018 00019 // General 00020 _NITE_DECLARE_VERSION(Version); 00021 00027 class Point3f : public NitePoint3f 00028 { 00029 public: 00033 Point3f() 00034 { 00035 x = y = z = 0.0f; 00036 } 00037 00044 Point3f(float x, float y, float z) 00045 { 00046 this->set(x, y, z); 00047 } 00048 00053 Point3f(const Point3f& other) 00054 { 00055 *this = other; 00056 } 00057 00065 void set(float x, float y, float z) 00066 { 00067 this->x = x; 00068 this->y = y; 00069 this->z = z; 00070 } 00071 00079 Point3f& operator=(const Point3f& other) 00080 { 00081 set(other.x, other.y, other.z); 00082 00083 return *this; 00084 } 00085 00092 bool operator==(const Point3f& other) const 00093 { 00094 return x == other.x && y == other.y && z == other.z; 00095 } 00096 00103 bool operator!=(const Point3f& other) const 00104 { 00105 return !operator==(other); 00106 } 00107 }; 00108 00113 class Plane : public NitePlane 00114 { 00115 public: 00121 Plane() 00122 { 00123 this->point = Point3f(); 00124 this->normal = Point3f(); 00125 } 00126 00133 Plane(const Point3f& point, const Point3f& normal) 00134 { 00135 this->point = point; 00136 this->normal = normal; 00137 } 00138 }; 00139 00144 class Quaternion : public NiteQuaternion 00145 { 00146 public: 00151 Quaternion() 00152 { 00153 x = y = z = w = 0; 00154 } 00155 00164 Quaternion(float w, float x, float y, float z) 00165 { 00166 this->x = x; 00167 this->y = y; 00168 this->z = z; 00169 this->w = w; 00170 } 00171 }; 00172 00177 class BoundingBox : public NiteBoundingBox 00178 { 00179 public: 00183 BoundingBox() 00184 {} 00185 00193 BoundingBox(const Point3f& min, const Point3f& max) 00194 { 00195 this->min = min; 00196 this->max = max; 00197 } 00198 }; 00199 00204 template <class T> 00205 class Array 00206 { 00207 public: 00211 Array() : m_size(0), m_data(NULL) {} 00219 void setData(int size, T* data) {m_data = data; m_size = size;} 00223 const T& operator[](int index) const {return m_data[index];} 00228 int getSize() const {return m_size;} 00233 bool isEmpty() const {return m_size == 0;} 00234 private: 00235 Array(const Array&); 00236 Array& operator=(const Array&); 00237 00238 int m_size; 00239 T* m_data; 00240 }; 00241 00242 // UserTracker 00247 typedef short int UserId; 00248 00259 class PoseData : protected NitePoseData 00260 { 00261 public: 00267 PoseType getType() const {return (PoseType)type;} 00268 00274 bool isHeld() const {return (state & NITE_POSE_STATE_IN_POSE) != 0;} 00275 00281 bool isEntered() const {return (state & NITE_POSE_STATE_ENTER) != 0;} 00282 00288 bool isExited() const {return (state & NITE_POSE_STATE_EXIT) != 0;} 00289 }; 00290 00307 class UserMap : private NiteUserMap 00308 { 00309 public: 00318 const UserId* getPixels() const {return pixels;} 00319 00326 int getWidth() const {return width;} 00327 00335 int getHeight() const {return height;} 00336 00344 int getStride() const {return stride;} 00345 00346 friend class UserTrackerFrameRef; 00347 }; 00348 00358 class SkeletonJoint : private NiteSkeletonJoint 00359 { 00360 public: 00368 JointType getType() const {return (JointType)jointType;} 00369 00380 const Point3f& getPosition() const {return (Point3f&)position;} 00381 00389 float getPositionConfidence() const {return positionConfidence;} 00390 00400 const Quaternion& getOrientation() const {return (Quaternion&)orientation;} 00401 00409 float getOrientationConfidence() const {return orientationConfidence;} 00410 }; 00435 class Skeleton : private NiteSkeleton 00436 { 00437 public: 00447 const SkeletonJoint& getJoint(JointType type) const {return (SkeletonJoint&)joints[type];} 00457 SkeletonState getState() const {return (SkeletonState)state;} 00458 }; 00459 00460 00472 class UserData : private NiteUserData 00473 { 00474 public: 00481 UserId getId() const {return id;} 00482 00490 const BoundingBox& getBoundingBox() const {return (const BoundingBox&)boundingBox;} 00491 00499 const Point3f& getCenterOfMass() const {return (const Point3f&)centerOfMass;} 00500 00506 bool isNew() const {return (state & NITE_USER_STATE_NEW) != 0;} 00507 00513 bool isVisible() const {return (state & NITE_USER_STATE_VISIBLE) != 0;} 00514 00522 bool isLost() const {return (state & NITE_USER_STATE_LOST) != 0;} 00523 00531 const Skeleton& getSkeleton() const {return (const Skeleton&)skeleton;} 00532 00541 const PoseData& getPose(PoseType type) const {return (const PoseData&)poses[type];} 00542 }; 00543 00554 class UserTrackerFrameRef 00555 { 00556 public: 00560 UserTrackerFrameRef() : m_pFrame(NULL), m_userTrackerHandle(NULL) 00561 {} 00562 00566 ~UserTrackerFrameRef() 00567 { 00568 release(); 00569 } 00570 00575 UserTrackerFrameRef(const UserTrackerFrameRef& other) : m_pFrame(NULL) 00576 { 00577 *this = other; 00578 } 00579 00585 UserTrackerFrameRef& operator=(const UserTrackerFrameRef& other) 00586 { 00587 setReference(other.m_userTrackerHandle, other.m_pFrame); 00588 niteUserTrackerFrameAddRef(m_userTrackerHandle, m_pFrame); 00589 00590 return *this; 00591 } 00592 00600 bool isValid() const 00601 { 00602 return m_pFrame != NULL; 00603 } 00604 00611 void release() 00612 { 00613 if (m_pFrame != NULL) 00614 { 00615 niteUserTrackerFrameRelease(m_userTrackerHandle, m_pFrame); 00616 } 00617 m_pFrame = NULL; 00618 m_userTrackerHandle = NULL; 00619 } 00620 00629 const UserData* getUserById(UserId id) const 00630 { 00631 for (int i = 0; i < m_users.getSize(); ++i) 00632 { 00633 if (m_users[i].getId() == id) 00634 { 00635 return &m_users[i]; 00636 } 00637 } 00638 return NULL; 00639 } 00640 00646 const Array<UserData>& getUsers() const {return m_users;} 00647 00655 float getFloorConfidence() const {return m_pFrame->floorConfidence;} 00656 00663 const Plane& getFloor() const {return (const Plane&)m_pFrame->floor;} 00664 00673 openni::VideoFrameRef getDepthFrame() {return m_depthFrame;} 00674 00682 const UserMap& getUserMap() const {return static_cast<const UserMap&>(m_pFrame->userMap);} 00683 00689 uint64_t getTimestamp() const {return m_pFrame->timestamp;} 00690 00698 int getFrameIndex() const {return m_pFrame->frameIndex;} 00699 private: 00700 friend class User; 00701 friend class UserTracker; 00702 00703 Array<UserData> m_users; 00704 00705 void setReference(NiteUserTrackerHandle userTrackerHandle, NiteUserTrackerFrame* pFrame) 00706 { 00707 release(); 00708 m_userTrackerHandle = userTrackerHandle; 00709 m_pFrame = pFrame; 00710 m_depthFrame._setFrame(pFrame->pDepthFrame); 00711 m_users.setData(m_pFrame->userCount, (UserData*)m_pFrame->pUser); 00712 00713 } 00714 00715 NiteUserTrackerFrame* m_pFrame; 00716 NiteUserTrackerHandle m_userTrackerHandle; 00717 openni::VideoFrameRef m_depthFrame; 00718 }; 00719 00745 class UserTracker 00746 { 00747 public: 00762 class NewFrameListener 00763 { 00764 public: 00769 NewFrameListener() : m_pUserTracker(NULL) 00770 { 00771 m_userTrackerCallbacks.readyForNextFrame = newFrameCallback; 00772 } 00773 00780 virtual void onNewFrame(UserTracker&) = 0; 00781 00782 private: 00783 NiteUserTrackerCallbacks m_userTrackerCallbacks; 00784 00785 NiteUserTrackerCallbacks& getCallbacks() {return m_userTrackerCallbacks;} 00786 00787 static void ONI_CALLBACK_TYPE newFrameCallback(void* pCookie) 00788 { 00789 NewFrameListener* pListener = (NewFrameListener*)pCookie; 00790 pListener->onNewFrame(*pListener->m_pUserTracker); 00791 } 00792 00793 00794 friend class UserTracker; 00795 void setUserTracker(UserTracker* pUserTracker) 00796 { 00797 m_pUserTracker = pUserTracker; 00798 } 00799 00800 UserTracker* m_pUserTracker; 00801 }; 00802 00810 UserTracker() : m_userTrackerHandle(NULL) 00811 {} 00812 00816 ~UserTracker() 00817 { 00818 destroy(); 00819 } 00820 00835 Status create(openni::Device* pDevice = NULL) 00836 { 00837 if (isValid()) 00838 { 00839 // tracker already active 00840 return STATUS_OUT_OF_FLOW; 00841 } 00842 00843 if (pDevice == NULL) 00844 { 00845 return (Status)niteInitializeUserTracker(&m_userTrackerHandle); 00846 } 00847 return (Status)niteInitializeUserTrackerByDevice(pDevice, &m_userTrackerHandle); 00848 } 00849 00858 void destroy() 00859 { 00860 if (isValid()) 00861 { 00862 niteShutdownUserTracker(m_userTrackerHandle); 00863 m_userTrackerHandle = NULL; 00864 } 00865 } 00866 00874 Status readFrame(UserTrackerFrameRef* pFrame) 00875 { 00876 NiteUserTrackerFrame *pNiteFrame = NULL; 00877 Status rc = (Status)niteReadUserTrackerFrame(m_userTrackerHandle, &pNiteFrame); 00878 pFrame->setReference(m_userTrackerHandle, pNiteFrame); 00879 00880 return rc; 00881 } 00882 00898 bool isValid() const 00899 { 00900 return m_userTrackerHandle != NULL; 00901 } 00902 00912 Status setSkeletonSmoothingFactor(float factor) 00913 { 00914 return (Status)niteSetSkeletonSmoothing(m_userTrackerHandle, factor); 00915 } 00916 00924 float getSkeletonSmoothingFactor() const 00925 { 00926 float factor; 00927 Status rc = (Status)niteGetSkeletonSmoothing(m_userTrackerHandle, &factor); 00928 if (rc != STATUS_OK) 00929 { 00930 factor = 0; 00931 } 00932 return factor; 00933 } 00934 00950 Status startSkeletonTracking(UserId id) 00951 { 00952 return (Status)niteStartSkeletonTracking(m_userTrackerHandle, id); 00953 } 00954 00963 void stopSkeletonTracking(UserId id) 00964 { 00965 niteStopSkeletonTracking(m_userTrackerHandle, id); 00966 } 00967 00978 Status startPoseDetection(UserId user, PoseType type) 00979 { 00980 return (Status)niteStartPoseDetection(m_userTrackerHandle, (NiteUserId)user, (NitePoseType)type); 00981 } 00982 00992 void stopPoseDetection(UserId user, PoseType type) 00993 { 00994 niteStopPoseDetection(m_userTrackerHandle, (NiteUserId)user, (NitePoseType)type); 00995 } 00996 01005 void addNewFrameListener(NewFrameListener* pListener) 01006 { 01007 niteRegisterUserTrackerCallbacks(m_userTrackerHandle, &pListener->getCallbacks(), pListener); 01008 pListener->setUserTracker(this); 01009 } 01010 01019 void removeNewFrameListener(NewFrameListener* pListener) 01020 { 01021 niteUnregisterUserTrackerCallbacks(m_userTrackerHandle, &pListener->getCallbacks()); 01022 pListener->setUserTracker(NULL); 01023 } 01024 01047 Status convertJointCoordinatesToDepth(float x, float y, float z, float* pOutX, float* pOutY) const 01048 { 01049 return (Status)niteConvertJointCoordinatesToDepth(m_userTrackerHandle, x, y, z, pOutX, pOutY); 01050 } 01051 01074 Status convertDepthCoordinatesToJoint(int x, int y, int z, float* pOutX, float* pOutY) const 01075 { 01076 return (Status)niteConvertDepthCoordinatesToJoint(m_userTrackerHandle, x, y, z, pOutX, pOutY); 01077 } 01078 01079 private: 01080 NiteUserTrackerHandle m_userTrackerHandle; 01081 }; 01082 01083 01084 // HandTracker 01085 typedef short int HandId; 01086 01100 class GestureData : protected NiteGestureData 01101 { 01102 public: 01109 GestureType getType() const {return (GestureType)type;} 01110 01115 const Point3f& getCurrentPosition() const {return (Point3f&)currentPosition;} 01116 01122 bool isComplete() const {return (state & NITE_GESTURE_STATE_COMPLETED) != 0;} 01123 01130 bool isInProgress() const {return (state & NITE_GESTURE_STATE_IN_PROGRESS) != 0;} 01131 }; 01132 01143 class HandData : protected NiteHandData 01144 { 01145 public: 01151 HandId getId() const {return id;} 01152 01158 const Point3f& getPosition() const {return (Point3f&)position;} 01159 01165 bool isNew() const {return (state & NITE_HAND_STATE_NEW) != 0;} 01166 01173 bool isLost() const {return state == NITE_HAND_STATE_LOST;} 01174 01180 bool isTracking() const {return (state & NITE_HAND_STATE_TRACKED) != 0;} 01181 01187 bool isTouchingFov() const {return (state & NITE_HAND_STATE_TOUCHING_FOV) != 0;} 01188 }; 01189 01198 class HandTrackerFrameRef 01199 { 01200 public: 01206 HandTrackerFrameRef() : m_pFrame(NULL), m_handTracker(NULL) 01207 {} 01208 01212 ~HandTrackerFrameRef() 01213 { 01214 release(); 01215 } 01216 01223 HandTrackerFrameRef(const HandTrackerFrameRef& other) : m_pFrame(NULL) 01224 { 01225 *this = other; 01226 } 01227 01233 HandTrackerFrameRef& operator=(const HandTrackerFrameRef& other) 01234 { 01235 setReference(other.m_handTracker, other.m_pFrame); 01236 niteHandTrackerFrameAddRef(m_handTracker, m_pFrame); 01237 01238 return *this; 01239 } 01240 01246 bool isValid() const 01247 { 01248 return m_pFrame != NULL; 01249 } 01250 01257 void release() 01258 { 01259 if (m_pFrame != NULL) 01260 { 01261 niteHandTrackerFrameRelease(m_handTracker, m_pFrame); 01262 } 01263 m_pFrame = NULL; 01264 m_handTracker = NULL; 01265 } 01266 01272 const Array<HandData>& getHands() const {return m_hands;} 01273 01279 const Array<GestureData>& getGestures() const {return m_gestures;} 01280 01287 openni::VideoFrameRef getDepthFrame() const 01288 { 01289 return m_depthFrame; 01290 } 01291 01297 uint64_t getTimestamp() const {return m_pFrame->timestamp;} 01298 01306 int getFrameIndex() const {return m_pFrame->frameIndex;} 01307 private: 01308 friend class HandTracker; 01309 01310 void setReference(NiteHandTrackerHandle handTracker, NiteHandTrackerFrame* pFrame) 01311 { 01312 release(); 01313 m_handTracker = handTracker; 01314 m_pFrame = pFrame; 01315 m_depthFrame._setFrame(pFrame->pDepthFrame); 01316 01317 m_hands.setData(m_pFrame->handCount, (HandData*)m_pFrame->pHands); 01318 m_gestures.setData(m_pFrame->gestureCount, (GestureData*)m_pFrame->pGestures); 01319 } 01320 01321 NiteHandTrackerFrame* m_pFrame; 01322 NiteHandTrackerHandle m_handTracker; 01323 openni::VideoFrameRef m_depthFrame; 01324 01325 Array<HandData> m_hands; 01326 Array<GestureData> m_gestures; 01327 }; 01328 01357 class HandTracker 01358 { 01359 public: 01374 class NewFrameListener 01375 { 01376 public: 01381 NewFrameListener() : m_pHandTracker(NULL) 01382 { 01383 m_handTrackerCallbacks.readyForNextFrame = newFrameCallback; 01384 } 01391 virtual void onNewFrame(HandTracker&) = 0; 01392 private: 01393 friend class HandTracker; 01394 NiteHandTrackerCallbacks m_handTrackerCallbacks; 01395 01396 NiteHandTrackerCallbacks& getCallbacks() {return m_handTrackerCallbacks;} 01397 01398 static void ONI_CALLBACK_TYPE newFrameCallback(void* pCookie) 01399 { 01400 NewFrameListener* pListener = (NewFrameListener*)pCookie; 01401 pListener->onNewFrame(*pListener->m_pHandTracker); 01402 } 01403 01404 void setHandTracker(HandTracker* pHandTracker) 01405 { 01406 m_pHandTracker = pHandTracker; 01407 } 01408 HandTracker* m_pHandTracker; 01409 }; 01410 01418 HandTracker() : m_handTrackerHandle(NULL) 01419 {} 01420 01424 ~HandTracker() 01425 { 01426 destroy(); 01427 } 01428 01443 Status create(openni::Device* pDevice = NULL) 01444 { 01445 if (isValid()) 01446 { 01447 // tracker already active 01448 return STATUS_OUT_OF_FLOW; 01449 } 01450 01451 if (pDevice == NULL) 01452 { 01453 return (Status)niteInitializeHandTracker(&m_handTrackerHandle); 01454 // Pick a device 01455 } 01456 return (Status)niteInitializeHandTrackerByDevice(pDevice, &m_handTrackerHandle); 01457 } 01458 01467 void destroy() 01468 { 01469 if (isValid()) 01470 { 01471 niteShutdownHandTracker(m_handTrackerHandle); 01472 m_handTrackerHandle = NULL; 01473 } 01474 } 01475 01483 Status readFrame(HandTrackerFrameRef* pFrame) 01484 { 01485 NiteHandTrackerFrame *pNiteFrame = NULL; 01486 Status rc = (Status)niteReadHandTrackerFrame(m_handTrackerHandle, &pNiteFrame); 01487 pFrame->setReference(m_handTrackerHandle, pNiteFrame); 01488 01489 return rc; 01490 } 01491 01507 bool isValid() const 01508 { 01509 return m_handTrackerHandle != NULL; 01510 } 01511 01520 Status setSmoothingFactor(float factor) 01521 { 01522 return (Status)niteSetHandSmoothingFactor(m_handTrackerHandle, factor); 01523 } 01524 01532 float getSmoothingFactor() const 01533 { 01534 float factor; 01535 Status rc = (Status)niteGetHandSmoothingFactor(m_handTrackerHandle, &factor); 01536 if (rc != STATUS_OK) 01537 { 01538 factor = 0; 01539 } 01540 return factor; 01541 } 01542 01562 Status startHandTracking(const Point3f& position, HandId* pNewHandId) 01563 { 01564 return (Status)niteStartHandTracking(m_handTrackerHandle, (const NitePoint3f*)&position, pNewHandId); 01565 } 01566 01573 void stopHandTracking(HandId id) 01574 { 01575 niteStopHandTracking(m_handTrackerHandle, id); 01576 } 01577 01586 void addNewFrameListener(NewFrameListener* pListener) 01587 { 01588 niteRegisterHandTrackerCallbacks(m_handTrackerHandle, &pListener->getCallbacks(), pListener); 01589 pListener->setHandTracker(this); 01590 } 01591 01600 void removeNewFrameListener(NewFrameListener* pListener) 01601 { 01602 niteUnregisterHandTrackerCallbacks(m_handTrackerHandle, &pListener->getCallbacks()); 01603 pListener->setHandTracker(NULL); 01604 } 01605 01632 Status startGestureDetection(GestureType type) 01633 { 01634 return (Status)niteStartGestureDetection(m_handTrackerHandle, (NiteGestureType)type); 01635 } 01636 01643 void stopGestureDetection(GestureType type) 01644 { 01645 niteStopGestureDetection(m_handTrackerHandle, (NiteGestureType)type); 01646 } 01647 01670 Status convertHandCoordinatesToDepth(float x, float y, float z, float* pOutX, float* pOutY) const 01671 { 01672 return (Status)niteConvertHandCoordinatesToDepth(m_handTrackerHandle, x, y, z, pOutX, pOutY); 01673 } 01674 01697 Status convertDepthCoordinatesToHand(int x, int y, int z, float* pOutX, float* pOutY) const 01698 { 01699 return (Status)niteConvertDepthCoordinatesToHand(m_handTrackerHandle, x, y, z, pOutX, pOutY); 01700 } 01701 01702 private: 01703 NiteHandTrackerHandle m_handTrackerHandle; 01704 }; 01705 01713 class NiTE 01714 { 01715 public: 01721 static Status initialize() 01722 { 01723 return (Status)niteInitialize(); 01724 } 01725 01730 static void shutdown() 01731 { 01732 niteShutdown(); 01733 } 01734 01744 static Version getVersion() 01745 { 01746 NiteVersion version = niteGetVersion(); 01747 union 01748 { 01749 NiteVersion* pC; 01750 Version* pCpp; 01751 } a; 01752 a.pC = &version; 01753 return *a.pCpp; 01754 } 01755 private: 01756 NiTE(); 01757 }; 01758 01759 } // namespace nite 01760 01761 #endif // _NITE_H_
Generated on Thu Jun 6 2013 17:48:15 for NiTE 2.0 by
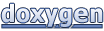