PxVehicleDrive4W Class Reference
[Vehicle]
Data structure with instanced dynamics data and configuration data of a vehicle with up to 4 driven wheels and up to 16 non-driven wheels.
More...
#include <PxVehicleDrive4W.h>
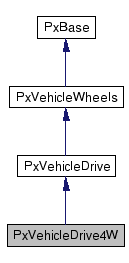
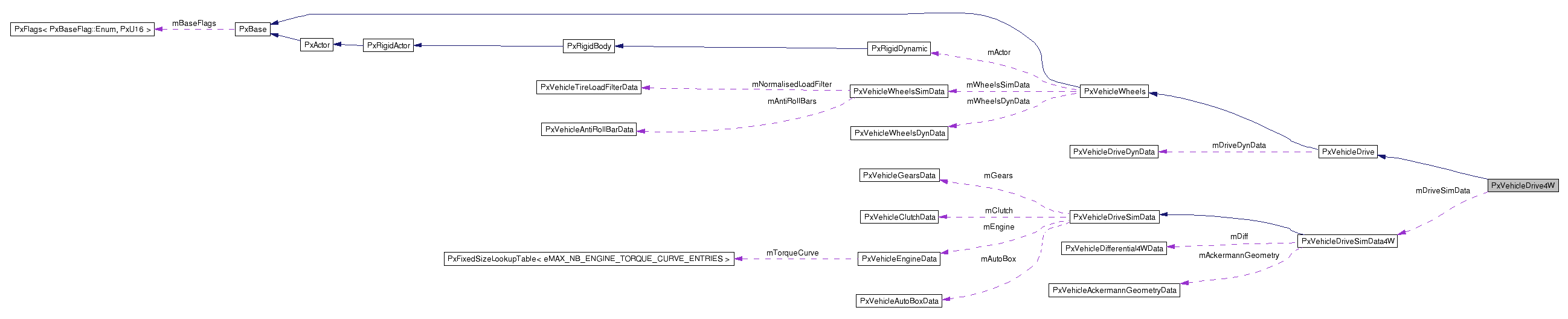
Public Member Functions | |
void | free () |
Deallocate a PxVehicleDrive4W instance. | |
void | setup (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData, const PxVehicleDriveSimData4W &driveData, const PxU32 nbNonDrivenWheels) |
Set up a vehicle using simulation data for the wheels and drive model. | |
void | setToRestState () |
Set a vehicle to its rest state. Aside from the rigid body transform, this will set the vehicle and rigid body to the state they were in immediately after setup or create. | |
PxVehicleDrive4W (PxBaseFlags baseFlags) | |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
Static Public Member Functions | |
static PxVehicleDrive4W * | allocate (const PxU32 nbWheels) |
Allocate a PxVehicleDrive4W instance for a 4WDrive vehicle with nbWheels (= 4 + number of un-driven wheels). | |
static PxVehicleDrive4W * | create (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData, const PxVehicleDriveSimData4W &driveData, const PxU32 nbNonDrivenWheels) |
Allocate and set up a vehicle using simulation data for the wheels and drive model. | |
static PxVehicleDrive4W * | createObject (PxU8 *&address, PxDeserializationContext &context) |
static void | getBinaryMetaData (PxOutputStream &stream) |
Public Attributes | |
PxVehicleDriveSimData4W | mDriveSimData |
Simulation data that describes the configuration of the vehicle's drive model. | |
Protected Member Functions | |
PxVehicleDrive4W () | |
~PxVehicleDrive4W () | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. | |
Private Member Functions | |
bool | isValid () const |
Test if the instanced dynamics and configuration data has legal values. | |
Friends | |
class | PxVehicleUpdate |
Detailed Description
Data structure with instanced dynamics data and configuration data of a vehicle with up to 4 driven wheels and up to 16 non-driven wheels.Constructor & Destructor Documentation
PxVehicleDrive4W::PxVehicleDrive4W | ( | ) | [protected] |
PxVehicleDrive4W::~PxVehicleDrive4W | ( | ) | [inline, protected] |
PxVehicleDrive4W::PxVehicleDrive4W | ( | PxBaseFlags | baseFlags | ) | [inline] |
Member Function Documentation
static PxVehicleDrive4W* PxVehicleDrive4W::allocate | ( | const PxU32 | nbWheels | ) | [static] |
Allocate a PxVehicleDrive4W instance for a 4WDrive vehicle with nbWheels (= 4 + number of un-driven wheels).
- Parameters:
-
[in] nbWheels is the number of vehicle wheels (= 4 + number of un-driven wheels)
- Returns:
- The instantiated vehicle.
static PxVehicleDrive4W* PxVehicleDrive4W::create | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData, | |||
const PxVehicleDriveSimData4W & | driveData, | |||
const PxU32 | nbNonDrivenWheels | |||
) | [static] |
Allocate and set up a vehicle using simulation data for the wheels and drive model.
- Parameters:
-
[in] physics is a PxPhysics instance that is needed to create special vehicle constraints that are maintained by the vehicle. [in] vehActor is a PxRigidDynamic instance that is used to represent the vehicle in the PhysX SDK. [in] wheelsData describes the configuration of all suspension/tires/wheels of the vehicle. The vehicle instance takes a copy of this data. [in] driveData describes the properties of the vehicle's drive model (gears/engine/clutch/differential/autobox). The vehicle instance takes a copy of this data. [in] nbNonDrivenWheels is the number of wheels on the vehicle that cannot be connected to the differential (= numWheels - 4).
- Note:
- It is assumed that the first shapes of the actor are the wheel shapes, followed by the chassis shapes. To break this assumption use PxVehicleWheelsSimData::setWheelShapeMapping.
wheelsData must contain data for at least 4 wheels. Unwanted wheels can be disabled with PxVehicleWheelsSimData::disableWheel after calling setup.
- Returns:
- The instantiated vehicle.
static PxVehicleDrive4W* PxVehicleDrive4W::createObject | ( | PxU8 *& | address, | |
PxDeserializationContext & | context | |||
) | [static] |
void PxVehicleDrive4W::free | ( | ) |
static void PxVehicleDrive4W::getBinaryMetaData | ( | PxOutputStream & | stream | ) | [static] |
Reimplemented from PxVehicleDrive.
virtual const char* PxVehicleDrive4W::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Reimplemented from PxVehicleDrive.
virtual bool PxVehicleDrive4W::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxVehicleDrive.
References PxBase::isKindOf().
bool PxVehicleDrive4W::isValid | ( | ) | const [private] |
Test if the instanced dynamics and configuration data has legal values.
Reimplemented from PxVehicleDrive.
void PxVehicleDrive4W::setToRestState | ( | ) |
Set a vehicle to its rest state. Aside from the rigid body transform, this will set the vehicle and rigid body to the state they were in immediately after setup or create.
- Note:
- Calling setToRestState invalidates the cached raycast hit planes under each wheel meaning that suspension line raycasts need to be performed at least once with PxVehicleSuspensionRaycasts before calling PxVehicleUpdates.
- See also:
- setup, create, PxVehicleSuspensionRaycasts, PxVehicleUpdates
Reimplemented from PxVehicleDrive.
void PxVehicleDrive4W::setup | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData, | |||
const PxVehicleDriveSimData4W & | driveData, | |||
const PxU32 | nbNonDrivenWheels | |||
) |
Set up a vehicle using simulation data for the wheels and drive model.
- Parameters:
-
[in] physics is a PxPhysics instance that is needed to create special vehicle constraints that are maintained by the vehicle. [in] vehActor is a PxRigidDynamic instance that is used to represent the vehicle in the PhysX SDK. [in] wheelsData describes the configuration of all suspension/tires/wheels of the vehicle. The vehicle instance takes a copy of this data. [in] driveData describes the properties of the vehicle's drive model (gears/engine/clutch/differential/autobox). The vehicle instance takes a copy of this data. [in] nbNonDrivenWheels is the number of wheels on the vehicle that cannot be connected to the differential (= numWheels - 4).
- Note:
- It is assumed that the first shapes of the actor are the wheel shapes, followed by the chassis shapes. To break this assumption use PxVehicleWheelsSimData::setWheelShapeMapping.
wheelsData must contain data for at least 4 wheels. Unwanted wheels can be disabled with PxVehicleWheelsSimData::disableWheel after calling setup.
Friends And Related Function Documentation
friend class PxVehicleUpdate [friend] |
Reimplemented from PxVehicleDrive.
Member Data Documentation
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com