![]() |
Kabylake Intel(R) Firmware Support Package (FSP) Integration Guide
|
MemInfoHob.h
Go to the documentation of this file.
160 UINT16 tFAW; ///< Number of tCK cycles for the channel DIMM's minimum four activate window delay time.
161 UINT16 tRAS; ///< Number of tCK cycles for the channel DIMM's minimum active to precharge delay time.
162 UINT16 tRCDtRP; ///< Number of tCK cycles for the channel DIMM's minimum RAS# to CAS# delay time and Row Precharge delay time.
163 UINT16 tREFI; ///< Number of tCK cycles for the channel DIMM's minimum Average Periodic Refresh Interval.
164 UINT16 tRFC; ///< Number of tCK cycles for the channel DIMM's minimum refresh recovery delay time.
165 UINT16 tRFC2; ///< Number of tCK cycles for the channel DIMM's minimum refresh recovery delay time.
166 UINT16 tRFC4; ///< Number of tCK cycles for the channel DIMM's minimum refresh recovery delay time.
167 UINT16 tRPab; ///< Number of tCK cycles for the channel DIMM's minimum row precharge delay time for all banks.
168 UINT16 tRRD; ///< Number of tCK cycles for the channel DIMM's minimum row active to row active delay time.
169 UINT16 tRRD_L; ///< Number of tCK cycles for the channel DIMM's minimum row active to row active delay time for same bank groups.
170 UINT16 tRRD_S; ///< Number of tCK cycles for the channel DIMM's minimum row active to row active delay time for different bank groups.
171 UINT16 tRTP; ///< Number of tCK cycles for the channel DIMM's minimum internal read to precharge command delay time.
173 UINT16 tWTR; ///< Number of tCK cycles for the channel DIMM's minimum internal write to read command delay time.
174 UINT16 tWTR_L; ///< Number of tCK cycles for the channel DIMM's minimum internal write to read command delay time for same bank groups.
175 UINT16 tWTR_S; ///< Number of tCK cycles for the channel DIMM's minimum internal write to read command delay time for different bank groups.
181 UINT8 DR; ///< Number of tCK cycles between transactions when switching between Ranks (in the same DIMM).
193 UINT8 ModulePartNum[20]; ///< Module part number for DDR3 is 18 bytes however for DRR4 20 bytes as per JEDEC Spec, so reserving 20 bytes
195 UINT8 SpdDramDeviceType; ///< Save SPD DramDeviceType information needed for SMBIOS structure creation.
197 UINT8 SpdModuleMemoryBusWidth; ///< Save SPD ModuleMemoryBusWidth information needed for SMBIOS structure creation.
198 UINT8 SpdSave[MAX_SPD_SAVE]; ///< Save SPD Manufacturing information needed for SMBIOS structure creation.
219 MRC_TA_TIMING tRd2Rd; ///< Deprecated and moved to CHANNEL_INFO. Read-to-Read Turn Around Timings
220 MRC_TA_TIMING tRd2Wr; ///< Deprecated and moved to CHANNEL_INFO. Read-to-Write Turn Around Timings
221 MRC_TA_TIMING tWr2Rd; ///< Deprecated and moved to CHANNEL_INFO. Write-to-Read Turn Around Timings
222 MRC_TA_TIMING tWr2Wr; ///< Deprecated and moved to CHANNEL_INFO. Write-to-Write Turn Around Timings
233 UINT16 ConfiguredMemoryClockSpeed; ///< The configured clock speed to the memory device, in megahertz (MHz)
243 UINT32 DefaultXmptCK[MAX_XMP_PROFILE_NUM];///< Stores the tCK value read from SPD XMP profiles if they exist.
244 UINT8 XmpProfileEnable; ///< If XMP capable DIMMs are detected, this will indicate which XMP Profiles are common among all DIMMs.
UINT8 SpdDramDeviceType
Save SPD DramDeviceType information needed for SMBIOS structure creation.
Definition: MemInfoHob.h:195
UINT8 SpdModuleMemoryBusWidth
Save SPD ModuleMemoryBusWidth information needed for SMBIOS structure creation.
Definition: MemInfoHob.h:197
UINT8 SpdModuleType
Save SPD ModuleType information needed for SMBIOS structure creation.
Definition: MemInfoHob.h:196
#define MAX_SPD_SAVE
Defines taken from MRC so avoid having to include MrcInterface.h.
Definition: MemInfoHob.h:88
Generated on Thu Jun 28 2018 21:44:49 for Kabylake Intel(R) Firmware Support Package (FSP) Integration Guide by
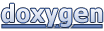