GD32F10x USB-Device
V1.0.0
GD32F10x USB-Device
|
usb_core.c
Go to the documentation of this file.
uint8_t USB_CtlTransmitStatus(USB_CORE_HANDLE *pudev)
Transmit status stage on the control pipe.
Definition: usb_core.c:570
#define _ToggleDTG_RX(EpID)
Toggle and Clear EPRX_DTG bit in the endpoint control and status register.
Definition: usb_regs.h:414
uint8_t USB_CtlContinueTx(USB_CORE_HANDLE *pudev, uint8_t *pbuf, uint16_t Len)
Continue transmitting data on the control pipe.
Definition: usb_core.c:522
void USB_EP_ClrStall(USB_CORE_HANDLE *pudev, uint8_t EpAddr)
Clear endpoint stalled status.
Definition: usb_core.c:437
#define IER_MASK
Interrupt flag mask which decide what event should be handled by application.
Definition: usb_core.h:38
Definition: usb_core.h:207
void USB_EP_Tx(USB_CORE_HANDLE *pudev, uint8_t EpAddr, uint8_t *pbuf, uint16_t BufLen)
Endpoint prepare to transmit data.
Definition: usb_core.c:346
uint8_t USB_CtlTx(USB_CORE_HANDLE *pudev, uint8_t *pbuf, uint16_t Len)
Transmit data on the control pipe.
Definition: usb_core.c:504
void USB_EP_Init(USB_CORE_HANDLE *pudev, uint8_t EpAddr, uint8_t EpType, uint16_t EpMps)
Endpoint initialization.
Definition: usb_core.c:152
void USB_EP_BufConfig(USB_CORE_HANDLE *pudev, uint8_t EpAddr, uint8_t EpKind, uint32_t BufAddr)
Configure buffer for endpoint.
Definition: usb_core.c:98
#define _Set_Status_Out(EpID)
Set and Clear directly STATUS_OUT state of endpoint.
Definition: usb_regs.h:387
#define _SetEPType(EpID, Type)
Endpoint type setting and getting(bits EP_CTL[1:0] in endpoint control and status register) ...
Definition: usb_regs.h:327
uint8_t USB_CtlReceiveStatus(USB_CORE_HANDLE *pudev)
Receive status stage on the control pipe.
Definition: usb_core.c:584
uint8_t USB_EP_GetStatus(USB_CORE_HANDLE *pudev, uint8_t EpAddr)
Get the endpoint status.
Definition: usb_core.c:485
uint16_t USB_GetRxCount(USB_CORE_HANDLE *pudev, uint8_t EpID)
Get the received data length.
Definition: usb_core.c:601
void FreeUserBuffer(uint8_t EpID, uint8_t Dir)
Free buffer used from application by toggling the SW_BUF byte.
Definition: usb_buf.c:36
#define _SetEPRxTxStatus(EpID, StateRx, StateTx)
Rx and Tx transfer status setting (bits EPRX_STA[1:0] & EPTX_STA[1:0])
Definition: usb_regs.h:367
Device Driver Header file.
#define _SetEPDoubleBuff(EpID)
Set and Clear directly double buffered feature of endpoint.
Definition: usb_regs.h:396
#define _SetEPRxStatus(EpID, State)
Rx transfer status setting and getting (bits EPRX_STA[1:0])
Definition: usb_regs.h:352
void USB_EP_Stall(USB_CORE_HANDLE *pudev, uint8_t EpAddr)
Set an endpoint to STALL status.
Definition: usb_core.c:405
void USB_EP_SetAddress(USB_CORE_HANDLE *pudev, uint8_t Addr)
Set USB device and endpoints address.
Definition: usb_core.c:465
void USB_EP_DeInit(USB_CORE_HANDLE *pudev, uint8_t EpAddr)
Configure the endpoint when it is disabled.
Definition: usb_core.c:251
#define _ToggleDTG_TX(EpID)
Toggle and Clear EPTX_DTG bit in the endpoint control and status register.
Definition: usb_regs.h:423
void USB_EP_Rx(USB_CORE_HANDLE *pudev, uint8_t EpAddr, uint8_t *pbuf, uint16_t BufLen)
Endpoint prepare to receive data.
Definition: usb_core.c:309
uint8_t USB_CtlRx(USB_CORE_HANDLE *pudev, uint8_t *pbuf, uint16_t Len)
Receive data on the control pipe.
Definition: usb_core.c:538
void UserCopyToBuffer(uint8_t *UsrBuf, uint16_t BufAddr, uint16_t Bytes)
Copy a buffer from user memory area to the allocation buffer area.
Definition: usb_buf.c:55
#define _SetEPDblBufAddr(EpID, Buf0Addr, Buf1Addr)
Sets a double buffer endpoint addresses.
Definition: usb_regs.h:567
uint8_t USB_CtlContinueRx(USB_CORE_HANDLE *pudev, uint8_t *pbuf, uint16_t Len)
Continue receive data on the contrl pipe.
Definition: usb_core.c:556
#define _SetEPTxStatus(EpID, State)
Tx transfer status setting and getting (bits EPTX_STA[1:0])
Definition: usb_regs.h:338
Generated on Fri Feb 6 2015 14:56:35 for GD32F10x USB-Device by
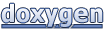