GD32F10x USB-Device
V1.0.0
GD32F10x USB-Device
|
Macros | |
#define | _SetEPType(EpID, Type) (_SetEPxCSR(EpID, ((_GetEPxCSR(EpID) & EP_CTL_MASK) | Type))) |
Endpoint type setting and getting(bits EP_CTL[1:0] in endpoint control and status register) More... | |
#define | _GetEPType(EpID) (_GetEPxCSR(EpID) & EP_CTL) |
#define | _SetEPTxStatus(EpID, State) |
Tx transfer status setting and getting (bits EPTX_STA[1:0]) More... | |
#define | _GetEPTxStatus(EpID) ((uint16_t)_GetEPxCSR(EpID) & EPTX_STA) |
#define | _SetEPRxStatus(EpID, State) |
Rx transfer status setting and getting (bits EPRX_STA[1:0]) More... | |
#define | _GetEPRxStatus(EpID) ((uint16_t)_GetEPxCSR(EpID) & EPRX_STA) |
#define | _SetEPRxTxStatus(EpID, StateRx, StateTx) |
Rx and Tx transfer status setting (bits EPRX_STA[1:0] & EPTX_STA[1:0]) More... | |
#define | _SetEP_KIND(EpID) (_SetEPxCSR(EpID, ((_GetEPxCSR(EpID) | EP_KCTL) & EPCSR_MASK))) |
Set and Clear endpoint kind (bit EP_KCTL). More... | |
#define | _ClearEP_KIND(EpID) (_SetEPxCSR(EpID, (_GetEPxCSR(EpID) & EPKCTL_MASK))) |
#define | _Set_Status_Out(EpID) _SetEP_KIND(EpID) |
Set and Clear directly STATUS_OUT state of endpoint. More... | |
#define | _Clear_Status_Out(EpID) _ClearEP_KIND(EpID) |
#define | _SetEPDoubleBuff(EpID) _SetEP_KIND(EpID) |
Set and Clear directly double buffered feature of endpoint. More... | |
#define | _ClearEPDoubleBuff(EpID) _ClearEP_KIND(EpID) |
#define | _ClearEPRX_ST(EpID) (_SetEPxCSR(EpID, _GetEPxCSR(EpID) & 0x7FFF & EPCSR_MASK)) |
Clear bit EPRX_ST / EPTX_ST in the endpoint control and status register. More... | |
#define | _ClearEPTX_ST(EpID) (_SetEPxCSR(EpID, _GetEPxCSR(EpID) & 0xFF7F & EPCSR_MASK)) |
#define | _ToggleDTG_RX(EpID) (_SetEPxCSR(EpID, EPRX_DTG | (_GetEPxCSR(EpID) & EPCSR_MASK))) |
Toggle and Clear EPRX_DTG bit in the endpoint control and status register. More... | |
#define | _ClearDTG_RX(EpID) if((_GetEPxCSR(EpID) & EPRX_DTG) != 0) _ToggleDTG_RX(EpID) |
#define | _ToggleDTG_TX(EpID) (_SetEPxCSR(EpID, EPTX_DTG | (_GetEPxCSR(EpID) & EPCSR_MASK))) |
Toggle and Clear EPTX_DTG bit in the endpoint control and status register. More... | |
#define | _ClearDTG_TX(EpID) if((_GetEPxCSR(EpID) & EPTX_DTG) != 0) _ToggleDTG_TX(EpID) |
#define | _ToggleSWBUF_TX(EpID) _ToggleDTG_RX(EpID) |
Toggle SW_BUF bit in the double buffered endpoint. More... | |
#define | _ToggleSWBUF_RX(EpID) _ToggleDTG_TX(EpID) |
#define | _SetEPAddress(EpID, Addr) _SetEPxCSR(EpID, (_GetEPxCSR(EpID) & EPCSR_MASK) | Addr) |
Set and Get endpoint address. More... | |
#define | _GetEPAddress(EpID) ((uint8_t)(_GetEPxCSR(EpID) & EP_AR)) |
#define | _GetEPTXARn(EpID) ((uint32_t *)((_GetBAR() + EpID * 8) * 2 + PBA_ADDR)) |
Get endpoint transmission buffer address. More... | |
#define | _GetEPTXCNTx(EpID) ((uint32_t *)((_GetBAR() + EpID * 8 + 2) * 2 + PBA_ADDR)) |
Get endpoint transmission byte count. More... | |
#define | _GetEPRXARn(EpID) ((uint32_t *)((_GetBAR() + EpID * 8 + 4) * 2 + PBA_ADDR)) |
Get endpoint reception buffer address. More... | |
#define | _GetEPRXCNTx(EpID) ((uint32_t *)((_GetBAR() + EpID * 8 + 6) * 2 + PBA_ADDR)) |
Get endpoint reception byte count. More... | |
#define | _SetEPTxAddr(EpID, Addr) (*_GetEPTXARn(EpID) = (Addr & ~((uint16_t)1))) |
Set Tx/Rx buffer address. More... | |
#define | _SetEPRxAddr(EpID, Addr) (*_GetEPRXARn(EpID) = (Addr & ~((uint16_t)1))) |
#define | _GetEPTxAddr(EpID) ((uint16_t)*_GetEPTXARn(EpID)) |
Get Tx/Rx buffer address. More... | |
#define | _GetEPRxAddr(EpID) ((uint16_t)*_GetEPRXARn(EpID)) |
#define | _BlocksOf32(pdwReg, Count) |
Set the reception buffer byte count register when 1 block is 32 bytes. More... | |
#define | _BlocksOf2(pdwReg, Count) |
Set the reception buffer byte count register when 1 block is 2 bytes. More... | |
#define | _SetEPRxDblBuf0Count(EpID, Count) |
Set buffer0 reception byte count when use double buffer. More... | |
#define | _SetEPTxCount(EpID, Count) (*_GetEPTXCNTx(EpID) = Count) |
Set Tx/Rx buffer byte count. More... | |
#define | _SetEPRxCount(EpID, Count) |
#define | _GetEPTxCount(EpID) ((uint16_t) (*_GetEPTXCNTx(EpID)) & EPTXCNTR_CNT) |
Get Tx/Rx buffer byte count. More... | |
#define | _GetEPRxCount(EpID) ((uint16_t) (*_GetEPRXCNTx(EpID)) & EPRXCNTR_CNT) |
#define | _SetEPDblBuf0Addr(EpID, Buf0Addr) {_SetEPTxAddr(EpID, Buf0Addr);} |
Sets buffer 0/1 address when use double buffer. More... | |
#define | _SetEPDblBuf1Addr(EpID, Buf1Addr) {_SetEPRxAddr(EpID, Buf1Addr);} |
#define | _SetEPDblBufAddr(EpID, Buf0Addr, Buf1Addr) |
Sets a double buffer endpoint addresses. More... | |
#define | _GetEPDblBuf0Addr(EpID) (_GetEPTxAddr(EpID)) |
Get a double buffer endpoint buffer 0/1 address. More... | |
#define | _GetEPDblBuf1Addr(EpID) (_GetEPRxAddr(EpID)) |
#define | _SetEPDblBuf0Count(EpID, Dir, Count) |
Set buffer 0/1 byte count register in a double buffer endpoint. More... | |
#define | _SetEPDblBuf1Count(EpID, Dir, Count) |
#define | _SetEPDblBuffCount(EpID, Dir, Count) |
#define | _GetEPDblBuf0Count(EpID) (_GetEPTxCount(EpID)) |
Get buffer 0/1 byte count. More... | |
#define | _GetEPDblBuf1Count(EpID) (_GetEPRxCount(EpID)) |
#define | _SetDouBleBuffEPStall(EpID, Dir) |
Set double buffer endpoint status to STALL. More... | |
Detailed Description
Macro Definition Documentation
#define _BlocksOf2 | ( | pdwReg, | |
Count | |||
) |
Set the reception buffer byte count register when 1 block is 2 bytes.
- Parameters
-
pdwReg reception buffer byte count register Count byte count value
- Return values
-
None
Definition at line 510 of file usb_regs.h.
#define _BlocksOf32 | ( | pdwReg, | |
Count | |||
) |
Set the reception buffer byte count register when 1 block is 32 bytes.
- Parameters
-
pdwReg reception buffer byte count register Count byte count value
- Return values
-
None
Definition at line 497 of file usb_regs.h.
#define _ClearEPRX_ST | ( | EpID | ) | (_SetEPxCSR(EpID, _GetEPxCSR(EpID) & 0x7FFF & EPCSR_MASK)) |
Clear bit EPRX_ST / EPTX_ST in the endpoint control and status register.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 405 of file usb_regs.h.
#define _GetEPDblBuf0Addr | ( | EpID | ) | (_GetEPTxAddr(EpID)) |
Get a double buffer endpoint buffer 0/1 address.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 577 of file usb_regs.h.
#define _GetEPDblBuf0Count | ( | EpID | ) | (_GetEPTxCount(EpID)) |
Get buffer 0/1 byte count.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 613 of file usb_regs.h.
#define _GetEPRXARn | ( | EpID | ) | ((uint32_t *)((_GetBAR() + EpID * 8 + 4) * 2 + PBA_ADDR)) |
Get endpoint reception buffer address.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 465 of file usb_regs.h.
#define _GetEPRXCNTx | ( | EpID | ) | ((uint32_t *)((_GetBAR() + EpID * 8 + 6) * 2 + PBA_ADDR)) |
Get endpoint reception byte count.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 472 of file usb_regs.h.
#define _GetEPTxAddr | ( | EpID | ) | ((uint16_t)*_GetEPTXARn(EpID)) |
Get Tx/Rx buffer address.
- Parameters
-
EpID endpoint identifier
- Return values
-
Address of the buffer
Definition at line 488 of file usb_regs.h.
#define _GetEPTXARn | ( | EpID | ) | ((uint32_t *)((_GetBAR() + EpID * 8) * 2 + PBA_ADDR)) |
Get endpoint transmission buffer address.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 451 of file usb_regs.h.
#define _GetEPTXCNTx | ( | EpID | ) | ((uint32_t *)((_GetBAR() + EpID * 8 + 2) * 2 + PBA_ADDR)) |
Get endpoint transmission byte count.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 458 of file usb_regs.h.
#define _GetEPTxCount | ( | EpID | ) | ((uint16_t) (*_GetEPTXCNTx(EpID)) & EPTXCNTR_CNT) |
Get Tx/Rx buffer byte count.
- Parameters
-
EpID endpoint identifier
- Return values
-
Byte count value
Definition at line 547 of file usb_regs.h.
#define _Set_Status_Out | ( | EpID | ) | _SetEP_KIND(EpID) |
Set and Clear directly STATUS_OUT state of endpoint.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 387 of file usb_regs.h.
#define _SetDouBleBuffEPStall | ( | EpID, | |
Dir | |||
) |
Set double buffer endpoint status to STALL.
- Parameters
-
EpID endpoint identifier Dir endpoint direction
- Return values
-
None
Definition at line 622 of file usb_regs.h.
#define _SetEP_KIND | ( | EpID | ) | (_SetEPxCSR(EpID, ((_GetEPxCSR(EpID) | EP_KCTL) & EPCSR_MASK))) |
Set and Clear endpoint kind (bit EP_KCTL).
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 378 of file usb_regs.h.
#define _SetEPAddress | ( | EpID, | |
Addr | |||
) | _SetEPxCSR(EpID, (_GetEPxCSR(EpID) & EPCSR_MASK) | Addr) |
Set and Get endpoint address.
- Parameters
-
EpID endpoint identifier Addr endpoint address
- Return values
-
None
Definition at line 442 of file usb_regs.h.
#define _SetEPDblBuf0Addr | ( | EpID, | |
Buf0Addr | |||
) | {_SetEPTxAddr(EpID, Buf0Addr);} |
Sets buffer 0/1 address when use double buffer.
- Parameters
-
EpID endpoint identifier Buf0Addr buffer0 address Buf1Addr buffer1 address
- Return values
-
None
Definition at line 557 of file usb_regs.h.
#define _SetEPDblBuf0Count | ( | EpID, | |
Dir, | |||
Count | |||
) |
Set buffer 0/1 byte count register in a double buffer endpoint.
- Parameters
-
EpID endpoint identifier Dir endpoint direction DBUF_EP_OUT = OUT DBUF_EP_IN = IN Count byte count value
- Return values
-
None
Definition at line 589 of file usb_regs.h.
#define _SetEPDblBuf1Count | ( | EpID, | |
Dir, | |||
Count | |||
) |
Definition at line 596 of file usb_regs.h.
#define _SetEPDblBufAddr | ( | EpID, | |
Buf0Addr, | |||
Buf1Addr | |||
) |
Sets a double buffer endpoint addresses.
- Parameters
-
EpID endpoint identifier Buf0Addr buffer0 address Buf1Addr buffer1 address
- Return values
-
None
Definition at line 567 of file usb_regs.h.
#define _SetEPDblBuffCount | ( | EpID, | |
Dir, | |||
Count | |||
) |
Definition at line 603 of file usb_regs.h.
#define _SetEPDoubleBuff | ( | EpID | ) | _SetEP_KIND(EpID) |
Set and Clear directly double buffered feature of endpoint.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 396 of file usb_regs.h.
#define _SetEPRxCount | ( | EpID, | |
Count | |||
) |
Definition at line 536 of file usb_regs.h.
#define _SetEPRxDblBuf0Count | ( | EpID, | |
Count | |||
) |
Set buffer0 reception byte count when use double buffer.
- Parameters
-
EpID endpoint identifier Count byte count value
- Return values
-
None
Definition at line 523 of file usb_regs.h.
#define _SetEPRxStatus | ( | EpID, | |
State | |||
) |
Rx transfer status setting and getting (bits EPRX_STA[1:0])
- Parameters
-
EpID endpoint identifier State new state
- Return values
-
None
Definition at line 352 of file usb_regs.h.
#define _SetEPRxTxStatus | ( | EpID, | |
StateRx, | |||
StateTx | |||
) |
Rx and Tx transfer status setting (bits EPRX_STA[1:0] & EPTX_STA[1:0])
- Parameters
-
EpID endpoint identifier StateRx new Rx state StateTx new Tx state
- Return values
-
None
Definition at line 367 of file usb_regs.h.
#define _SetEPTxAddr | ( | EpID, | |
Addr | |||
) | (*_GetEPTXARn(EpID) = (Addr & ~((uint16_t)1))) |
Set Tx/Rx buffer address.
- Parameters
-
EpID endpoint identifier Addr address to be set (must be word aligned)
- Return values
-
None
Definition at line 480 of file usb_regs.h.
#define _SetEPTxCount | ( | EpID, | |
Count | |||
) | (*_GetEPTXCNTx(EpID) = Count) |
Set Tx/Rx buffer byte count.
- Parameters
-
EpID endpoint identifier Count byte count value
- Return values
-
None
Definition at line 535 of file usb_regs.h.
#define _SetEPTxStatus | ( | EpID, | |
State | |||
) |
Tx transfer status setting and getting (bits EPTX_STA[1:0])
- Parameters
-
EpID endpoint identifier State new state
- Return values
-
None
Definition at line 338 of file usb_regs.h.
#define _SetEPType | ( | EpID, | |
Type | |||
) | (_SetEPxCSR(EpID, ((_GetEPxCSR(EpID) & EP_CTL_MASK) | Type))) |
Endpoint type setting and getting(bits EP_CTL[1:0] in endpoint control and status register)
- Parameters
-
EpID endpoint identifier Type endpoint type
- Return values
-
None
Definition at line 327 of file usb_regs.h.
#define _ToggleDTG_RX | ( | EpID | ) | (_SetEPxCSR(EpID, EPRX_DTG | (_GetEPxCSR(EpID) & EPCSR_MASK))) |
Toggle and Clear EPRX_DTG bit in the endpoint control and status register.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 414 of file usb_regs.h.
#define _ToggleDTG_TX | ( | EpID | ) | (_SetEPxCSR(EpID, EPTX_DTG | (_GetEPxCSR(EpID) & EPCSR_MASK))) |
Toggle and Clear EPTX_DTG bit in the endpoint control and status register.
- Parameters
-
EpID endpoint identifier
- Return values
-
None
Definition at line 423 of file usb_regs.h.
#define _ToggleSWBUF_TX | ( | EpID | ) | _ToggleDTG_RX(EpID) |
Toggle SW_BUF bit in the double buffered endpoint.
- Parameters
-
EpID double buffered endpoint identifier
- Return values
-
None
Definition at line 432 of file usb_regs.h.
Generated on Fri Feb 6 2015 14:56:36 for GD32F10x USB-Device by
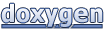