GD32F10x USB-Device
V1.0.0
GD32F10x USB-Device
|
usb_regs.h File Reference
USB cell registers definition and handle macros. More...
#include "usb_conf.h"
Go to the source code of this file.
Macros | |
#define | REG_BASE (0x40005C00L) |
#define | PBA_ADDR (0x40006000L) |
#define | CTLR ((__IO unsigned *)(REG_BASE + 0x40)) |
#define | IFR ((__IO unsigned *)(REG_BASE + 0x44)) |
#define | SR ((__IO unsigned *)(REG_BASE + 0x48)) |
#define | AR ((__IO unsigned *)(REG_BASE + 0x4C)) |
#define | BAR ((__IO unsigned *)(REG_BASE + 0x50)) |
#define | EP0CSR ((__IO unsigned *)(REG_BASE)) |
#define | EP0_OUT ((uint8_t)0x00) |
First bit is direction(0 for Rx and 1 for Tx) | |
#define | EP0_IN ((uint8_t)0x80) |
#define | EP1_OUT ((uint8_t)0x01) |
#define | EP1_IN ((uint8_t)0x81) |
#define | EP2_OUT ((uint8_t)0x02) |
#define | EP2_IN ((uint8_t)0x82) |
#define | EP3_OUT ((uint8_t)0x03) |
#define | EP3_IN ((uint8_t)0x83) |
#define | EP4_OUT ((uint8_t)0x04) |
#define | EP4_IN ((uint8_t)0x84) |
#define | EP5_OUT ((uint8_t)0x05) |
#define | EP5_IN ((uint8_t)0x85) |
#define | EP6_OUT ((uint8_t)0x06) |
#define | EP6_IN ((uint8_t)0x86) |
#define | EP7_OUT ((uint8_t)0x07) |
#define | EP7_IN ((uint8_t)0x87) |
#define | EP0 ((uint8_t)0) |
#define | EP1 ((uint8_t)1) |
#define | EP2 ((uint8_t)2) |
#define | EP3 ((uint8_t)3) |
#define | EP4 ((uint8_t)4) |
#define | EP5 ((uint8_t)5) |
#define | EP6 ((uint8_t)6) |
#define | EP7 ((uint8_t)7) |
#define | CTLR_STIE (0x8000) |
CTLR control register bits definitions. More... | |
#define | CTLR_PMOUIE (0x4000) |
#define | CTLR_ERRIE (0x2000) |
#define | CTLR_WKUPIE (0x1000) |
#define | CTLR_SPSIE (0x0800) |
#define | CTLR_RSTIE (0x0400) |
#define | CTLR_SOFIE (0x0200) |
#define | CTLR_ESOFIE (0x0100) |
#define | CTLR_RSREQ (0x0010) |
#define | CTLR_SETSPS (0x0008) |
#define | CTLR_LOWM (0x0004) |
#define | CTLR_CLOSE (0x0002) |
#define | CTLR_SETRST (0x0001) |
#define | IFR_STIF (0x8000) |
IFR interrupt events bits definitions. More... | |
#define | IFR_PMOUIF (0x4000) |
#define | IFR_ERRIF (0x2000) |
#define | IFR_WKUPIF (0x1000) |
#define | IFR_SPSIF (0x0800) |
#define | IFR_RSTIF (0x0400) |
#define | IFR_SOFIF (0x0200) |
#define | IFR_ESOFIF (0x0100) |
#define | IFR_DIR (0x0010) |
#define | IFR_EPNUM (0x000F) |
#define | CLR_STIF (~IFR_STIF) |
#define | CLR_PMOUIF (~IFR_PMOUIF) |
#define | CLR_ERRIF (~IFR_ERRIF) |
#define | CLR_WKUPIF (~IFR_WKUPIF) |
#define | CLR_SPSIF (~IFR_SPSIF) |
#define | CLR_RSTIF (~IFR_RSTIF) |
#define | CLR_SOFIF (~IFR_SOFIF) |
#define | CLR_ESOFIF (~IFR_ESOFIF) |
#define | SR_RXDP (0x8000) |
SR status register bit definitions. More... | |
#define | SR_RXDM (0x4000) |
#define | SR_LOCK (0x2000) |
#define | SR_SOFLN (0x1800) |
#define | SR_FCNT (0x07FF) |
#define | AR_USBEN (0x80) |
AR device address register bit definitions. More... | |
#define | AR_USBADDR (0x7F) |
#define | EPRX_ST (0x8000) |
EPCSR endpoint control and status register bit definitions. More... | |
#define | EPRX_DTG (0x4000) |
#define | EPRX_STA (0x3000) |
#define | EP_SETUP (0x0800) |
#define | EP_CTL (0x0600) |
#define | EP_KCTL (0x0100) |
#define | EPTX_ST (0x0080) |
#define | EPTX_DTG (0x0040) |
#define | EPTX_STA (0x0030) |
#define | EP_AR (0x000F) |
#define | EPCSR_MASK (EPRX_ST|EP_SETUP|EP_CTL|EP_KCTL|EPTX_ST|EP_AR) |
Endpoint control and status register mask (no toggle fields) | |
#define | EP_BULK (0x0000) |
EP_CTL[1:0] endpoint type control. More... | |
#define | EP_CONTROL (0x0200) |
#define | EP_ISO (0x0400) |
#define | EP_INTERRUPT (0x0600) |
#define | EP_CTL_MASK (~EP_CTL & EPCSR_MASK) |
#define | EPKCTL_MASK (~EP_KCTL & EPCSR_MASK) |
Endpoint kind control mask. | |
#define | EPTX_DISABLED (0x0000) |
TX_STA[1:0] status for Tx transfer. More... | |
#define | EPTX_STALL (0x0010) |
#define | EPTX_NAK (0x0020) |
#define | EPTX_VALID (0x0030) |
#define | EPTX_DTGMASK (EPTX_STA | EPCSR_MASK) |
#define | EPRX_DISABLED (0x0000) |
RX_STA[1:0] status for Rx transfer. More... | |
#define | EPRX_STALL (0x1000) |
#define | EPRX_NAK (0x2000) |
#define | EPRX_VALID (0x3000) |
#define | EPRX_DTGMASK (EPRX_STA | EPCSR_MASK) |
#define | EPRXCNTR_BLKSIZ (0x8000) |
Endpoint receive/transmission counter register bit definitions. | |
#define | EPRXCNTR_BLKNUM (0x7C00) |
#define | EPRXCNTR_CNT (0x03FF) |
#define | EPTXCNTR_CNT (0x03FF) |
#define | BLKSIZE_OFFSET (0x01) |
Endpoint receive/transmission counter register bit offset. | |
#define | BLKNUM_OFFSET (0x05) |
#define | RXCNT_OFFSET (0x0A) |
#define | TXCNT_OFFSET (0x0A) |
#define | BLKSIZE32_MASK (0x1f) |
#define | BLKSIZE2_MASK (0x01) |
#define | BLKSIZE32_OFFSETMASK (0x05) |
#define | BLKSIZE2_OFFSETMASK (0x01) |
#define | _SetCTLR(RegValue) (*CTLR = (uint16_t)RegValue) |
#define | _GetCTLR() ((uint16_t) *CTLR) |
#define | _SetIFR(RegValue) (*IFR = (uint16_t)RegValue) |
#define | _GetIFR() ((uint16_t) *IFR) |
#define | _SetAR(RegValue) (*AR = (uint16_t)RegValue) |
#define | _GetAR() ((uint16_t) *AR) |
#define | _SetBAR(RegValue) (*BAR = (uint16_t)(RegValue & 0xFFF8)) |
#define | _GetBAR() ((uint16_t) *BAR) |
#define | _GetSR() ((uint16_t) *SR) |
#define | _SetEPxCSR(EpID, RegValue) (*(EP0CSR + EpID) = (uint16_t)RegValue) |
#define | _GetEPxCSR(EpID) ((uint16_t)(*(EP0CSR + EpID))) |
#define | _SetEPType(EpID, Type) (_SetEPxCSR(EpID, ((_GetEPxCSR(EpID) & EP_CTL_MASK) | Type))) |
Endpoint type setting and getting(bits EP_CTL[1:0] in endpoint control and status register) More... | |
#define | _GetEPType(EpID) (_GetEPxCSR(EpID) & EP_CTL) |
#define | _SetEPTxStatus(EpID, State) |
Tx transfer status setting and getting (bits EPTX_STA[1:0]) More... | |
#define | _GetEPTxStatus(EpID) ((uint16_t)_GetEPxCSR(EpID) & EPTX_STA) |
#define | _SetEPRxStatus(EpID, State) |
Rx transfer status setting and getting (bits EPRX_STA[1:0]) More... | |
#define | _GetEPRxStatus(EpID) ((uint16_t)_GetEPxCSR(EpID) & EPRX_STA) |
#define | _SetEPRxTxStatus(EpID, StateRx, StateTx) |
Rx and Tx transfer status setting (bits EPRX_STA[1:0] & EPTX_STA[1:0]) More... | |
#define | _SetEP_KIND(EpID) (_SetEPxCSR(EpID, ((_GetEPxCSR(EpID) | EP_KCTL) & EPCSR_MASK))) |
Set and Clear endpoint kind (bit EP_KCTL). More... | |
#define | _ClearEP_KIND(EpID) (_SetEPxCSR(EpID, (_GetEPxCSR(EpID) & EPKCTL_MASK))) |
#define | _Set_Status_Out(EpID) _SetEP_KIND(EpID) |
Set and Clear directly STATUS_OUT state of endpoint. More... | |
#define | _Clear_Status_Out(EpID) _ClearEP_KIND(EpID) |
#define | _SetEPDoubleBuff(EpID) _SetEP_KIND(EpID) |
Set and Clear directly double buffered feature of endpoint. More... | |
#define | _ClearEPDoubleBuff(EpID) _ClearEP_KIND(EpID) |
#define | _ClearEPRX_ST(EpID) (_SetEPxCSR(EpID, _GetEPxCSR(EpID) & 0x7FFF & EPCSR_MASK)) |
Clear bit EPRX_ST / EPTX_ST in the endpoint control and status register. More... | |
#define | _ClearEPTX_ST(EpID) (_SetEPxCSR(EpID, _GetEPxCSR(EpID) & 0xFF7F & EPCSR_MASK)) |
#define | _ToggleDTG_RX(EpID) (_SetEPxCSR(EpID, EPRX_DTG | (_GetEPxCSR(EpID) & EPCSR_MASK))) |
Toggle and Clear EPRX_DTG bit in the endpoint control and status register. More... | |
#define | _ClearDTG_RX(EpID) if((_GetEPxCSR(EpID) & EPRX_DTG) != 0) _ToggleDTG_RX(EpID) |
#define | _ToggleDTG_TX(EpID) (_SetEPxCSR(EpID, EPTX_DTG | (_GetEPxCSR(EpID) & EPCSR_MASK))) |
Toggle and Clear EPTX_DTG bit in the endpoint control and status register. More... | |
#define | _ClearDTG_TX(EpID) if((_GetEPxCSR(EpID) & EPTX_DTG) != 0) _ToggleDTG_TX(EpID) |
#define | _ToggleSWBUF_TX(EpID) _ToggleDTG_RX(EpID) |
Toggle SW_BUF bit in the double buffered endpoint. More... | |
#define | _ToggleSWBUF_RX(EpID) _ToggleDTG_TX(EpID) |
#define | _SetEPAddress(EpID, Addr) _SetEPxCSR(EpID, (_GetEPxCSR(EpID) & EPCSR_MASK) | Addr) |
Set and Get endpoint address. More... | |
#define | _GetEPAddress(EpID) ((uint8_t)(_GetEPxCSR(EpID) & EP_AR)) |
#define | _GetEPTXARn(EpID) ((uint32_t *)((_GetBAR() + EpID * 8) * 2 + PBA_ADDR)) |
Get endpoint transmission buffer address. More... | |
#define | _GetEPTXCNTx(EpID) ((uint32_t *)((_GetBAR() + EpID * 8 + 2) * 2 + PBA_ADDR)) |
Get endpoint transmission byte count. More... | |
#define | _GetEPRXARn(EpID) ((uint32_t *)((_GetBAR() + EpID * 8 + 4) * 2 + PBA_ADDR)) |
Get endpoint reception buffer address. More... | |
#define | _GetEPRXCNTx(EpID) ((uint32_t *)((_GetBAR() + EpID * 8 + 6) * 2 + PBA_ADDR)) |
Get endpoint reception byte count. More... | |
#define | _SetEPTxAddr(EpID, Addr) (*_GetEPTXARn(EpID) = (Addr & ~((uint16_t)1))) |
Set Tx/Rx buffer address. More... | |
#define | _SetEPRxAddr(EpID, Addr) (*_GetEPRXARn(EpID) = (Addr & ~((uint16_t)1))) |
#define | _GetEPTxAddr(EpID) ((uint16_t)*_GetEPTXARn(EpID)) |
Get Tx/Rx buffer address. More... | |
#define | _GetEPRxAddr(EpID) ((uint16_t)*_GetEPRXARn(EpID)) |
#define | _BlocksOf32(pdwReg, Count) |
Set the reception buffer byte count register when 1 block is 32 bytes. More... | |
#define | _BlocksOf2(pdwReg, Count) |
Set the reception buffer byte count register when 1 block is 2 bytes. More... | |
#define | _SetEPRxDblBuf0Count(EpID, Count) |
Set buffer0 reception byte count when use double buffer. More... | |
#define | _SetEPTxCount(EpID, Count) (*_GetEPTXCNTx(EpID) = Count) |
Set Tx/Rx buffer byte count. More... | |
#define | _SetEPRxCount(EpID, Count) |
#define | _GetEPTxCount(EpID) ((uint16_t) (*_GetEPTXCNTx(EpID)) & EPTXCNTR_CNT) |
Get Tx/Rx buffer byte count. More... | |
#define | _GetEPRxCount(EpID) ((uint16_t) (*_GetEPRXCNTx(EpID)) & EPRXCNTR_CNT) |
#define | _SetEPDblBuf0Addr(EpID, Buf0Addr) {_SetEPTxAddr(EpID, Buf0Addr);} |
Sets buffer 0/1 address when use double buffer. More... | |
#define | _SetEPDblBuf1Addr(EpID, Buf1Addr) {_SetEPRxAddr(EpID, Buf1Addr);} |
#define | _SetEPDblBufAddr(EpID, Buf0Addr, Buf1Addr) |
Sets a double buffer endpoint addresses. More... | |
#define | _GetEPDblBuf0Addr(EpID) (_GetEPTxAddr(EpID)) |
Get a double buffer endpoint buffer 0/1 address. More... | |
#define | _GetEPDblBuf1Addr(EpID) (_GetEPRxAddr(EpID)) |
#define | _SetEPDblBuf0Count(EpID, Dir, Count) |
Set buffer 0/1 byte count register in a double buffer endpoint. More... | |
#define | _SetEPDblBuf1Count(EpID, Dir, Count) |
#define | _SetEPDblBuffCount(EpID, Dir, Count) |
#define | _GetEPDblBuf0Count(EpID) (_GetEPTxCount(EpID)) |
Get buffer 0/1 byte count. More... | |
#define | _GetEPDblBuf1Count(EpID) (_GetEPRxCount(EpID)) |
#define | _SetDouBleBuffEPStall(EpID, Dir) |
Set double buffer endpoint status to STALL. More... | |
Typedefs | |
typedef enum _DBUF_EP_DIR | DBUF_EP_DIR |
USB double buffer endpoint direction. | |
Enumerations | |
enum | USB_SPEED { USB_SPEED_LOW, USB_SPEED_FULL } |
USB device speed. | |
enum | _DBUF_EP_DIR { DBUF_EP_IN, DBUF_EP_OUT, DBUF_EP_ERR } |
USB double buffer endpoint direction. | |
Detailed Description
USB cell registers definition and handle macros.
- Author
- MCU SD
- Version
- V1.0.0
- Date
- 26-Dec-2014
Definition in file usb_regs.h.
Generated on Fri Feb 6 2015 14:56:36 for GD32F10x USB-Device by
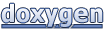