STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_ts.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_ts.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32l4r9i_eval_ts.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L4R9I_EVAL_TS_H 00039 #define __STM32L4R9I_EVAL_TS_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32l4r9i_eval.h" 00047 #include "stm32l4r9i_eval_lcd.h" 00048 #include "stm32l4r9i_eval_io.h" 00049 00050 /* Include TouchScreen component driver */ 00051 #if defined(USE_GVO_390x390) 00052 /* Include touch screen FT3X67 component Driver */ 00053 #include "../Components/ft3x67/ft3x67.h" 00054 #endif 00055 00056 #if defined(USE_ROCKTECH_480x272) 00057 /* Include touch screen FT5336 component Driver */ 00058 #include "../Components/ft5336/ft5336.h" 00059 #endif 00060 00061 /** @addtogroup BSP 00062 * @{ 00063 */ 00064 00065 /** @addtogroup STM32L4R9I_EVAL 00066 * @{ 00067 */ 00068 00069 /** @defgroup STM32L4R9I_EVAL_TS STM32L4R9I_EVAL TS 00070 * @{ 00071 */ 00072 00073 /** @defgroup STM32L4R9I_EVAL_TS_Exported_Constants Exported Constants 00074 * @{ 00075 */ 00076 #if defined(USE_GVO_390x390) 00077 /** @brief With FT3X67 : maximum 2 touches detected simultaneously 00078 */ 00079 #define TS_MAX_NB_TOUCH ((uint32_t) FT3X67_MAX_DETECTABLE_TOUCH) 00080 #endif 00081 00082 #if defined(USE_ROCKTECH_480x272) 00083 /** @brief With FT5336 : maximum 5 touches detected simultaneously 00084 */ 00085 #define TS_MAX_NB_TOUCH ((uint32_t) FT5336_MAX_DETECTABLE_TOUCH) 00086 #endif 00087 00088 #define TS_NO_IRQ_PENDING ((uint8_t) 0) 00089 #define TS_IRQ_PENDING ((uint8_t) 1) 00090 00091 #define TS_SWAP_NONE ((uint8_t) 0x01) 00092 #define TS_SWAP_X ((uint8_t) 0x02) 00093 #define TS_SWAP_Y ((uint8_t) 0x04) 00094 #define TS_SWAP_XY ((uint8_t) 0x08) 00095 00096 #define TS_ORIENTATION_PORTRAIT ((uint8_t) 0) 00097 #define TS_ORIENTATION_LANDSCAPE ((uint8_t) 1) 00098 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32L4R9I_EVAL_TS_Exported_Types Exported Types 00104 * @{ 00105 */ 00106 /** 00107 * @brief TS_StateTypeDef 00108 * Define TS State structure 00109 */ 00110 typedef struct 00111 { 00112 uint8_t touchDetected; /*!< Total number of active touches detected at last scan */ 00113 uint16_t touchX[TS_MAX_NB_TOUCH]; /*!< Touch X[0], X[1] coordinates on 12 bits */ 00114 uint16_t touchY[TS_MAX_NB_TOUCH]; /*!< Touch Y[0], Y[1] coordinates on 12 bits */ 00115 uint8_t touchWeight[TS_MAX_NB_TOUCH]; /*!< Touch_Weight[0], Touch_Weight[1] : weight property of touches */ 00116 uint8_t touchEventId[TS_MAX_NB_TOUCH]; /*!< Touch_EventId[0], Touch_EventId[1] : take value of type @ref TS_TouchEventTypeDef */ 00117 uint8_t touchArea[TS_MAX_NB_TOUCH]; /*!< Touch_Area[0], Touch_Area[1] : touch area of each touch */ 00118 uint32_t gestureId; /*!< type of gesture detected : take value of type @ref TS_GestureIdTypeDef */ 00119 } TS_StateTypeDef; 00120 00121 /** 00122 * @brief TS_StatusTypeDef 00123 * Define BSP_TS_xxx() functions possible return value, 00124 * when status is returned by those functions. 00125 */ 00126 typedef enum 00127 { 00128 TS_OK = 0x00, /*!< Touch Ok */ 00129 TS_ERROR = 0x01, /*!< Touch Error */ 00130 TS_TIMEOUT = 0x02, /*!< Touch Timeout */ 00131 TS_DEVICE_NOT_FOUND = 0x03 /*!< Touchscreen device not found */ 00132 } TS_StatusTypeDef; 00133 00134 /** 00135 * @brief TS_GestureIdTypeDef 00136 * Define Possible managed gesture identification values returned by touch screen 00137 * driver. 00138 */ 00139 typedef enum 00140 { 00141 GEST_ID_NO_GESTURE = 0x00, /*!< Gesture not defined / recognized */ 00142 GEST_ID_MOVE_UP = 0x01, /*!< Gesture Move Up */ 00143 GEST_ID_MOVE_RIGHT = 0x02, /*!< Gesture Move Right */ 00144 GEST_ID_MOVE_DOWN = 0x03, /*!< Gesture Move Down */ 00145 GEST_ID_MOVE_LEFT = 0x04, /*!< Gesture Move Left */ 00146 GEST_ID_ZOOM_IN = 0x05, /*!< Gesture Zoom In */ 00147 GEST_ID_ZOOM_OUT = 0x06, /*!< Gesture Zoom Out */ 00148 GEST_ID_SINGLE_CLICK = 0x07, /*!< Gesture Single Click */ 00149 GEST_ID_DOUBLE_CLICK = 0x08, /*!< Gesture Double Click */ 00150 GEST_ID_NB_MAX = 0x09 /*!< max number of gesture id */ 00151 } TS_GestureIdTypeDef; 00152 00153 /** 00154 * @brief TS_TouchEventTypeDef 00155 * Define Possible touch events kind as returned values 00156 * by touch screen IC Driver. 00157 */ 00158 typedef enum 00159 { 00160 TOUCH_EVENT_NO_EVT = 0x00, /*!< Touch Event : undetermined */ 00161 TOUCH_EVENT_PRESS_DOWN = 0x01, /*!< Touch Event Press Down */ 00162 TOUCH_EVENT_LIFT_UP = 0x02, /*!< Touch Event Lift Up */ 00163 TOUCH_EVENT_CONTACT = 0x03, /*!< Touch Event Contact */ 00164 TOUCH_EVENT_NB_MAX = 0x04 /*!< max number of touch events kind */ 00165 } TS_TouchEventTypeDef; 00166 00167 /** 00168 * @} 00169 */ 00170 00171 /** @defgroup STM32L4R9I_EVAL_TS_Exported_Functions Exported Functions 00172 * @{ 00173 */ 00174 00175 #if defined(USE_GVO_390x390) 00176 00177 #include "stm32l4r9i_eval_dsi_ts.h" 00178 00179 #define BSP_TS_Init BSP_DSI_TS_Init 00180 #define BSP_TS_DeInit BSP_DSI_TS_DeInit 00181 00182 #define BSP_TS_GetState BSP_DSI_TS_GetState 00183 #define BSP_TS_GestureConfig BSP_DSI_TS_GestureConfig 00184 #define BSP_TS_Get_GestureId BSP_DSI_TS_Get_GestureId 00185 #define BSP_TS_ResetTouchData BSP_DSI_TS_ResetTouchData 00186 00187 #define BSP_TS_ITConfig BSP_DSI_TS_ITConfig 00188 #define BSP_TS_ITGetStatus BSP_DSI_TS_ITGetStatus 00189 #define BSP_TS_ITClear BSP_DSI_TS_ITClear 00190 00191 #endif /* USE_GVO_390x390 */ 00192 00193 00194 #if defined(USE_ROCKTECH_480x272) 00195 00196 #include "stm32l4r9i_eval_rgb_ts.h" 00197 00198 #define BSP_TS_Init BSP_RGB_TS_Init 00199 #define BSP_TS_DeInit BSP_RGB_TS_DeInit 00200 00201 #define BSP_TS_GetState BSP_RGB_TS_GetState 00202 #define BSP_TS_Get_GestureId BSP_RGB_TS_Get_GestureId 00203 #define BSP_TS_ResetTouchData BSP_RGB_TS_ResetTouchData 00204 00205 #define BSP_TS_ITConfig BSP_RGB_TS_ITConfig 00206 #define BSP_TS_ITGetStatus BSP_RGB_TS_ITGetStatus 00207 #define BSP_TS_ITClear BSP_RGB_TS_ITClear 00208 00209 #endif /* USE_ROCKTECH_480x272 */ 00210 00211 /** 00212 * @} 00213 */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 /** 00220 * @} 00221 */ 00222 00223 /** 00224 * @} 00225 */ 00226 00227 00228 #ifdef __cplusplus 00229 } 00230 #endif 00231 00232 #endif /* __STM32L4R9I_EVAL_TS_H */ 00233 00234 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
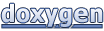