STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_idd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_idd.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage the 00006 * Idd measurement driver for STM32L4R9I_EVAL board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l4r9i_eval_idd.h" 00039 #include "stm32l4r9i_eval_io.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32L4R9I_EVAL 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32L4R9I_EVAL_IDD STM32L4R9I_EVAL IDD 00050 * @brief This file includes the Idd driver for STM32L4R9I_EVAL board. 00051 * It allows user to measure MCU Idd current on board, especially in 00052 * different low power modes. 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32L4R9I_EVAL_IDD_Private_Variables Private Variables 00057 * @{ 00058 */ 00059 static IDD_DrvTypeDef *IddDrv = NULL; 00060 00061 /** 00062 * @} 00063 */ 00064 00065 /** @defgroup STM32L4R9I_EVAL_IDD_Exported_Functions Exported Functions 00066 * @{ 00067 */ 00068 00069 /** 00070 * @brief Configures IDD measurement component. 00071 * @retval IDD_OK if no problem during initialization 00072 */ 00073 uint8_t BSP_IDD_Init(void) 00074 { 00075 IDD_ConfigTypeDef iddconfig = {0}; 00076 uint8_t mfxstm32l152_id = 0; 00077 uint8_t ret = 0; 00078 00079 if (BSP_IO_Init() == IO_ERROR) 00080 { 00081 BSP_ErrorHandler(); 00082 } 00083 00084 /* wake up mfx component in case it went to standby mode */ 00085 mfxstm32l152_idd_drv.WakeUp(IDD_I2C_ADDRESS); 00086 HAL_Delay(5); 00087 00088 /* Read ID and verify if the MFX is ready */ 00089 mfxstm32l152_id = mfxstm32l152_idd_drv.ReadID(IDD_I2C_ADDRESS); 00090 00091 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00092 { 00093 /* Initialize the Idd driver structure */ 00094 IddDrv = &mfxstm32l152_idd_drv; 00095 00096 /* Initialize the Idd driver */ 00097 if(IddDrv->Init != NULL) 00098 { 00099 IddDrv->Init(IDD_I2C_ADDRESS); 00100 } 00101 00102 /* Configure Idd component with default values */ 00103 iddconfig.AmpliGain = EVAL_IDD_AMPLI_GAIN; 00104 iddconfig.VddMin = EVAL_IDD_VDD_MIN; 00105 iddconfig.Shunt0Value = EVAL_IDD_SHUNT0_VALUE; 00106 iddconfig.Shunt1Value = EVAL_IDD_SHUNT1_VALUE; 00107 iddconfig.Shunt2Value = EVAL_IDD_SHUNT2_VALUE; 00108 iddconfig.Shunt3Value = 0; 00109 iddconfig.Shunt4Value = EVAL_IDD_SHUNT4_VALUE; 00110 iddconfig.Shunt0StabDelay = EVAL_IDD_SHUNT0_STABDELAY; 00111 iddconfig.Shunt1StabDelay = EVAL_IDD_SHUNT1_STABDELAY; 00112 iddconfig.Shunt2StabDelay = EVAL_IDD_SHUNT2_STABDELAY; 00113 iddconfig.Shunt3StabDelay = 0; 00114 iddconfig.Shunt4StabDelay = EVAL_IDD_SHUNT4_STABDELAY; 00115 iddconfig.ShuntNbOnBoard = MFXSTM32L152_IDD_SHUNT_NB_4; 00116 iddconfig.ShuntNbUsed = MFXSTM32L152_IDD_SHUNT_NB_4; 00117 iddconfig.VrefMeasurement = MFXSTM32L152_IDD_VREF_AUTO_MEASUREMENT_ENABLE; 00118 iddconfig.Calibration = MFXSTM32L152_IDD_AUTO_CALIBRATION_ENABLE; 00119 iddconfig.PreDelayUnit = MFXSTM32L152_IDD_PREDELAY_20_MS; 00120 iddconfig.PreDelayValue = 0x7F; 00121 iddconfig.MeasureNb = 100; 00122 iddconfig.DeltaDelayUnit= MFXSTM32L152_IDD_DELTADELAY_0_5_MS; 00123 iddconfig.DeltaDelayValue = 10; 00124 BSP_IDD_Config(iddconfig); 00125 00126 ret = IDD_OK; 00127 } 00128 else 00129 { 00130 ret = IDD_ERROR; 00131 } 00132 00133 return ret; 00134 } 00135 00136 /** 00137 * @brief Unconfigures IDD measurement component. 00138 * @retval IDD_OK if no problem during deinitialization 00139 */ 00140 void BSP_IDD_DeInit(void) 00141 { 00142 if(IddDrv->DeInit!= NULL) 00143 { 00144 IddDrv->DeInit(IDD_I2C_ADDRESS); 00145 } 00146 } 00147 00148 /** 00149 * @brief Reset Idd measurement component. 00150 * @retval None 00151 */ 00152 void BSP_IDD_Reset(void) 00153 { 00154 if(IddDrv->Reset != NULL) 00155 { 00156 IddDrv->Reset(IDD_I2C_ADDRESS); 00157 } 00158 } 00159 00160 /** 00161 * @brief Turn Idd measurement component in low power (standby/sleep) mode 00162 * @retval None 00163 */ 00164 void BSP_IDD_LowPower(void) 00165 { 00166 if(IddDrv->LowPower != NULL) 00167 { 00168 IddDrv->LowPower(IDD_I2C_ADDRESS); 00169 } 00170 } 00171 00172 /** 00173 * @brief Start Measurement campaign 00174 * @retval None 00175 */ 00176 void BSP_IDD_StartMeasure(void) 00177 { 00178 00179 /* Activate the OPAMP used ny the MFX to measure the current consumption */ 00180 BSP_IO_ConfigPin(IDD_AMP_CONTROL_PIN, IO_MODE_OUTPUT); 00181 BSP_IO_WritePin(IDD_AMP_CONTROL_PIN, GPIO_PIN_RESET); 00182 00183 if(IddDrv->Start != NULL) 00184 { 00185 IddDrv->Start(IDD_I2C_ADDRESS); 00186 } 00187 } 00188 00189 /** 00190 * @brief Configure Idd component 00191 * @param IddConfig: structure of idd parameters 00192 * @retval None 00193 */ 00194 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig) 00195 { 00196 if(IddDrv->Config != NULL) 00197 { 00198 IddDrv->Config(IDD_I2C_ADDRESS, IddConfig); 00199 } 00200 } 00201 00202 /** 00203 * @brief Get Idd current value. 00204 * @param IddValue: Pointer on u32 to store Idd. Value unit is 10 nA. 00205 * @retval None 00206 */ 00207 void BSP_IDD_GetValue(uint32_t *IddValue) 00208 { 00209 /* De-activate the OPAMP used ny the MFX to measure the current consumption */ 00210 BSP_IO_ConfigPin(IDD_AMP_CONTROL_PIN, IO_MODE_INPUT); 00211 00212 if(IddDrv->GetValue != NULL) 00213 { 00214 IddDrv->GetValue(IDD_I2C_ADDRESS, IddValue); 00215 } 00216 } 00217 00218 /** 00219 * @brief Enable Idd interrupt that warn end of measurement 00220 * @retval None 00221 */ 00222 void BSP_IDD_EnableIT(void) 00223 { 00224 if(IddDrv->EnableIT != NULL) 00225 { 00226 IddDrv->EnableIT(IDD_I2C_ADDRESS); 00227 } 00228 } 00229 00230 /** 00231 * @brief Clear Idd interrupt that warn end of measurement 00232 * @retval None 00233 */ 00234 void BSP_IDD_ClearIT(void) 00235 { 00236 if(IddDrv->ClearIT != NULL) 00237 { 00238 IddDrv->ClearIT(IDD_I2C_ADDRESS); 00239 } 00240 } 00241 00242 /** 00243 * @brief Get Idd interrupt status 00244 * @retval status 00245 */ 00246 uint8_t BSP_IDD_GetITStatus(void) 00247 { 00248 if(IddDrv->GetITStatus != NULL) 00249 { 00250 return (IddDrv->GetITStatus(IDD_I2C_ADDRESS)); 00251 } 00252 else 00253 { 00254 return IDD_ERROR; 00255 } 00256 } 00257 00258 /** 00259 * @brief Disable Idd interrupt that warn end of measurement 00260 * @retval None 00261 */ 00262 void BSP_IDD_DisableIT(void) 00263 { 00264 if(IddDrv->DisableIT != NULL) 00265 { 00266 IddDrv->DisableIT(IDD_I2C_ADDRESS); 00267 } 00268 } 00269 00270 /** 00271 * @brief Get Error Code . 00272 * @retval Error code or error status 00273 */ 00274 uint8_t BSP_IDD_ErrorGetCode(void) 00275 { 00276 if(IddDrv->ErrorGetSrc != NULL) 00277 { 00278 if((IddDrv->ErrorGetSrc(IDD_I2C_ADDRESS) & MFXSTM32L152_IDD_ERROR_SRC) != RESET) 00279 { 00280 if(IddDrv->ErrorGetCode != NULL) 00281 { 00282 return IddDrv->ErrorGetCode(IDD_I2C_ADDRESS); 00283 } 00284 else 00285 { 00286 return IDD_ERROR; 00287 } 00288 } 00289 else 00290 { 00291 return IDD_ERROR; 00292 } 00293 } 00294 else 00295 { 00296 return IDD_ERROR; 00297 } 00298 } 00299 00300 00301 /** 00302 * @brief Enable error interrupt that warn end of measurement 00303 * @retval None 00304 */ 00305 void BSP_IDD_ErrorEnableIT(void) 00306 { 00307 if(IddDrv->ErrorEnableIT != NULL) 00308 { 00309 IddDrv->ErrorEnableIT(IDD_I2C_ADDRESS); 00310 } 00311 } 00312 00313 /** 00314 * @brief Clear Error interrupt that warn end of measurement 00315 * @retval None 00316 */ 00317 void BSP_IDD_ErrorClearIT(void) 00318 { 00319 if(IddDrv->ErrorClearIT != NULL) 00320 { 00321 IddDrv->ErrorClearIT(IDD_I2C_ADDRESS); 00322 } 00323 } 00324 00325 /** 00326 * @brief Get Error interrupt status 00327 * @retval Status 00328 */ 00329 uint8_t BSP_IDD_ErrorGetITStatus(void) 00330 { 00331 if(IddDrv->ErrorGetITStatus != NULL) 00332 { 00333 return (IddDrv->ErrorGetITStatus(IDD_I2C_ADDRESS)); 00334 } 00335 else 00336 { 00337 return 0; 00338 } 00339 } 00340 00341 /** 00342 * @brief Disable Error interrupt 00343 * @retval None 00344 */ 00345 void BSP_IDD_ErrorDisableIT(void) 00346 { 00347 if(IddDrv->ErrorDisableIT != NULL) 00348 { 00349 IddDrv->ErrorDisableIT(IDD_I2C_ADDRESS); 00350 } 00351 } 00352 00353 /** 00354 * @brief Wake up Idd measurement component. 00355 * @retval None 00356 */ 00357 void BSP_IDD_WakeUp(void) 00358 { 00359 if(IddDrv->WakeUp != NULL) 00360 { 00361 IddDrv->WakeUp(IDD_I2C_ADDRESS); 00362 } 00363 } 00364 00365 /** 00366 * @} 00367 */ 00368 00369 /** 00370 * @} 00371 */ 00372 00373 /** 00374 * @} 00375 */ 00376 00377 /** 00378 * @} 00379 */ 00380 00381 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00382
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
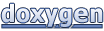